Does If Represent Condition In An If Then Statement
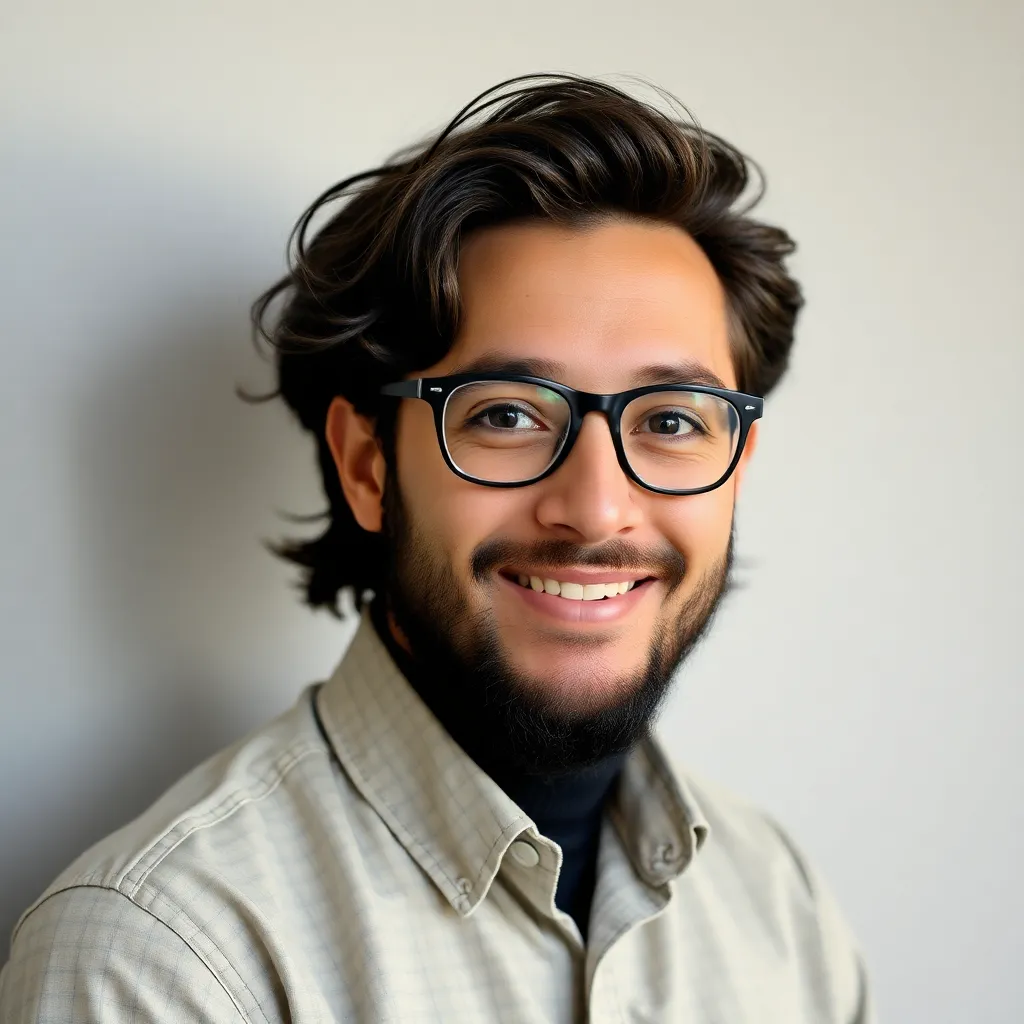
Kalali
Apr 07, 2025 · 6 min read
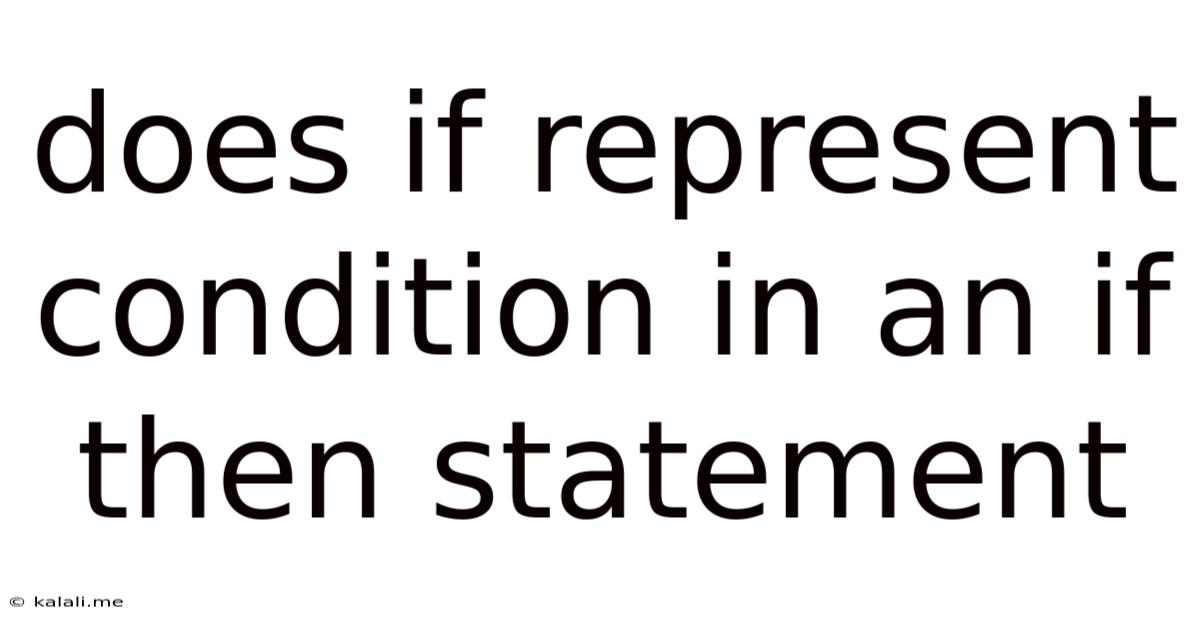
Table of Contents
Does "if" Represent a Condition in an If-Then Statement? A Deep Dive
The seemingly simple "if-then" statement is a cornerstone of programming logic. Understanding its core function, particularly the role of the "if" clause in representing a condition, is crucial for any programmer, regardless of experience level. This article dives deep into the concept, exploring its nuances, variations, and practical applications across various programming languages. We'll go beyond the basics, examining how conditions are evaluated, the different types of conditions used, and how to effectively utilize "if-then" statements for robust and efficient code.
Understanding the Core Function: Conditionals and Control Flow
At its heart, an "if-then" statement (or conditional statement) is a fundamental control flow mechanism. Control flow dictates the order in which instructions in a program are executed. Without conditional statements, programs would execute instructions sequentially, lacking the ability to make decisions or respond to different scenarios. The "if" keyword introduces a condition that determines whether a block of code (the "then" part) will be executed.
The fundamental structure is simple:
if (condition) {
// Code to execute if the condition is true
}
The crucial component is the condition
enclosed in parentheses. This condition is an expression that evaluates to either true
or false
. If the condition evaluates to true
, the code within the curly braces {}
is executed. If it evaluates to false
, the code is skipped.
