Convert Deciaml To Integer In Apex
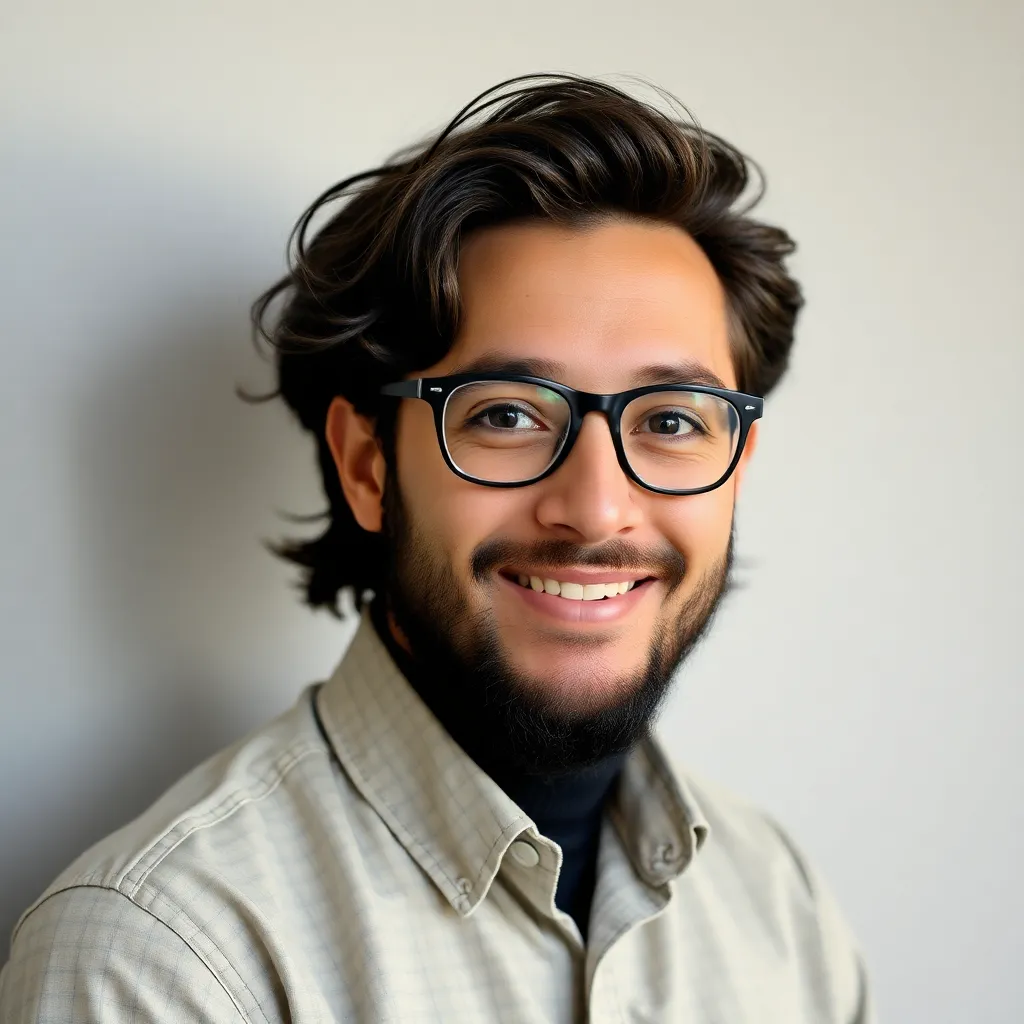
Kalali
May 23, 2025 · 3 min read
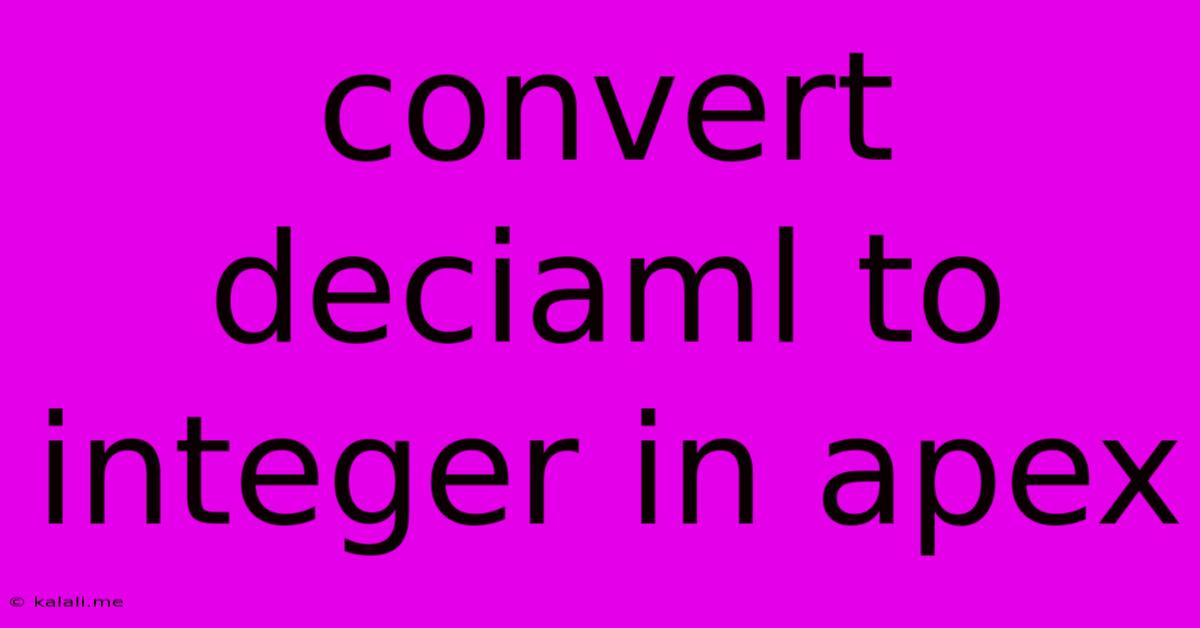
Table of Contents
Converting Decimal to Integer in Apex: A Comprehensive Guide
This article provides a detailed explanation of how to convert Decimal values to Integer values in Apex, a programming language used in Salesforce. We'll explore various methods, discuss their implications, and offer best practices for ensuring data integrity and efficient code. Understanding this conversion is crucial for many Apex development tasks, particularly when working with data types that require whole numbers.
