Bash Loop Through Files In Directory
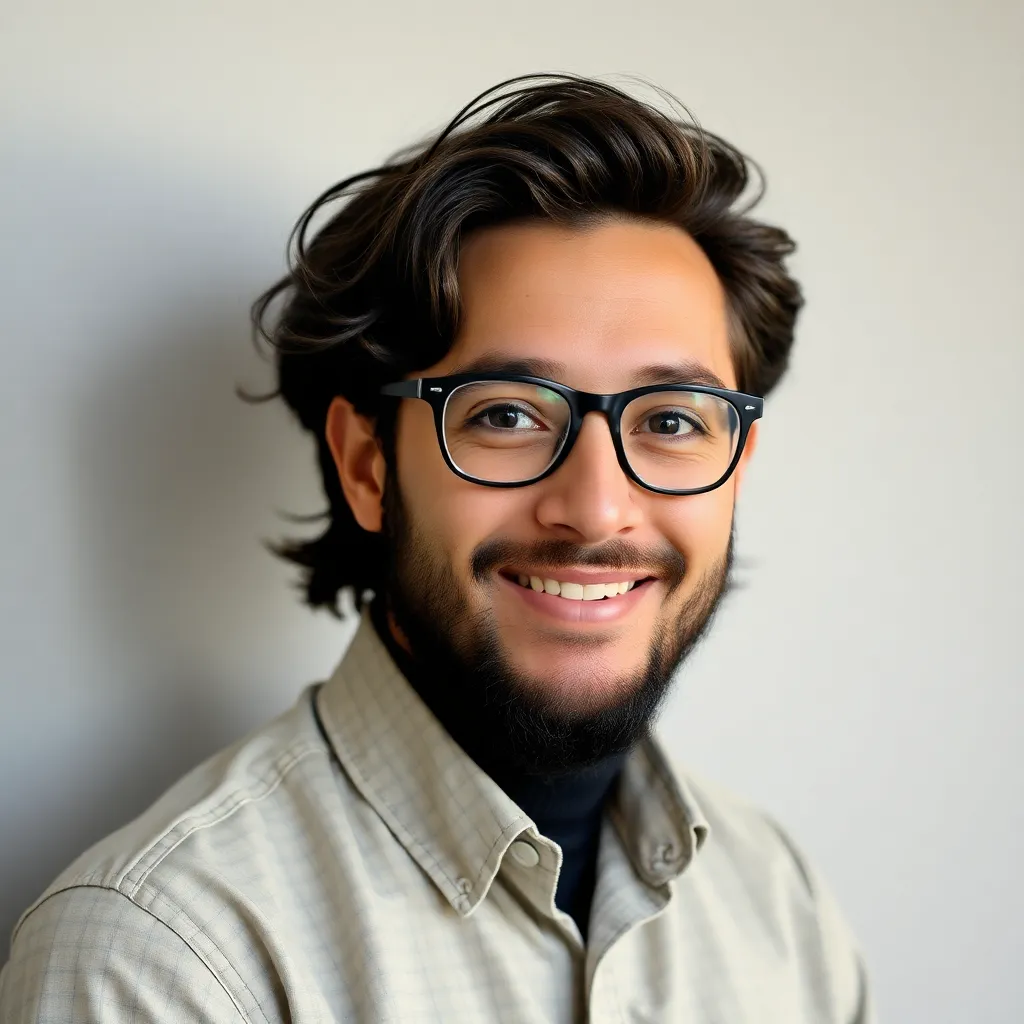
Kalali
May 28, 2025 · 3 min read
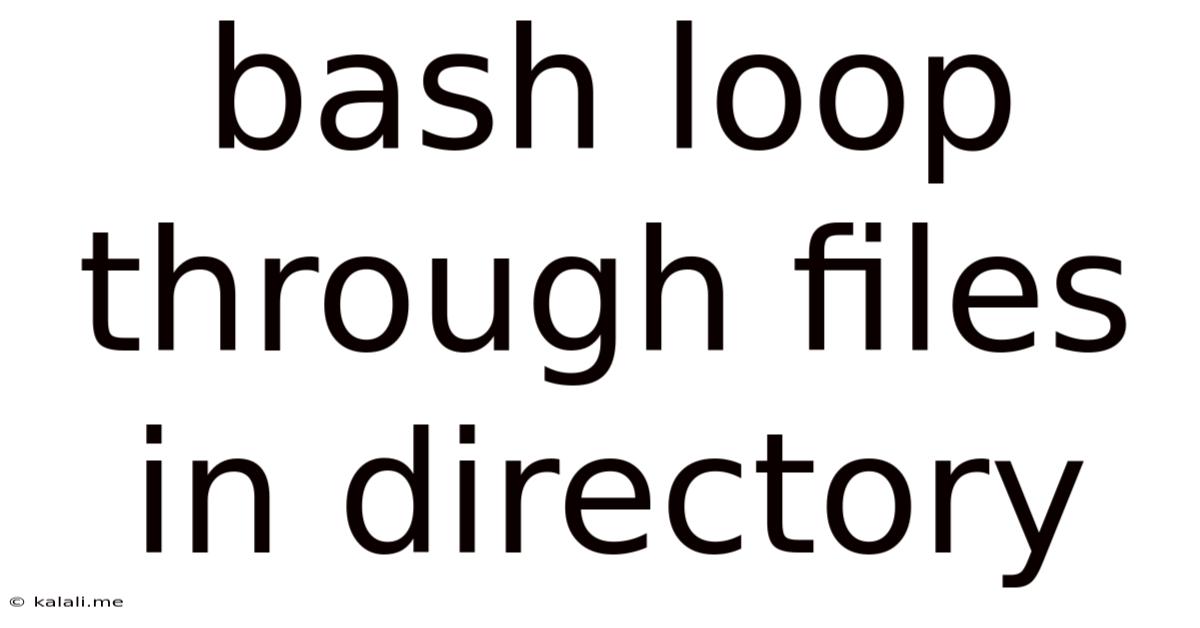
Table of Contents
Bash Loop Through Files in a Directory: A Comprehensive Guide
This guide provides a comprehensive overview of how to iterate through files within a specific directory using bash scripting. We'll cover various scenarios and techniques, from simple loops to more advanced methods for handling different file types and situations. Understanding these techniques is crucial for automating tasks and managing files efficiently within your Linux or macOS environment.
