Add Background Color In Js Lwc
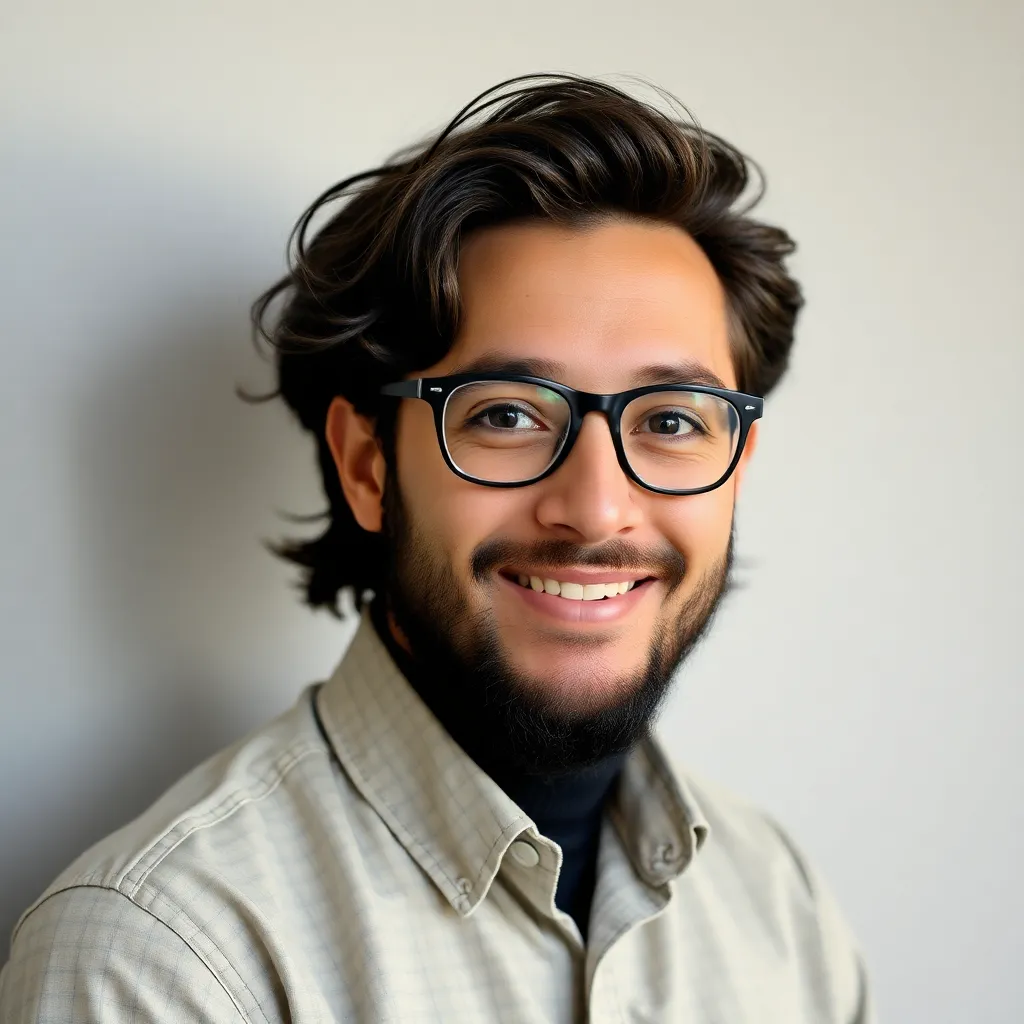
Kalali
May 24, 2025 · 3 min read
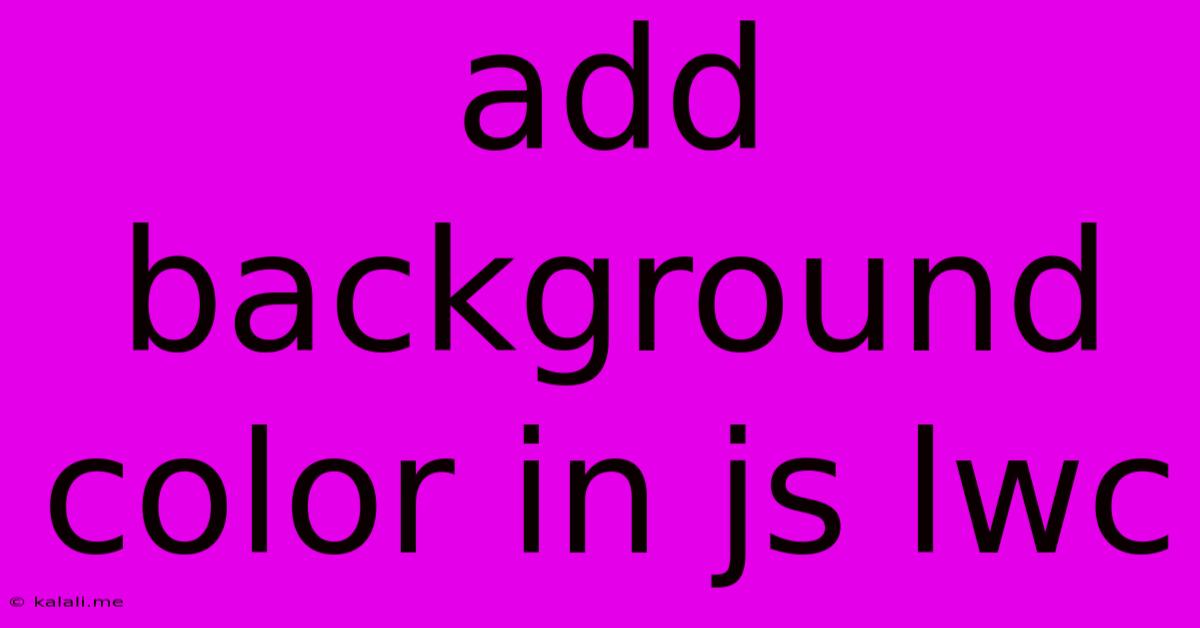
Table of Contents
Adding Background Color in JS LWC: A Comprehensive Guide
Adding background color to your Lightning Web Components (LWC) can significantly enhance the visual appeal and user experience. This guide provides a comprehensive approach, covering various methods and best practices to achieve this effectively. This article covers using JavaScript within your LWC to dynamically control background colors, ensuring your components are both visually appealing and performant.
Understanding the Methods
There are several ways to add background color in your LWC's JavaScript: directly manipulating the DOM element's style attribute, using CSS custom properties (variables), and leveraging CSS classes. Each method has its advantages and disadvantages, and the best choice depends on your specific needs and project structure.
Method 1: Direct DOM Manipulation
This approach involves directly accessing the DOM element and modifying its style
attribute. While simple for small, isolated tasks, it's generally less preferred for larger applications due to its lack of maintainability and potential conflicts with other styling mechanisms.
import { LightningElement, api } from 'lwc';
export default class MyComponent extends LightningElement {
@api backgroundColor = 'lightblue';
renderedCallback() {
const element = this.template.querySelector('.my-element');
if (element) {
element.style.backgroundColor = this.backgroundColor;
}
}
}
This element's background color is dynamically set.
Caveats: This method directly modifies the DOM, making it less flexible for complex styling scenarios. Changes to the backgroundColor
property won't automatically update the style unless renderedCallback
is used.
Method 2: Using CSS Custom Properties (Variables)
This approach uses CSS variables (--my-background-color
) within your component's CSS file. This offers better separation of concerns and is generally the recommended method for dynamic styling.
import { LightningElement, api } from 'lwc';
export default class MyComponent extends LightningElement {
@api backgroundColor = 'lightblue';
}
:host {
--my-background-color: lightblue;
}
.my-element {
