Bash For Each Line In File
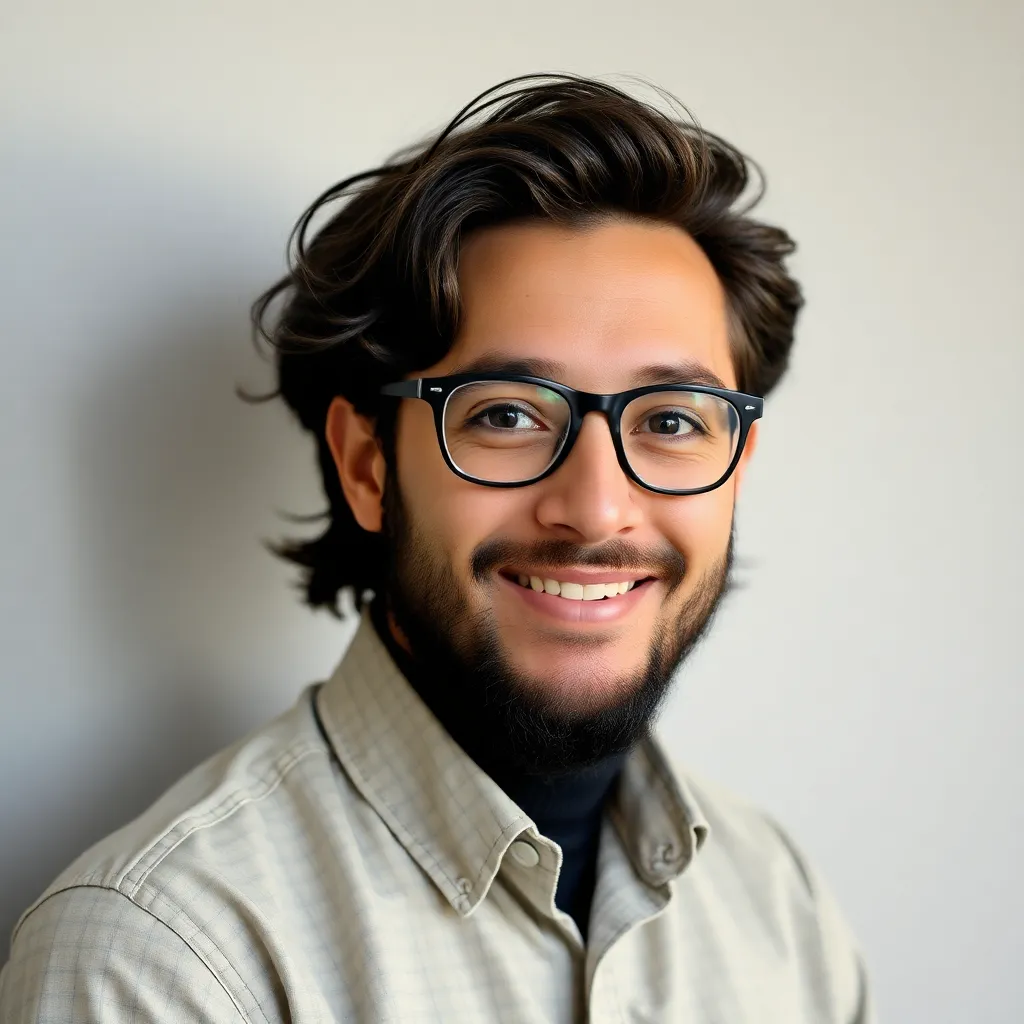
Kalali
May 24, 2025 · 3 min read
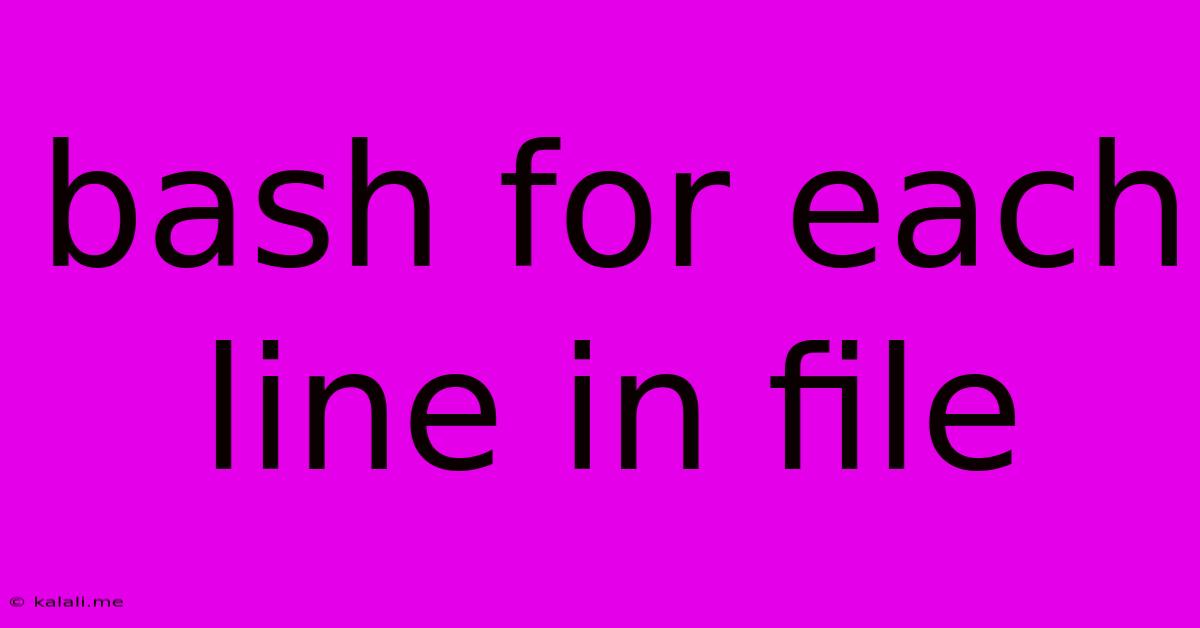
Table of Contents
Bash For Each Line in File: A Comprehensive Guide
This guide provides a comprehensive overview of how to process each line in a file using Bash scripting. Learning this fundamental skill is crucial for automating tasks and managing data efficiently in a Linux or macOS environment. We'll cover various techniques, including while read
, for
loops with input redirection, and the more efficient mapfile
. Understanding these methods will empower you to write powerful and robust Bash scripts.
Why Process Files Line by Line?
Many tasks involving text files require processing each line individually. Examples include:
- Data extraction: Pulling specific information from log files, configuration files, or CSV datasets.
- Data transformation: Converting data formats, cleaning data, or applying transformations to individual entries.
- Data filtering: Selecting lines that match specific criteria.
- File manipulation: Modifying or creating new files based on the content of an existing file.
Let's delve into the different ways to achieve this:
Method 1: The while read
loop
This is a classic and widely used approach. It reads the file line by line, assigning each line to a variable.
while IFS= read -r line; do
# Process each line here
echo "Processing: $line"
done < "my_file.txt"
IFS=
: This sets the Internal Field Separator to the default, preventing unexpected splitting of lines containing whitespace.-r
: This option prevents backslash escapes from being interpreted.line
: This variable holds the content of each line.< "my_file.txt"
: This redirects the contents ofmy_file.txt
to thewhile
loop's standard input.
Example: Extracting specific information
Let's say my_file.txt
contains IP addresses:
192.168.1.1
10.0.0.2
172.16.0.1
We can modify the script to only print IP addresses starting with 192
:
while IFS= read -r line; do
if [[ "$line" == 192.* ]]; then
echo "Found IP: $line"
fi
done < "my_file.txt"
