Bash Read Lines Into Multiple Variables
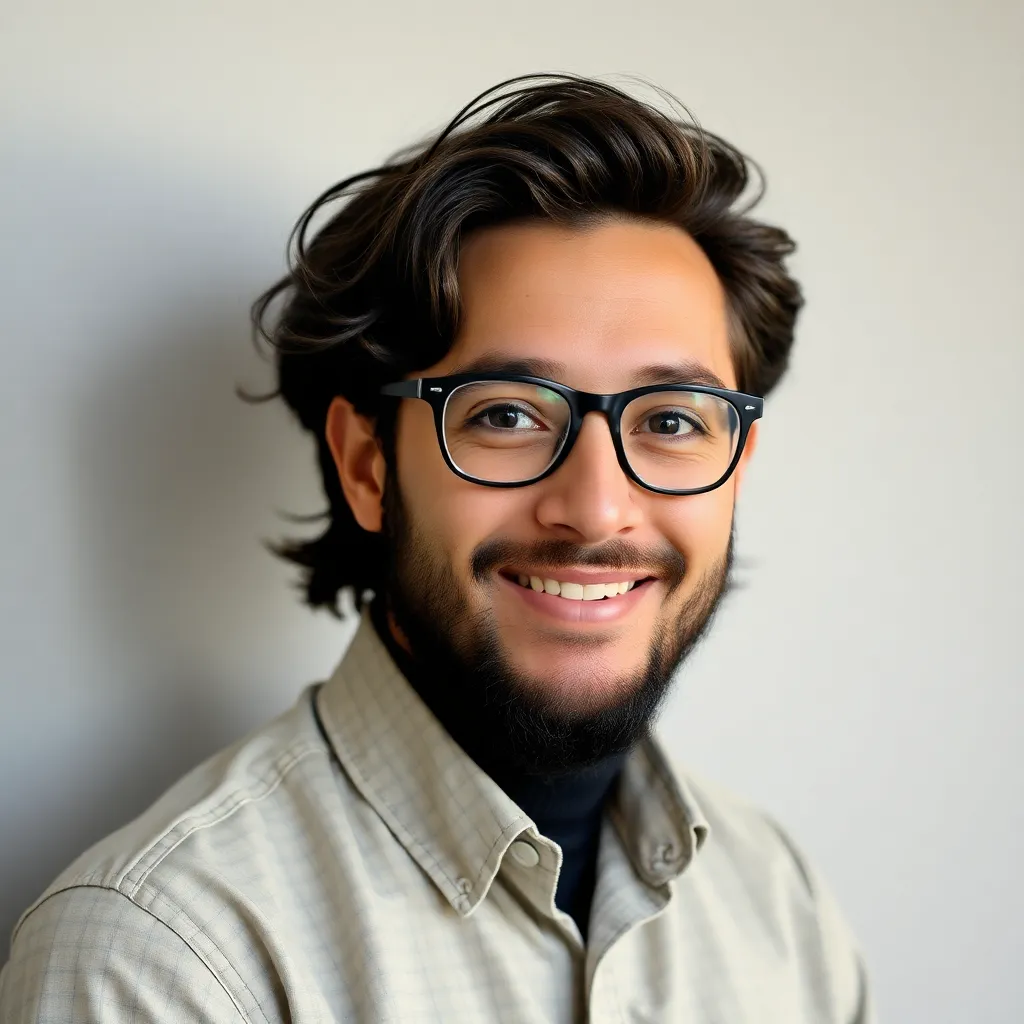
Kalali
May 25, 2025 · 3 min read
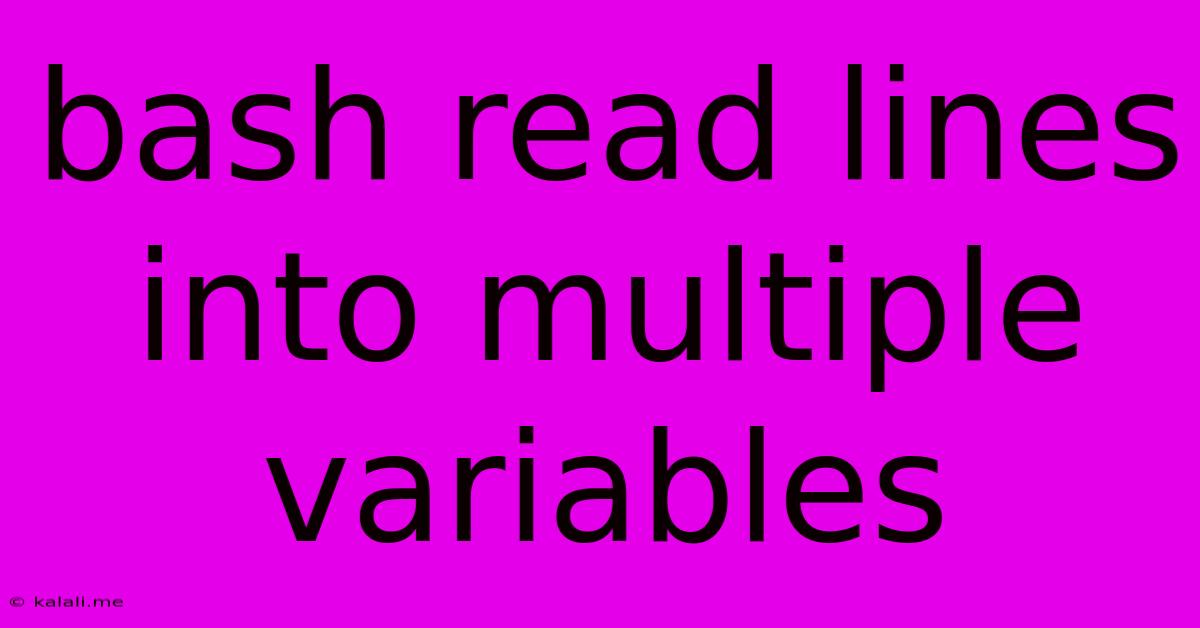
Table of Contents
Reading Multiple Lines into Multiple Variables in Bash
This article explores efficient methods for reading multiple lines from standard input or a file into separate variables within a Bash script. This is a common task in scripting, especially when processing configuration files or structured data. We'll cover various approaches, from simple techniques suitable for a fixed number of lines to more robust solutions that handle an arbitrary number.
Knowing how to efficiently handle multiple lines of input is crucial for anyone working with bash scripting and data processing. This guide offers solutions ranging from straightforward methods for predictable input to more dynamic approaches for handling variable line counts.
Method 1: Direct Variable Assignment (Fixed Number of Lines)
The simplest approach is suitable when you know the exact number of lines you need to read. This involves using the read
command multiple times, assigning each line to a separate variable.
read var1
read var2
read var3
echo "Variable 1: $var1"
echo "Variable 2: $var2"
echo "Variable 3: $var3"
This method is straightforward but limited to a predefined number of lines. If the input has fewer lines than variables, some variables will remain empty. If it has more, the extra lines will be ignored.
Method 2: Using a Loop (Variable Number of Lines)
For a more flexible solution that handles an arbitrary number of lines, a loop is essential. This allows the script to dynamically read lines until the end of input is reached.
while IFS= read -r line; do
# Process each line individually. Example: adding to an array.
lines+=("$line")
done < input.txt
# Access the lines using array indexing.
echo "Line 1: ${lines[0]}"
echo "Line 2: ${lines[1]}"
echo "Last Line: ${lines[-1]}"
This script reads each line from input.txt
into the lines
array. The IFS= read -r line
