Custom Menu Add Item Run Multiple Function
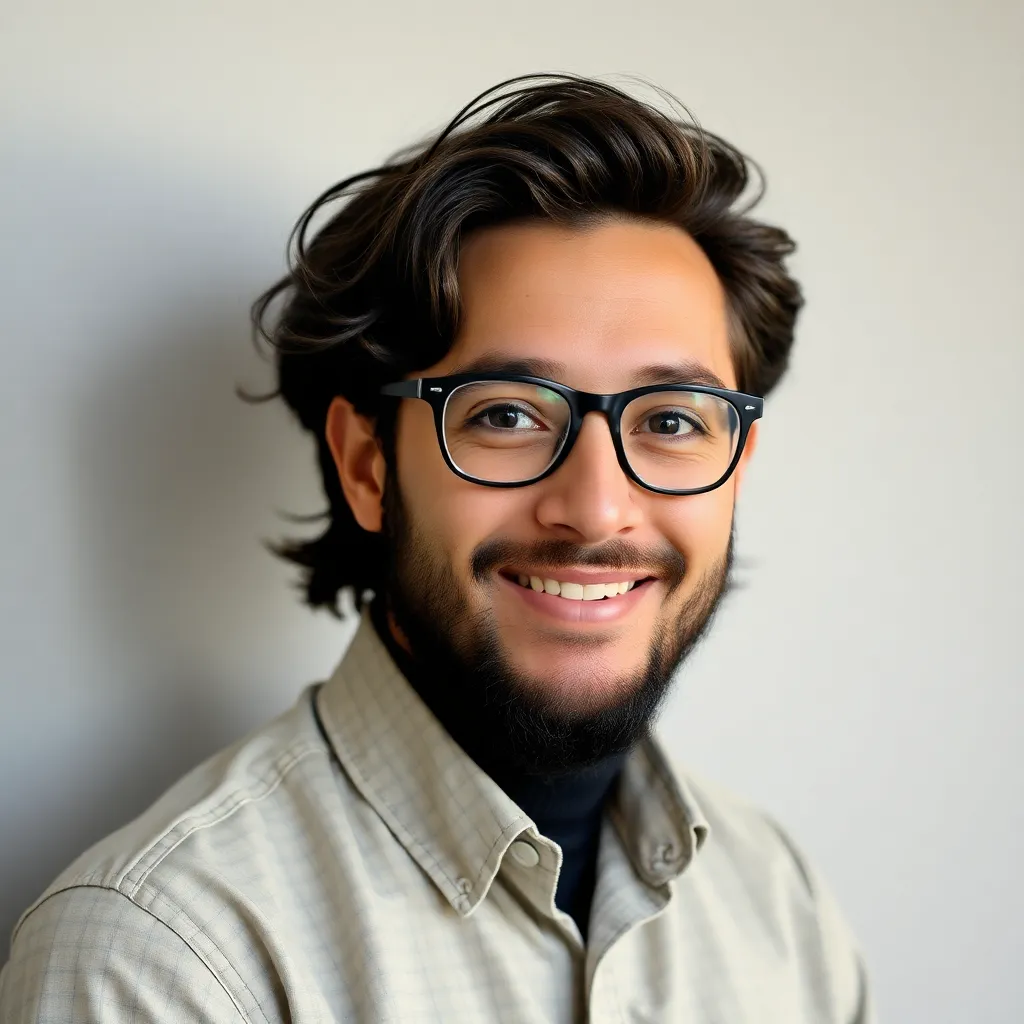
Kalali
May 24, 2025 · 3 min read
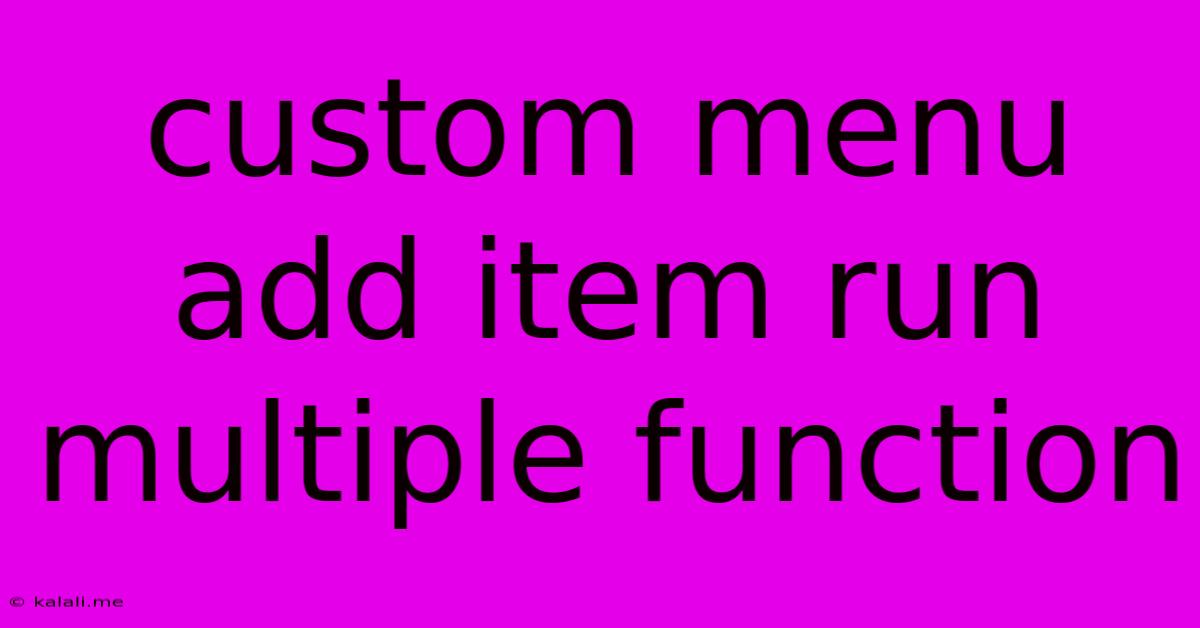
Table of Contents
Custom Menu Add Item: Running Multiple Functions in WordPress
Adding custom menu items in WordPress is a common task, often used to enhance navigation or provide quick access to specific functionalities. However, you might need a single menu item to trigger multiple actions. This article will guide you through creating a custom menu item that executes multiple functions upon clicking, enhancing your WordPress site's functionality and user experience. This approach is beneficial for streamlining your site's administration and providing a cleaner, more user-friendly interface.
This tutorial assumes a basic understanding of WordPress theme development, PHP, and the WordPress functions API. We'll explore different methods for achieving this, balancing simplicity with flexibility.
Method 1: Using a Single Function with Multiple Actions
The simplest approach involves creating a single function that encapsulates all the desired actions. This is ideal for situations where the actions are closely related and easily managed within a single function.
First, let's create the function:
function my_custom_menu_item_function() {
// Action 1: Redirect to a specific page
wp_redirect( get_permalink( 123 ) ); // Replace 123 with your page ID
exit;
// Action 2: Update a custom post meta
update_post_meta( get_the_ID(), 'my_custom_meta', 'new_value' );
// Action 3: Send an email notification (requires additional setup)
// ... your email sending code here ...
}
Next, we'll add the menu item using the add_action
hook. This hook will execute our function when the menu item is clicked.
add_action( 'admin_menu', 'add_my_custom_menu_item' );
function add_my_custom_menu_item() {
add_menu_page( 'My Custom Page', 'My Custom Menu Item', 'manage_options', 'my-custom-menu-slug', 'my_custom_menu_item_function' );
}
Remember to replace placeholders like 'my-custom-menu-slug'
with your own unique slug.
This method is concise but might become less manageable as the number of actions increases.
Method 2: Using Multiple Functions with do_action
For better organization and scalability, consider using multiple functions and the WordPress do_action
