Error Casting Generic Object To Type Java
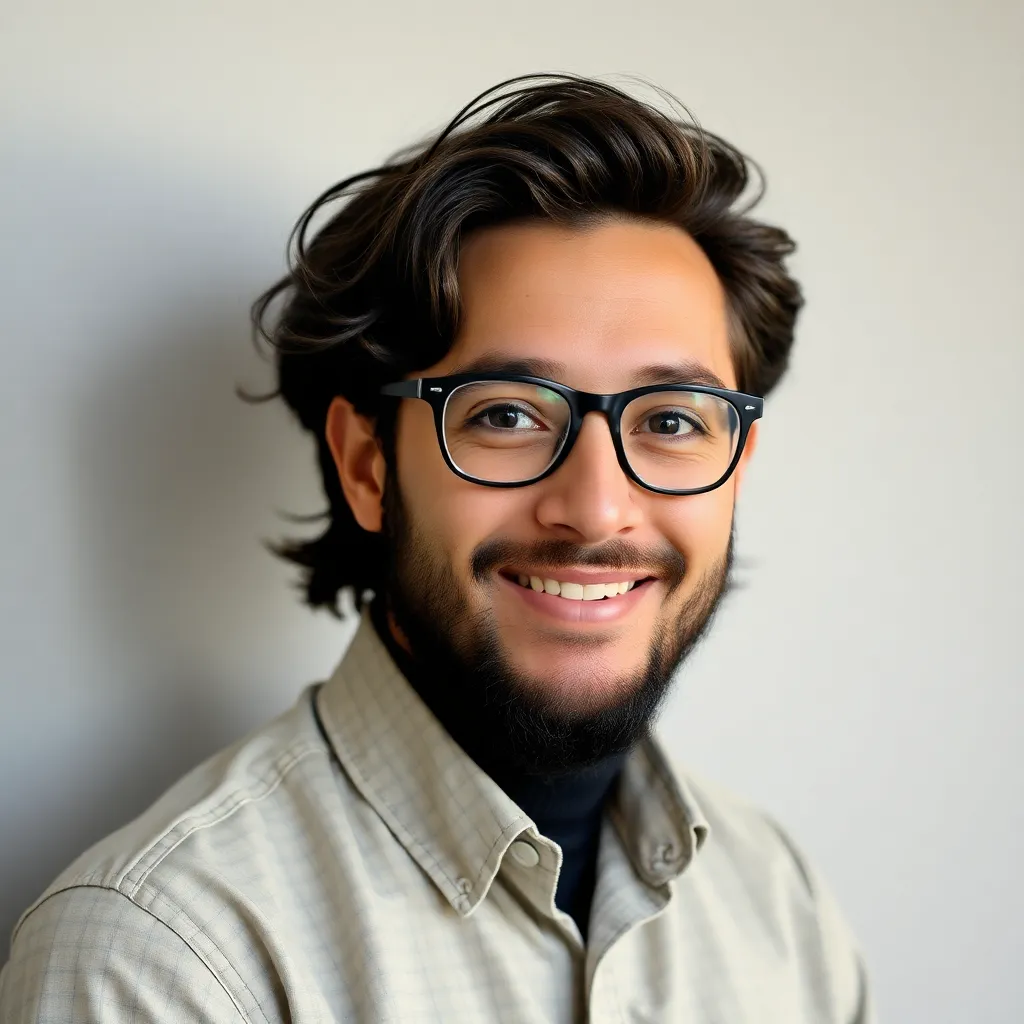
Kalali
May 23, 2025 · 3 min read
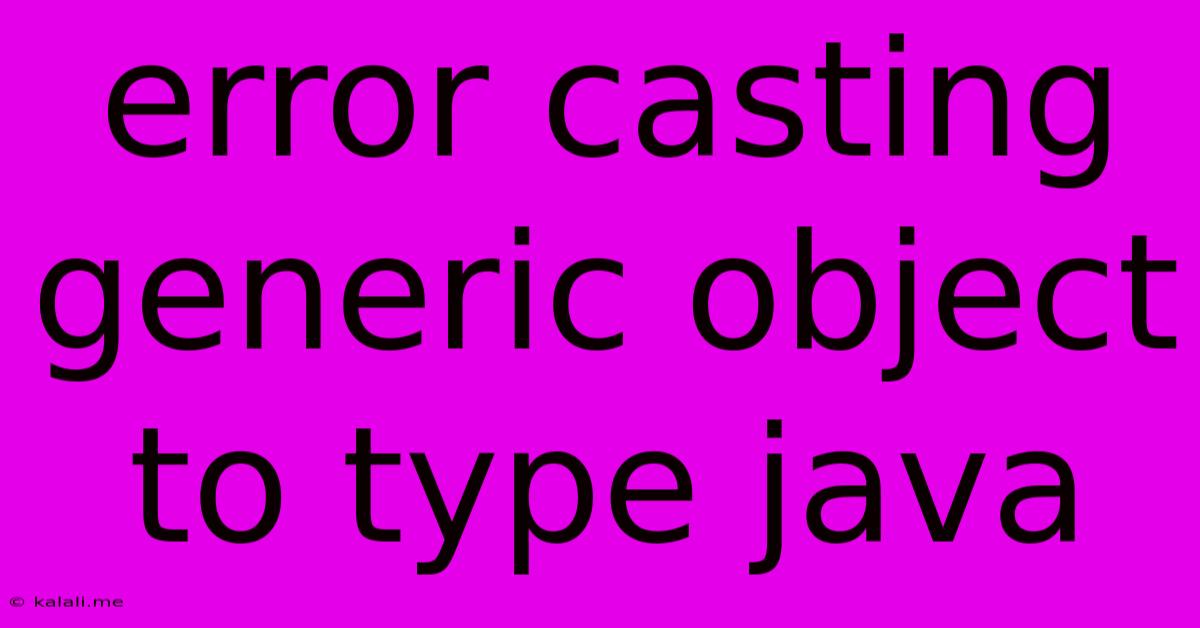
Table of Contents
Error Casting Generic Object to Type in Java: Causes and Solutions
Meta Description: Encountering "error casting generic object to type" in Java? This comprehensive guide dives into the root causes of this common issue, offering practical solutions and best practices to avoid it. Learn about type erasure, generics limitations, and safe casting techniques.
