Add Style Elements In Js Lwc
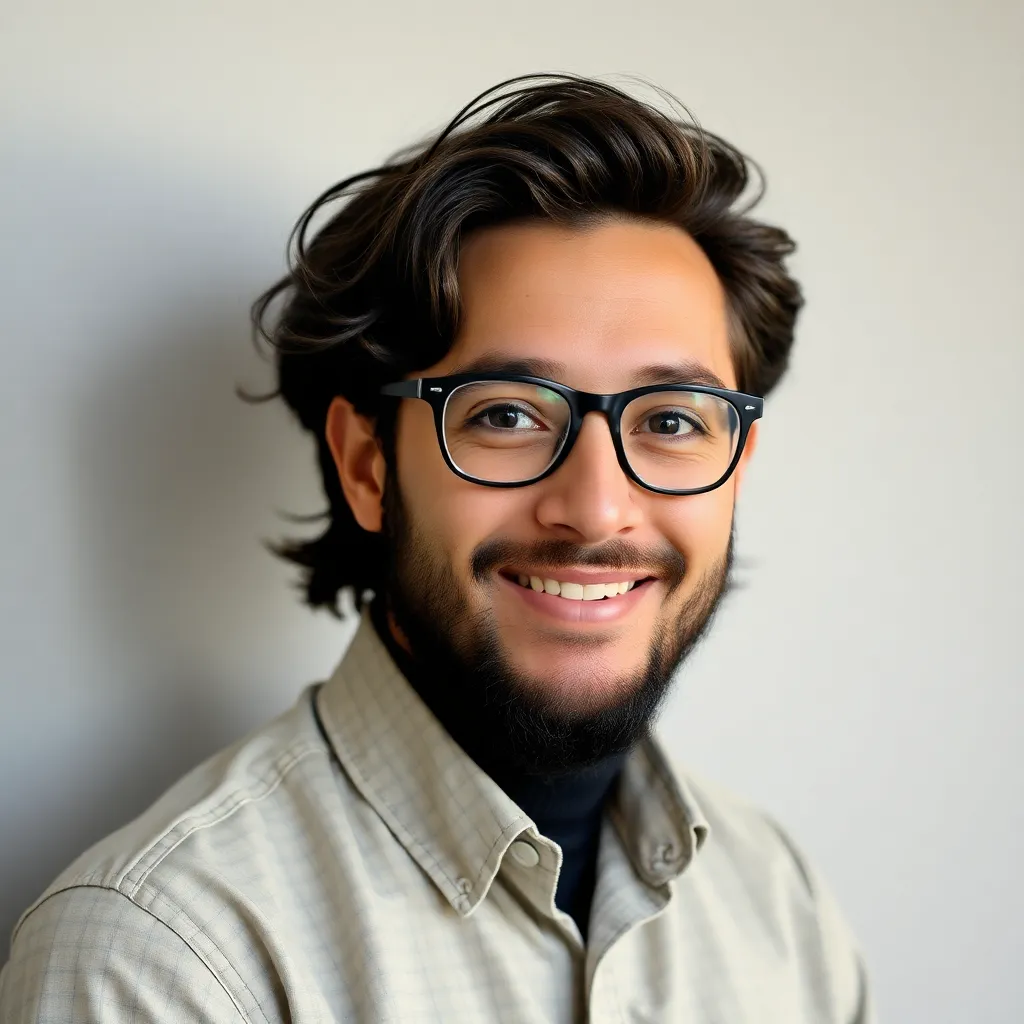
Kalali
May 23, 2025 · 3 min read
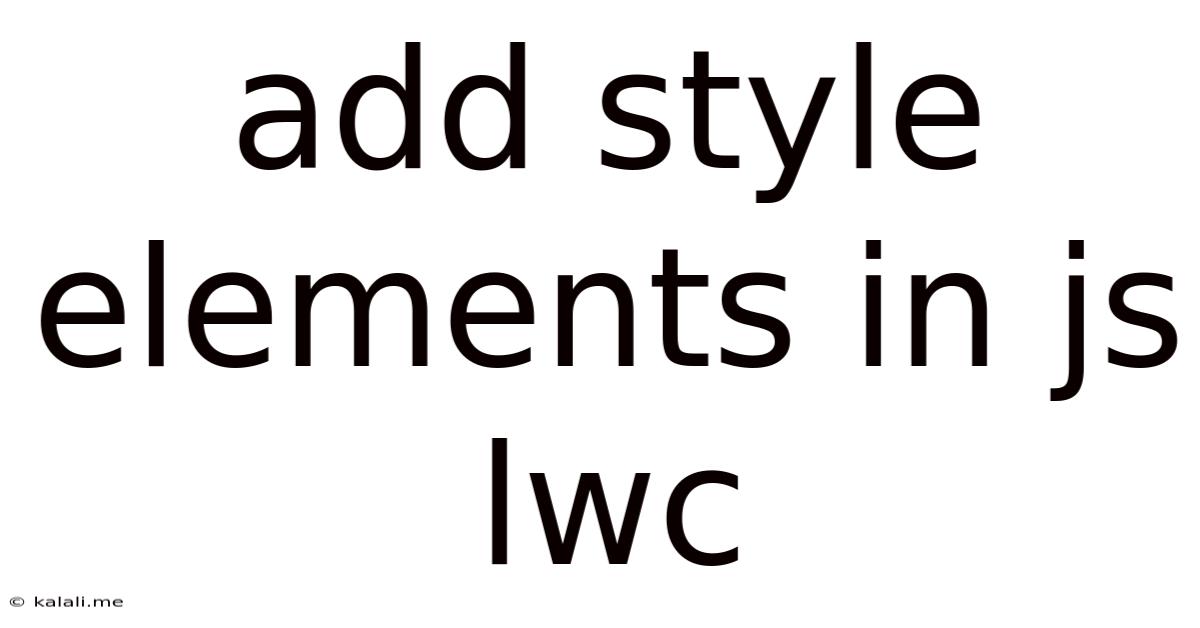
Table of Contents
Adding Style Elements in JS LWC: A Comprehensive Guide
This article provides a comprehensive guide on how to effectively add style elements to your Lightning Web Components (LWC) using JavaScript. We'll explore various techniques, best practices, and considerations for maintaining a clean and maintainable codebase. Understanding these methods will allow you to create visually appealing and functional LWC components.
