Bash Too Few X's In File
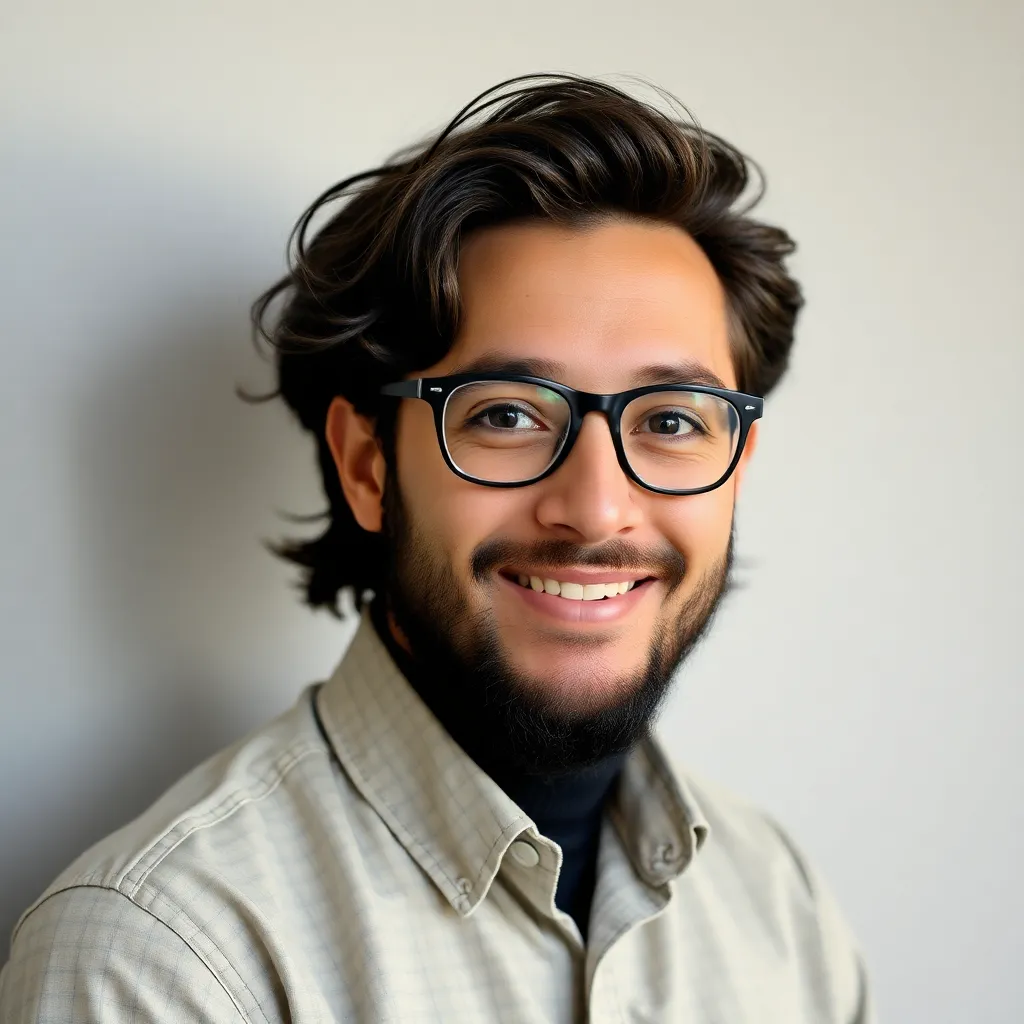
Kalali
May 23, 2025 · 4 min read
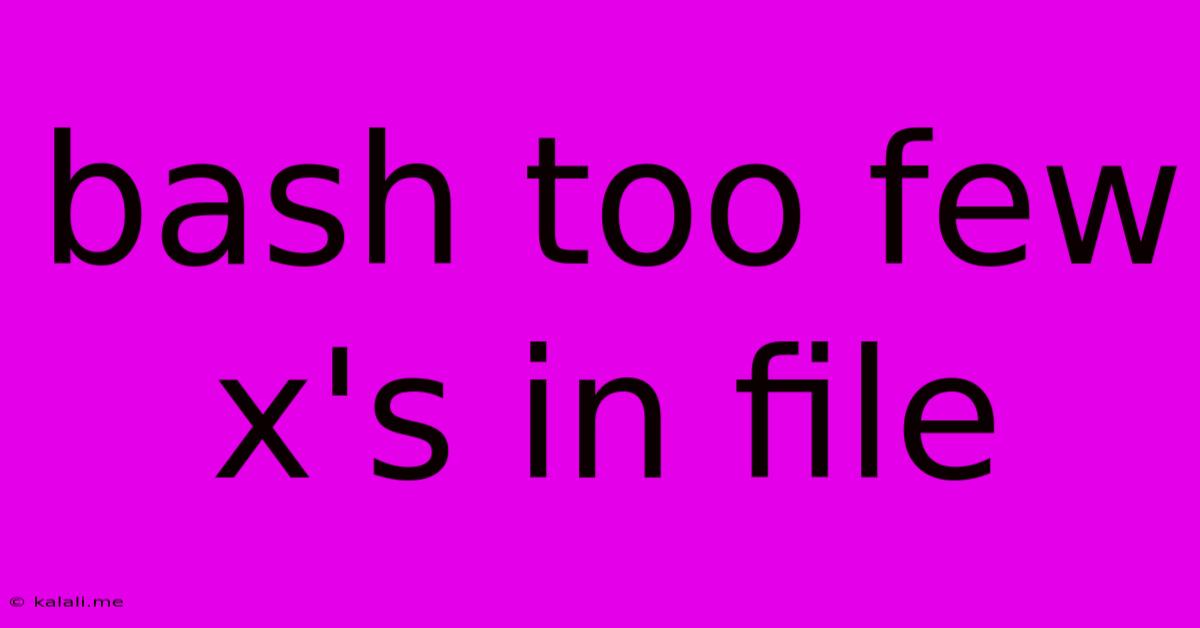
Table of Contents
Bash: Too Few Arguments in File Processing – Troubleshooting and Solutions
This article tackles a common issue faced by bash script users: the "too few arguments" error when processing files. This error, often seen as ./your_script.sh: line X: your_command: too few arguments
, arises when a command within your script expects more input than it receives. This usually happens when you're working with file input and your script isn't correctly handling cases where the expected file or data isn't present. Let's explore the root causes and how to effectively resolve them.
