Checbox.com Pass Variable To Url Variable
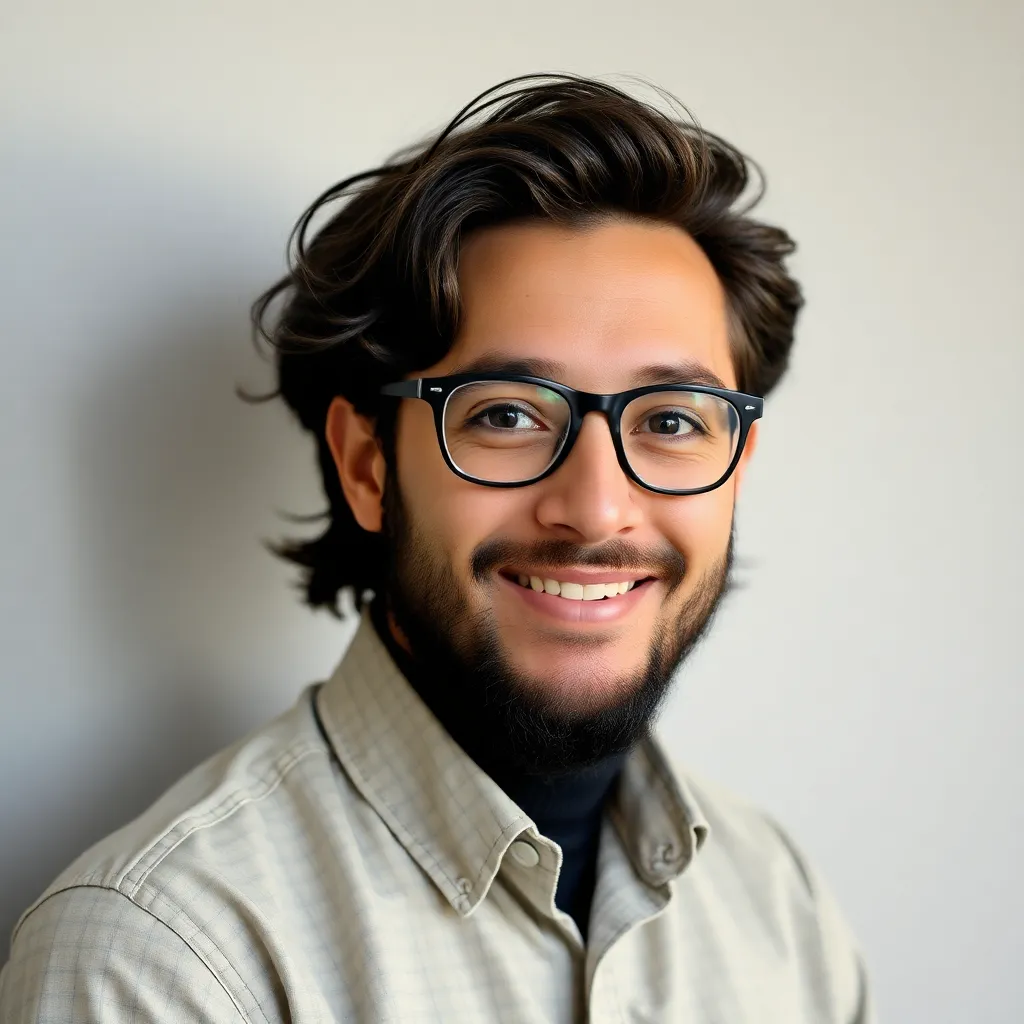
Kalali
May 25, 2025 · 3 min read
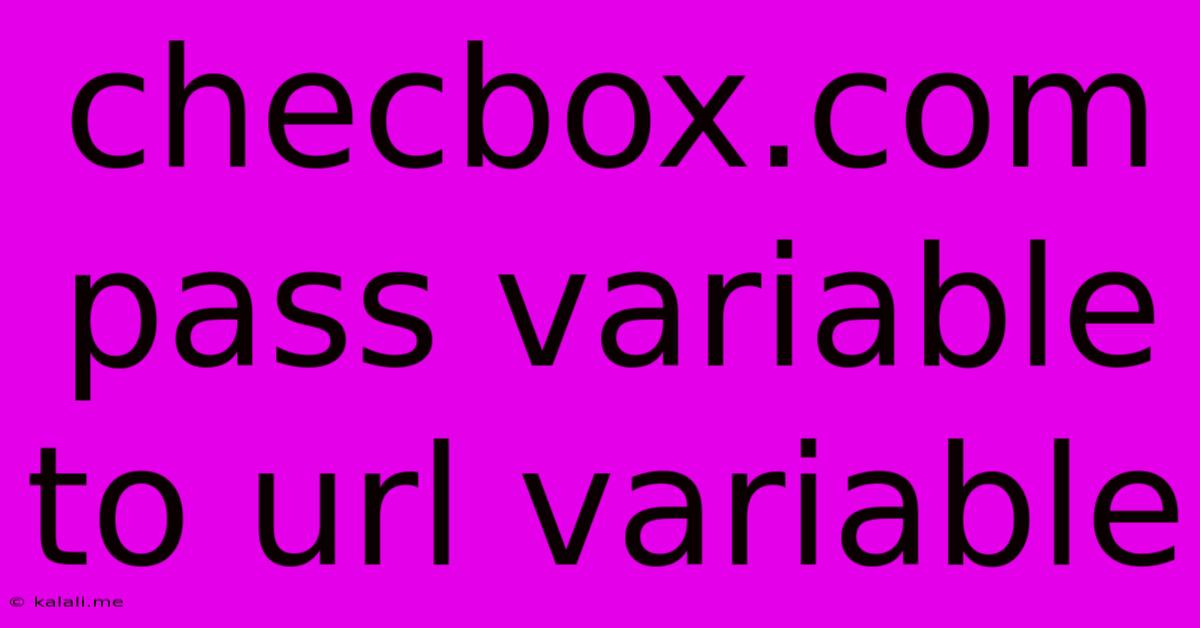
Table of Contents
Passing Checkbox Values to a URL with checkbox.com
This article explains how to pass the value of a checkbox selected using checkbox.com to a URL variable. This is a common task in web development, allowing you to dynamically update a URL based on user interaction, which is useful for filtering, sorting, or customizing web pages. While checkbox.com itself doesn't directly offer a built-in function for this, we can achieve this using simple JavaScript. This method is adaptable regardless of the specific checkbox library used.
Understanding the Problem and Solution:
The core issue is capturing the state (checked or unchecked) of a checkbox and incorporating that information into a URL string. The solution involves using JavaScript's addEventListener
to detect changes in the checkbox's state, then constructing and updating the URL accordingly. This updated URL can then be used to redirect the user or to submit a form.
Implementing the Solution:
Let's assume you have a checkbox with the ID myCheckbox
. We'll use JavaScript to listen for changes to this checkbox's checked
property. Here's the code:
const checkbox = document.getElementById('myCheckbox');
const urlBase = "https://yourwebsite.com/page?filter="; // Replace with your base URL
checkbox.addEventListener('change', function() {
let url = urlBase;
if (this.checked) {
url += "true"; // Or any value representing "checked"
} else {
url += "false"; // Or any value representing "unchecked"
}
window.location.href = url; // Redirect to the updated URL
});
Explanation:
-
document.getElementById('myCheckbox')
: This line retrieves a reference to the checkbox element with the ID "myCheckbox". Replace'myCheckbox'
with the actual ID of your checkbox. -
addEventListener('change', function(){...})
: This sets up an event listener that triggers a function whenever the checkbox's state changes (i.e., when it's checked or unchecked). -
let url = urlBase;
: This initializes a variableurl
with your base URL. Replace"https://yourwebsite.com/page?filter="
