Get Line Cstring Assign To Pointer C
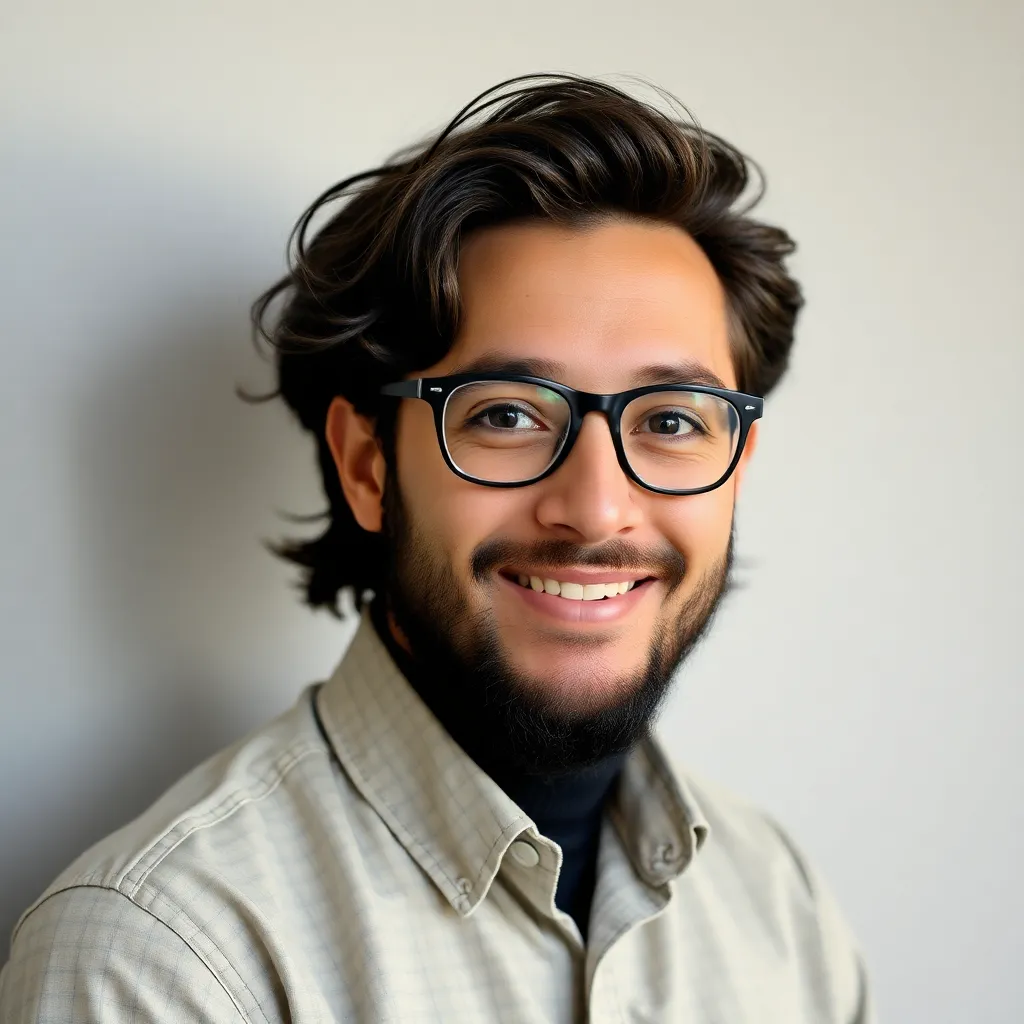
Kalali
May 23, 2025 · 3 min read
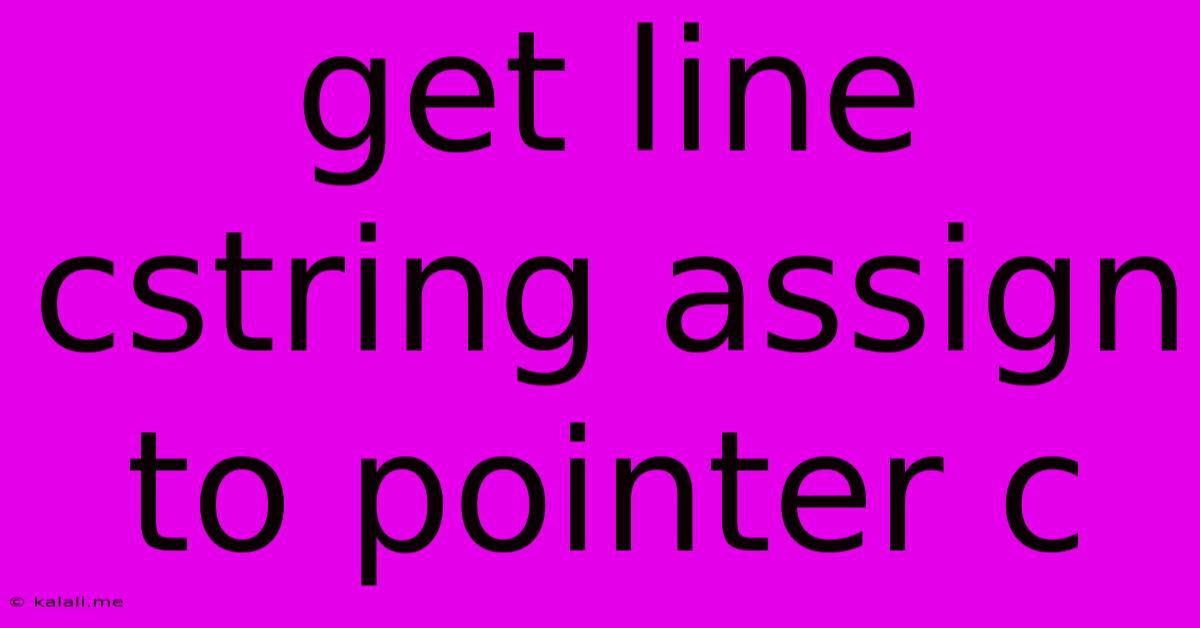
Table of Contents
Getting a C String Assigned to a Pointer in C
This article will guide you through the process of assigning a C-string (null-terminated string) to a character pointer in C. Understanding this fundamental concept is crucial for working with strings in C programming. We'll cover different methods, potential pitfalls, and best practices to ensure your code is efficient and error-free.