Understanding the Error
The core problem is a mismatch between what your script expects and what it receives. Many bash commands, especially those interacting with files (like sed
, awk
, grep
, cut
), require specific arguments. If a file is missing, empty, or doesn't contain the expected data format, these commands will fail with the "too few arguments" message. This isn't necessarily an error in your bash syntax itself; rather, it's a logical error in how your script handles file processing.
Common Scenarios and Solutions
Let's dive into the most frequent scenarios leading to this error and how to fix them:
1. Missing or Empty Input Files
- Problem: Your script assumes a file exists and attempts to process it, but the file is missing or empty.
- Solution: Implement robust error handling using
-f
(file exists) withtest
or[ ]
, and checks for file size using-s
(file size greater than zero). Here's how:
if [ -f "$INPUT_FILE" ] && [ -s "$INPUT_FILE" ]; then
# Process the file if it exists and is not empty
awk '{print $1}' "$INPUT_FILE"
else
echo "Error: Input file '$INPUT_FILE' is missing or empty." >&2
exit 1
fi
This code snippet first checks if the file exists (-f
) and then checks if its size is greater than zero (-s
). Only if both conditions are true, will the awk
command be executed. >&2
redirects error messages to standard error.
2. Incorrect File Paths or Names
- Problem: You might have a typo in the file path or use an incorrect file name as input to your commands.
- Solution: Double-check your file paths carefully. Use absolute paths (
/path/to/your/file.txt
) to avoid ambiguity. Consider using variable assignments for file paths to make your script more readable and maintainable.
3. Unexpected Data Format in Input Files
- Problem: Your script relies on a specific data format within the input file (e.g., comma-separated values, specific column structure), but the file doesn't adhere to this format. Commands expecting certain delimiters will fail if these are missing.
- Solution: Use input validation to check the file contents. For instance, you might count the number of lines or columns to ensure the file meets your script's expectations. Consider using tools like
head
orwc
to inspect the file before processing. Regular expressions can be particularly helpful in validating data formats.
4. Forgetting Arguments in Loops
- Problem: When iterating through files in a loop, you might accidentally omit necessary arguments within the loop body.
- Solution: Carefully review your loop structure. Ensure that the arguments you pass to commands inside the loop are correctly supplied for each iteration. Debugging using
echo
statements to print the values of variables passed to commands can be extremely helpful.
5. Incorrect Command Usage
- Problem: It's possible that the command itself is being used incorrectly, regardless of the file input. Refer to the command's manual page (
man command_name
) to ensure you understand its arguments and usage. - Solution: Carefully consult the manual page (
man command_name
) for the specific command giving the error. Pay close attention to the required and optional arguments.
Best Practices for Robust Scripting
- Always validate input: Check for file existence, size, and data format before processing.
- Use descriptive variable names: Makes your code easier to understand and debug.
- Implement proper error handling: Catch errors gracefully and provide informative error messages.
- Use comments generously: Clearly explain the purpose of each section of your script.
- Test thoroughly: Run your script with various inputs, including edge cases (empty files, unexpected formats).
By implementing these strategies, you can significantly reduce the likelihood of encountering the "too few arguments" error and create more robust and reliable bash scripts. Remember, proactive error handling is key to building successful and efficient file processing scripts.
Latest Posts
Latest Posts
-
How Many Cups Are In 3 Quarts Of Water
Jun 30, 2025
-
25 Cents A Minute For An Hour
Jun 30, 2025
-
In Music What Does Allegro Mean Math Answer Key Pdf
Jun 30, 2025
-
What Is 1 5 Of A Tablespoon
Jun 30, 2025
-
How Long Does It Take To Drive Through Illinois
Jun 30, 2025
Related Post
Thank you for visiting our website which covers about Bash Too Few X's In File . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.