Bash Set Variable When Other Variables Evaluation With
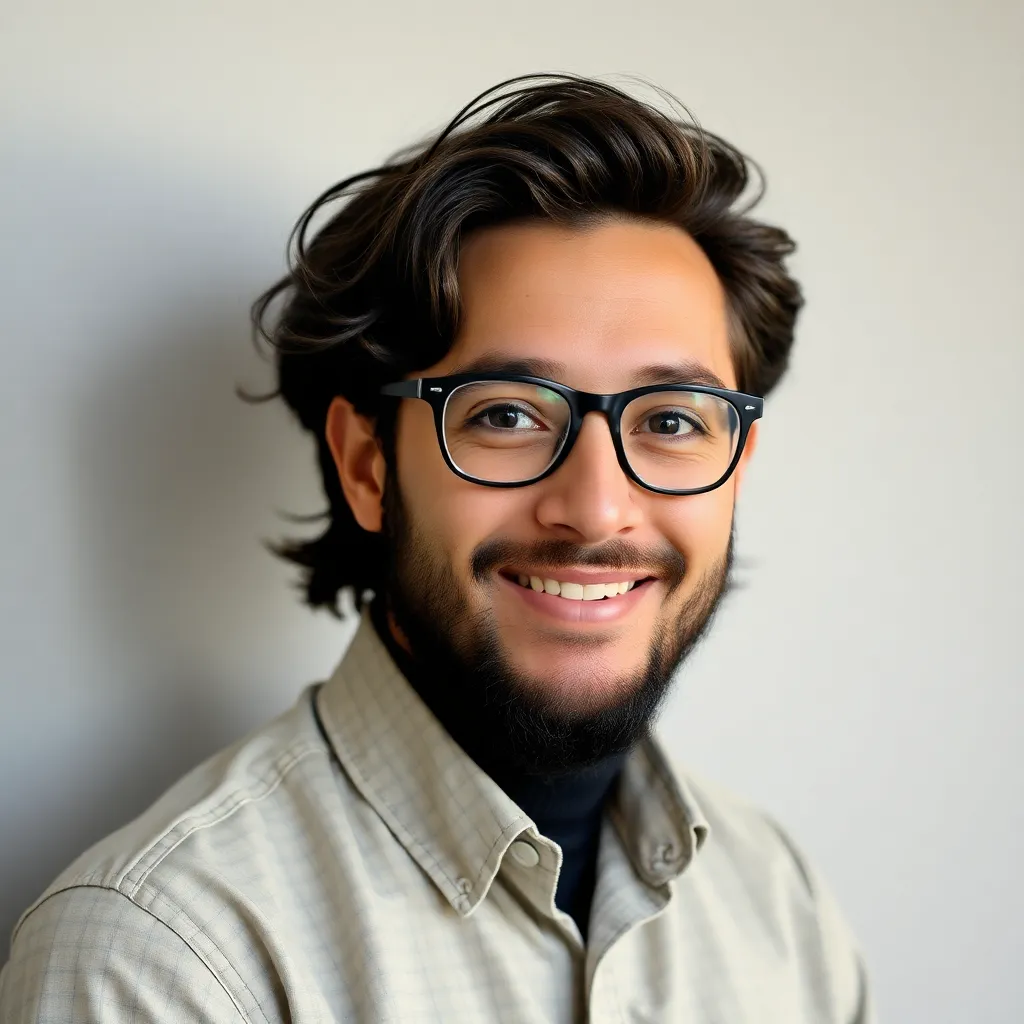
Kalali
May 24, 2025 · 3 min read
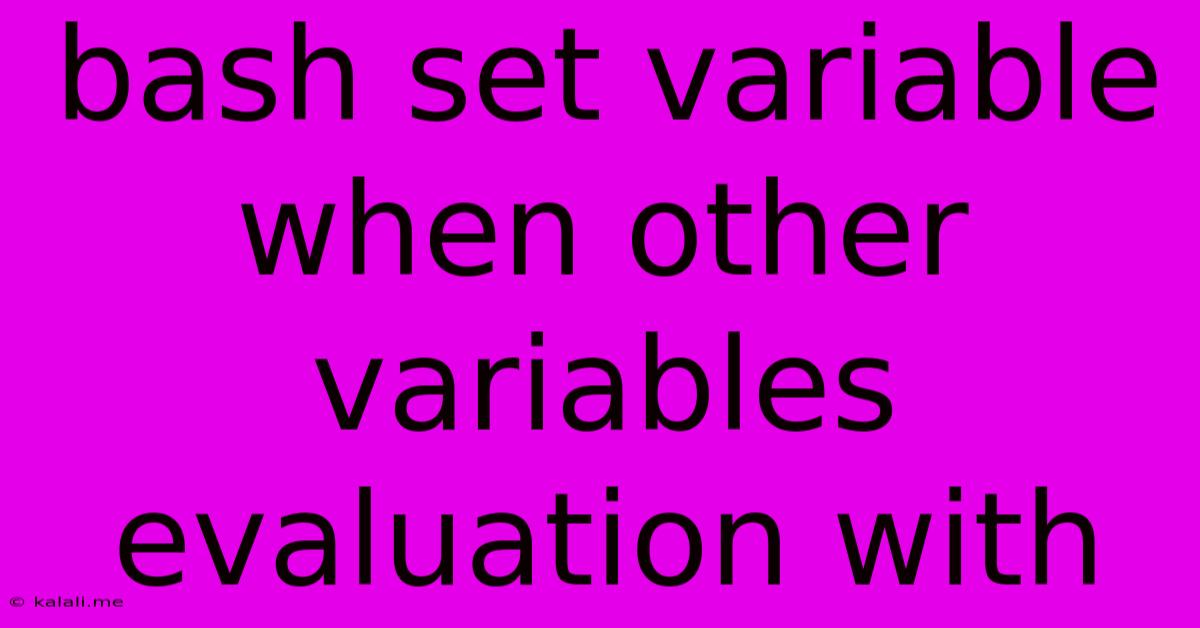
Table of Contents
Bash: Setting Variables Based on Other Variable Evaluations
Setting variables in Bash scripting based on the evaluation of other variables is a fundamental task. This allows for dynamic and adaptable scripts that can react differently depending on various conditions. This article will explore different methods to achieve this, covering various scenarios and best practices. Understanding these techniques is crucial for writing efficient and robust Bash scripts.