Understanding C Strings and Pointers
Before diving into assignment, let's refresh our understanding. In C, a string is essentially an array of characters terminated by a null character ('\0'). A character pointer (char *
) holds the memory address of the first character of the string. Therefore, assigning a string to a pointer means assigning the memory address of the string's first character to the pointer.
Method 1: Direct Assignment (Using String Literals)
The simplest way to assign a string literal to a character pointer is through direct assignment:
#include
int main() {
char *myString;
myString = "Hello, world!";
printf("%s\n", myString); // Output: Hello, world!
return 0;
}
This method is concise and works well for constant strings. However, it's crucial to remember that you cannot modify the string assigned this way. Attempting to do so leads to undefined behavior, as the string literal is typically stored in a read-only memory segment.
Method 2: Assignment Using strcpy()
(For Modifiable Strings)
For situations where you need to modify the string, you must allocate memory dynamically and then copy the string using the strcpy()
function from the <string.h>
header file:
#include
#include
#include
int main() {
char *myString;
myString = (char *)malloc(20 * sizeof(char)); // Allocate memory (adjust size as needed)
if (myString == NULL) {
fprintf(stderr, "Memory allocation failed!\n");
return 1; // Indicate an error
}
strcpy(myString, "Hello, world!");
printf("%s\n", myString); // Output: Hello, world!
strcpy(myString, "This string is modified!");
printf("%s\n", myString); // Output: This string is modified!
free(myString); // Free the allocated memory to prevent memory leaks
myString = NULL; // Good practice to set the pointer to NULL after freeing
return 0;
}
This approach involves:
- Memory Allocation:
malloc()
allocates sufficient memory to hold the string. Always remember to check for allocation failure (NULL pointer). The size should be at least one character more than the string length to accommodate the null terminator. - String Copying:
strcpy()
copies the source string into the allocated memory pointed to bymyString
. - Memory Deallocation:
free()
releases the dynamically allocated memory. This is extremely important to prevent memory leaks. SettingmyString
to NULL after freeing is a good practice to avoid dangling pointers.
Method 3: Using strncpy()
for Safer Copying
While strcpy()
is efficient, it's susceptible to buffer overflow if the destination buffer is too small. strncpy()
offers a safer alternative by allowing you to specify the maximum number of characters to copy:
#include
#include
#include
int main() {
char *myString;
myString = (char *)malloc(20 * sizeof(char)); // Allocate memory
if (myString == NULL) {
fprintf(stderr, "Memory allocation failed!\n");
return 1;
}
strncpy(myString, "A longer string than the buffer!", 19); // Copy at most 19 characters
myString[19] = '\0'; // Ensure null termination (crucial with strncpy)
printf("%s\n", myString); // Output: A longer string than the
free(myString);
myString = NULL;
return 0;
}
Important Considerations:
- Memory Management: Always allocate sufficient memory and deallocate it when finished to avoid memory leaks.
- Null Termination: Ensure your strings are always null-terminated. Functions like
printf()
andstrcpy()
rely on this null character to know where the string ends. - Error Handling: Check for errors, particularly memory allocation failures.
- Buffer Overflow: Be mindful of potential buffer overflows, especially when using
strcpy()
.strncpy()
provides a safer alternative but requires careful handling of null termination.
By understanding these methods and best practices, you can confidently work with C strings and pointers, creating robust and efficient C programs. Remember to always prioritize memory safety and handle potential errors gracefully.
Latest Posts
Latest Posts
-
Why Does A Car Stall Manual
May 25, 2025
-
Where Do I Need Arc Fault Breakers
May 25, 2025
-
3 Way Switch 4 Way Switch
May 25, 2025
-
Proc Macro Crate Build Data Is Missing Dylib Path
May 25, 2025
-
Can You Hurdle In High School Football
May 25, 2025
Related Post
Thank you for visiting our website which covers about Get Line Cstring Assign To Pointer C . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.