Google Sheet Script Pass Sheet To Function
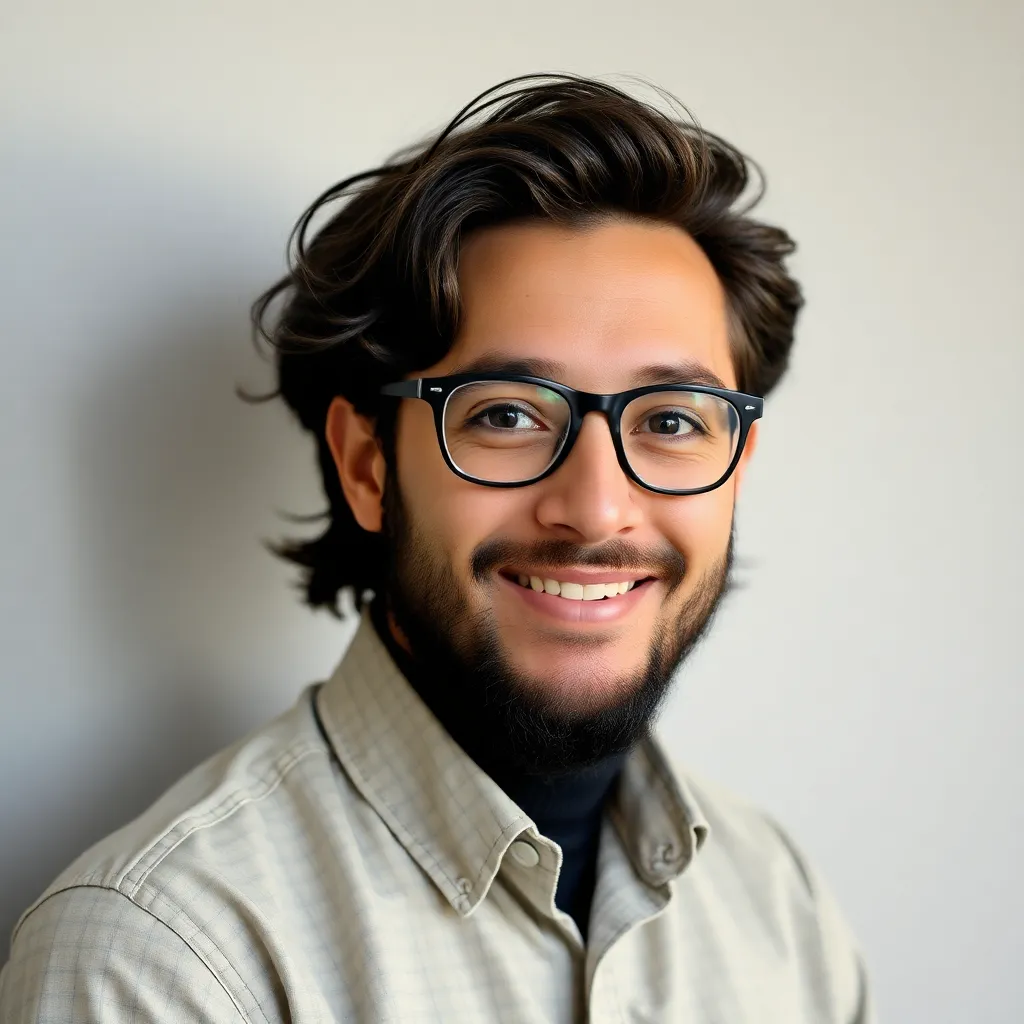
Kalali
May 23, 2025 · 3 min read
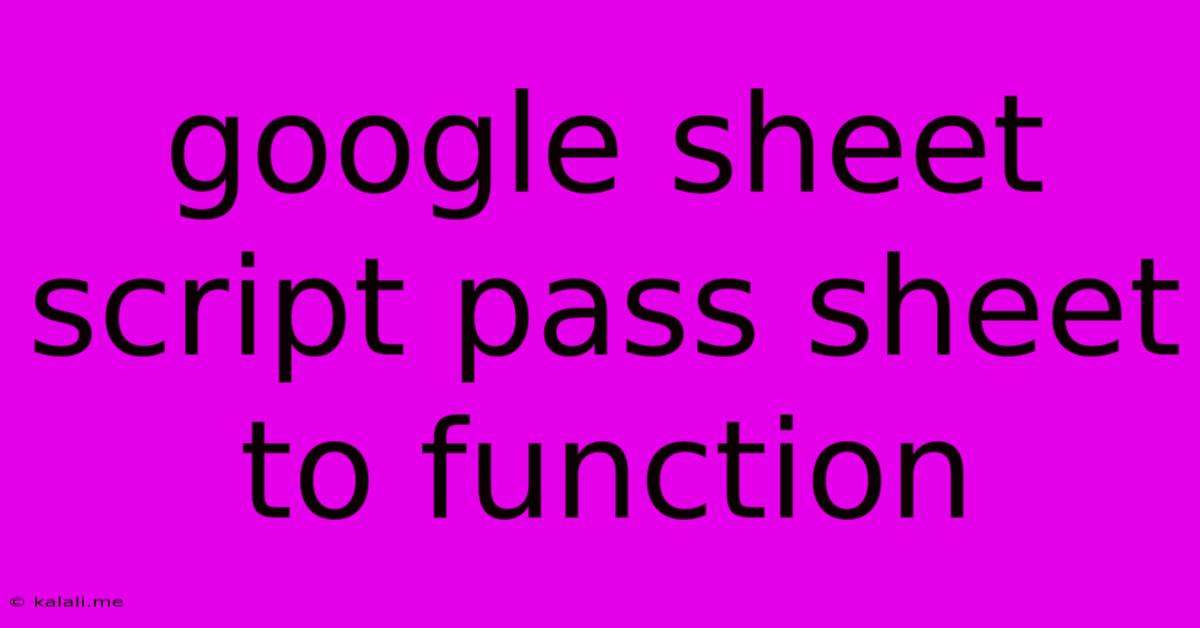
Table of Contents
Passing Google Sheets to Google Apps Script Functions: A Comprehensive Guide
This article will guide you through the various methods of passing Google Sheets and their data to Google Apps Script functions. Understanding this process is crucial for automating tasks and building powerful custom solutions within Google Sheets. Whether you're a beginner or an experienced scripter, this guide will equip you with the knowledge to efficiently handle sheet data within your scripts.
