Bash Remove Last Character From String
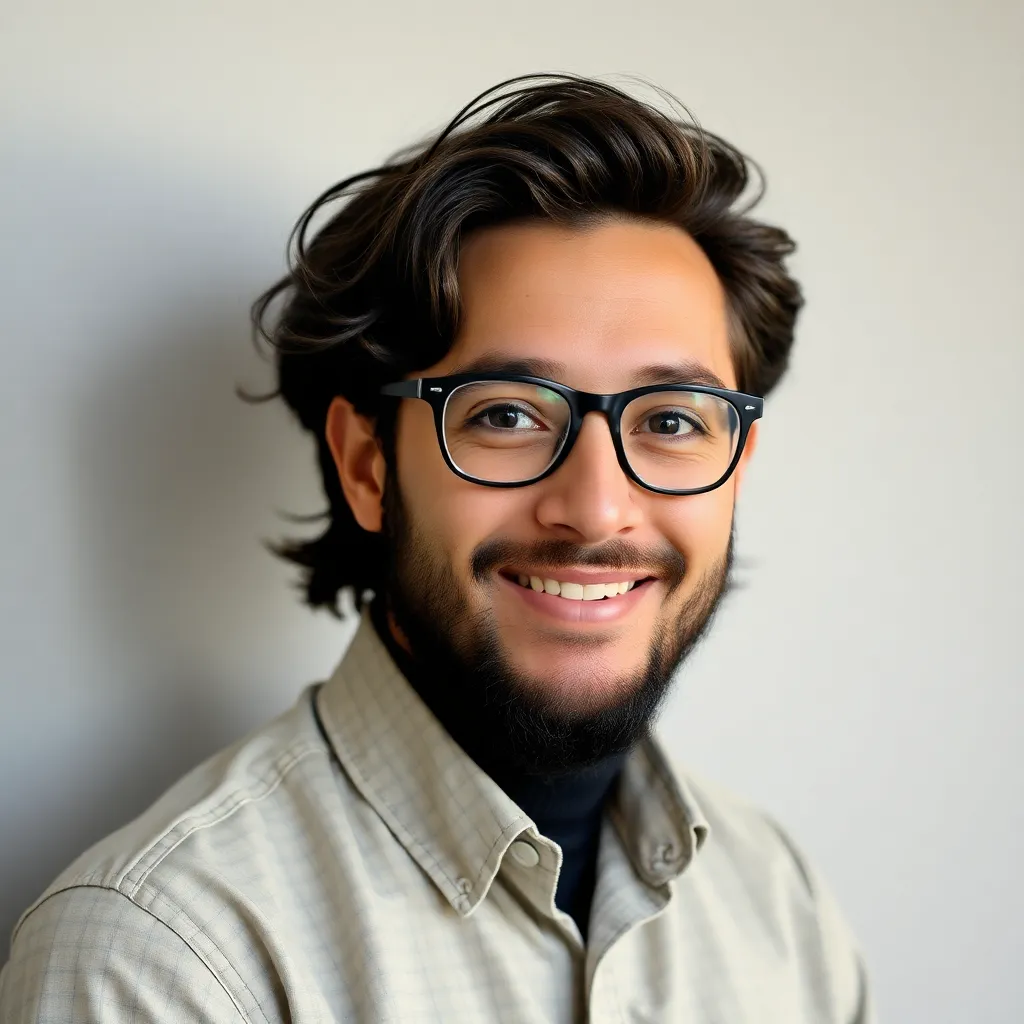
Kalali
May 24, 2025 · 3 min read
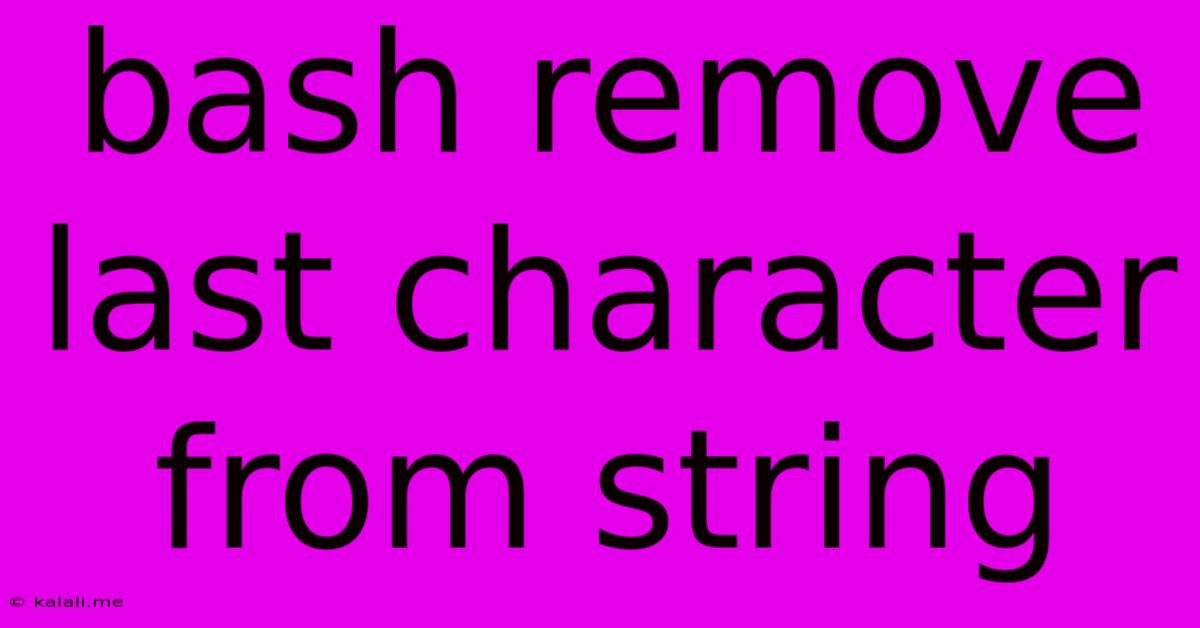
Table of Contents
Bash Remove Last Character From String: A Comprehensive Guide
Removing the last character from a string in Bash scripting is a common task, useful in various scenarios like data processing, file manipulation, and text cleanup. This guide provides several effective methods, ranging from simple parameter expansion to more robust techniques using sed
and awk
. We'll explore each approach, detailing its pros and cons and providing practical examples. This guide covers substring manipulation, string length, and efficient ways to handle potential errors.
Understanding the Problem: The core challenge lies in manipulating strings within Bash, a powerful shell but not inherently designed for complex string operations. We need methods that can reliably extract substrings, accounting for potential edge cases like empty strings or strings with only one character.
Method 1: Parameter Expansion (Most Efficient)
Bash's built-in parameter expansion offers the simplest and most efficient way to remove the last character. This method leverages the ${variable%pattern}
construct, which removes the shortest matching suffix pattern from the variable.
myString="Hello World!"
newString="${myString%?}"
echo "$newString" # Output: Hello World
%?
removes the shortest matching suffix pattern (a single character in this case). This is the most efficient method because it avoids external commands, making it ideal for performance-sensitive scripts.
Pros: Fast, efficient, built-in functionality. Cons: Doesn't handle empty strings gracefully (will produce an empty string, which might be desired or not, depending on your use-case).
Method 2: Using sed
(Versatile and Powerful)
sed
(stream editor) provides a more versatile solution, suitable for more complex scenarios. It allows for sophisticated string manipulation, including removing characters from the end of a string.
myString="Hello World!"
newString=$(echo "$myString" | sed 's/.$//')
echo "$newString" # Output: Hello World
The s/.$//
command in sed
substitutes (s) the last character (.$) with nothing (//). The .
matches any character except a newline, and $
anchors the match to the end of the line.
