Bash Set Variable With Indirect Reference Name
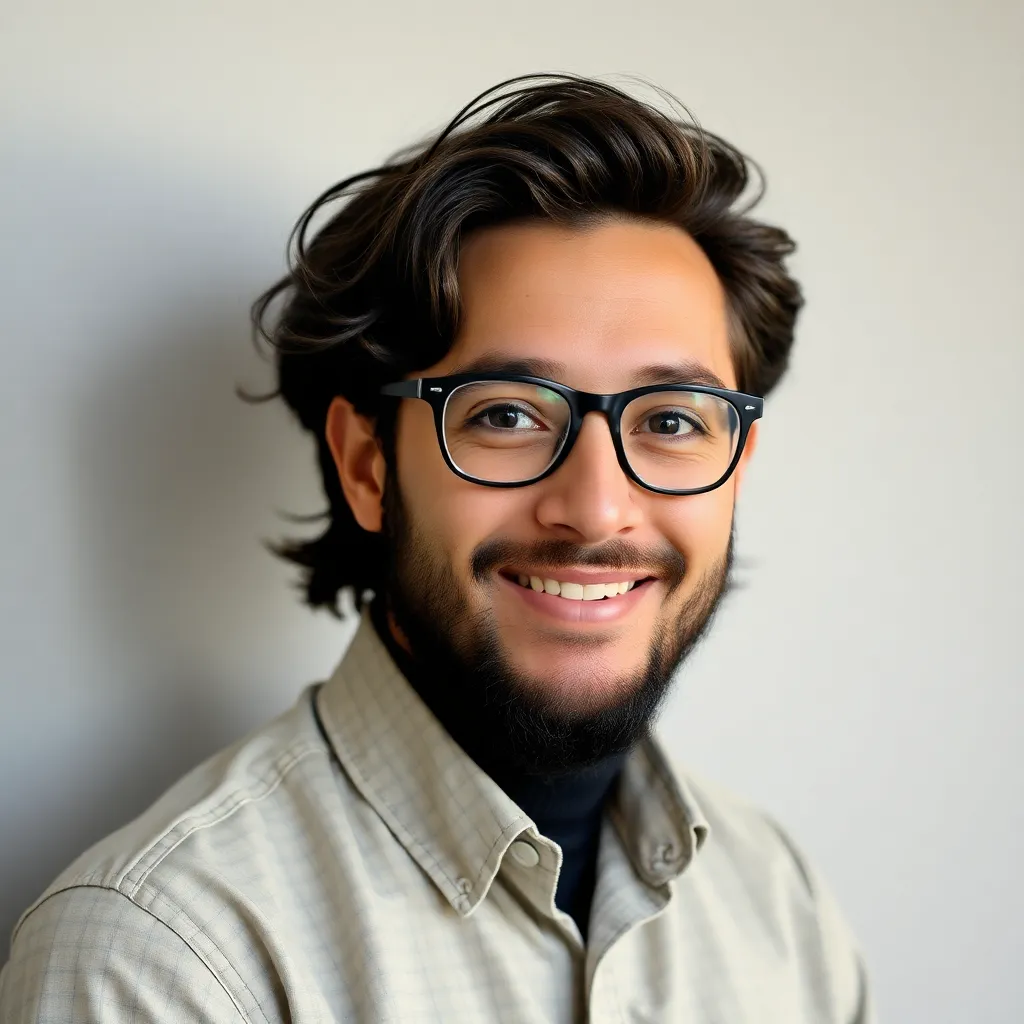
Kalali
May 25, 2025 · 3 min read
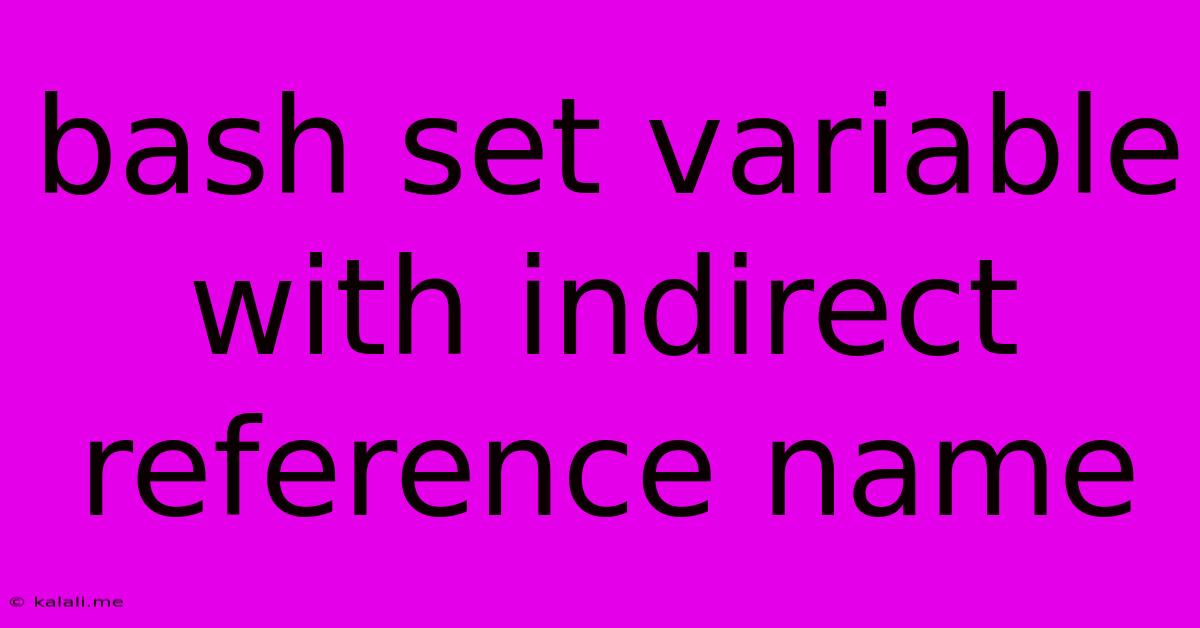
Table of Contents
Bash Set Variable with Indirect Reference Name: A Comprehensive Guide
This article will guide you through the intricacies of setting variables in Bash using indirect referencing. Understanding this technique is crucial for writing robust and dynamic Bash scripts, particularly when dealing with variable names stored in other variables. We'll cover the fundamental methods and provide practical examples to solidify your understanding. This will allow you to create more powerful and flexible scripting solutions.
In Bash, indirect referencing allows you to manipulate variable names dynamically. Instead of directly accessing a variable, you use the value of another variable to determine the target variable's name. This is particularly useful when the names of variables are generated during runtime or are stored within an array or other data structure.
Understanding the !
Operator
The core of indirect referencing in Bash lies in the !
operator, also known as the indirection operator. It's used to dereference a variable, meaning it resolves the variable name stored within another variable. Let's illustrate this with an example:
var_name="my_variable"
my_variable="Hello, world!"
echo "${!var_name}" # Output: Hello, world!
In this example, var_name
holds the string "my_variable". The expression ${!var_name}
tells Bash to use the value of var_name
("my_variable") as the name of the variable to access. Therefore, the script outputs the value of my_variable
.
Setting Variables with Indirect Referencing
Setting a variable using indirect referencing follows a similar pattern. We leverage the same !
operator, but this time on the left-hand side of the assignment:
var_name="my_variable"
value="This is a test"
eval "${var_name}=\"${value}\"" #Sets my_variable to "This is a test"
echo "${my_variable}" # Output: This is a test
Here, eval
is crucial. It executes the string passed to it as a Bash command. Without eval
, Bash would try to assign "my_variable" to the variable whose name is literally "${var_name}", which would likely fail. eval
dynamically constructs the assignment command.
Important Note: While eval