construct is crucial for correctly handling lines containing spaces or special characters. IFS=
prevents word splitting, and -r
prevents backslash escapes from being interpreted. This is a robust method for processing any number of lines.
Method 3: Read into an Array Directly (Variable Number of Lines)
Bash 4 and later offer a more concise way to read multiple lines into an array directly using mapfile
.
mapfile -t lines < input.txt
echo "Number of lines: ${#lines[@]}"
echo "Line 1: ${lines[0]}"
echo "Last Line: ${lines[-1]}"
mapfile
(or its alias readarray
) reads lines from the specified file or standard input into the lines
array. The -t
option removes trailing newlines from each line. This is often the preferred and most efficient method for reading multiple lines into an array.
Choosing the Right Method
-
Fixed Number of Lines: Use direct variable assignment (Method 1) only if you're certain about the number of input lines.
-
Variable Number of Lines: Use either a
while
loop (Method 2) ormapfile
(Method 3).mapfile
is generally preferred for its conciseness and efficiency, especially for larger files. Thewhile
loop provides more control if you need to perform conditional processing on individual lines.
Remember to handle potential errors, such as the file not existing. Consider adding error checking to your scripts to make them more robust. This article provides a solid foundation for efficiently managing multiple lines of input within your Bash scripts, leading to cleaner and more maintainable code. By mastering these techniques, you can significantly improve your Bash scripting capabilities.
Latest Posts
Latest Posts
-
Wiring A Ceiling Fan With Two Switches And Remote
May 25, 2025
-
Cannot Compute Exact P Value With Ties
May 25, 2025
-
How To Get Companions Out Of Power Armor
May 25, 2025
-
Its No Fun When The Rabbit Has The Gun
May 25, 2025
-
How Do Hockey Players Know When To Sub
May 25, 2025
Related Post
Thank you for visiting our website which covers about Bash Read Lines Into Multiple Variables . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.