Camera Based On Quaternion In C Opengl
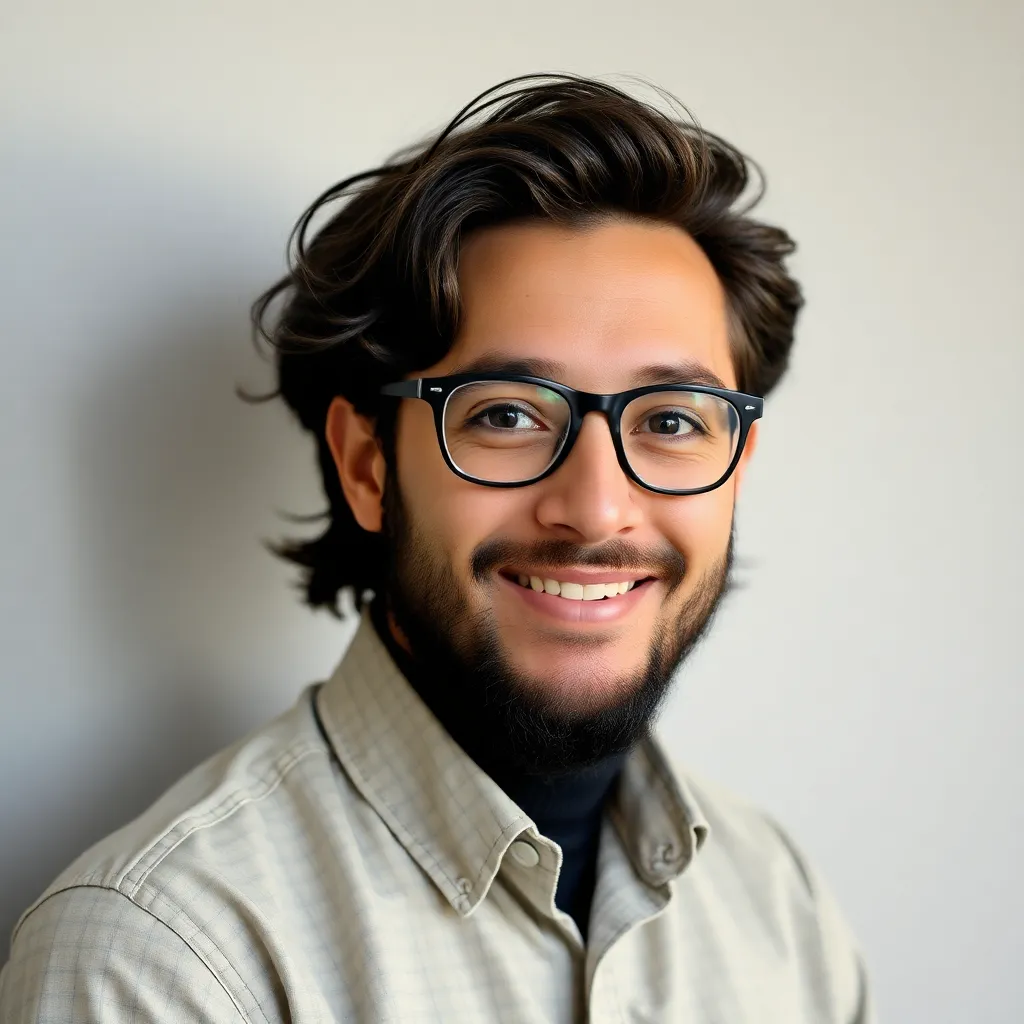
Kalali
May 24, 2025 · 3 min read
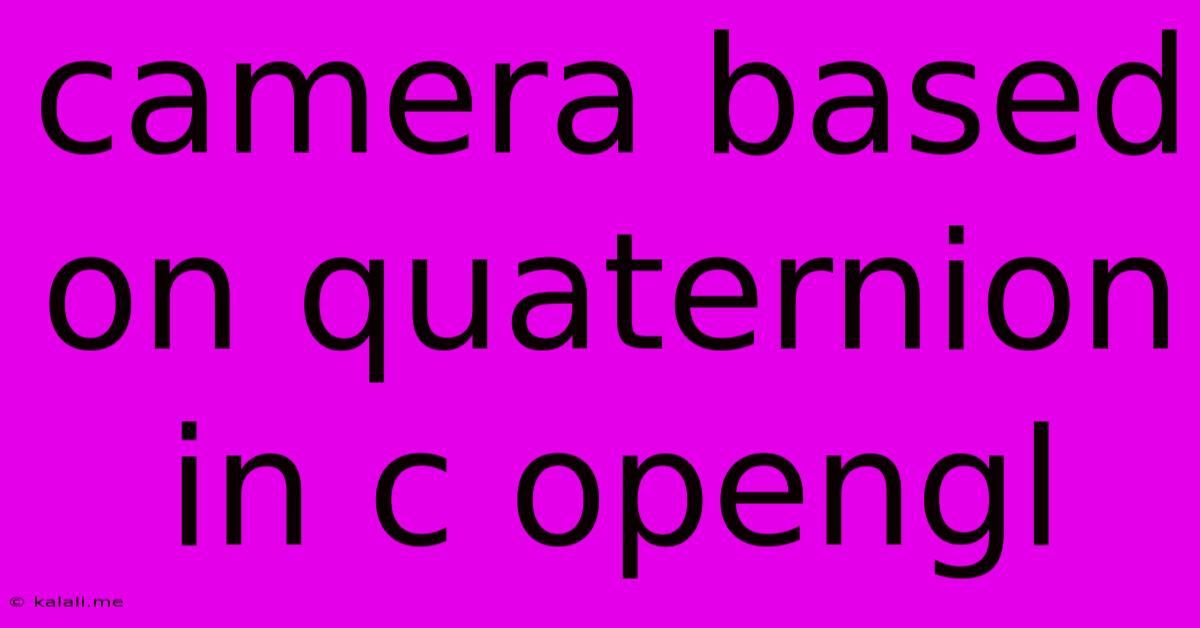
Table of Contents
Camera Control using Quaternions in C++ OpenGL
This article explores the implementation of a camera system in C++ using OpenGL, leveraging the power of quaternions for intuitive and efficient rotation control. Quaternions offer significant advantages over Euler angles, particularly in avoiding gimbal lock and providing smooth, predictable camera movements. This will provide a comprehensive guide, from understanding the fundamentals to implementing a fully functional camera class.