is powerful, it can pose security risks if not handled carefully. Always sanitize user inputs before using them with eval
to prevent command injection vulnerabilities.
Alternative Method using Parameter Expansion
A safer and often preferred alternative avoids eval
altogether, using parameter expansion:
var_name="my_variable"
value="This is a safer test"
declare -g "$var_name"="$value" #Set my_variable to "This is a safer test"
echo "${my_variable}" # Output: This is a safer test
The declare -g
command declares a global variable. The -g
flag is optional, but crucial if you need to modify a global variable from within a function. This approach is cleaner and less vulnerable to potential security exploits compared to eval
.
Advanced Scenarios and Best Practices
Indirect referencing finds applications in various advanced scenarios:
- Dynamic array manipulation: Iterating through an array of variable names and modifying their values.
- Configuration file parsing: Reading configuration values from a file and dynamically assigning them to variables.
- Command-line argument processing: Handling options and arguments in a more flexible manner.
Best Practices:
- Prefer
declare
overeval
: Whenever possible, opt fordeclare
to minimize security risks. - Sanitize user input: Strictly validate and sanitize user-provided data before employing indirect referencing.
- Use meaningful variable names: Maintain clarity and readability by choosing descriptive variable names.
- Comment your code: Explain the logic behind your indirect referencing to improve maintainability.
By mastering indirect referencing in Bash, you elevate your scripting capabilities, enabling you to create more powerful, flexible, and efficient scripts for automation and system administration tasks. Remember to prioritize security and code readability to ensure your scripts are both effective and maintainable.
Latest Posts
Latest Posts
-
How Do You Tell The Sex Of A Bearded Dragon
May 25, 2025
-
Color Code Nissan Radio Wiring Diagram
May 25, 2025
-
Manga Where Mc Is Reincarnated A Jester
May 25, 2025
-
If I Would Then I Could
May 25, 2025
-
Hot Water Tank Pressure Relief Valve Leaking
May 25, 2025
Related Post
Thank you for visiting our website which covers about Bash Set Variable With Indirect Reference Name . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.