Bash How To Set A Variable
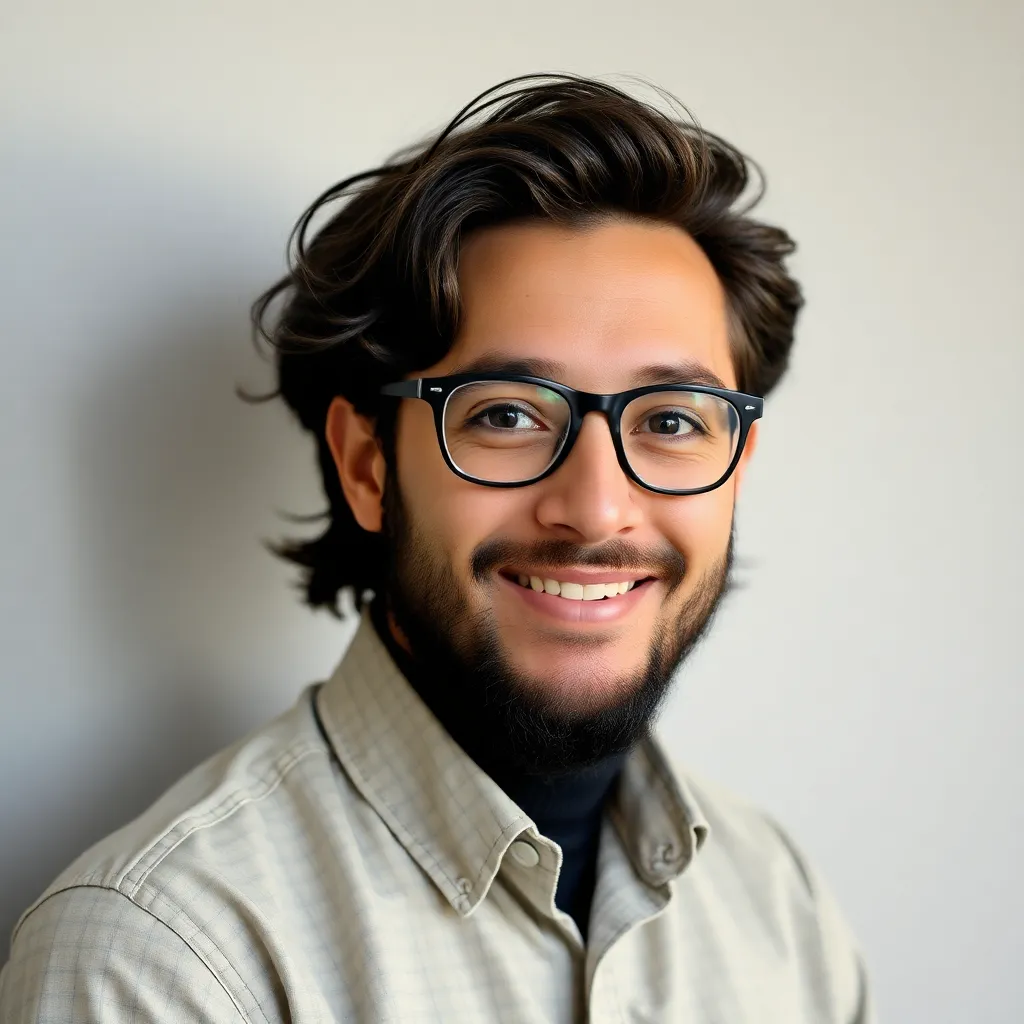
Kalali
May 19, 2025 · 3 min read
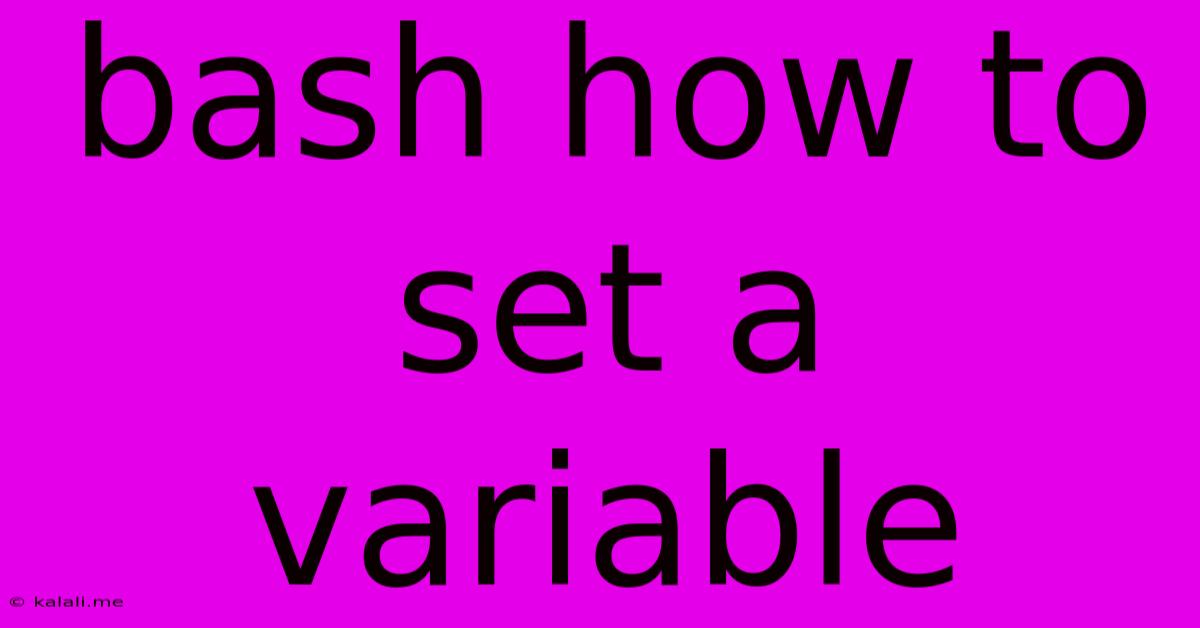
Table of Contents
Bash: How to Set a Variable – A Comprehensive Guide
Setting variables in Bash scripting is fundamental to creating dynamic and efficient scripts. This guide provides a comprehensive overview of how to declare, assign, and utilize variables effectively in your Bash scripts, covering various techniques and best practices. This will cover basic variable assignment, different quoting methods, and advanced techniques like environment variables and array variables. Understanding these concepts is crucial for anyone looking to write robust and reusable Bash scripts.
Variables in Bash are used to store data that your scripts can access and manipulate. They provide a way to make your scripts more flexible and reusable by allowing you to change values without modifying the core script logic.
Basic Variable Assignment
The simplest way to assign a value to a variable in Bash is using the following syntax:
variable_name=value
For example:
my_variable="Hello, world!"
This assigns the string "Hello, world!" to the variable my_variable
. Note that there should be no spaces around the equals sign.
Important Considerations:
- Variable Names: Variable names are case-sensitive.
my_variable
,My_Variable
, andMY_VARIABLE
are all considered different variables. - Quoting: While not always strictly necessary for simple assignments, quoting your values is best practice, especially when dealing with spaces or special characters. This prevents unexpected word splitting and globbing issues. We'll explore quoting more thoroughly in the next section.
- Data Types: Bash is loosely typed, meaning you don't explicitly declare the data type of a variable. The interpreter infers the type based on the assigned value.
Using Quotes Effectively
Quoting your variable assignments is crucial for handling spaces and special characters correctly. Here's a breakdown of the different quoting mechanisms:
- Double Quotes (" "): Allow variable expansion and command substitution within the string. This means other variables within the string will be replaced with their values.
name="John Doe"
greeting="Hello, $name!" # Variable expansion occurs here
