C Recast Uint8 To Array Of Int32
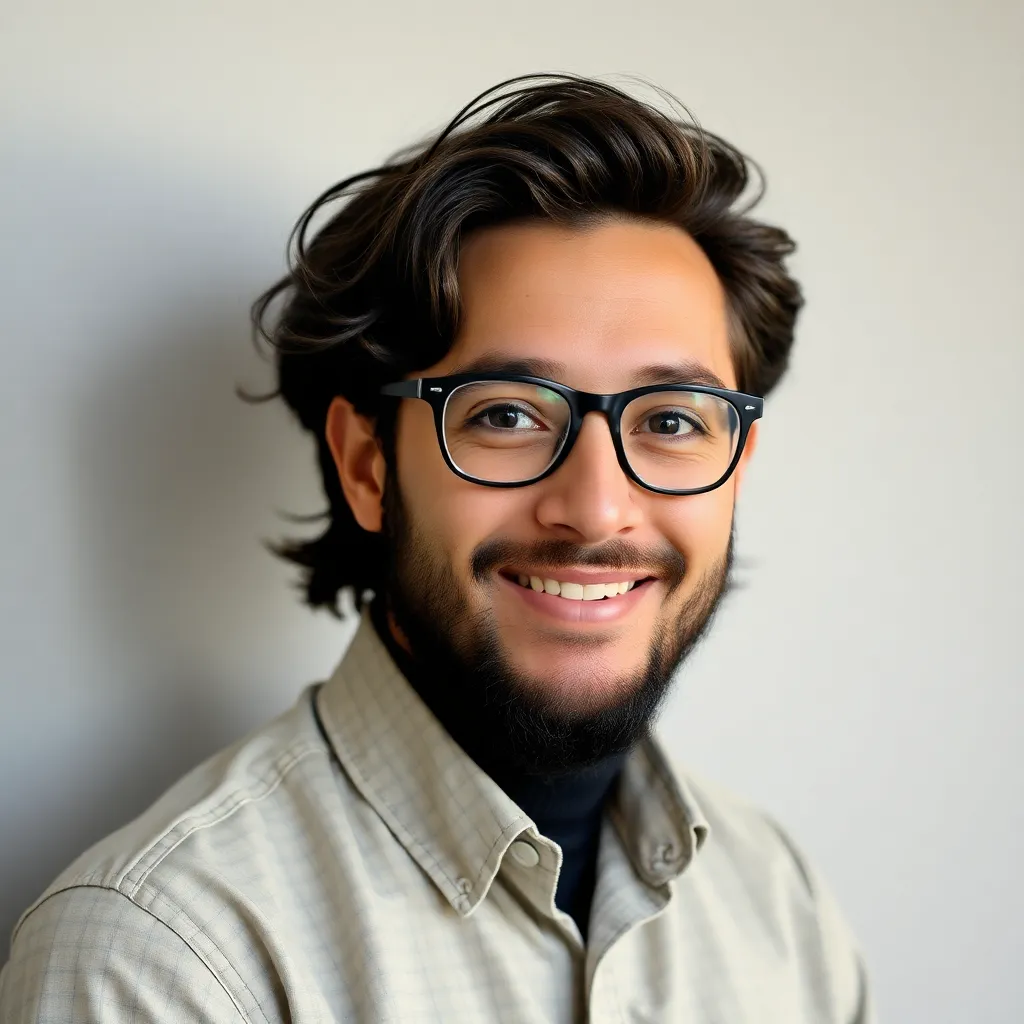
Kalali
May 24, 2025 · 3 min read
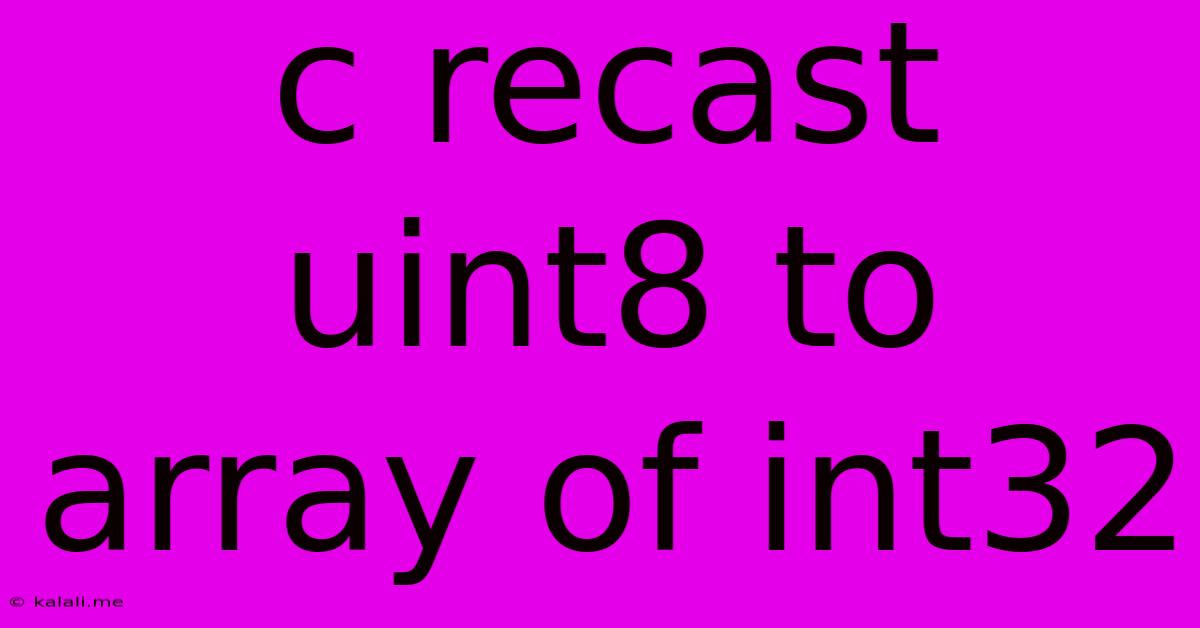
Table of Contents
C: Recasting uint8 to an Array of int32
This article details how to recast a uint8_t
array into an array of int32_t
in C, explaining the process, potential pitfalls, and best practices. Understanding this conversion is crucial for tasks involving data manipulation, image processing, and working with various data formats. We'll cover both direct casting and safer, more robust methods.