The Role of "if" in Defining the Condition
The "if" keyword doesn't just introduce the conditional block; it explicitly declares that what follows is a condition that must be evaluated for truthiness. Without the "if", the following code would be meaningless:
(x > 5) { //This is invalid syntax in most languages
//This code won't execute.
}
The "if" keyword provides the necessary syntax to tell the programming language to treat the parenthesized expression as a condition. It's the essential marker that sets the stage for decision-making within the program's flow.
Types of Conditions Used in "if" Statements
The conditions within "if" statements can take various forms, including:
1. Relational Operators:
These operators compare two values and return a boolean result (true
or false
). Common relational operators include:
>
(greater than):x > 5
(true if x is greater than 5)<
(less than):y < 10
(true if y is less than 10)>=
(greater than or equal to):z >= 0
(true if z is greater than or equal to 0)<=
(less than or equal to):a <= b
(true if a is less than or equal to b)==
(equal to):p == q
(true if p is equal to q) Note: Avoid confusing==
(comparison) with=
(assignment).!=
(not equal to):m != n
(true if m is not equal to n)
2. Logical Operators:
These operators combine or modify boolean expressions:
&&
(logical AND):x > 5 && y < 10
(true only if both conditions are true)||
(logical OR):x > 5 || y < 10
(true if at least one condition is true)!
(logical NOT):! (x > 5)
(true if x is not greater than 5)
3. Equality Operators:
These specifically test for equality (or inequality) between values. While ==
is covered above, it's important to emphasize the difference between this and assignment (=
).