background-color: var(--my-background-color);
}
This element's background color is set using a CSS variable.
Advantages: Clean separation of concerns, improved maintainability, easier to update and manage styles across your component. Changes in the backgroundColor
property will automatically propagate to the CSS variable.
Method 3: Toggling CSS Classes
This method involves adding or removing CSS classes based on component state. It's particularly useful for managing multiple background colors or visual states.
import { LightningElement, api } from 'lwc';
export default class MyComponent extends LightningElement {
@api backgroundColor = 'lightblue';
get classList() {
return this.backgroundColor === 'lightblue' ? 'lightblue-background' : 'darkblue-background';
}
}
.lightblue-background {
background-color: lightblue;
}
.darkblue-background {
background-color: darkblue;
}
This element's background color is controlled by CSS classes.
Advantages: Highly maintainable and scalable for managing complex states and visual changes. Offers excellent separation of concerns between JavaScript logic and CSS styles.
Best Practices
- Use CSS Custom Properties: Prioritize using CSS custom properties (variables) for better maintainability and separation of concerns.
- Avoid Inline Styles: Directly manipulating
style
attributes should be avoided except in very specific, limited cases. - Leverage CSS Classes: For managing multiple visual states or background colors, using CSS classes is the most effective and organized approach.
- Consider Accessibility: Ensure sufficient color contrast for accessibility compliance.
By employing these methods and adhering to best practices, you can effectively add background color to your LWCs, creating visually appealing and well-structured components. Remember to choose the approach that best suits your project's complexity and maintainability requirements.
Latest Posts
Latest Posts
-
Why Is My Cold Water Warm
May 24, 2025
-
Startupx To Start Linux Shell From Tty
May 24, 2025
-
How To Copy A Block In Minecraft
May 24, 2025
-
What Is The Word For Start Of The Problem
May 24, 2025
-
How To Gunzip All Files In A Directory
May 24, 2025
Related Post
Thank you for visiting our website which covers about Add Background Color In Js Lwc . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.