Apex Action To Remove Permission Set Assignment
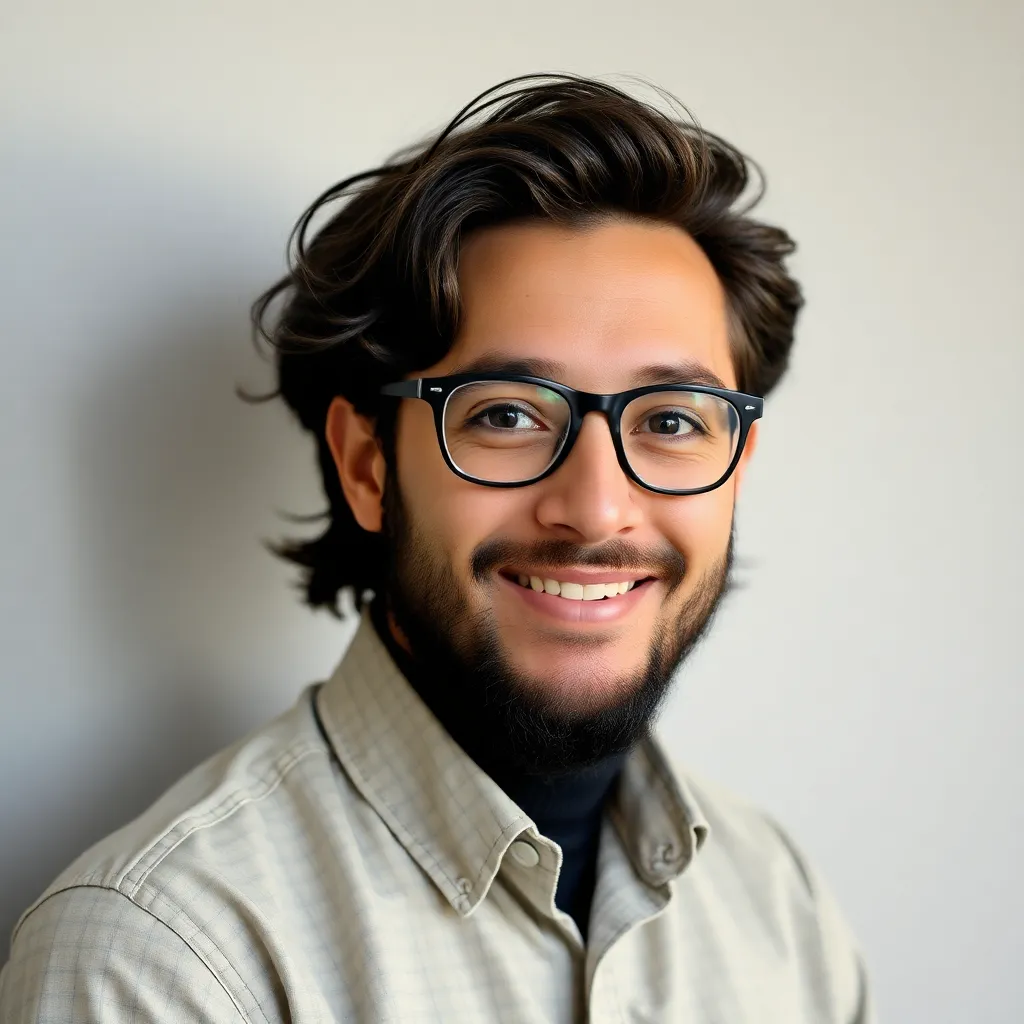
Kalali
May 23, 2025 · 3 min read
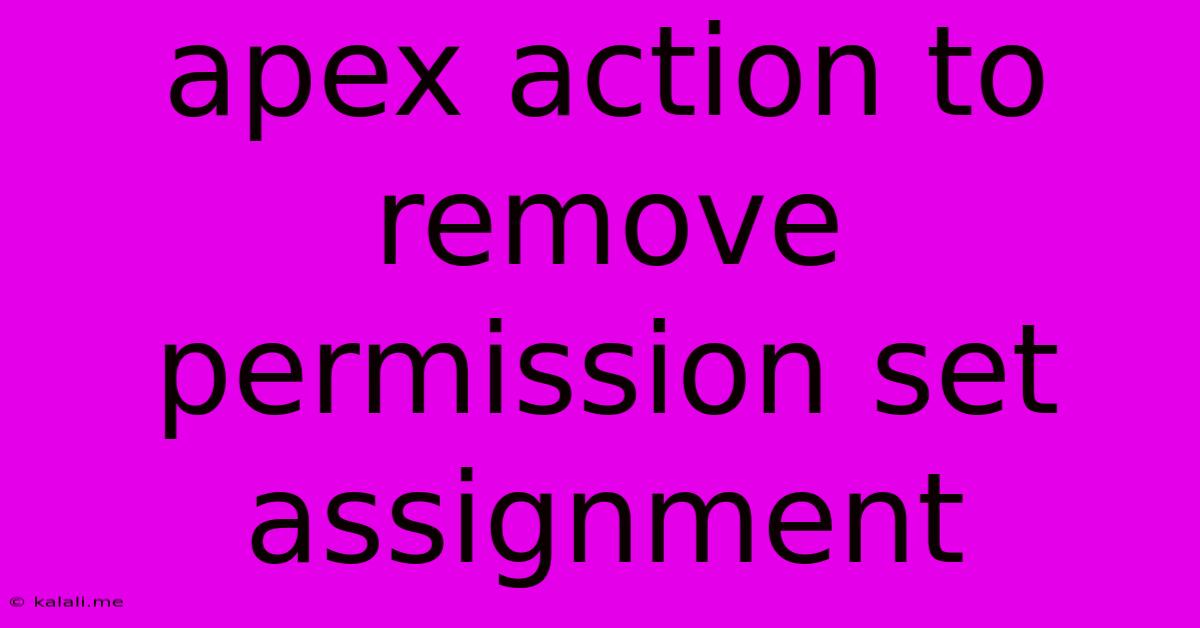
Table of Contents
Apex Action to Remove Permission Set Assignment: A Comprehensive Guide
Removing permission set assignments via Apex is a crucial task for Salesforce administrators and developers needing programmatic control over user access. This guide provides a comprehensive understanding of how to efficiently and effectively remove permission set assignments using Apex, including best practices and troubleshooting tips. This article covers removing assignments from individual users, multiple users, and even handling potential errors.
