Building Rotos From Axis And Angle Quaternion
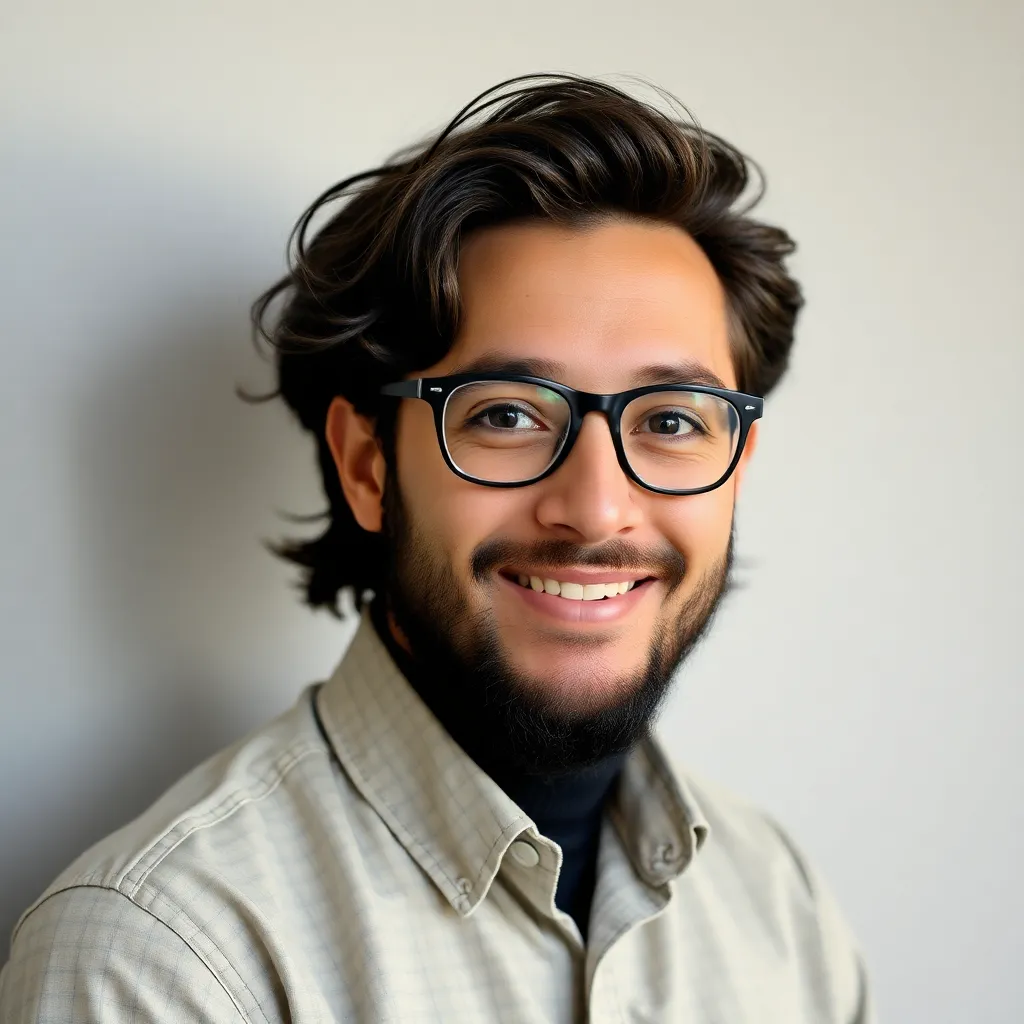
Kalali
May 23, 2025 · 3 min read
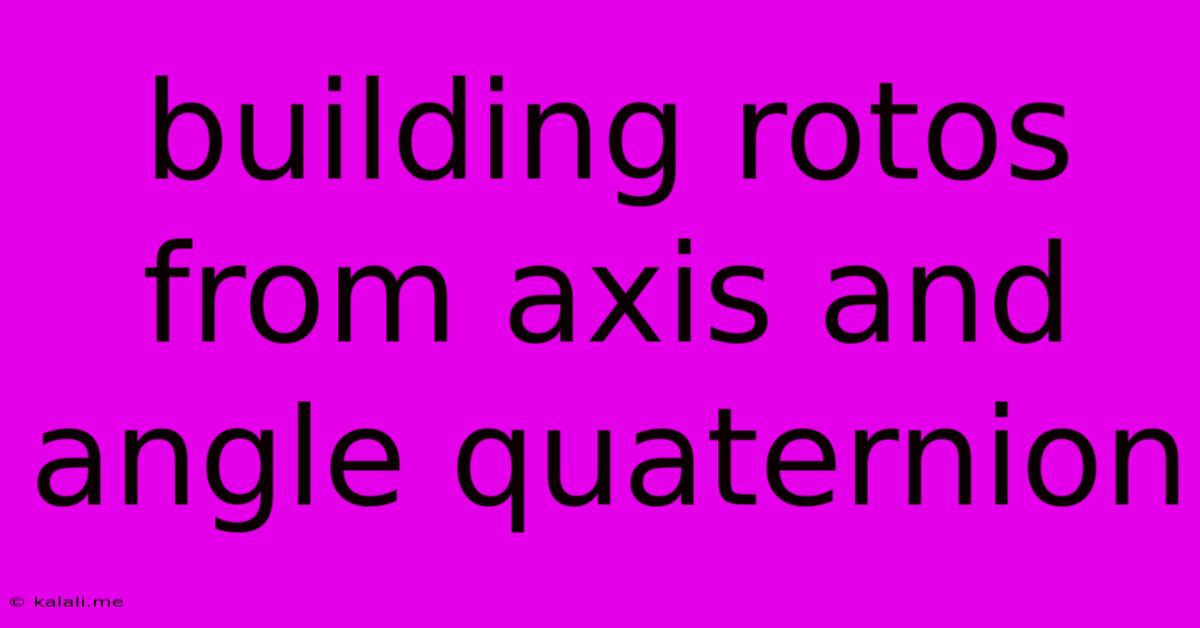
Table of Contents
Building Rotations from Axis and Angle: A Quaternion Approach
Understanding rotations is crucial in various fields, from 3D graphics and game development to robotics and aerospace engineering. While there are several ways to represent rotations (e.g., rotation matrices, Euler angles), quaternions offer a particularly elegant and efficient solution, especially when dealing with complex rotations or chained rotations. This article delves into how to construct a quaternion representing a rotation given an axis and an angle, explaining the underlying mathematics and providing practical examples. This method avoids the issues of gimbal lock often associated with Euler angles.
What are Quaternions?
Quaternions are extensions of complex numbers, expressed as: q = w + xi + yj + zk
, where w
, x
, y
, and z
are real numbers, and i
, j
, and k
are imaginary units with the properties: i² = j² = k² = ijk = -1
. In the context of rotations, quaternions provide a compact and efficient way to represent rotations in three-dimensional space. They avoid the complexities and potential singularities of other rotation representations.
Deriving the Quaternion from Axis and Angle
Given a rotation axis represented by a unit vector v = (x, y, z) and a rotation angle θ (in radians), the corresponding quaternion q
is calculated as follows:
q = cos(θ/2) + sin(θ/2)(xi + yj + zk)
This formula elegantly combines the axis and angle information into a single quaternion. Let's break it down:
cos(θ/2)
: This is the scalar part (w) of the quaternion.sin(θ/2)(xi + yj + zk)
: This is the vector part (xi + yj + zk) of the quaternion, scaled bysin(θ/2)
. The vector part directly corresponds to the rotation axis.
Example: Rotating 90 degrees around the Z-axis
Let's consider a rotation of 90 degrees (π/2 radians) around the positive Z-axis. Our axis vector v is (0, 0, 1), and θ = π/2.
- Calculate
cos(θ/2)
:cos(π/4) ≈ 0.707
- Calculate
sin(θ/2)
:sin(π/4) ≈ 0.707