echo "$greeting" # Output: Hello, John Doe!
- Single Quotes (' '): Prevent variable expansion and command substitution. The string is treated literally.
literal_string='This string contains $unsubstituted_variable'
echo '$literal_string' #Output: $literal_string
- Backticks (``): Used for command substitution. The command inside the backticks is executed, and its output is assigned to the variable. While functional, it's generally recommended to use
$(...)
for better readability and nested command substitution.
date_string=`date`
echo "$date_string"
Recommended Approach: Use double quotes unless you explicitly need to prevent variable expansion, in which case use single quotes. Prefer $(...)
over backticks for command substitution.
Advanced Techniques
Environment Variables
Environment variables are special variables that are accessible by all processes and subshells launched by the current shell. They're typically set using export
.
export MY_ENV_VAR="This is an environment variable"
Array Variables
Bash also supports arrays. You can assign values to array elements like this:
my_array=("apple" "banana" "cherry")
echo "${my_array[0]}" # Output: apple
Unsetting Variables
To remove a variable and its value, use the unset
command:
unset my_variable
Best Practices
- Use descriptive variable names that clearly indicate their purpose.
- Consistently use quoting to avoid unexpected behavior.
- Avoid using uppercase letters for user-defined variables to prevent accidental conflicts with environment variables.
- Comment your code to explain the purpose of your variables.
By mastering these techniques, you'll be able to write more robust, efficient, and maintainable Bash scripts. Remember to practice and experiment to solidify your understanding of variable handling in Bash. This will greatly improve the overall quality and readability of your scripts.
Latest Posts
Latest Posts
-
How Much Is 4 Oz Chocolate Chips
Jul 13, 2025
-
How Many Times Does 9 Go Into 70
Jul 13, 2025
-
4 Pics 1 Word Cheat 8 Letters
Jul 13, 2025
-
220 Kilometers Per Hour To Miles Per Hour
Jul 13, 2025
-
Which Number Produces A Rational Number When Added To 0 25
Jul 13, 2025
Related Post
Thank you for visiting our website which covers about Bash How To Set A Variable . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.