with your actual base URL and the parameter name you want to use. -
if (this.checked) { ... } else { ... }
: This conditional statement checks the checkbox'schecked
property. If it's checked, it appends "true" (or your chosen value) to the URL; otherwise, it appends "false". -
window.location.href = url;
: This line redirects the browser to the updated URL.
Advanced Techniques:
-
Multiple Checkboxes: For multiple checkboxes, you'll need to loop through each checkbox, checking its state and appending its value to the URL. You might use a delimiter (e.g., comma) to separate the values.
-
More Complex Values: Instead of just "true" and "false", you can pass more meaningful values. For example, if you have checkboxes representing different categories, you could append the category name to the URL.
-
Using a Form: Instead of directly redirecting, you could use this JavaScript to populate a hidden form field and submit the form. This offers more flexibility, especially for complex scenarios or when you need to send other data along with the checkbox value.
Example with Multiple Checkboxes:
const checkboxes = document.querySelectorAll('input[type="checkbox"]');
const urlBase = "https://yourwebsite.com/page?categories=";
checkboxes.forEach(checkbox => {
checkbox.addEventListener('change', function() {
let selectedCategories = [];
checkboxes.forEach(cb => {
if (cb.checked) {
selectedCategories.push(cb.value);
}
});
window.location.href = urlBase + selectedCategories.join(',');
});
});
Remember to replace placeholder values with your actual IDs, URLs, and values. This comprehensive approach provides a robust solution for handling checkbox values in URL parameters, greatly improving user experience and website functionality. Always remember to test your implementation thoroughly to ensure it works as expected.
Latest Posts
Latest Posts
-
How To Remove Stickers From Car
May 25, 2025
-
Do Not Grieve The Holy Spirit
May 25, 2025
-
Email Or E Mail Or Email
May 25, 2025
-
Whole Wheat Flour Vs Whole Grain Flour
May 25, 2025
-
How To Get Rid Of Silverfish Bugs
May 25, 2025
Related Post
Thank you for visiting our website which covers about Checbox.com Pass Variable To Url Variable . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.