Google Sheets Script Save Range As Variable
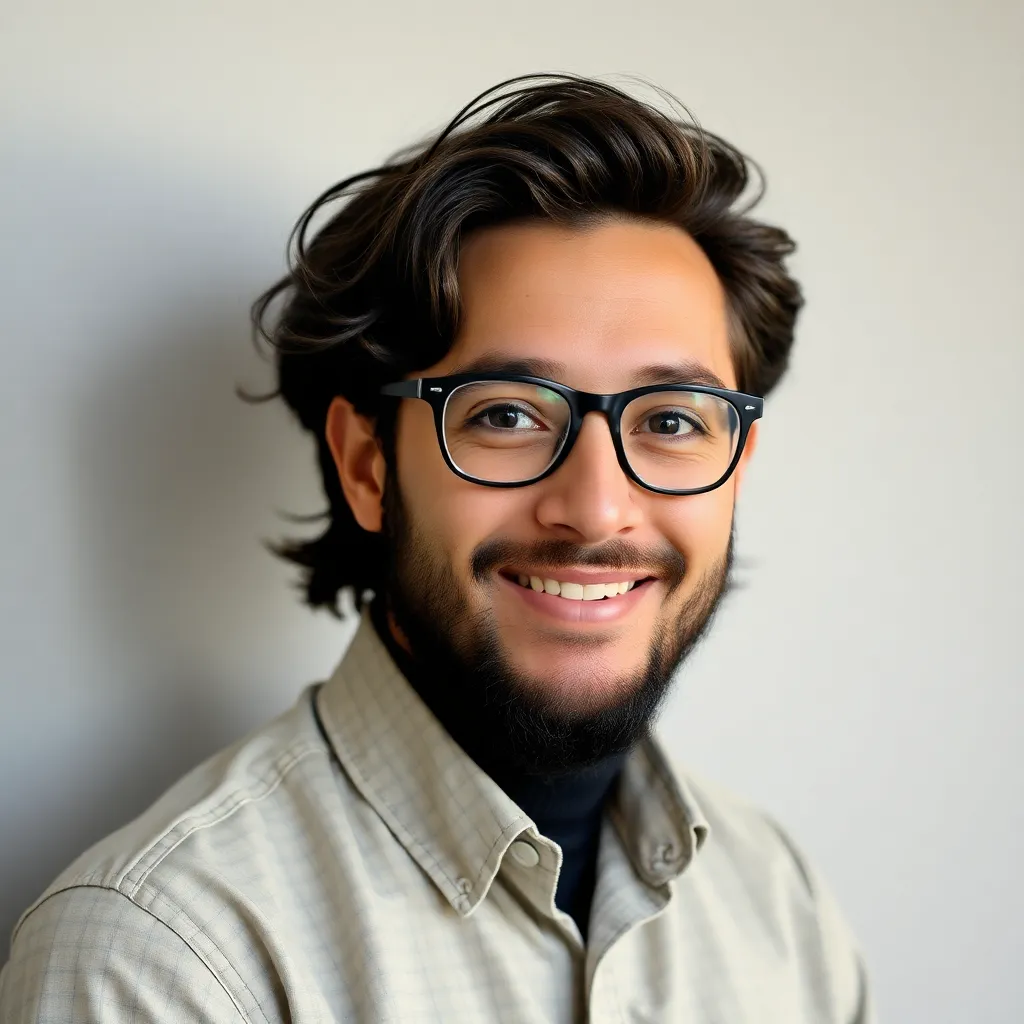
Kalali
May 25, 2025 · 3 min read
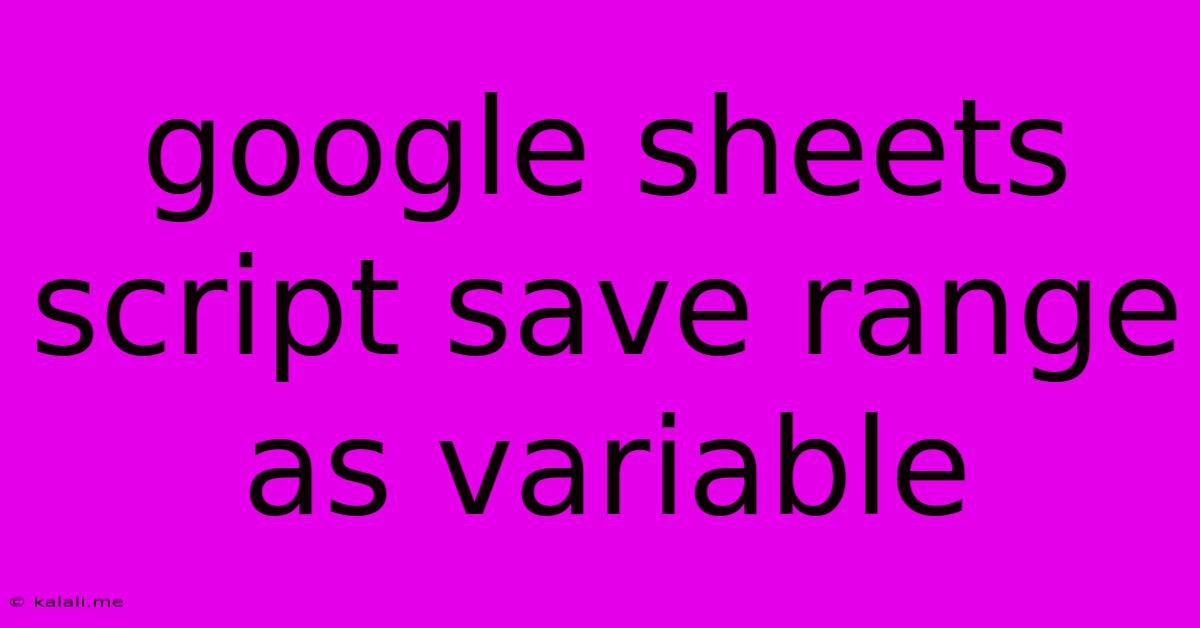
Table of Contents
Saving a Google Sheets Range as a Variable in Google Apps Script
This article will guide you through the process of saving a range of cells from a Google Sheet as a variable within a Google Apps Script. This is a fundamental task for many automation and data manipulation projects, allowing you to efficiently work with specific portions of your spreadsheet data. Whether you're processing data, formatting cells, or building complex functions, understanding how to handle ranges as variables is crucial.
