Google Sheets Script Save Range As Var
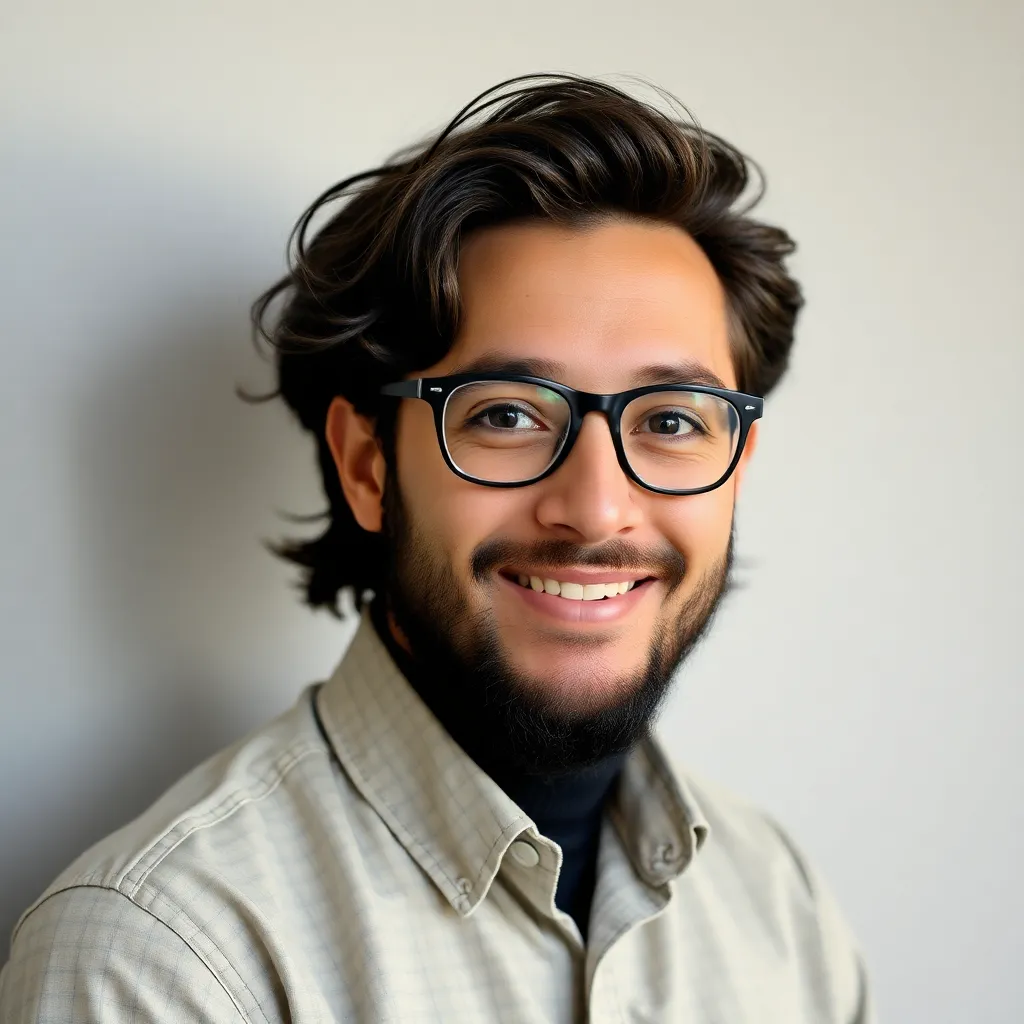
Kalali
May 27, 2025 · 3 min read
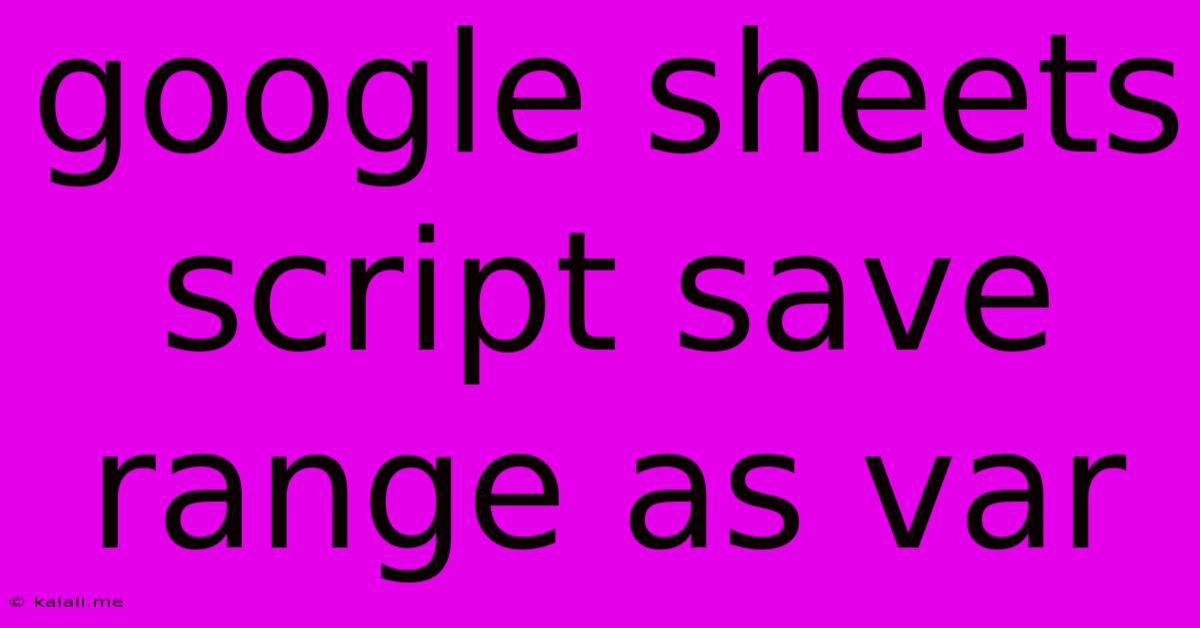
Table of Contents
Saving a Google Sheets Range as a Variable in Google Apps Script
This article will guide you through the process of saving a range of cells from a Google Sheet as a variable within a Google Apps Script. This is a fundamental task for many automation and data manipulation projects in Google Sheets. We'll cover various methods and scenarios to help you choose the best approach for your specific needs. Understanding how to handle ranges effectively is crucial for building robust and efficient Google Sheets scripts.
Why Save Ranges as Variables?
Before diving into the code, let's understand the benefits of storing a range of cells as a variable:
- Efficiency: Processing data directly from the variable is significantly faster than repeatedly accessing the spreadsheet.
- Readability: Your script becomes cleaner and easier to understand.
- Reusability: You can easily reuse the data stored in the variable throughout your script.
- Flexibility: Variables allow for dynamic range selection based on user input or other factors.
Methods for Saving Ranges as Variables
There are several ways to achieve this, each with its own strengths:
1. Using getRange()
and getValues()
This is the most common method. getRange()
selects the desired range, and getValues()
retrieves the data as a two-dimensional array.
function saveRangeAsVariable() {
// Get the active spreadsheet and sheet.
const ss = SpreadsheetApp.getActiveSpreadsheet();
const sheet = ss.getActiveSheet();
// Define the range (adjust A1:B2 as needed).
const range = sheet.getRange("A1:B2");
// Get the values as a 2D array.
const rangeValues = range.getValues();
// Now 'rangeValues' contains the data. You can process it further.
Logger.log(rangeValues); // Log the array to the Apps Script execution log.
//Example of accessing specific cell values:
let cellA1Value = rangeValues[0][0]; //Get value of cell A1
Logger.log("Value of A1: " + cellA1Value);
}
