Bash Read A File Line By Line
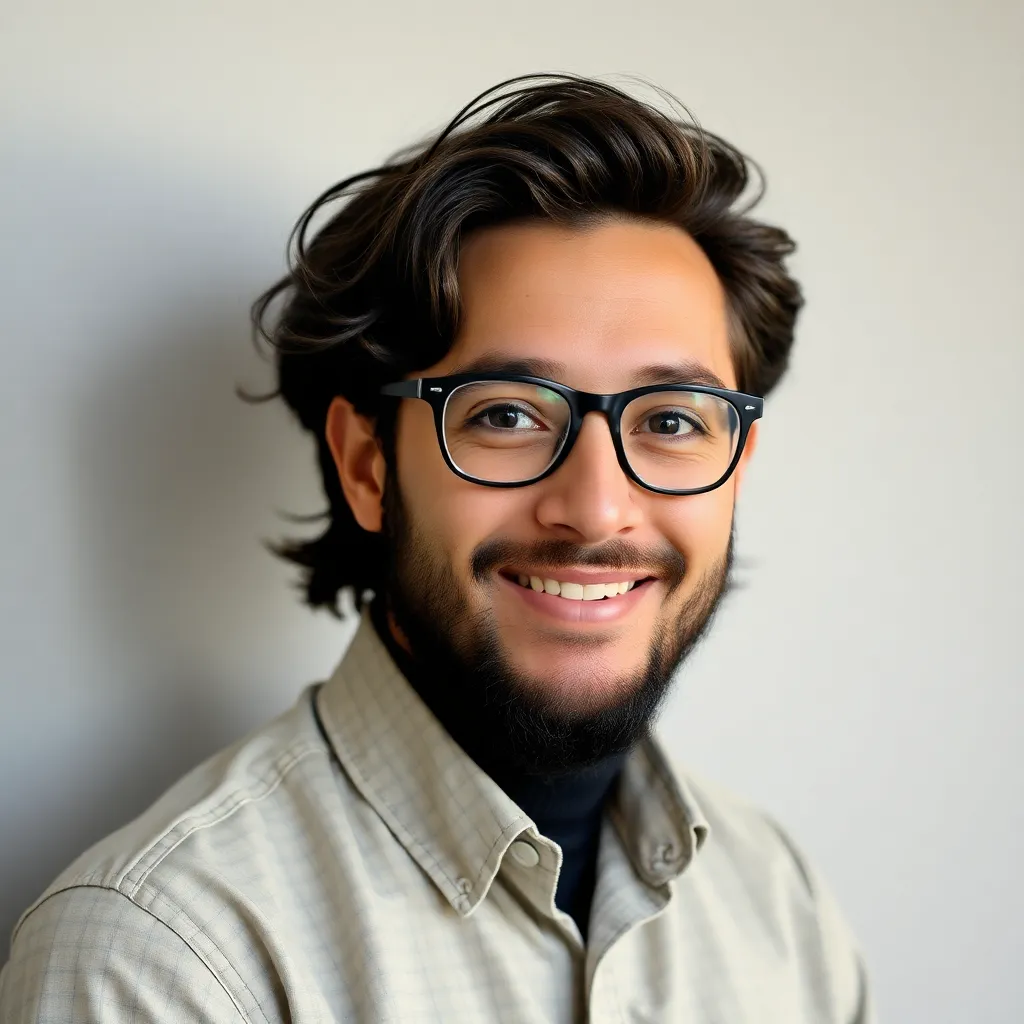
Kalali
May 21, 2025 · 3 min read
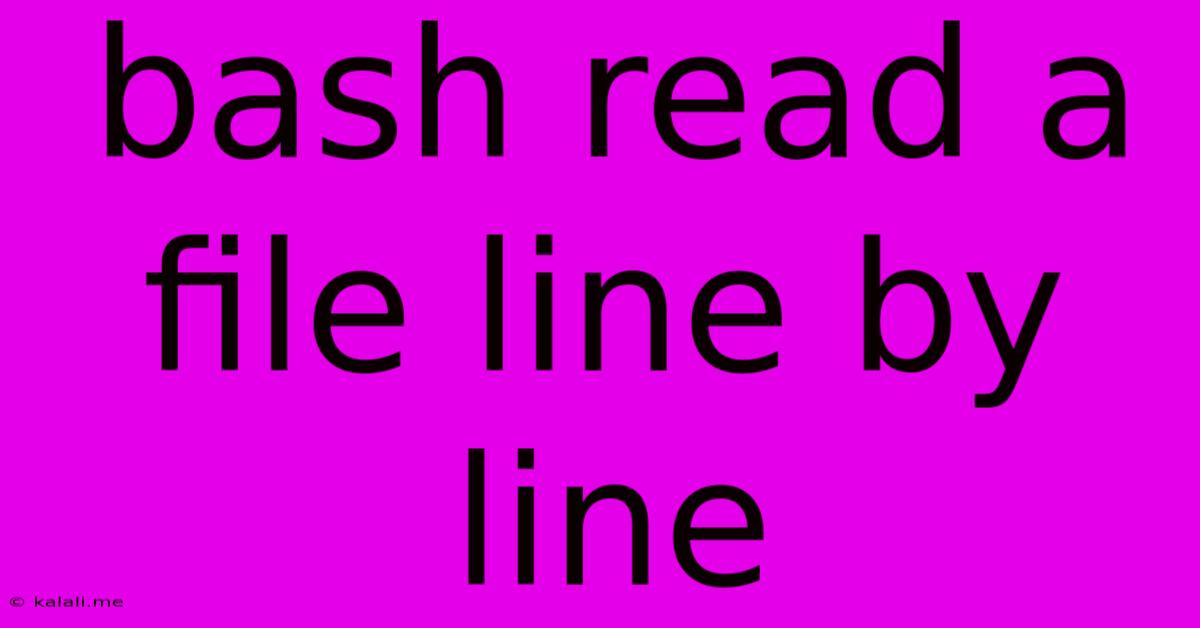
Table of Contents
Bash: Reading a File Line by Line
Reading files line by line is a fundamental task in Bash scripting. This process allows for efficient processing of large files without loading the entire file into memory at once, preventing potential memory issues and improving performance. This article explores several effective methods for achieving this, along with explanations and examples. Understanding these techniques is crucial for writing robust and efficient Bash scripts.
Understanding the while
loop and read
command
The core of line-by-line file reading in Bash involves the while
loop and the read
command. The while
loop continues to execute as long as a condition is true, and read
reads a single line from the standard input (usually a file). Let's see a basic implementation:
while IFS= read -r line; do
echo "$line"
done < "my_file.txt"
IFS=
: This sets the Internal Field Separator to null, preventing unexpected word splitting.read -r
: The-r
option prevents backslashes from being interpreted literally. This is important for handling files containing backslashes.line
: This variable stores the current line read from the file.echo "$line"
: This prints the current line. Crucially, the line is double-quoted to prevent word splitting and globbing.< "my_file.txt"
: This redirects the content of "my_file.txt" to the standard input of thewhile
loop.
Handling different file types and scenarios
The above method works well for simple text files. However, you might encounter files with different characteristics, demanding more sophisticated approaches.
Reading files with empty lines
Empty lines might be crucial data in some cases, so handling them correctly is essential. The basic while
loop shown above will successfully read and process empty lines. No special handling is necessary.
Processing specific lines based on criteria
Often, you need to process only lines matching specific criteria. You can incorporate conditional statements (if
, elif
, else
) within the while
loop to filter lines.
while IFS= read -r line; do
if [[ "$line" == *"keyword"* ]]; then
