Check If A File Exists Bash
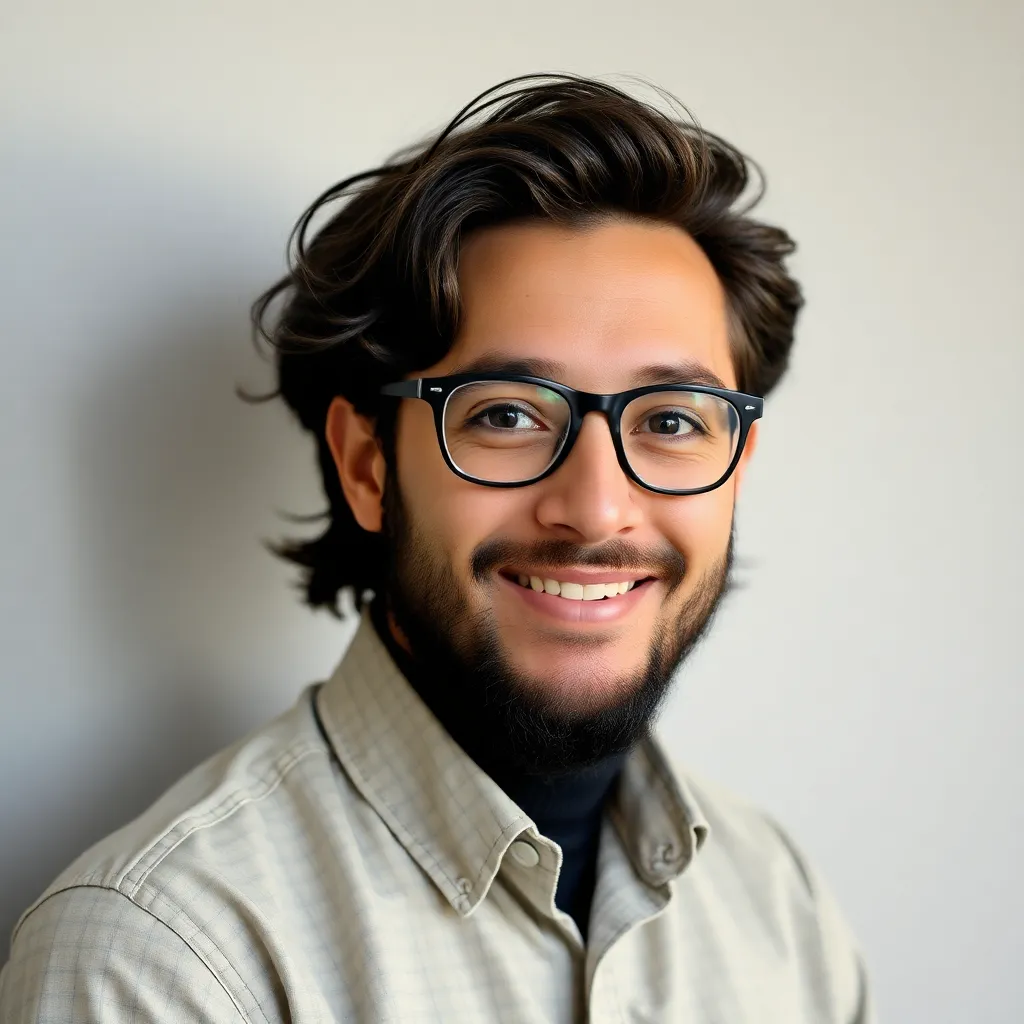
Kalali
May 19, 2025 · 3 min read
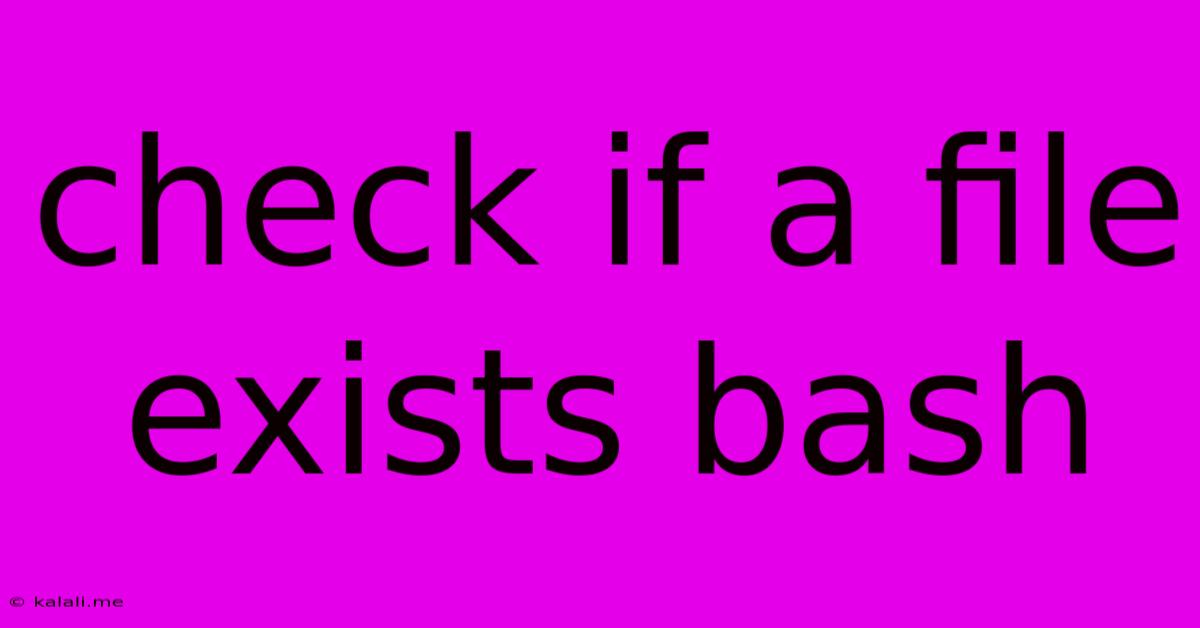
Table of Contents
Checking if a File Exists in Bash: A Comprehensive Guide
This guide provides several methods to check if a file exists in a Bash script, covering different scenarios and offering best practices for robust code. Knowing how to reliably verify file existence is crucial for any scripting task, preventing errors and ensuring your scripts run smoothly. This article will explore various approaches, ranging from simple checks to more advanced techniques handling symbolic links and permissions.