4. Membership Operators (Python):
Python offers membership operators (in
and not in
) that test if a value exists within a sequence (like a list or string).
in
:'a' in 'abc'
(true because 'a' is in 'abc')not in
:'d' not in 'abc'
(true because 'd' is not in 'abc')
Conditional Statements Beyond the Basic "if":
The power of conditional logic extends beyond the simple "if" statement. Many languages support:
if-else
: This allows for executing one block of code if the condition is true and a different block if it's false.
if (x > 5) {
// Code if x > 5
} else {
// Code if x <= 5
}
if-else if-else
(or chained if-else): This handles multiple conditions sequentially. The first condition that evaluates to true causes its corresponding code block to execute, and the rest are skipped.
if (x > 10) {
// Code if x > 10
} else if (x > 5) {
// Code if x > 5 but x <= 10
} else {
// Code if x <= 5
}
- Nested "if" statements: You can place "if" statements inside other "if" statements, creating complex conditional logic. This is useful for handling situations with multiple layers of conditions.
if (x > 5) {
if (y < 10) {
// Code if x > 5 and y < 10
}
}
- Switch Statements (or Case Statements): Some languages (like C++, Java, JavaScript, and others) provide switch statements, which are optimized for testing a single variable against multiple possible values. They are often more efficient than chained
if-else if
structures when dealing with many possible values.
Short-Circuiting in Logical Operators
Logical AND (&&
) and OR (||
) operators often exhibit short-circuiting behavior. This means that the second operand is only evaluated if necessary to determine the overall result.
&&
: If the first operand isfalse
, the entire expression isfalse
regardless of the second operand's value, so the second operand isn't evaluated.||
: If the first operand istrue
, the entire expression istrue
regardless of the second operand's value, so the second operand isn't evaluated.
This short-circuiting can be useful for optimizing code and avoiding potential errors (e.g., attempting to access an element of a null object).
Common Pitfalls and Best Practices
- Avoid Deep Nesting: Excessive nesting of "if" statements can make code difficult to read and maintain. Consider refactoring complex conditional logic into smaller, more manageable functions.
- Use Meaningful Variable Names: Choose descriptive names for variables used in your conditions to improve readability and understanding.
- Proper Indentation: Consistent indentation is crucial for clarity in conditional statements, particularly with nested structures. Most IDEs automatically handle indentation, but it's still a good practice to review it manually.
- Error Handling: Consider potential errors or invalid input that might affect your conditions. Add error handling (e.g., using
try-catch
blocks) to gracefully handle unexpected situations. - Test Thoroughly: Test your conditional logic extensively to ensure it behaves as expected under all possible scenarios. Use unit tests to automate this process.
- Use the Correct Equality Operator: Always use
==
for comparison and=
for assignment. This is a common source of errors for beginners.
Examples Across Different Languages
While the core concept remains the same, the syntax of "if-then" statements varies slightly across different programming languages. Here are examples demonstrating the fundamental "if" statement in a few popular languages:
Python:
x = 10
if x > 5:
print("x is greater than 5")
JavaScript:
let x = 10;
if (x > 5) {
console.log("x is greater than 5");
}
Java:
int x = 10;
if (x > 5) {
System.out.println("x is greater than 5");
}
C++:
int x = 10;
if (x > 5) {
std::cout << "x is greater than 5" << std::endl;
}
C#:
int x = 10;
if (x > 5) {
Console.WriteLine("x is greater than 5");
}
These examples highlight the consistent role of "if" in representing a condition that determines the execution flow of the code. The specific syntax may differ, but the underlying principle remains the same.
Conclusion
The "if" keyword is not merely a syntactic element; it's the crucial component that enables conditional logic, the very heart of decision-making in programming. By mastering the use of "if" statements and understanding the different types of conditions and control structures, programmers can build powerful and flexible applications that respond intelligently to various scenarios. Remember to prioritize code readability, error handling, and thorough testing to create robust and maintainable programs. The effective use of conditional statements is paramount to creating efficient, reliable, and elegant code.
Latest Posts
Latest Posts
-
How Many Square Feet In One Yard
Apr 10, 2025
-
14 Hours Is How Many Minutes
Apr 10, 2025
-
How Many Meters Is 30 Yards
Apr 10, 2025
-
How Many Inches Are In 4 Meters
Apr 10, 2025
-
How Tall Is 90 Cm In Feet
Apr 10, 2025
Related Post
Thank you for visiting our website which covers about Does If Represent Condition In An If Then Statement . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.