Bash For Loop 1 To 10
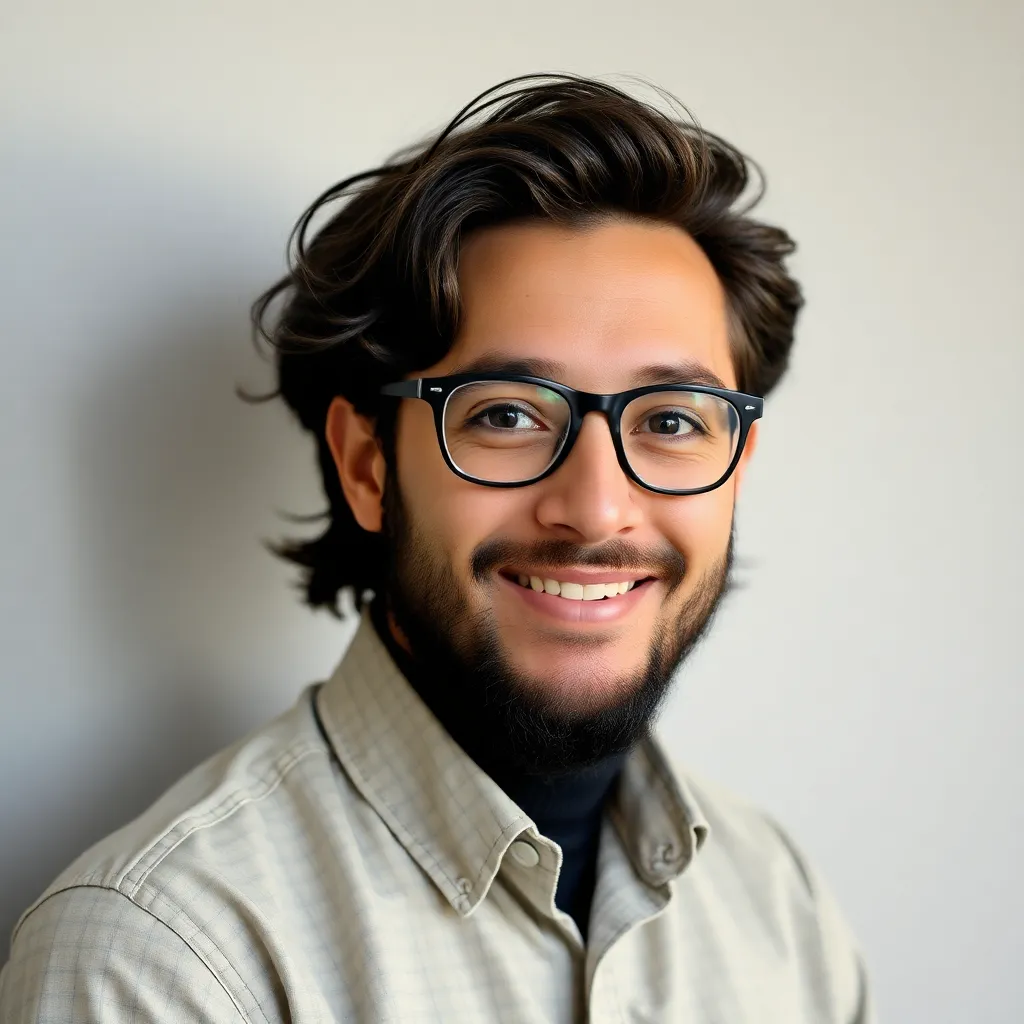
Kalali
May 19, 2025 · 3 min read
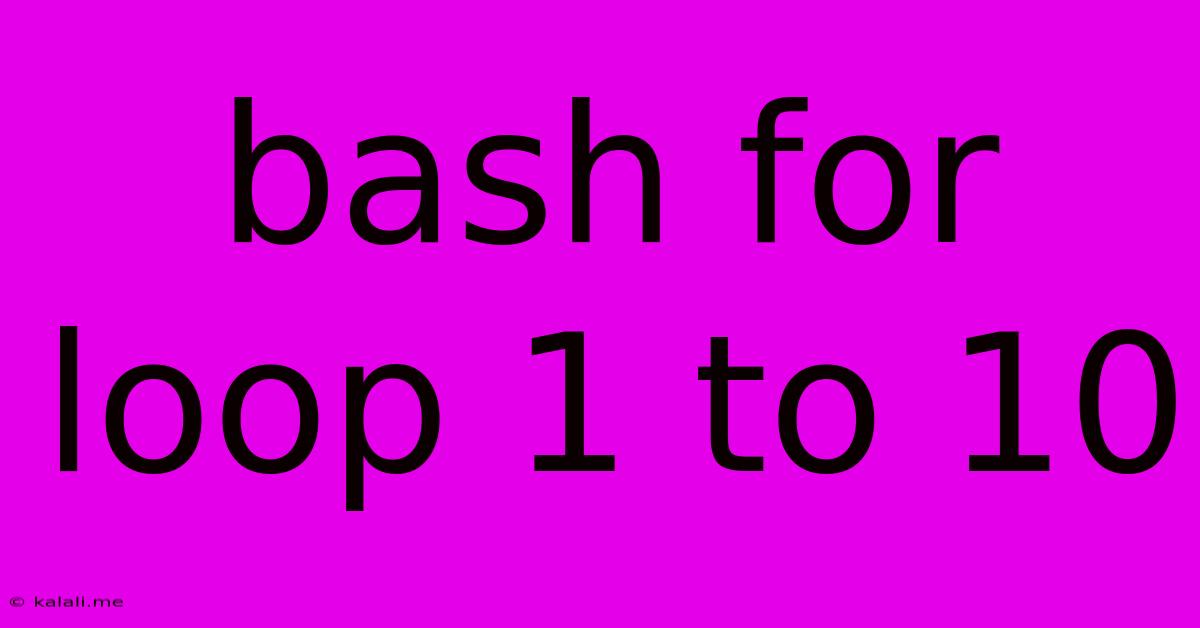
Table of Contents
Bash For Loop 1 to 10: A Comprehensive Guide
This article provides a comprehensive guide on how to create a for
loop in Bash that iterates from 1 to 10. We'll explore various methods, highlighting their advantages and disadvantages, and offering best practices for writing clean and efficient Bash scripts. Understanding Bash for
loops is fundamental for any scripting enthusiast aiming to automate tasks and manage system processes effectively. This tutorial will cover everything from basic syntax to more advanced techniques.
Method 1: Using Sequence Generation with {1..10}
The simplest and most common approach uses brace expansion to generate a sequence of numbers:
for i in {1..10}; do
echo "Iteration: $i"
done
This concise method directly creates the sequence 1 through 10. The $i
variable holds the current value in each iteration. This is ideal for simple loops where you need to iterate through a known, consecutive range.
Advantages: Readability and simplicity.
Disadvantages: Limited flexibility if you need a non-consecutive sequence or a different step size.
Method 2: Using seq
Command
The seq
command provides more control over the sequence generation:
for i in $(seq 1 10); do
echo "Iteration: $i"
done
seq 1 10
generates the numbers 1 through 10, separated by spaces. The $(...)
performs command substitution, providing the output of seq
to the for
loop. This approach offers more flexibility, allowing you to specify a different step size (e.g., seq 1 2 10
for even numbers).
Advantages: More flexible than brace expansion, allowing control over step size and starting/ending numbers.
Disadvantages: Slightly less readable than brace expansion, and using command substitution can be less efficient for very large sequences.
Method 3: Using C-style
For Loop