hook. This allows for a more modular approach, making it easier to maintain and extend the functionality.
First, create individual functions for each action:
function my_custom_action_1() {
// Action 1: Redirect to a page
wp_redirect( get_permalink( 123 ) );
exit;
}
function my_custom_action_2() {
// Action 2: Update custom post meta
update_post_meta( get_the_ID(), 'my_custom_meta', 'new_value' );
}
function my_custom_action_3() {
// Action 3: Send an email
// ... your email code here ...
}
Then, create a main function that uses do_action
to trigger these individual functions:
function my_custom_menu_item_function() {
do_action( 'my_custom_menu_item_actions' );
}
add_action( 'my_custom_menu_item_actions', 'my_custom_action_1' );
add_action( 'my_custom_menu_item_actions', 'my_custom_action_2' );
add_action( 'my_custom_menu_item_actions', 'my_custom_action_3' );
Finally, add the menu item as shown in Method 1, using my_custom_menu_item_function
as the callback.
This approach offers better organization and maintainability, particularly when dealing with complex actions or when different developers contribute to the code. Remember to use descriptive action hook names for clarity.
Choosing the Right Method
The best method depends on your specific needs. For simple tasks with a few related actions, Method 1 is sufficient. However, for more complex scenarios or larger projects, the modularity and maintainability of Method 2 are highly recommended. Prioritize code readability and organization for long-term success. Always thoroughly test your code after implementing these changes. Remember to replace placeholder values with your actual page IDs, meta keys, and other relevant data. Using well-named functions and hooks will greatly improve your code's clarity and maintainability.
Latest Posts
Latest Posts
-
30 X 30 Is How Many Square Feet
Jul 12, 2025
-
How Much Does A Half Oz Weigh
Jul 12, 2025
-
Calories In An Omelette With 3 Eggs
Jul 12, 2025
-
How Do You Say Great Grandmother In Spanish
Jul 12, 2025
-
What Is 873 Rounded To The Nearest Hundred
Jul 12, 2025
Related Post
Thank you for visiting our website which covers about Custom Menu Add Item Run Multiple Function . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.