Adding styles to your LWCs is crucial for creating a visually appealing user interface. While CSS is the primary method, sometimes you need to dynamically manipulate styles using JavaScript. This is particularly useful when you need to react to user interactions or data changes.
Method 1: Using template.querySelector
and style.setProperty
This is a common approach for directly manipulating the style of a specific element within your LWC template. You select the element using template.querySelector
and then modify its style properties using style.setProperty
.
import { LightningElement } from 'lwc';
export default class MyComponent extends LightningElement {
handleClick() {
const myElement = this.template.querySelector('.my-element');
if (myElement) {
myElement.style.setProperty('--my-color', 'red');
myElement.style.setProperty('font-size', '24px');
}
}
}
In your LWC template (myComponent.html
):
This text will change style on click.
This example changes the text color and font size of the element with the class my-element
when the button is clicked. Remember to use CSS variables (custom properties) whenever possible for better maintainability and reusability.
Method 2: Using CSS Variables (Custom Properties)
CSS variables offer a more elegant and maintainable way to manage styles dynamically. You define variables in your CSS and then update them using JavaScript. This approach promotes separation of concerns and keeps your styling cleaner.
import { LightningElement } from 'lwc';
export default class MyComponent extends LightningElement {
connectedCallback() {
this.template.querySelector(':host').style.setProperty('--my-bg-color', 'lightblue');
}
}
In your LWC CSS (myComponent.css
):
:host {
background-color: var(--my-bg-color, white); /* Default to white if variable is not set */
padding: 20px;
}
This example sets the background color of the component using a CSS variable. The connectedCallback
lifecycle hook is used to set the variable's value when the component is initialized. This approach is preferred as it keeps styling separate from javascript logic.
Method 3: Toggling CSS Classes
This method involves adding or removing CSS classes from an element to apply different styles. This is particularly useful when you have pre-defined styles in your CSS.
import { LightningElement } from 'lwc';
export default class MyComponent extends LightningElement {
isActive = false;
handleClick() {
this.isActive = !this.isActive;
}
}
In your LWC template (myComponent.html
):
This text will change style on click.
In your LWC CSS (myComponent.css
):
.active {
color: green;
font-weight: bold;
}
This example toggles the active
class, changing the text color and font weight when the button is clicked. This offers a clean separation between styling and logic.
Best Practices
- Use CSS whenever possible: JavaScript should be used for dynamic styling only when necessary. Static styles should always be defined in your CSS.
- Utilize CSS variables: They enhance maintainability and readability of your styles.
- Keep your JavaScript concise: Avoid over-complicating your JavaScript code for styling.
- Follow a consistent naming convention: This helps improve code readability and maintainability.
- Test your styles thoroughly: Ensure your styles work correctly across different browsers and devices.
By understanding and applying these methods and best practices, you can effectively add style elements to your LWCs, creating visually appealing and user-friendly components. Remember to prioritize a clean separation of concerns and maintainable code for long-term success.
Latest Posts
Latest Posts
-
How Many Tablespoons Are In A Hidden Valley Ranch Packet
Jul 10, 2025
-
Which Is The Best Summary Of The Passage
Jul 10, 2025
-
How Many Quarts Of Soil In A Cubic Foot
Jul 10, 2025
-
What Is 3 4 Of A Pound
Jul 10, 2025
-
How To Measure 1 8 Teaspoon With 1 4 Teaspoon
Jul 10, 2025
Related Post
Thank you for visiting our website which covers about Add Style Elements In Js Lwc . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.