This approach is perfect for situations where you need to work with the raw cell values. Remember that getValues()
returns a two-dimensional array, even if the range is a single cell.
2. Using getRange()
and getValue()
for Single Cells
If you only need the value of a single cell, getValue()
is more efficient than getValues()
.
function saveSingleCellAsVariable() {
const ss = SpreadsheetApp.getActiveSpreadsheet();
const sheet = ss.getActiveSheet();
const cell = sheet.getRange("A1");
const cellValue = cell.getValue();
Logger.log(cellValue);
}
This method avoids the overhead of processing a larger array when only a single value is needed.
3. Handling Different Data Types
Remember that getValues()
returns an array of arrays. The data type within each array element depends on the cell's contents. You might encounter numbers, strings, dates, booleans, or null values. Be mindful of this when processing the data. For example, you might need to use parseInt()
or other type conversion functions.
4. Error Handling
Robust scripts incorporate error handling. Consider what might go wrong (e.g., the range doesn't exist, the sheet is protected) and add try...catch
blocks to handle potential exceptions gracefully.
function saveRangeWithErrorHandler() {
try {
// Your range saving code from method 1 goes here...
} catch (e) {
Logger.log("Error: " + e);
}
}
By understanding these methods and incorporating error handling, you can effectively save and manipulate ranges of data within your Google Apps Script projects, building powerful and reliable spreadsheet automation tools. Remember to always adjust the range specification ("A1:B2" in the examples) to match your actual spreadsheet data.
Latest Posts
Latest Posts
-
25 Cents A Minute For An Hour
Jun 30, 2025
-
In Music What Does Allegro Mean Math Answer Key Pdf
Jun 30, 2025
-
What Is 1 5 Of A Tablespoon
Jun 30, 2025
-
How Long Does It Take To Drive Through Illinois
Jun 30, 2025
-
If I Was Born In 1988 How Old Am I
Jun 30, 2025
Related Post
Thank you for visiting our website which covers about Google Sheets Script Save Range As Var . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.