Bash Set Variable When Other Variables Evaluation
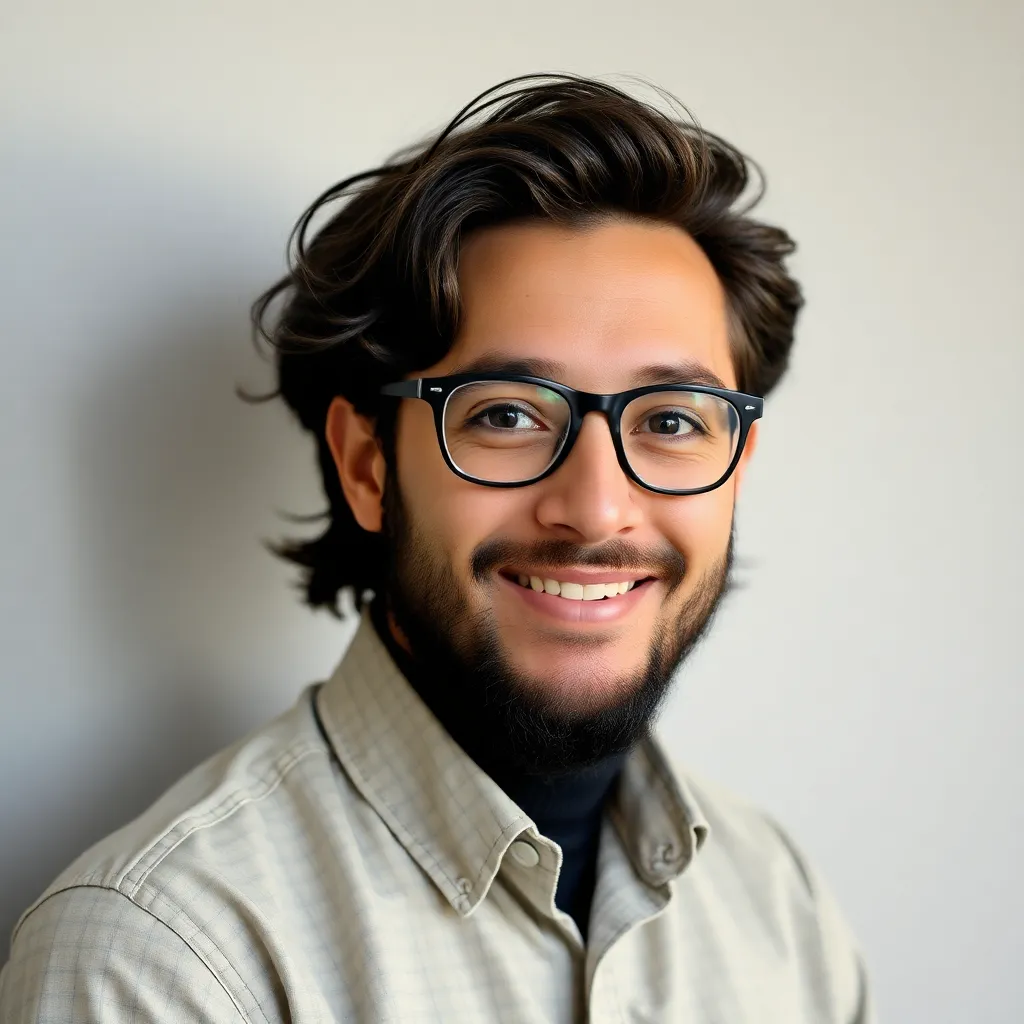
Kalali
May 23, 2025 · 3 min read
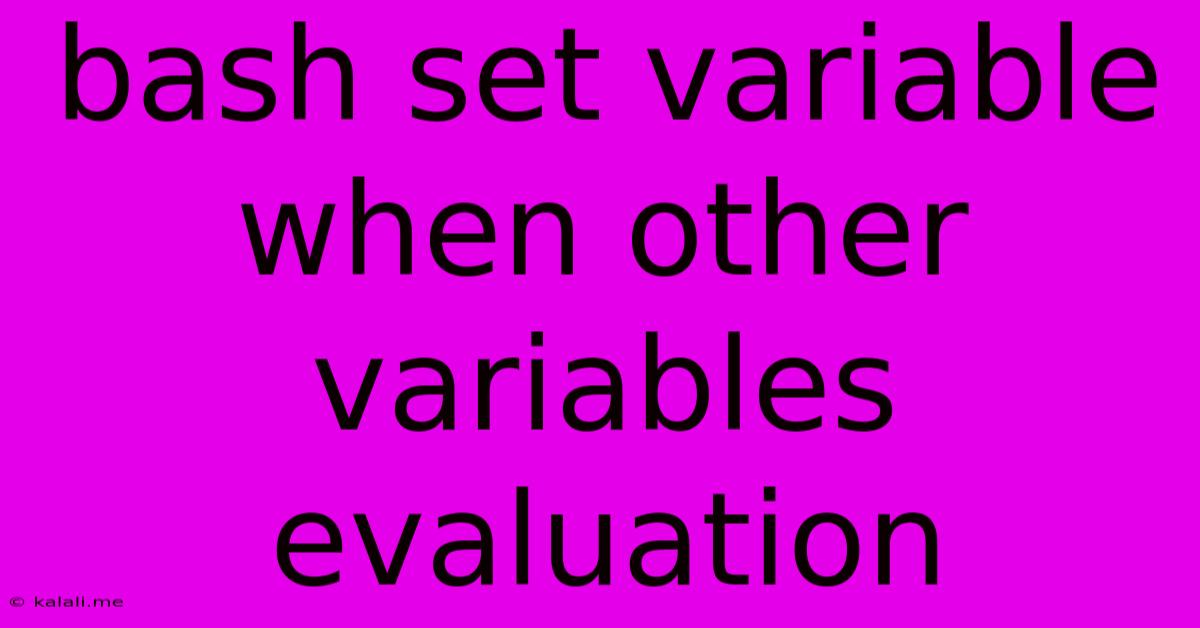
Table of Contents
Bash: Setting Variables Based on Other Variable Evaluations
This article explores how to dynamically set variables in Bash scripting based on the evaluation of other variables. This is a crucial technique for creating flexible and robust scripts that can adapt to different conditions. Understanding how to conditionally assign values allows for cleaner code and easier maintenance. We'll cover various methods, from simple if
statements to more advanced techniques using arithmetic expansion and parameter expansion.
Setting variables based on the evaluation of other variables is a cornerstone of conditional logic in Bash. This allows your scripts to react differently depending on the state of your system or user input. This is essential for creating robust and adaptable scripts.
Using if
statements for conditional variable assignment
The most straightforward approach involves using if
statements to check the value of one variable and then assign a value to another accordingly.
# Example: Setting a status variable based on a file's existence
if [ -f "/path/to/file.txt" ]; then
file_status="exists"
else
file_status="does not exist"
fi
echo "File status: $file_status"
This script checks if /path/to/file.txt
exists. If it does, file_status
is set to "exists"; otherwise, it's set to "does not exist". The -f
operator checks for the existence of a regular file. Remember to replace /path/to/file.txt
with your actual file path.
Utilizing arithmetic expansion for numerical comparisons
When dealing with numerical variables, arithmetic expansion provides a concise way to perform comparisons and assignments.
# Example: Setting a range variable based on a numerical value
number=15
range=$(( number >= 10 ? "high" : "low" ))
echo "Number range: $range"
This uses the conditional expression $(( expression ? value_if_true : value_if_false ))
. If number
is greater than or equal to 10, range
is set to "high"; otherwise, it's set to "low".
Employing parameter expansion for string manipulation and conditional assignments
Parameter expansion offers powerful tools for manipulating strings and performing conditional assignments within the expansion itself.