Understanding the Data Types
Before diving into the conversion, let's understand the data types involved:
-
uint8_t
: An unsigned 8-bit integer. It represents values from 0 to 255. This is often used for representing bytes or individual color components in images. -
int32_t
: A signed 32-bit integer. It represents a much wider range of values, typically from -2,147,483,648 to 2,147,483,647.
The core challenge lies in the differing sizes and signedness of these types. A naive approach might lead to unexpected results or data loss.
Methods for Recasting uint8_t
to int32_t
There are several ways to achieve this conversion, each with its own advantages and disadvantages:
1. Direct Casting (Potentially Risky)
The simplest, but potentially least safe, method involves direct casting:
#include
#include
int main() {
uint8_t uint8_array[] = {10, 20, 30, 40, 50};
size_t array_size = sizeof(uint8_array) / sizeof(uint8_array[0]);
// Direct casting - Potentially risky due to potential sign extension issues if not handled properly.
int32_t *int32_array = (int32_t *)uint8_array;
for (size_t i = 0; i < array_size; i++) {
printf("int32_array[%zu]: %d\n", i, int32_array[i]);
}
return 0;
}
Caution: This method simply reinterprets the memory occupied by uint8_array
as an array of int32_t
. While seemingly simple, this can lead to issues if the underlying architecture uses different endianness or if you misinterpret the resulting data. It's generally not recommended for robust code.
2. Safe Conversion using a Loop
A much safer and more controlled approach involves iterating through the uint8_t
array and explicitly converting each element:
#include
#include
int main() {
uint8_t uint8_array[] = {10, 20, 30, 40, 50, 255};
size_t array_size = sizeof(uint8_array) / sizeof(uint8_array[0]);
int32_t int32_array[array_size];
for (size_t i = 0; i < array_size; i++) {
int32_array[i] = (int32_t)uint8_array[i]; //Explicit conversion
}
for (size_t i = 0; i < array_size; i++) {
printf("int32_array[%zu]: %d\n", i, int32_array[i]);
}
return 0;
}
This method avoids the potential pitfalls of direct casting, providing a clear and controlled conversion process. This is the recommended approach for most scenarios.
3. Handling Larger Arrays and Memory Allocation
For very large arrays, allocating memory for the int32_t
array dynamically improves memory management:
#include
#include
#include
int main() {
uint8_t uint8_array[] = {10, 20, 30, 40, 50, 255};
size_t array_size = sizeof(uint8_array) / sizeof(uint8_array[0]);
int32_t *int32_array = (int32_t *)malloc(array_size * sizeof(int32_t));
if (int32_array == NULL) {
fprintf(stderr, "Memory allocation failed!\n");
return 1; // Indicate failure.
}
for (size_t i = 0; i < array_size; i++) {
int32_array[i] = (int32_t)uint8_array[i];
}
// ... (rest of your code using int32_array) ...
free(int32_array); // Crucial: Free the allocated memory
return 0;
}
Remember to always free dynamically allocated memory using free()
to prevent memory leaks.
Conclusion
While direct casting offers a concise solution, the safer and more reliable approach involves explicit type conversion within a loop. For large datasets, dynamic memory allocation is essential. Choosing the right method depends on the context and your prioritization of code brevity versus robustness. Always prioritize clear, maintainable code over overly compact solutions when dealing with data type conversions.
Latest Posts
Latest Posts
-
How To Repair Scratched Car Wheels
May 24, 2025
-
What Causes Ac To Freeze Up
May 24, 2025
-
How To Run For Loop In Parallel Python
May 24, 2025
-
Can You Brine A Frozen Turkey
May 24, 2025
-
How To Stop Glasses From Sliding Down Nose
May 24, 2025
Related Post
Thank you for visiting our website which covers about C Recast Uint8 To Array Of Int32 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.