This article will cover:
- Basic
for
loop iteration - Handling different file types
- Using
find
for more advanced searches - Processing files with specific extensions
- Error handling and robustness
Basic for
Loop Iteration
The simplest way to loop through files in a directory is using a for
loop. This method works well for basic scenarios where you need to process every file in a directory. However, it doesn't offer advanced filtering capabilities.
for file in /path/to/your/directory/*; do
echo "Processing: $file"
# Add your file processing commands here
done
Replace /path/to/your/directory
with the actual path to your directory. The *
acts as a wildcard, selecting all files and directories within the specified path. The loop iterates through each item, assigning it to the $file
variable. You can then perform operations on each $file
within the do...done
block.
Important Note: This approach includes directories. If you only want to process files, you'll need more sophisticated methods (discussed below).
Handling Different File Types
Often, you need to process only files of a specific type. For instance, you might want to only work with .txt
files or .jpg
images. You can achieve this using pattern matching within the for
loop:
for file in /path/to/your/directory/*.txt; do
echo "Processing text file: $file"
# Process the text file
done
This example only processes files ending with .txt
. You can adapt the pattern to match other file extensions or naming conventions. However, this approach still has limitations when dealing with complex file structures or hidden files.
Using find
for More Advanced Searches
The find
command offers a powerful and flexible way to locate files based on various criteria. Combined with xargs
, it provides a robust solution for iterating through files.
find /path/to/your/directory -type f -name "*.txt" -print0 | xargs -0 -I {} bash -c 'echo "Processing: {}"; # Your processing commands here'
find /path/to/your/directory
: Specifies the directory to search.-type f
: Filters for files only (excluding directories).-name "*.txt"
: Filters for files ending in.txt
.-print0
: Prints the filenames separated by null characters (safe for filenames with spaces or special characters).xargs -0 -I {}
: Passes the null-separated filenames tobash -c
, using{}
as a placeholder for each filename.
This method is significantly more robust and allows for complex filtering based on file type, size, modification time, and other attributes.
Processing Files with Specific Extensions
Building upon the previous examples, let's demonstrate processing files with multiple extensions:
find /path/to/your/directory -type f \( -name "*.txt" -o -name "*.log" \) -print0 | xargs -0 -I {} bash -c 'echo "Processing: {}"; # Your processing commands here'
The \( -name "*.txt" -o -name "*.log" \)
part uses parentheses and the -o
operator (OR) to match files ending in either .txt
or .log
.
Error Handling and Robustness
For production scripts, incorporating error handling is crucial. Check the exit status of commands using $?
to ensure your script handles failures gracefully.
for file in /path/to/your/directory/*.txt; do
some_command "$file"
if [ $? -ne 0 ]; then
echo "Error processing $file"
# Handle the error appropriately (e.g., log it, skip the file)
fi
done
By understanding and applying these techniques, you can efficiently and reliably loop through files in a directory using bash, allowing for automation of a wide variety of file processing tasks. Remember to always prioritize clear code, robust error handling, and security best practices when writing bash scripts.
Latest Posts
Latest Posts
-
What Is 1 5 Of A Tablespoon
Jun 30, 2025
-
How Long Does It Take To Drive Through Illinois
Jun 30, 2025
-
If I Was Born In 1988 How Old Am I
Jun 30, 2025
-
How Much Is 800 Grams Of Chicken
Jun 30, 2025
-
How Long Will It Take To Walk 20 Miles
Jun 30, 2025
Related Post
Thank you for visiting our website which covers about Bash Loop Through Files In Directory . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.