Bash also supports a C-style for
loop, offering the most control:
for (( i=1; i<=10; i++ )); do
echo "Iteration: $i"
done
This loop initializes i
to 1, continues as long as i
is less than or equal to 10, and increments i
by 1 in each iteration. This structure is familiar to programmers with C, C++, Java, and other C-like languages.
Advantages: Maximum control and flexibility, including the ability to easily use complex increment/decrement logic.
Disadvantages: Can be less readable for those unfamiliar with C-style syntax. It might be overkill for simple loops.
Best Practices and Considerations
- Variable Naming: Use descriptive variable names (
counter
,index
, etc.) to improve code readability. - Error Handling: For more robust scripts, incorporate error handling to gracefully manage unexpected situations.
- Efficiency: For extremely large loops, consider optimizing your code to minimize computational overhead. Method 1 is generally most efficient for simple sequences.
- Code Style: Maintain consistent indentation and spacing for better readability.
- Comments: Add comments to explain the purpose of your code.
Choosing the right method depends on your specific needs. For a simple loop iterating from 1 to 10, the brace expansion method is generally preferred for its readability and efficiency. However, for more complex scenarios requiring specific step sizes or intricate logic, the seq
command or the C-style loop offer greater flexibility. Remember to always prioritize clean, well-documented, and efficient code.
Latest Posts
Latest Posts
-
How Many Days Is In 11 Weeks
Jul 14, 2025
-
How Many Grams Are In One Tola Gold
Jul 14, 2025
-
How Many Oz In A Pound Of Freon
Jul 14, 2025
-
How Many Years Are In A Millennia
Jul 14, 2025
-
Words With C As The Second Letter
Jul 14, 2025
Related Post
Thank you for visiting our website which covers about Bash For Loop 1 To 10 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.