Bash Check If Variable Is Set
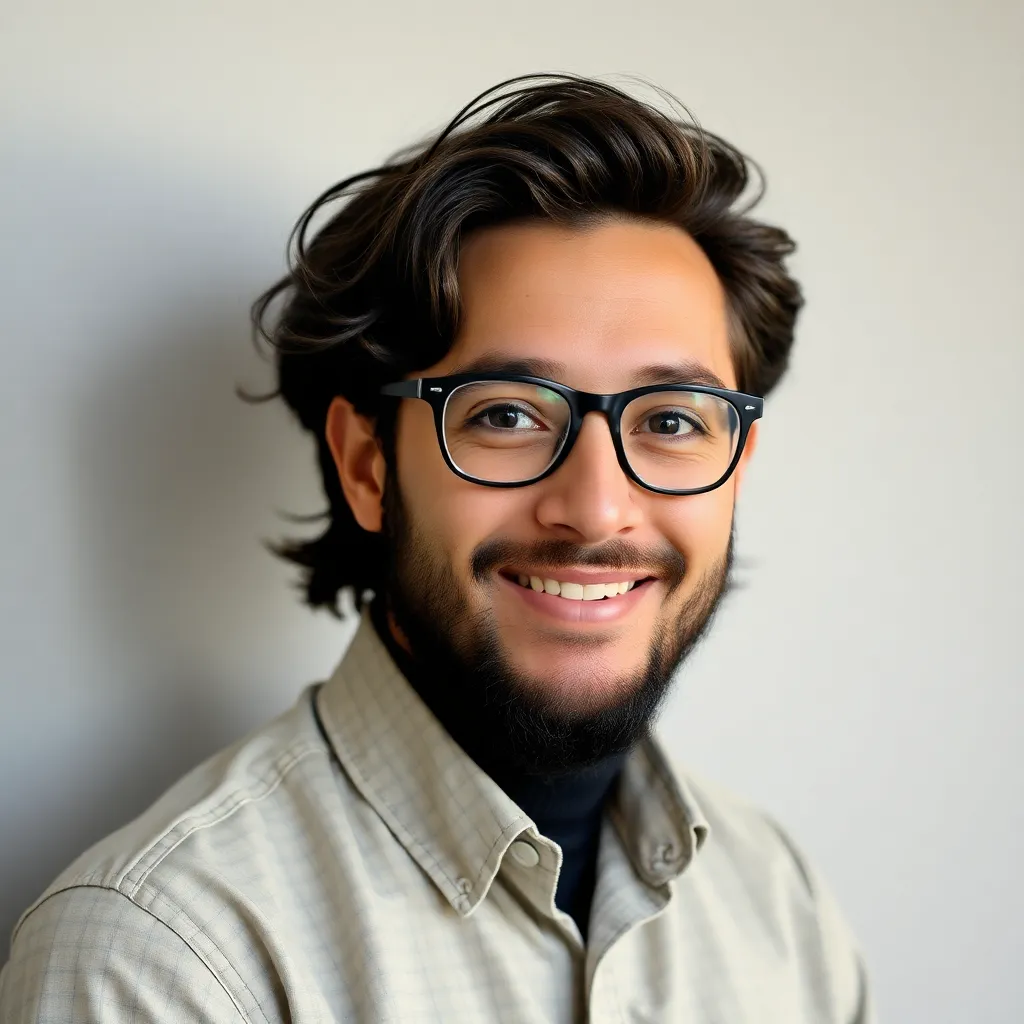
Kalali
May 19, 2025 · 3 min read
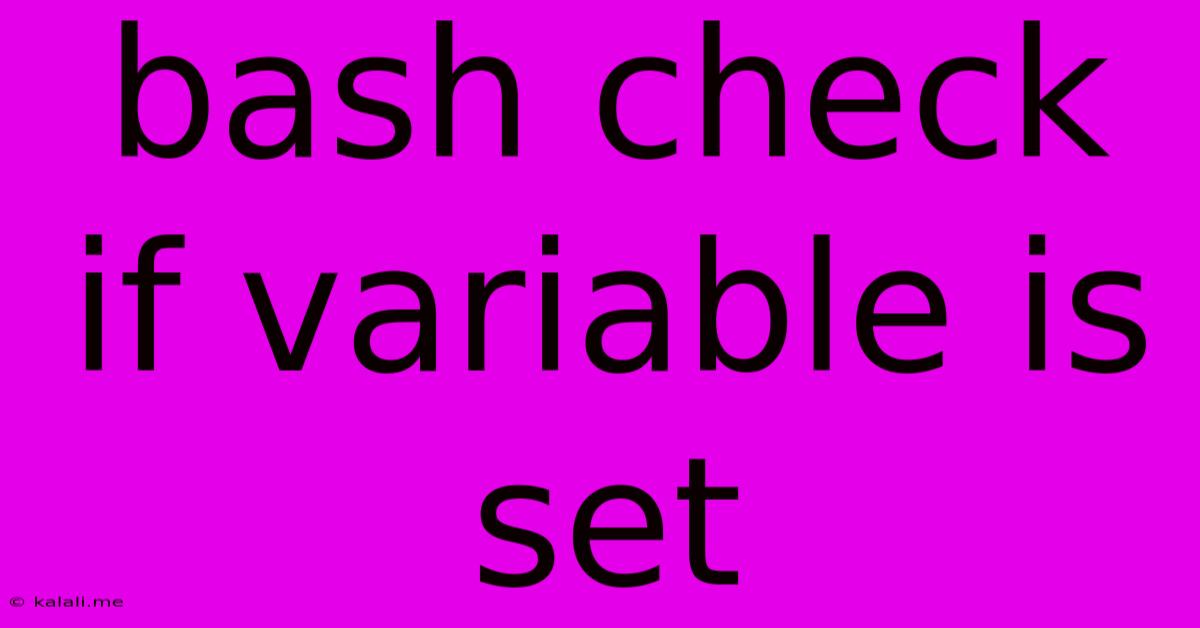
Table of Contents
Bash Check if Variable is Set: A Comprehensive Guide
This guide provides a thorough explanation of how to check if a variable is set in Bash scripting, covering various scenarios and best practices. Knowing whether a variable holds a value is crucial for robust and reliable scripts. Incorrectly handling unset variables can lead to unexpected errors and crashes. This article will equip you with the knowledge to avoid such pitfalls.