Java's generics, while powerful, can sometimes lead to runtime ClassCastException
errors when attempting to cast a generic object to a specific type. This article explores the reasons behind these errors and provides effective strategies for preventing them. Understanding these challenges is crucial for writing robust and error-free Java applications.
Understanding Type Erasure
The core of the problem lies in Java's implementation of generics: type erasure. At compile time, generic type information is largely removed. The JVM doesn't actually know the specific type of a List<String>
versus a List<Integer>
at runtime; both are treated as simply List
. This erasure is why you can't directly cast a List<?>
(a list of unknown type) to List<String>
without potentially encountering a ClassCastException
.
Common Scenarios and Causes of ClassCastException
Several situations frequently trigger this casting error:
-
Casting
List<?>
to a Specific Type: AList<?>
can hold objects of any type. Attempting a direct cast like(List<String>) myList
wheremyList
is aList<?>
will fail at runtime ifmyList
contains elements that aren't Strings. The compiler might even allow this, but the runtime will throw the exception. -
Incorrect Generic Type Inference: If the generic type isn't correctly inferred during compilation, unexpected type information might be lost, leading to runtime casting issues. Be meticulous with your generic type declarations.
-
Using Raw Types: Using raw types (e.g.,
List
instead ofList<String>
) is generally discouraged. Raw types bypass the type safety provided by generics, makingClassCastException
more likely. -
Mixing Generic and Non-Generic Code: Interactions between generic and non-generic code can obscure type information and introduce casting complications. Aim for consistency in your usage of generics.
Solutions and Best Practices
Here are strategies to handle these casting challenges:
- Use Type-Safe Methods: Instead of relying on casting, leverage methods that handle type checking internally. For example, use
instanceof
before casting:
List> myList = ...;
for (Object obj : myList) {
if (obj instanceof String) {
String str = (String) obj; // Safe cast
// Process str
}
}
- Bounded Wildcards: Use bounded wildcards (
<? extends String>
) to specify an upper bound for the generic type. This limits the types that can be stored in the list, making casting safer.
List extends String> myList = ...; // myList can hold Strings or subtypes of String
// You can't add elements to this list though
for(Object str:myList){
String s = (String) str; // Safe cast
}
-
Avoid Raw Types: Always favor parameterized types over raw types. The compiler's type checking capabilities will help you catch potential casting issues at compile time instead of runtime.
-
Careful Generic Type Declarations: Pay close attention to generic type declarations in your methods and classes. Ensure that the types are clearly specified and consistently used.
-
Check for Null: Before attempting any cast, always check for
null
values to preventNullPointerExceptions
. -
Generics and Inheritance: Be mindful of type parameters and inheritance. Casting issues can arise from improper relationships between generic types and their subclasses. Thoroughly examine your class hierarchies.
-
Debugging: Use a debugger to step through your code and examine the types of objects at runtime. This can help pinpoint the exact location and cause of the
ClassCastException
.
By understanding type erasure and adopting these best practices, you can significantly reduce the likelihood of encountering "error casting generic object to type" exceptions in your Java code, leading to more robust and maintainable applications. Careful attention to generic type handling is paramount for creating reliable Java programs.
Latest Posts
Latest Posts
-
Wire Size For 125 Amp Sub Panel
May 24, 2025
-
Teritoney Operator Handle Null In Apex
May 24, 2025
-
What Is The Mle Of Geometric
May 24, 2025
-
Where Do You Put Up Meaning
May 24, 2025
-
Is P Value The Same As Type 1 Error
May 24, 2025
Related Post
Thank you for visiting our website which covers about Error Casting Generic Object To Type Java . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.