Method 1: The -f
Test Operator
The simplest and most common way to check for a file's existence is using the -f
test operator within an if
statement. This operator returns true only if the specified file exists and is a regular file (not a directory or other special file type).
if [ -f "/path/to/your/file.txt" ]; then
echo "File exists!"
else
echo "File does not exist!"
fi
Replace /path/to/your/file.txt
with the actual path to your file. Remember to enclose the path in quotes, especially if it contains spaces. This method is efficient and widely used for its clarity.
Method 2: The [[ ]]
Operator (More Robust)
While the [ ]
(or test
) command works well, the [[ ]]
operator provides enhanced features, including better handling of special characters within filenames. It's generally recommended for more robust scripting.
if [[ -f "/path/to/your/file.txt" ]]; then
echo "File exists!"
else
echo "File does not exist!"
fi
The functionality remains the same, but the [[ ]]
offers improved parsing, making it more resilient to potential errors caused by unusual filenames.
Method 3: Checking for File Existence and Readability
Sometimes you need to ensure not only that the file exists, but also that your script has permission to read it. You can combine the -f
test with the -r
test (checking for read permissions).
if [[ -f "/path/to/your/file.txt" && -r "/path/to/your/file.txt" ]]; then
echo "File exists and is readable!"
else
echo "File does not exist or is not readable!"
fi
This approach adds an extra layer of security, preventing your script from failing due to insufficient permissions.
Method 4: Handling Symbolic Links
If you need to check for the existence of a file that might be a symbolic link, you'll need a slightly different approach. The -f
operator will return true even if the file is a symbolic link, but the linked file might not exist. To handle this, use the -e
operator which checks for existence regardless of file type, and then check the link status separately if needed.
file="/path/to/your/file.txt"
if [[ -e "$file" ]]; then
if [[ -L "$file" ]]; then
if [[ -e "$(readlink "$file")" ]]; then
echo "Symbolic link exists and points to an existing file."
else
echo "Symbolic link exists but points to a non-existent file."
fi
else
echo "Regular file exists."
fi
else
echo "File or symbolic link does not exist."
fi
This more complex example provides more granular control when dealing with symbolic links.
Best Practices and Considerations
- Always quote variables: This prevents word splitting and globbing issues, especially when dealing with filenames containing spaces or special characters.
- Use the
[[ ]]
operator: It offers better parsing and error handling compared to the[ ]
operator. - Consider file permissions: Check for readability (
-r
) or other permissions as needed, to avoid unexpected errors. - Handle symbolic links carefully: Use the
-L
andreadlink
commands for accurate checks when dealing with symbolic links. - Error Handling: Include
else
blocks to handle scenarios where the file doesn't exist, or permissions are insufficient. This enhances the robustness and predictability of your scripts.
By employing these methods and best practices, you can write robust and reliable Bash scripts that handle file existence checks effectively, reducing the risk of errors and improving overall script performance. Remember to always adapt your approach based on the specific needs of your script and the context of your file handling.
Latest Posts
Latest Posts
-
How Many Cups Is 1 Pound Of Cheese
Jul 12, 2025
-
30 X 30 Is How Many Square Feet
Jul 12, 2025
-
How Much Does A Half Oz Weigh
Jul 12, 2025
-
Calories In An Omelette With 3 Eggs
Jul 12, 2025
-
How Do You Say Great Grandmother In Spanish
Jul 12, 2025
Related Post
Thank you for visiting our website which covers about Check If A File Exists Bash . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.