Understanding the Context: Why Pass Sheets to Functions?
Passing Google Sheets (or specific ranges within them) to your Apps Script functions allows you to process data more efficiently and modularly. Instead of writing repetitive code for accessing and manipulating data, you can encapsulate these operations within reusable functions. This improves code readability, maintainability, and reduces the risk of errors.
Methods for Passing Sheets and Data
There are several ways to pass Sheets and their data to your Apps Script functions, each with its own advantages and use cases:
1. Passing the Spreadsheet Object
This method passes the entire Spreadsheet object to your function. This grants access to all sheets, properties, and functionalities of the spreadsheet.
function myFunction(spreadsheet) {
// Access the active sheet
let activeSheet = spreadsheet.getActiveSheet();
// Access a sheet by name
let sheetByName = spreadsheet.getSheetByName("Sheet1");
// Get data from a specific range
let data = sheetByName.getRange("A1:B10").getValues();
// Process the data...
Logger.log(data);
}
To use this, you would call the function like this within your spreadsheet: =myFunction(SpreadsheetApp.getActiveSpreadsheet())
This is generally less efficient for large spreadsheets as it loads the entire spreadsheet into memory.
2. Passing the Sheet Object
This offers a more targeted approach, providing access only to a specific sheet. This improves performance compared to passing the entire spreadsheet, particularly when dealing with large datasets.
function processSheet(sheet) {
let data = sheet.getDataRange().getValues();
// Process data from the sheet...
Logger.log(data);
}
function mainFunction() {
let ss = SpreadsheetApp.getActiveSpreadsheet();
let sheet = ss.getSheetByName("Sheet1");
processSheet(sheet);
}
3. Passing Data Ranges
The most efficient method, especially for large spreadsheets, is to pass only the necessary data range. This reduces memory usage and improves script performance significantly.
function processRange(dataRange) {
let values = dataRange.getValues();
//Process the 'values' array
Logger.log(values);
}
function mainFunction() {
let ss = SpreadsheetApp.getActiveSpreadsheet();
let sheet = ss.getSheetByName("Sheet1");
let range = sheet.getRange("A1:B10");
processRange(range);
}
This method is ideal when you only need to work with a specific portion of the sheet.
4. Using getActiveSpreadsheet()
and getActiveSheet()
within the Function
For simple scripts, you can directly access the active spreadsheet and sheet within the function without explicitly passing them as arguments. However, this approach lacks modularity and reusability.
function mySimpleFunction() {
let ss = SpreadsheetApp.getActiveSpreadsheet();
let sheet = ss.getActiveSheet();
let data = sheet.getRange("A1:A10").getValues();
Logger.log(data);
}
This approach is suitable for quick, one-off tasks but is less scalable for larger projects.
Choosing the Right Method
The best approach depends on your specific needs:
- For small spreadsheets or when needing access to the entire spreadsheet: Pass the Spreadsheet object.
- For larger spreadsheets or when working with a single sheet: Pass the Sheet object.
- For optimal performance with large datasets: Pass the data range.
- For simple, isolated tasks: Use
getActiveSpreadsheet()
andgetActiveSheet()
directly within the function.
By understanding and implementing these methods, you can significantly improve the efficiency and maintainability of your Google Apps Script projects that interact with Google Sheets. Remember to prioritize the most efficient method based on the size of your data and the complexity of your script. Always strive for clean, well-organized code for easier debugging and future modifications.
Latest Posts
Latest Posts
-
What Is A Quarter Of A Million
Jul 02, 2025
-
Which Of The Following Is True Concerning A Dao
Jul 02, 2025
-
How Long Can Catfish Live Out Of Water
Jul 02, 2025
-
Is Kanye West Related To Cornel West
Jul 02, 2025
-
Olivia Needs To Provide A Visual Summary
Jul 02, 2025
Related Post
Thank you for visiting our website which covers about Google Sheet Script Pass Sheet To Function . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.