Edit Title When Custom Post Type Saves
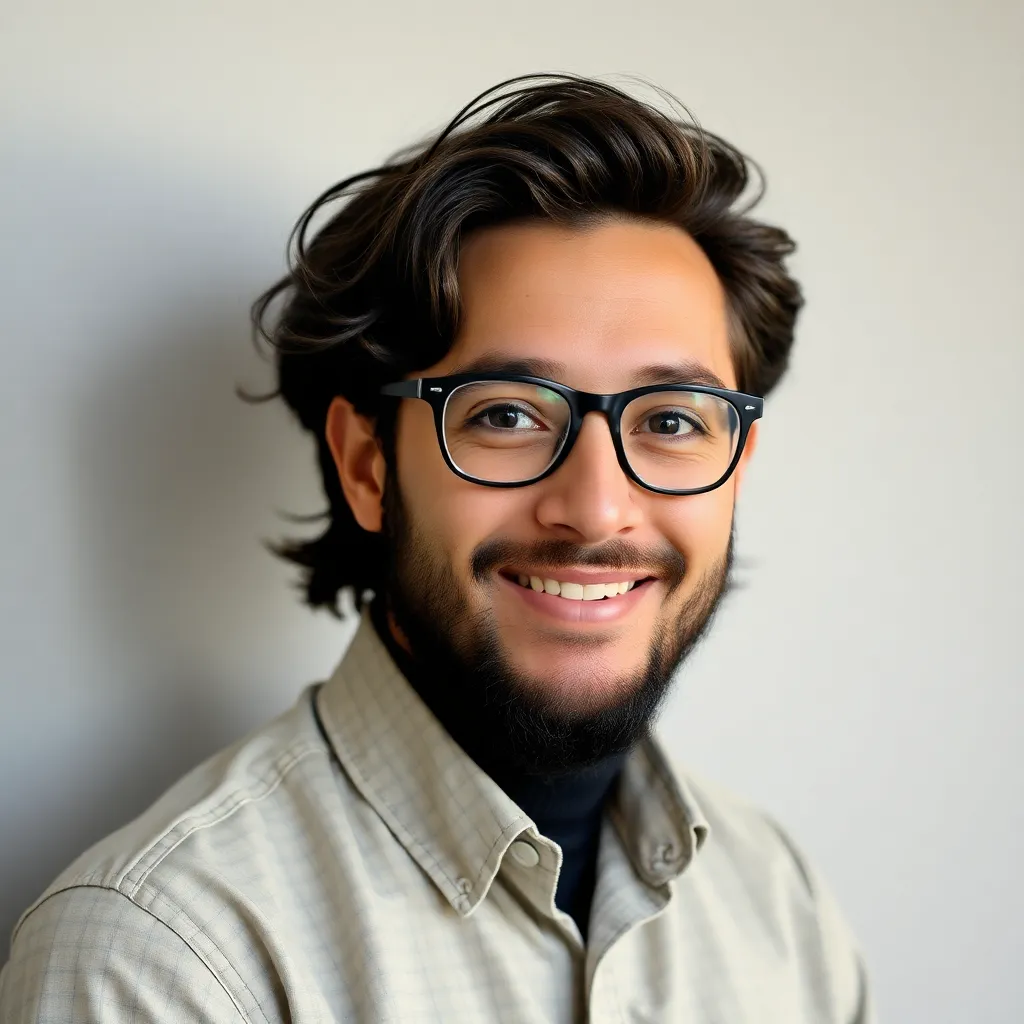
Kalali
May 23, 2025 · 3 min read
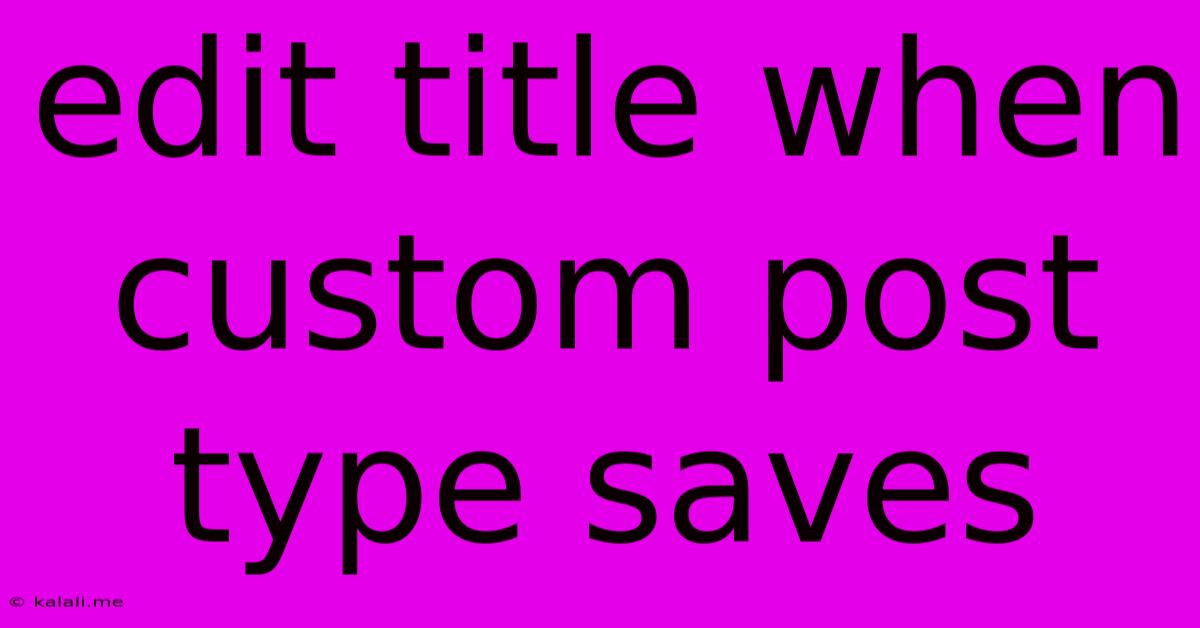
Table of Contents
Editing Post Titles When Custom Post Types Save: A WordPress Developer's Guide
This article provides a comprehensive guide for WordPress developers on how to modify post titles when custom post types are saved. We'll explore various methods, focusing on efficiency and best practices, ensuring your code is clean, robust, and easily maintainable. This is crucial for automating title generation, enforcing naming conventions, or performing complex title manipulations.
Modifying the title upon saving requires using WordPress's action hooks. Specifically, we'll leverage the save_post
action. However, directly manipulating the post title can be problematic if not handled correctly. Therefore, we'll emphasize secure coding practices to avoid unexpected behavior and data loss.
Understanding the save_post
Action Hook
The save_post
action hook fires after a post is saved. It provides an ideal location to intercept and modify the title before it's permanently stored in the database. The hook receives two arguments: the post_ID
and the post
object.
This allows us to access and manipulate the title using the $post->post_title
property. However, crucial checks are necessary to prevent infinite loops and unintended consequences.
Securely Modifying the Post Title
The following code snippet demonstrates a secure and efficient approach:
post_type != 'my_custom_post_type' ) return;
// Only modify title if it's not empty
if ( !empty( $post->post_title ) ) {
$original_title = $post->post_title;
$new_title = strtoupper($original_title); // Example modification: converting to uppercase
// Update the post title. Important: Use wp_update_post to avoid infinite loops.
$updated_post = array(
'ID' => $post_ID,
'post_title' => $new_title
);
wp_update_post( $updated_post );
}
}
?>
This code first checks for autosaves and user permissions. This prevents unnecessary modifications and protects against unauthorized changes. It then targets only 'my_custom_post_type' posts. The crucial aspect is using wp_update_post