Understanding Quaternions and their Advantages
Before diving into the code, it's crucial to grasp the basics of quaternions. They are a way of representing rotations in four-dimensional space, effectively encoding both the axis and angle of rotation. Unlike Euler angles (roll, pitch, yaw), quaternions prevent gimbal lock – a situation where two axes of rotation become aligned, resulting in a loss of one degree of freedom. This makes quaternions ideal for smooth and reliable camera manipulation in 3D environments. Furthermore, quaternion interpolation (slerping) provides elegant solutions for smooth camera transitions between different orientations.
Implementing the Quaternion Camera Class in C++
We'll create a Camera
class that manages camera position, orientation (using a quaternion), and projection. This class will encapsulate the necessary functions for camera control and rendering.
#include
#include
#include
#include // For converting Euler angles to quaternions (optional)
class Camera {
public:
glm::vec3 position;
glm::quat orientation;
glm::mat4 viewMatrix;
float fov;
float aspectRatio;
float nearPlane;
float farPlane;
Camera(glm::vec3 pos, glm::vec3 target, float fov, float aspectRatio, float nearPlane, float farPlane) :
position(pos), fov(fov), aspectRatio(aspectRatio), nearPlane(nearPlane), farPlane(farPlane) {
//Calculate initial orientation looking at target
glm::vec3 forward = glm::normalize(target - position);
glm::vec3 up = glm::vec3(0.0f, 1.0f, 0.0f);
glm::vec3 right = glm::cross(forward, up);
up = glm::cross(right, forward);
orientation = glm::quatLookAt(forward, up);
updateViewMatrix();
}
void updateViewMatrix() {
viewMatrix = glm::mat4_cast(orientation) * glm::translate(glm::mat4(1.0f), -position);
}
void rotate(glm::quat rotation) {
orientation = rotation * orientation; //Pre-multiply for correct order
updateViewMatrix();
}
//Example functions for rotating around specific axes using Euler angles converted to Quaternions.
void rotateX(float angle) {
rotate(glm::quat(glm::radians(angle), glm::vec3(1.0f, 0.0f, 0.0f)));
}
void rotateY(float angle) {
rotate(glm::quat(glm::radians(angle), glm::vec3(0.0f, 1.0f, 0.0f)));
}
void rotateZ(float angle) {
rotate(glm::quat(glm::radians(angle), glm::vec3(0.0f, 0.0f, 1.0f)));
}
glm::mat4 getProjectionMatrix(){
return glm::perspective(glm::radians(fov), aspectRatio, nearPlane, farPlane);
}
};
Integrating with OpenGL Rendering Pipeline
Once you have your Camera
class, integrating it into your OpenGL rendering loop is straightforward. You'll use the viewMatrix
and the projection matrix (obtained from getProjectionMatrix()
) within your shader's mvp
matrix calculation (Model-View-Projection):
//In your rendering loop:
glm::mat4 mvp = camera.getProjectionMatrix() * camera.viewMatrix * modelMatrix; //modelMatrix is your object's model matrix
//Pass mvp to your shader
glUniformMatrix4fv(mvpUniformLocation, 1, GL_FALSE, glm::value_ptr(mvp));
Handling User Input for Camera Control
You would then use user input (keyboard or mouse) to manipulate the camera. For example, WASD keys could control movement, and mouse movements could control rotation using rotateX
, rotateY
, rotateZ
functions or direct quaternion manipulation. Remember to handle mouse sensitivity and potentially implement smoothing techniques for a more polished feel.
This example provides a robust foundation. You can extend this by adding features like camera zooming, more sophisticated movement controls (e.g., spherical coordinates), and more elegant input handling. Remember to include the necessary GLM (OpenGL Mathematics) library for vector and quaternion operations. This framework allows for smooth, gimbal-lock-free camera control in your OpenGL applications, enhancing user experience and creating more immersive 3D environments.
Latest Posts
Latest Posts
-
How Do You Secure A Dishwasher To Granite Countertops
May 24, 2025
-
Does Cutting Hair Make It Grow Faster
May 24, 2025
-
Books On Mathematics In The Islamic Golden Age
May 24, 2025
-
Fallout 4 General Store Not Working
May 24, 2025
-
How Do I Get Dog Urine Smell Out Of Carpet
May 24, 2025
Related Post
Thank you for visiting our website which covers about Camera Based On Quaternion In C Opengl . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.