echo "Found keyword: $line"
fi
done < "my_file.txt"
This example prints only lines containing the "keyword" string.
Working with large files
For exceptionally large files, consider using techniques to improve efficiency further. Avoid unnecessary operations within the loop. Processing can be optimized by using tools like awk
or sed
for specific tasks rather than doing it within the Bash script itself.
Error Handling
It is always good practice to include error handling in your scripts. Check if the file exists before attempting to read it:
if [ -f "my_file.txt" ]; then
while IFS= read -r line; do
# Process the line
done < "my_file.txt"
else
echo "Error: File my_file.txt not found."
fi
This code snippet adds a check to ensure the file exists before proceeding.
Alternative approaches: mapfile
and readarray
For Bash versions 4.0 and above, mapfile
(or its synonym readarray
) provides a concise alternative. It reads all lines into an array, making accessing specific lines easier.
mapfile -t lines < "my_file.txt"
for line in "${lines[@]}"; do
echo "$line"
done
mapfile -t lines
: This reads all lines from the file into the arraylines
. The-t
option removes trailing newlines."${lines[@]}"
: This expands to all elements of the array, correctly handling lines containing spaces or special characters.
Conclusion
Reading files line by line in Bash is a common task with several solutions. The while
loop with read
is the fundamental method, offering flexibility and control. mapfile
provides a more compact alternative for newer Bash versions. Choosing the right method depends on your specific needs and the size and characteristics of the file you are processing. Remember to always use proper error handling and quoting to ensure the robustness and reliability of your scripts.
Latest Posts
Latest Posts
-
Does A Candle Heat Up A Room
May 21, 2025
-
Why Is A Toilet Called A John
May 21, 2025
-
Is Surgical Spirit The Same As White Spirit
May 21, 2025
-
How Do I Stop A Door From Swinging Open
May 21, 2025
-
Expression Meaning To Conform To The Accepted Standards
May 21, 2025
Related Post
Thank you for visiting our website which covers about Bash Read A File Line By Line . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.