to update the post title, avoiding potential infinite loops caused by directly modifying $post->post_title
.
Key considerations:
- Error Handling: Add error handling to gracefully manage potential issues, such as database errors.
- Testing: Thoroughly test your code to ensure it functions correctly and doesn't introduce unintended side effects.
- Custom Logic: Replace the example title modification (
strtoupper($original_title)
) with your specific logic to manipulate the title as needed. This could involve adding prefixes, suffixes, cleaning up formatting, or even generating titles based on other post meta data.
Advanced Techniques and Considerations
- Using Post Meta: For more complex title manipulations or storing additional information related to the title's creation, consider using post meta. This allows you to keep the logic separated and potentially more maintainable.
- External Data Sources: You could fetch data from external APIs or databases to influence the generated title. However, be mindful of performance implications and error handling.
- Filtering vs. Modifying: While we've focused on modifying the title directly, consider using the
pre_post_title
filter as an alternative approach. This filter allows you to modify the title before it's saved, offering a slightly different workflow.
By following these guidelines and implementing robust error handling, you can effectively and securely modify post titles when your custom post types are saved in WordPress, providing a cleaner and more efficient workflow. Remember to always prioritize secure coding practices and thorough testing.
Latest Posts
Latest Posts
-
Componentprofiler Component Level Profiling Has Not Been Enabled
May 23, 2025
-
Most Complex Roots A Nth Degree Polynomial
May 23, 2025
-
Mean And Prediction Intervals Formula In Multiple Regression
May 23, 2025
-
How To Check The Rpm Of Something Is Spinning
May 23, 2025
-
Water Coming Out Of Exhaust While Idling
May 23, 2025
Related Post
Thank you for visiting our website which covers about Edit Title When Custom Post Type Saves . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.