Understanding Permission Set Assignments
Before diving into the Apex code, it's important to grasp the concept of permission set assignments in Salesforce. Permission sets grant users specific permissions and functionalities within the Salesforce platform. These assignments can be managed manually through the Salesforce user interface or programmatically using Apex. Removing an assignment effectively revokes the associated permissions for a specific user.
Apex Code for Removing Permission Set Assignments
The core of this operation revolves around using the Database.delete()
method on PermissionSetAssignment
records. Here's how you can achieve this for a single user:
// Get the User ID
Id userId = UserInfo.getUserId(); // Or specify a different user ID
// Get the Permission Set ID
Id permissionSetId = [SELECT Id FROM PermissionSet WHERE Name = 'YourPermissionSetName'].Id;
// Query for the Permission Set Assignment
PermissionSetAssignment psa = [SELECT Id FROM PermissionSetAssignment WHERE AssigneeId = :userId AND PermissionSetId = :permissionSetId];
// Delete the Assignment
if (psa != null) {
delete psa;
System.debug('Permission set assignment deleted successfully.');
} else {
System.debug('Permission set assignment not found.');
}
Replace 'YourPermissionSetName'
with the actual name of your permission set. This code snippet first retrieves the relevant PermissionSetAssignment
record and then deletes it if found. Error handling is included to gracefully handle cases where the assignment doesn't exist.
Removing Assignments from Multiple Users
For removing assignments from multiple users, leverage SOQL queries and loops:
List userIds = new List();
// Add user IDs to the list
Id permissionSetId = [SELECT Id FROM PermissionSet WHERE Name = 'YourPermissionSetName'].Id;
List psasToDelete = [SELECT Id FROM PermissionSetAssignment WHERE AssigneeId IN :userIds AND PermissionSetId = :permissionSetId];
delete psasToDelete;
System.debug(psasToDelete.size() + ' permission set assignments deleted successfully.');
This approach is significantly more efficient than iterating through users individually, especially when dealing with a large number of users.
Best Practices for Apex Permission Set Management
- Error Handling: Always include robust error handling to catch potential exceptions, like
DMLException
. - Bulkification: Process records in batches (using
Database.insert
,Database.update
, andDatabase.delete
methods within loops) to improve performance, especially when dealing with large datasets. - Testing: Thoroughly test your Apex code in a sandbox environment before deploying it to production.
- Logging: Use
System.debug()
statements strategically to track the progress and identify potential issues during execution. - Security Considerations: Ensure your Apex code adheres to Salesforce security best practices. Avoid hardcoding sensitive information, such as user IDs and permission set IDs, directly into the code. Instead, use configuration or custom settings.
Troubleshooting Common Issues
- Permission Set Not Found: Double-check the permission set name in your SOQL query. Case sensitivity matters.
- Assignment Not Found: Verify that the user actually has the permission set assigned.
- DML Exceptions: Carefully examine the
DMLException
details to understand the cause of the error. Common causes include insufficient permissions or data integrity issues.
By following these guidelines and incorporating best practices, you can efficiently and reliably manage permission set assignments within your Salesforce organization using Apex, enhancing security and control over user access. Remember to adapt the code to your specific requirements and always thoroughly test before deploying to a production environment.
Latest Posts
Latest Posts
-
How Far From The Wall Is A Toilet Flange
May 23, 2025
-
How Long Did It Take To Copy A Book
May 23, 2025
-
Integration Of 1 X 4 1
May 23, 2025
-
Why Do Basketball Players Wipe Their Shoes
May 23, 2025
-
Probability Distribution Of X And X N
May 23, 2025
Related Post
Thank you for visiting our website which covers about Apex Action To Remove Permission Set Assignment . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.