Arithmetic Overflow Error Converting Expression To Data Type Int.
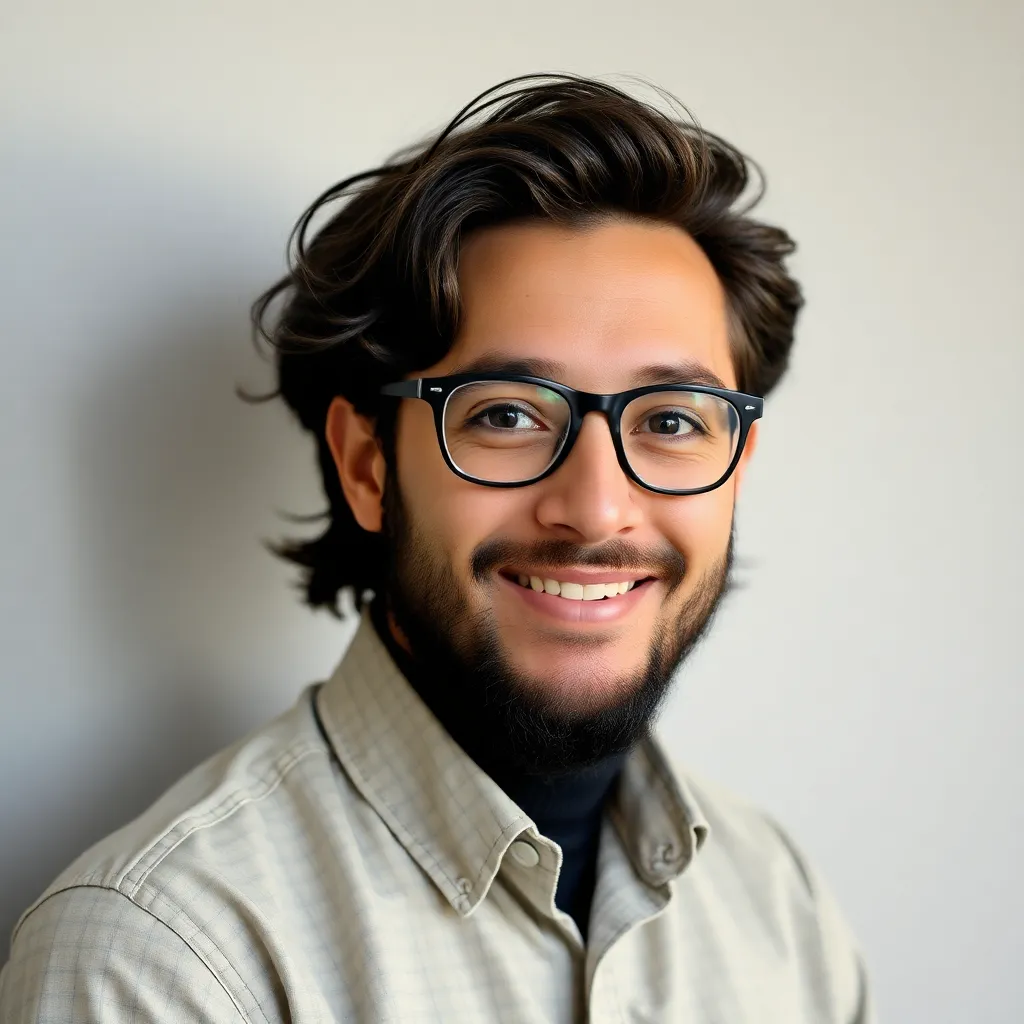
Kalali
May 20, 2025 · 3 min read
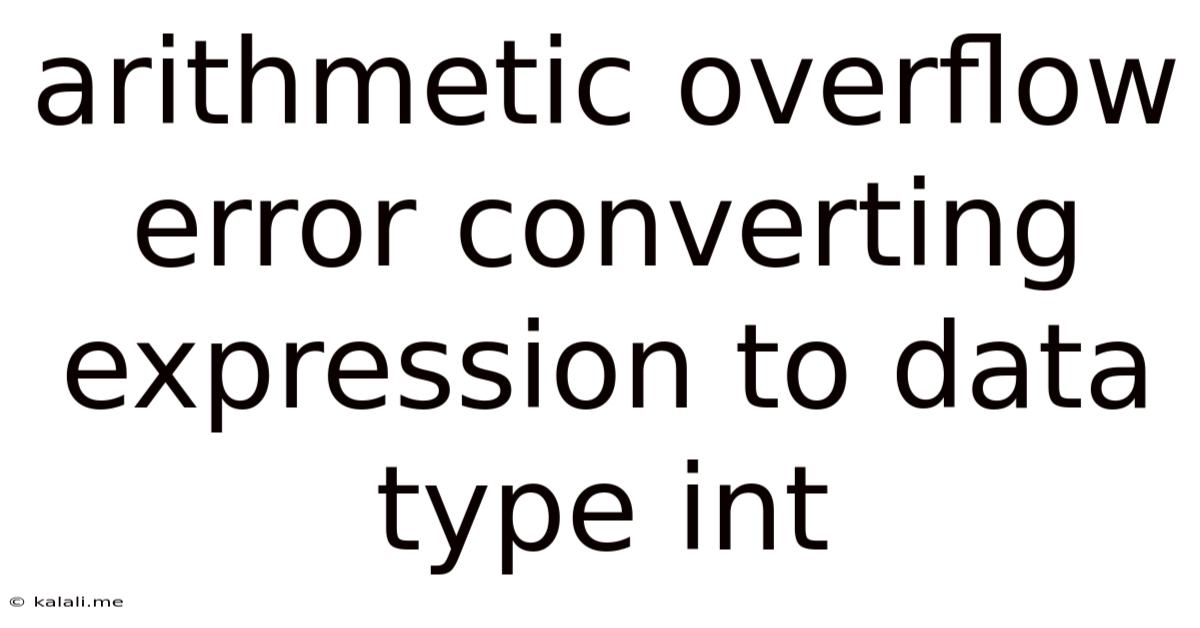
Table of Contents
Arithmetic Overflow Error Converting Expression to Data Type INT: A Comprehensive Guide
The dreaded "Arithmetic overflow error converting expression to data type int" is a common problem in programming, particularly when dealing with integer variables and calculations. This error occurs when the result of an arithmetic operation exceeds the maximum value that can be stored in an integer variable. This article will delve into the causes, troubleshooting techniques, and preventative measures for this error. Understanding this error is crucial for writing robust and reliable code.
What Causes Arithmetic Overflow Errors?
The root cause is simple: you're trying to squeeze a number too big into a container that's too small. Integers, in most programming languages, have a defined range. For example, a 32-bit signed integer can hold values from -2,147,483,648 to 2,147,483,647. If your calculation produces a result outside these bounds, an overflow occurs. This is particularly problematic in languages like C++, Java, and C# where integer types are often implicitly defined.
Several scenarios can trigger this error:
- Addition: Adding two large positive integers.
- Multiplication: Multiplying two relatively large integers.
- Subtraction: Subtracting a large negative number from a small positive number.
- Incrementing/Decrementing: Repeatedly incrementing or decrementing an integer that's already near its maximum or minimum value.
- Casting: Converting a larger data type (like a
long
orbigint
) to anint
, where the larger value exceeds theint
's capacity.
Common Programming Scenarios and Examples
Let's illustrate with a few code snippets (using C# for clarity, but the concept applies broadly):
int a = 2147483647; // Maximum value for a 32-bit int
int b = 1;
int c = a + b; // Overflow!
In this example, c
attempts to store a value exceeding the maximum int
value, resulting in an overflow.
Another example involving multiplication:
int x = 100000;
int y = 100000;
int z = x * y; // Overflow!
Here, the product of x
and y
is far larger than the maximum int
value.
How to Troubleshoot and Debug
-
Inspect Your Calculations: Carefully review all arithmetic operations in your code, paying close attention to the potential magnitudes of the involved numbers.