# Example: Setting a default value if a variable is unset or empty
username=${USER:-"Guest"}
echo "Username: $username"
This uses ${parameter:-word}
. If USER
is unset or empty, username
defaults to "Guest". Other similar operators include ${parameter:=word}
(assigns the word if unset), ${parameter:?word}
(exits with an error if unset), and ${parameter:+word}
(assigns the word if set).
Combining techniques for complex scenarios
For more complex scenarios, you can combine these techniques to create sophisticated conditional logic. Consider nesting if
statements or using arithmetic and parameter expansion within if
conditions.
# Example: Combining techniques
file_size=$(stat -c%s /path/to/file.txt 2>/dev/null)
if [ -f "/path/to/file.txt" ]; then
if (( file_size > 1024 )); then
file_description="Large file"
else
file_description="Small file"
fi
else
file_description="File does not exist"
fi
echo "File description: $file_description"
This example combines file existence checks with file size comparisons to provide a more detailed description.
Best Practices for Variable Assignment in Bash
- Use descriptive variable names: Choose names that clearly indicate the variable's purpose.
- Quote variables when necessary: This prevents word splitting and globbing issues.
- Comment your code: Explain the logic behind your variable assignments.
- Validate user input: Ensure that variables receive expected values to avoid errors.
- Error handling: Implement mechanisms to gracefully handle unexpected conditions.
By mastering these techniques, you can create highly dynamic and efficient Bash scripts that adapt to diverse situations, making your scripting more robust and maintainable. Remember to always prioritize clear, well-documented code for easier debugging and collaboration.
Latest Posts
Latest Posts
-
What Is 1 5 Of A Tablespoon
Jun 30, 2025
-
How Long Does It Take To Drive Through Illinois
Jun 30, 2025
-
If I Was Born In 1988 How Old Am I
Jun 30, 2025
-
How Much Is 800 Grams Of Chicken
Jun 30, 2025
-
How Long Will It Take To Walk 20 Miles
Jun 30, 2025
Related Post
Thank you for visiting our website which covers about Bash Set Variable When Other Variables Evaluation . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.