Bash Script Loop Through Text File Of Filenames
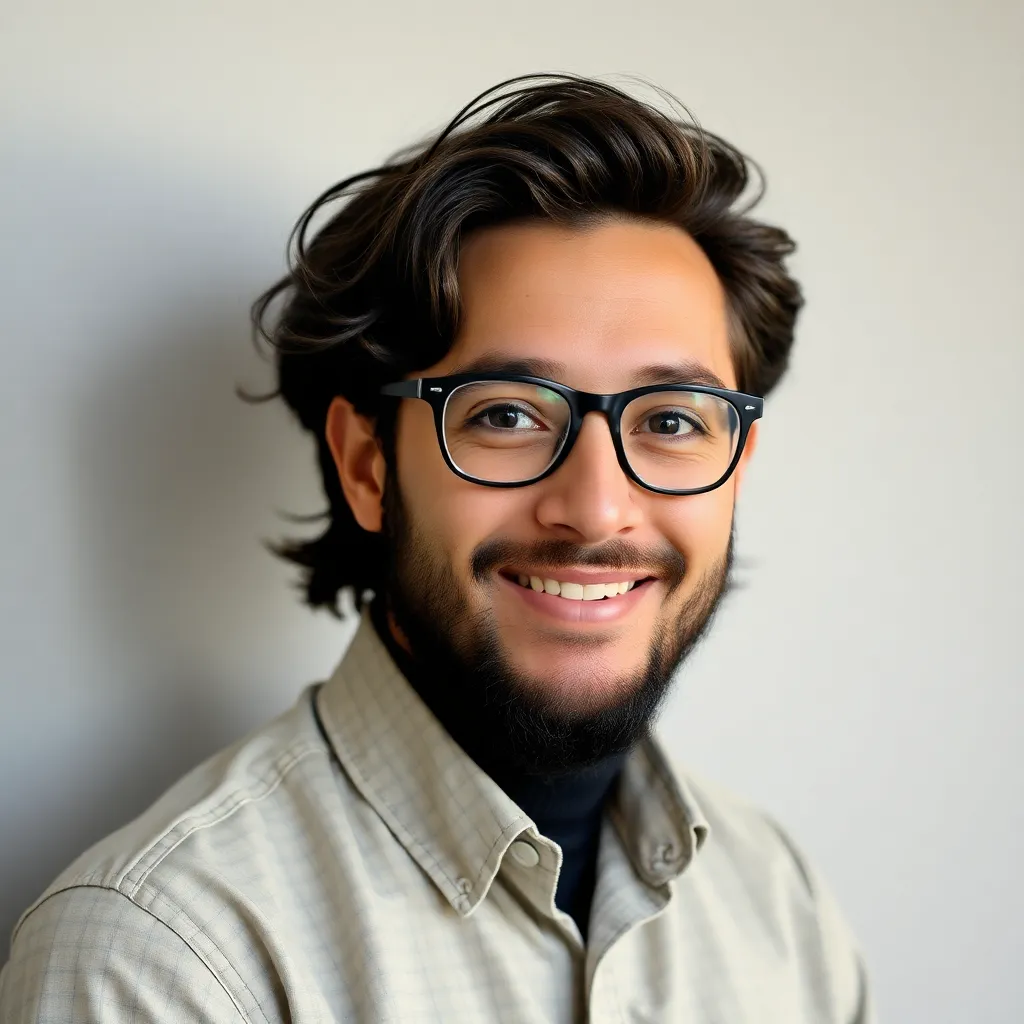
Kalali
May 24, 2025 · 3 min read
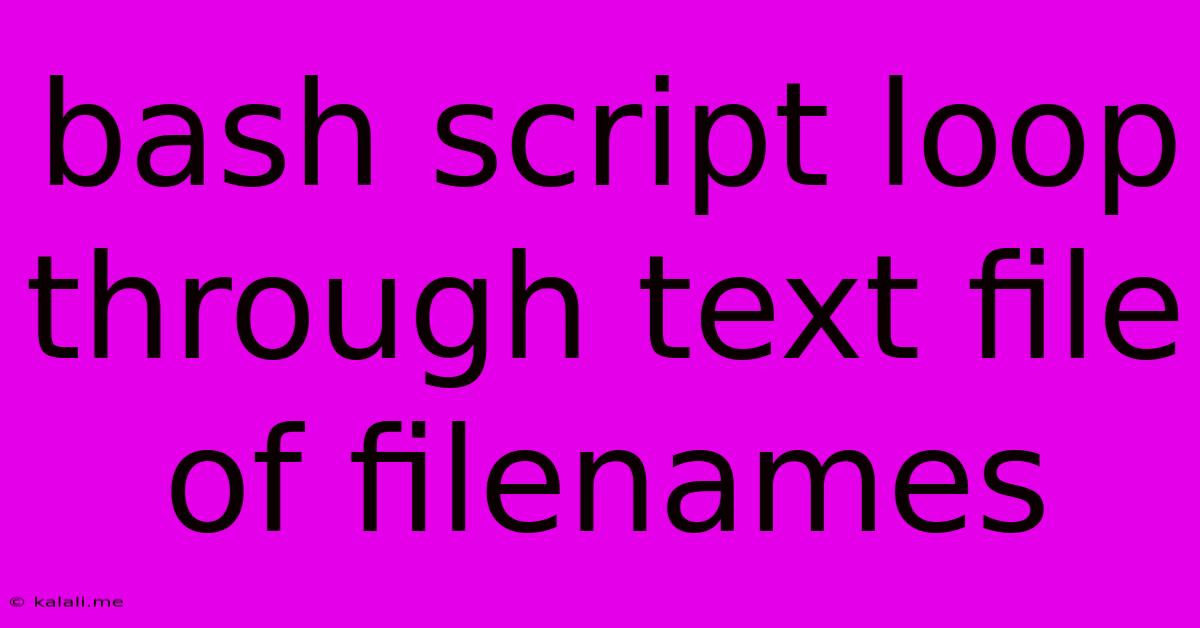
Table of Contents
Bash Script: Looping Through a Text File of Filenames
This article details how to efficiently process a list of filenames stored in a text file using a bash script. This is a common task in scripting, useful for automating file manipulation, processing, or analysis. We'll explore different looping methods and best practices for robustness and error handling. Understanding this technique is crucial for anyone working with bulk file operations in a Linux or macOS environment.
What We'll Cover:
- Reading filenames from a text file.
- Using
while
loops for efficient processing. - Incorporating error handling to manage unexpected situations.
- Advanced techniques for file type filtering and more complex operations.
Reading Filenames from a Text File
The foundation of our script lies in correctly reading the filenames from the input text file. Let's assume your filenames are listed one per line in a file named filenames.txt
.
A simple and effective method is using the while
loop with read
:
while IFS= read -r filename; do
# Process each filename here
echo "Processing: $filename"
done < filenames.txt
IFS= read -r filename
reads each line into the filename
variable. IFS=
prevents word splitting, and -r
prevents backslash escapes from being interpreted. The < filenames.txt
redirects the file's content into the loop.
Processing Each Filename
Inside the while
loop, you can perform any operation on each filename
. For example, to display the contents of each file:
while IFS= read -r filename; do
echo "Processing: $filename"
if [ -f "$filename" ]; then # Check if the file exists
cat "$filename"
else
echo "Error: File '$filename' not found."
fi
done < filenames.txt
This enhanced script includes a crucial check using [ -f "$filename" ]
to verify the file's existence before attempting to process it. This prevents errors and improves script robustness.
Advanced Techniques and Error Handling
Let's expand on this by incorporating more sophisticated error handling and file type filtering:
#!/bin/bash
# Check if the filenames file exists
if [ ! -f "filenames.txt" ]; then
echo "Error: filenames.txt not found."
exit 1
fi
while IFS= read -r filename; do
# Check if the file exists and is a regular file
if [ -f "$filename" ]; then
# Check if the file is a specific type (e.g., .txt)
if [[ "$filename" == *.txt ]]; then
echo "Processing .txt file: $filename"
#Process only .txt files here, for example:
grep "keyword" "$filename" > "${filename%.txt}.out" #save grep output to a new file
else
echo "Skipping non-.txt file: $filename"
fi
else
echo "Error: File '$filename' not found."
fi
done < filenames.txt
echo "Script completed."
exit 0
This improved script:
- Checks for the input file: The script now verifies if
filenames.txt
exists before proceeding. - Filters by file type: It only processes files ending with
.txt
, skipping others. You can easily adjust this to match other file extensions. - Adds more specific processing: It uses
grep
to search for a keyword "keyword" within each .txt file and saves the output to a new file. Remember to replace "keyword" with your actual search term. - Improved error messages: Provides more informative error messages to aid debugging.
- Uses exit codes:
exit 0
indicates successful completion, whileexit 1
signals an error.
This example showcases a powerful and adaptable framework. You can replace the grep
command with any other command-line tool or custom function to perform the desired operations on your files. Remember to adjust file paths and commands to fit your specific needs. This approach promotes clean, maintainable, and robust bash scripting for file processing.
Latest Posts
Latest Posts
-
How To Fix A Toilet That Wont Flush
May 24, 2025
-
Difference Between Convection Bake And Bake
May 24, 2025
-
Let The Chips Fall Where They May
May 24, 2025
-
Are Roma Tomatoes Determinate Or Indeterminate
May 24, 2025
-
How Do You Unclog A Garbage Disposal
May 24, 2025
Related Post
Thank you for visiting our website which covers about Bash Script Loop Through Text File Of Filenames . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.