-
Use Larger Data Types: Consider using data types with a wider range, such as
long
(64-bit integer),BigInteger
(arbitrary-precision integer), or evendecimal
if you need decimal precision. This is often the most straightforward solution. -
Check for Intermediate Results: Break down complex expressions into smaller, simpler calculations to identify where the overflow occurs. This helps pinpoint the problematic line of code.
-
Input Validation: If the values involved in the calculations come from user input or external sources, implement strict input validation to ensure they fall within acceptable ranges. Reject or handle values that are too large before they're used in calculations.
-
Use Debugging Tools: Utilize your IDE's debugging capabilities (breakpoints, watch variables) to step through the code and monitor the values of variables at each stage of the calculation.
-
Exception Handling: Implement
try-catch
blocks to gracefully handle theOverflowException
. This prevents your program from crashing abruptly and allows you to take appropriate actions, such as logging the error or displaying an informative message to the user. This is especially important for production-level code.
Preventive Measures and Best Practices
- Code Reviews: Regular code reviews are essential to catch potential overflow errors before they reach production. A fresh pair of eyes can often spot subtle issues easily missed by the original author.
- Unit Testing: Write comprehensive unit tests that cover various scenarios, including edge cases that might lead to overflows.
- Documentation: Clearly document the expected ranges of variables and the potential impact of large values.
By understanding the causes and employing the troubleshooting and preventative measures outlined above, you can effectively mitigate the risk of "Arithmetic overflow error converting expression to data type int" and build more robust and reliable applications. Remember, prevention is always better than cure.
Latest Posts
Latest Posts
-
In My Mind Or On My Mind
May 20, 2025
-
How Long Can Engine Oil Last
May 20, 2025
-
Does Tea Bags Go Out Of Date
May 20, 2025
-
How Long For Fridge To Get Cold
May 20, 2025
-
What Colour Is Power Steering Fluid
May 20, 2025
Related Post
Thank you for visiting our website which covers about Arithmetic Overflow Error Converting Expression To Data Type Int. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.