Understanding Variable Evaluation in Bash
Before delving into specific examples, it's crucial to grasp how Bash evaluates variables. Bash performs variable substitution, replacing variable names with their values before the command is executed. This process occurs within double quotes ("
), allowing for complex expressions. Single quotes ('
) prevent variable expansion.
For example:
my_var="Hello"
echo "The value is: $my_var" # Output: The value is: Hello
echo 'The value is: $my_var' # Output: The value is: $my_var
Methods for Setting Variables Based on Other Variable Evaluations
Several approaches exist for setting variables based on other variable evaluations. The most common and versatile methods include conditional statements and arithmetic expansion.
1. Conditional Statements (if, elif, else)**
Conditional statements are the cornerstone of controlling program flow based on variable values. if
, elif
(else if), and else
allow you to execute different code blocks depending on whether a condition is true or false.
status="success"
if [ "$status" == "success" ]; then
result="Operation completed successfully"
elif [ "$status" == "failure" ]; then
result="Operation failed"
else
result="Unknown status"
fi
echo "$result"
This script checks the status
variable and assigns a corresponding message to the result
variable. Note the use of double brackets [ ]
(or test
) for condition evaluation and proper quoting to prevent errors.
2. Arithmetic Expansion**
Arithmetic expansion allows performing arithmetic operations on variables and assigning the results to new variables.
count=10
new_count=$((count + 5))
echo "New count: $new_count" # Output: New count: 15
This example adds 5 to the count
variable and assigns the result to new_count
. The $(( ))
syntax is used for arithmetic expansion.
3. Case Statements**
Case statements provide a concise way to handle multiple possible values of a variable.
environment="production"
case "$environment" in
"development")
db_host="localhost"
;;
"testing")
db_host="testdb.example.com"
;;
"production")
db_host="prod.example.com"
;;
*)
db_host="unknown"
;;
esac
echo "Database host: $db_host"
This script sets the db_host
variable based on the environment
variable's value. The *)
acts as a default case if none of the specified values match.
4. Using Parameter Expansion with Conditional Operators**
Bash offers powerful parameter expansion features combined with conditional operators for concise variable assignments.
file_exists="/tmp/my_file.txt"
file_size=${#file_exists:+${file_exists}} # Check if file exists
echo "File size: $file_size"
This example will assign a file's size to a variable only if the file exists. It leverages parameter expansion to check for variable existence and subsequently obtain file size. If the file does not exist, the variable remains empty.
Best Practices
- Use double quotes: Always enclose variable substitutions within double quotes to prevent word splitting and globbing issues.
- Error handling: Include checks to handle unexpected variable values or situations.
- Readability: Prioritize code clarity and use comments to explain complex logic.
- Maintainability: Structure your scripts logically and modularly for easy modification and debugging.
By mastering these methods, you can create dynamic and robust Bash scripts capable of adapting to diverse situations based on variable evaluations, enhancing the flexibility and power of your automation tasks. Remember to always test your scripts thoroughly to ensure correct behavior under various conditions.
Latest Posts
Latest Posts
-
Mother And I Or Mother And Me
Jul 18, 2025
-
How Many Oz In One Water Bottle
Jul 18, 2025
-
How Many Dimes In A 5 Roll
Jul 18, 2025
-
How Do You Say Basil In Spanish
Jul 18, 2025
-
How Many Cookies Are In A Dozen
Jul 18, 2025
Related Post
Thank you for visiting our website which covers about Bash Set Variable When Other Variables Evaluation With . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.