Method 2: for
loop with input redirection
This method is similar to while read
, but uses a for
loop instead. It's often considered slightly less readable for this specific task.
for line in $(cat "my_file.txt"); do
# Process each line here
echo "Processing: $line"
done
Important Note: This approach can lead to issues if lines contain spaces. It's generally recommended to use the while read
method for better robustness.
Method 3: mapfile
(Bash 4.0 and later)
This is a more efficient and modern approach, especially for larger files. mapfile
reads the entire file into an array, allowing for faster access.
mapfile -t lines < "my_file.txt"
for line in "${lines[@]}"; do
# Process each line here
echo "Processing: $line"
done
-t
: This removes trailing newlines from each line.${lines[@]}
: This expands to all elements of thelines
array.
Choosing the Right Method:
- For most cases, especially when dealing with files containing spaces or special characters, the
while read
loop is the most reliable and recommended method. - For very large files where memory is a concern, consider using a more specialized tool like
awk
orsed
for line-by-line processing. - For Bash versions 4.0 and later, and when memory is not a constraint,
mapfile
offers a more efficient approach.
This guide provides a solid foundation for handling line-by-line file processing in Bash. Remember to choose the method that best suits your needs and always prioritize robustness and readability in your scripts. By mastering these techniques, you’ll significantly enhance your Bash scripting capabilities.
Latest Posts
Latest Posts
-
How Long Does It Take To Do An Alignment
May 25, 2025
-
Results Of Convergence Of Empirical Distribution To True Distribution
May 25, 2025
-
Bash Set Variable With Indirect Reference Name
May 25, 2025
-
Best Drill Bit For Stainless Steel
May 25, 2025
-
Chain Of Atoms With Harmoic Coupling
May 25, 2025
Related Post
Thank you for visiting our website which covers about Bash For Each Line In File . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.