Understanding Decimal and Integer Data Types in Apex
Before diving into the conversion process, let's briefly review the core differences between Decimal and Integer data types:
- Decimal: Represents numbers with fractional parts (e.g., 3.14, -2.5). It offers higher precision and is suitable for calculations requiring decimal points.
- Integer: Represents whole numbers without fractional parts (e.g., 10, -5, 0). It's generally more efficient for calculations involving only whole numbers.
Converting between these types is essential when your data source provides decimals, but your processing or storage requires integers. Improper conversion can lead to data loss or unexpected errors.
Methods for Converting Decimal to Integer in Apex
Apex offers several ways to convert a Decimal value to an Integer:
1. Using the Integer.valueOf()
Method
This is the most straightforward and recommended method for converting a Decimal to an Integer. The valueOf()
method truncates the fractional part of the Decimal, effectively rounding it down towards zero.
Decimal decimalValue = 12.99;
Integer intValue = Integer.valueOf(decimalValue); // intValue will be 12
System.debug(intValue); // Output: 12
decimalValue = -5.7;
intValue = Integer.valueOf(decimalValue); // intValue will be -5
System.debug(intValue); // Output: -5
Important Note: If the Decimal value is outside the range of an Integer (-2,147,483,648 to 2,147,483,647), an exception will be thrown.
2. Casting (Type Conversion)**
While less explicit than Integer.valueOf()
, casting also works effectively:
Decimal decimalValue = 25.5;
Integer intValue = (Integer)decimalValue; // intValue will be 25
System.debug(intValue); // Output: 25
Like Integer.valueOf()
, casting truncates the fractional part. It also throws an exception for values outside the Integer range.
3. Using Math.round()
for Rounding (Not Truncation)**
If you need to round the Decimal to the nearest Integer (instead of truncating), use the Math.round()
method. This method rounds up or down depending on the fractional part.
Decimal decimalValue = 12.5;
Integer intValue = Integer.valueOf(Math.round(decimalValue)); // intValue will be 13
System.debug(intValue); // Output: 13
decimalValue = 12.4;
intValue = Integer.valueOf(Math.round(decimalValue)); // intValue will be 12
System.debug(intValue); //Output: 12
Best Practices and Considerations
- Error Handling: Always include error handling (e.g.,
try-catch
blocks) to gracefully handle potential exceptions, particularly when dealing with user input or external data sources that might contain values outside the Integer range. - Data Loss: Be aware that converting a Decimal to an Integer inherently involves potential data loss if the Decimal has a non-zero fractional part. Carefully consider whether truncation or rounding is appropriate for your specific use case.
- Code Readability: Use
Integer.valueOf()
for clarity; it explicitly communicates your intention to convert a Decimal to an Integer. - Performance: While the performance differences between these methods are usually negligible, for extremely performance-sensitive applications, benchmarking might be necessary to determine the optimal approach.
By understanding these methods and following best practices, you can confidently and effectively convert Decimal values to Integers in your Apex code, ensuring data integrity and efficient program execution. Remember to always choose the method that best suits your specific rounding and error-handling requirements.
Latest Posts
Latest Posts
-
If Your 35 What Year Was You Born
Jul 12, 2025
-
How Many Cups Is 1 Pound Of Cheese
Jul 12, 2025
-
30 X 30 Is How Many Square Feet
Jul 12, 2025
-
How Much Does A Half Oz Weigh
Jul 12, 2025
-
Calories In An Omelette With 3 Eggs
Jul 12, 2025
Related Post
Thank you for visiting our website which covers about Convert Deciaml To Integer In Apex . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.