Bash Convert Ordered Pairs To Associative Array
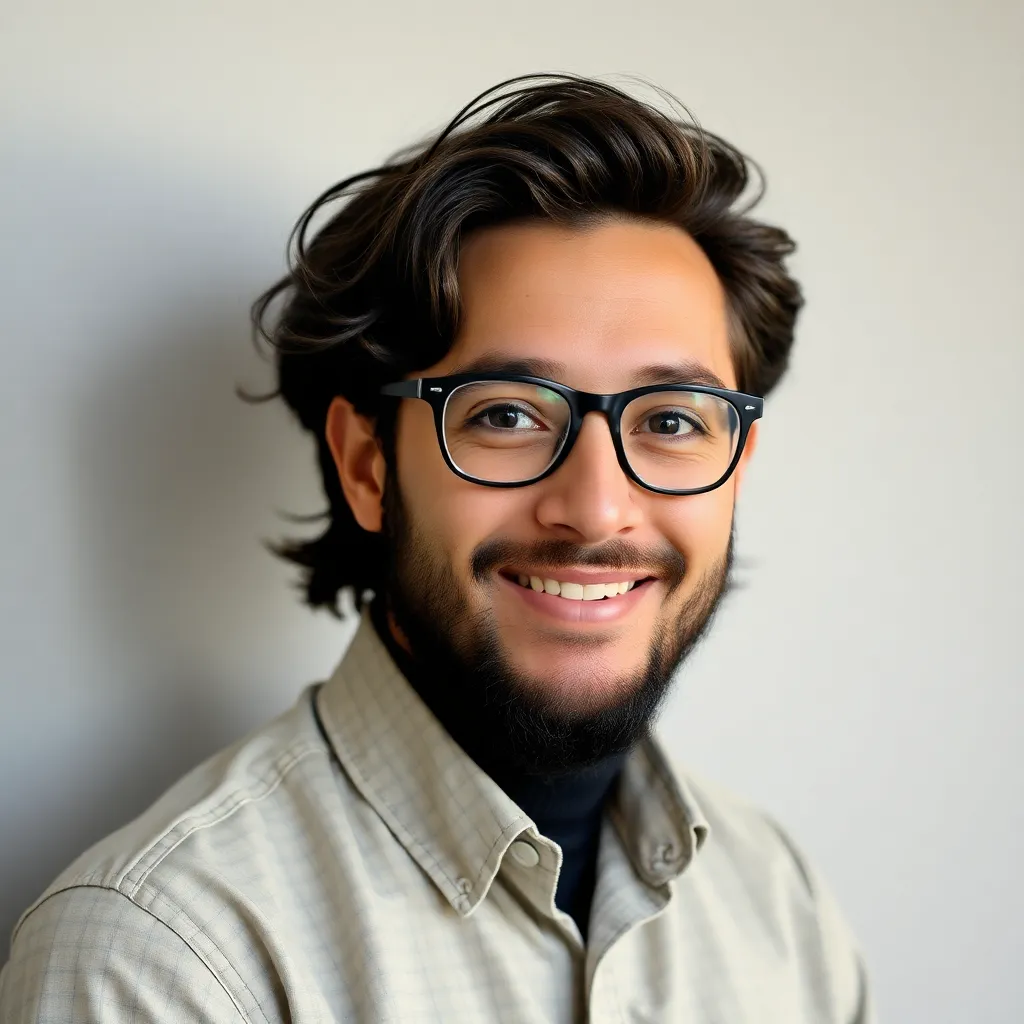
Kalali
May 25, 2025 · 3 min read
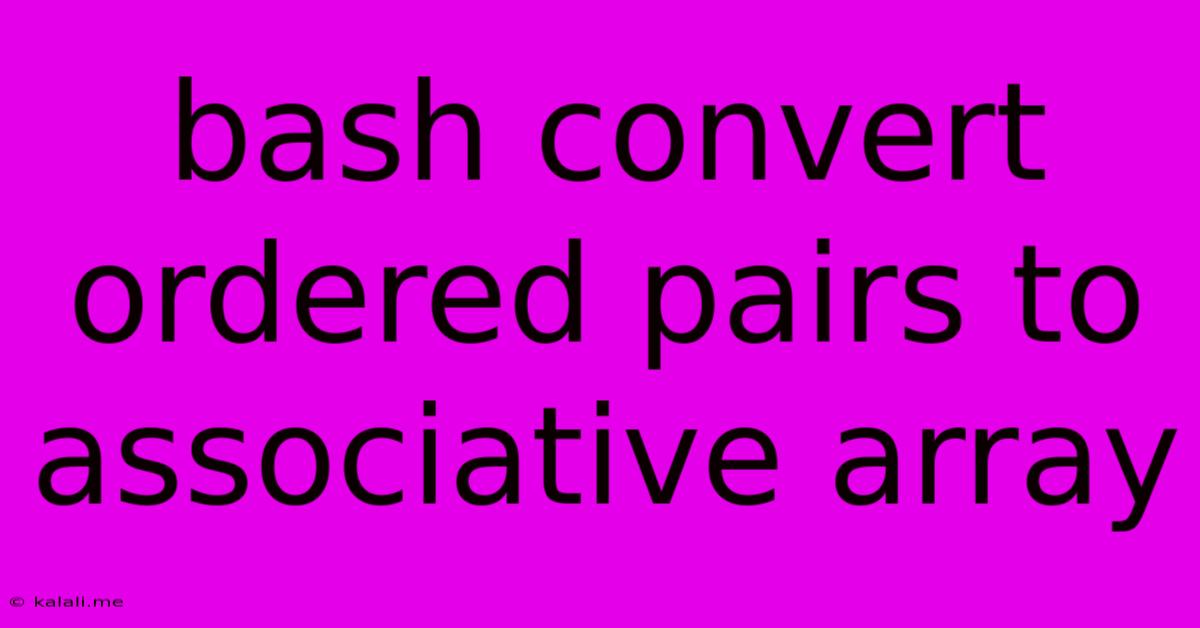
Table of Contents
Bash: Converting Ordered Pairs to an Associative Array
This article details how to efficiently convert a list of ordered pairs in bash into an associative array. This is a common task in scripting when you need to work with key-value pairs from a file or other source. We'll cover several methods, focusing on clarity, efficiency, and best practices for bash scripting. Understanding this will allow you to easily manage data structures in your bash scripts and improve their functionality.
Understanding Associative Arrays in Bash
Before diving into the conversion, let's quickly review associative arrays in bash. Unlike indexed arrays, which use numerical indices, associative arrays utilize string keys to access values. This makes them ideal for representing key-value pairs, like those found in configuration files or data sets. Associative arrays are declared using the declare -A
command.
Method 1: Using a Loop and read
This method is straightforward and easy to understand. We'll assume your ordered pairs are in a format like key=value
, one pair per line, within a variable or file.
ordered_pairs="name=John
age=30
city=New York"
declare -A associative_array
while IFS='=' read -r key value; do
associative_array[$key]=$value
done <<< "$ordered_pairs"
# Accessing values
echo "Name: ${associative_array[name]}"
echo "Age: ${associative_array[age]}"
echo "City: ${associative_array[city]}"
This script uses a while
loop and the read
command to process each line. IFS='='
sets the Internal Field Separator to '=', allowing read
to split each line into key
and value
. The <<<
operator provides the string as input to the loop.
Method 2: Using awk
for more complex scenarios
If your data is more complex, or if it's stored in a file, awk
provides a more robust solution. This is especially useful if your key-value pairs aren't separated by a simple '=' sign.
Let's assume your ordered pairs are in a file named data.txt
with a slightly different format:
Name: John
Age: 30
City: New York