Why is checking for set variables important? Unhandled unset variables can cause your Bash scripts to fail unexpectedly. Checking ensures your script gracefully handles situations where a variable might not have been assigned a value, leading to more stable and robust code.
Method 1: Using the -v
operator
The most straightforward and recommended way to check if a variable is set is using the -v
operator within a conditional statement. This operator returns a true value if the variable is set and has a non-null value.
if [[ -v my_variable ]]; then
echo "Variable 'my_variable' is set."
else
echo "Variable 'my_variable' is not set."
fi
This snippet checks if my_variable
is defined. The double square brackets [[ ]]
are crucial for proper evaluation of the -v
operator. Single brackets [ ]
(or test
) will not work correctly.
Method 2: Using Parameter Expansion with :-
Parameter expansion provides a more concise way to check for a set variable and optionally assign a default value if it's not set. The :-
operator expands to the variable's value if it's set; otherwise, it expands to a default value.
my_variable="${my_variable:-'Default Value'}"
echo "The value of my_variable is: $my_variable"
This code snippet assigns 'Default Value' to my_variable
if it's not already set. This avoids errors that might occur if you try to use an unset variable later in the script. This is particularly useful for handling optional command-line arguments or configuration settings.
Method 3: Using Parameter Expansion with :+
The :+
operator is similar to :-
, but it expands to the variable's value only if it's set and non-empty. This is useful when you want to distinguish between a variable that's explicitly set to an empty string and one that's completely unset.
my_variable="${my_variable:+Existing Value}"
echo "The value of my_variable is: $my_variable"
In this case, if my_variable
is set to an empty string (""
), my_variable
will remain unchanged. Only if it's set to a non-empty string will it be replaced by 'Existing Value'.
Method 4: Checking for Null Values
Sometimes, you need to differentiate between a variable that's set to an empty string and a variable that's completely unset. The -z
operator checks for an empty string, while -v
only checks if the variable exists. Combine these for comprehensive checks.
if [[ -v my_variable && -z "$my_variable" ]]; then
echo "Variable 'my_variable' is set but empty."
elif [[ -v my_variable ]]; then
echo "Variable 'my_variable' is set and has a value."
else
echo "Variable 'my_variable' is not set."
fi
This example thoroughly examines my_variable
's status, accounting for all possibilities: unset, set and empty, and set with a value.
Best Practices for Handling Unset Variables
- Always check: Develop a habit of checking for unset variables before using them. This preventative measure saves countless debugging headaches.
- Default values: Assign default values when appropriate using parameter expansion (
:-
or:+
). This ensures your script operates predictably even if variables are not explicitly set. - Clear error handling: If an unset variable represents a critical error, handle it appropriately. Use
exit
with a non-zero status code to signal failure. - Informative error messages: When a variable is unset and this is an error condition, provide users with clear error messages explaining what went wrong and how to fix it.
By following these methods and best practices, you can write more robust and reliable Bash scripts that gracefully handle various situations, preventing unexpected failures due to unset variables. Remember to choose the method that best suits your specific needs and context.
Latest Posts
Latest Posts
-
Red And Black Wiring Light Switch
May 19, 2025
-
How To Light A Candle Without Lighter
May 19, 2025
-
Rear Sub Frame Corroded But Not Seriously Weakened
May 19, 2025
-
Set Google As My Homepage On Iphone
May 19, 2025
-
1 Litre Of Water Weight In Kg
May 19, 2025
Related Post
Thank you for visiting our website which covers about Bash Check If Variable Is Set . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.