Pros: Handles edge cases like empty strings gracefully (returns an empty string), provides more flexibility for complex string operations. Cons: Slightly less efficient than parameter expansion due to the overhead of invoking an external command.
Method 3: Using awk
(Another Powerful Tool)
awk
(a pattern scanning and text processing language) is another powerful tool that can achieve this. It's particularly useful when combined with other string manipulation tasks.
myString="Hello World!"
newString=$(echo "$myString" | awk '{print substr($0,1,length($0)-1)}')
echo "$newString" # Output: Hello World
The substr
function extracts a substring from the input string ($0
). It starts at the first character (1), and the length is calculated as the total length minus 1.
Pros: Flexible, can be combined with other awk
functionalities.
Cons: Similar to sed
, slightly less efficient than parameter expansion due to the overhead of an external command.
Choosing the Right Method:
- For simple, performance-critical tasks, parameter expansion is the preferred method.
- For more complex scenarios or when handling potential edge cases like empty strings gracefully,
sed
orawk
offer better flexibility and robustness.sed
is generally faster thanawk
in this specific task.
Error Handling and Robustness
While the examples above work for most cases, consider adding error handling for a more robust script. For instance, you could check the string length before attempting to remove the last character:
myString="Hello World!"
stringLength=${#myString}
if (( stringLength > 0 )); then
newString="${myString%?}"
else
echo "String is empty, nothing to remove."
fi
echo "$newString"
This enhanced script prevents errors when dealing with empty strings. Remember to choose the method that best suits your needs, prioritizing efficiency and robustness based on the context of your script. Understanding the strengths and weaknesses of each approach ensures you write clean, efficient, and reliable Bash scripts.
Latest Posts
Latest Posts
-
How To Run For Loop In Parallel Python
May 24, 2025
-
Can You Brine A Frozen Turkey
May 24, 2025
-
How To Stop Glasses From Sliding Down Nose
May 24, 2025
-
Feet And Inches To Decimal Feet
May 24, 2025
-
Provoke By Saying Do It Do It Do It
May 24, 2025
Related Post
Thank you for visiting our website which covers about Bash Remove Last Character From String . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.