- Construct the quaternion:
q ≈ 0.707 + 0.707k
Therefore, the quaternion representing this rotation is approximately q = 0.707 + 0.0i + 0.0j + 0.707k
.
Implementing in Code (Conceptual)
While specific implementation details vary depending on the programming language and library used, the core logic remains consistent. A function to create a quaternion from axis and angle would generally look like this:
import math
def quaternion_from_axis_angle(axis, angle):
"""Creates a quaternion from a rotation axis and angle."""
axis = normalize(axis) #Ensure axis is a unit vector
half_angle = angle / 2.0
sin_half_angle = math.sin(half_angle)
cos_half_angle = math.cos(half_angle)
return [cos_half_angle, axis[0] * sin_half_angle, axis[1] * sin_half_angle, axis[2] * sin_half_angle]
def normalize(v):
norm = math.sqrt(sum(x**2 for x in v))
return [x/norm for x in v] if norm > 0 else v
Applications and Further Exploration
This method of constructing quaternions is fundamental in various applications:
- 3D Game Development: Character animation, object manipulation, and camera control.
- Robotics: Robot arm kinematics, orientation tracking, and motion planning.
- Computer Graphics: Modeling, rendering, and animation.
- Virtual Reality (VR) and Augmented Reality (AR): Head tracking and object manipulation.
Further exploration could involve understanding quaternion multiplication for combining rotations, quaternion normalization for maintaining accuracy, and converting quaternions to other rotation representations like rotation matrices. Understanding these concepts provides a powerful toolkit for handling rotations efficiently and elegantly.
Latest Posts
Latest Posts
-
How Do I Send An Evite Reminder
Jul 15, 2025
-
When Performing A Self Rescue When Should You Swim To Shore
Jul 15, 2025
-
How Many Decaliters Are In A Liter
Jul 15, 2025
-
What Note Sits In The Middle Of The Grand Staff
Jul 15, 2025
-
Did Lynette Shave Her Head In Real Life
Jul 15, 2025
Related Post
Thank you for visiting our website which covers about Building Rotos From Axis And Angle Quaternion . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.