Understanding the Basics:
Google Apps Script interacts with Google Sheets using objects. The Spreadsheet
object represents the entire spreadsheet, while the Sheet
object represents a single sheet within the spreadsheet. Within a sheet, data is organized into Range
objects. These Range
objects are what we'll be focusing on. To effectively work with data in your script, you'll need to learn how to select and manipulate these ranges.
Methods for Saving a Range as a Variable:
There are several methods for saving a range as a variable, each with its own advantages depending on how you intend to use the data.
1. Using getRange()
to Save a Range as a Variable
The most common method is using the getRange()
method. This method allows you to specify the range using various notations:
-
By row and column indices:
sheet.getRange(row, column, numRows, numCols)
row
: The starting row number (1-based index).column
: The starting column number (1-based index).numRows
: The number of rows in the range.numCols
: The number of columns in the range.
-
By A1 notation:
sheet.getRange("A1:B2")
This is a more readable way, particularly for smaller ranges.
Example:
function saveRangeAsVariable() {
// Get the active spreadsheet and sheet.
const ss = SpreadsheetApp.getActiveSpreadsheet();
const sheet = ss.getActiveSheet();
// Save a range (from A1 to B2) as a variable.
const myRange = sheet.getRange("A1:B2");
// Access values from the range.
const values = myRange.getValues();
Logger.log(values); // Logs the values to the Apps Script execution log.
// You can now work with 'myRange' and 'values' throughout your script.
// Example: Set values in another range.
sheet.getRange("C1:D2").setValues(values);
}
2. Saving Values Directly
Sometimes, you only need the values within a range, not the range object itself. In such cases, you can directly get the values using getValues()
:
function saveRangeValues() {
const ss = SpreadsheetApp.getActiveSpreadsheet();
const sheet = ss.getActiveSheet();
const values = sheet.getRange("A1:B2").getValues();
Logger.log(values);
}
This method is more memory-efficient if you don't need to perform further manipulations on the range object itself.
3. Handling Large Ranges Efficiently
For very large ranges, consider using iterative processing to avoid exceeding memory limits. Instead of loading the entire range into memory at once, process it in smaller chunks:
function processLargeRange(sheet, range) {
const numRows = range.getNumRows();
const numCols = range.getNumCols();
for (let i = 1; i <= numRows; i++) {
const rowRange = range.offset(i - 1, 0, 1, numCols); //Process row by row
const rowValues = rowRange.getValues();
//Process rowValues
}
}
Best Practices:
- Use descriptive variable names: Make your code easier to understand and maintain.
- Handle errors: Use
try...catch
blocks to gracefully handle potential errors. - Comment your code: Explain what your code does, especially for complex operations.
By mastering these techniques, you'll be well-equipped to handle and manipulate data within your Google Sheets using Google Apps Script, opening up a world of automation possibilities. Remember to consult the Google Apps Script documentation for further details and advanced functionalities related to the Range
object and its methods.
Latest Posts
Latest Posts
-
How Many Tablespoons Are In A Hidden Valley Ranch Packet
Jul 10, 2025
-
Which Is The Best Summary Of The Passage
Jul 10, 2025
-
How Many Quarts Of Soil In A Cubic Foot
Jul 10, 2025
-
What Is 3 4 Of A Pound
Jul 10, 2025
-
How To Measure 1 8 Teaspoon With 1 4 Teaspoon
Jul 10, 2025
Related Post
Thank you for visiting our website which covers about Google Sheets Script Save Range As Variable . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.