Here's how to use awk
to convert this:
declare -A associative_array
while IFS=':' read -r key value; do
associative_array["$(echo "$key" | tr -d '[:space:]')"]="$(echo "$value" | tr -d '[:space:]')"
done < <(awk -F':' '{print $1":"$2}' data.txt)
# Accessing values
echo "Name: ${associative_array[Name]}"
echo "Age: ${associative_array[Age]}"
echo "City: ${associative_array[City]}"
This uses awk
to split each line using ':' as the field separator and then a while
loop to populate the associative array. The tr
command removes leading/trailing whitespace from the keys and values.
Method 3: Handling potential errors and edge cases
Real-world data is often messy. Let's improve robustness by adding error handling:
ordered_pairs="name=John
age=30
city=New York
invalid_pair"
declare -A associative_array
while IFS='=' read -r key value; do
if [[ -n "$key" && -n "$value" ]]; then # Check for empty keys or values
associative_array[$key]=$value
else
echo "Warning: Invalid pair encountered: $key=$value"
fi
done <<< "$ordered_pairs"
This script adds a check to ensure both key
and value
are not empty, providing more resilient error handling.
Choosing the Right Method
The best method depends on your specific needs:
- Method 1: Ideal for simple, single-line ordered pairs stored in a variable.
- Method 2: Best for complex data formats or when reading from files.
- Method 3: Recommended for production scripts to handle potential errors gracefully.
Remember to tailor these examples to your exact data format and requirements. Proper error handling and efficient parsing are key to creating robust and reliable bash scripts. By mastering these techniques, you can effectively manage key-value data within your bash scripts, leading to cleaner, more maintainable code.
Latest Posts
Latest Posts
-
Mother And I Or Mother And Me
Jul 18, 2025
-
How Many Oz In One Water Bottle
Jul 18, 2025
-
How Many Dimes In A 5 Roll
Jul 18, 2025
-
How Do You Say Basil In Spanish
Jul 18, 2025
-
How Many Cookies Are In A Dozen
Jul 18, 2025
Related Post
Thank you for visiting our website which covers about Bash Convert Ordered Pairs To Associative Array . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.