Graphql Using And In Where Clause Lwc Example
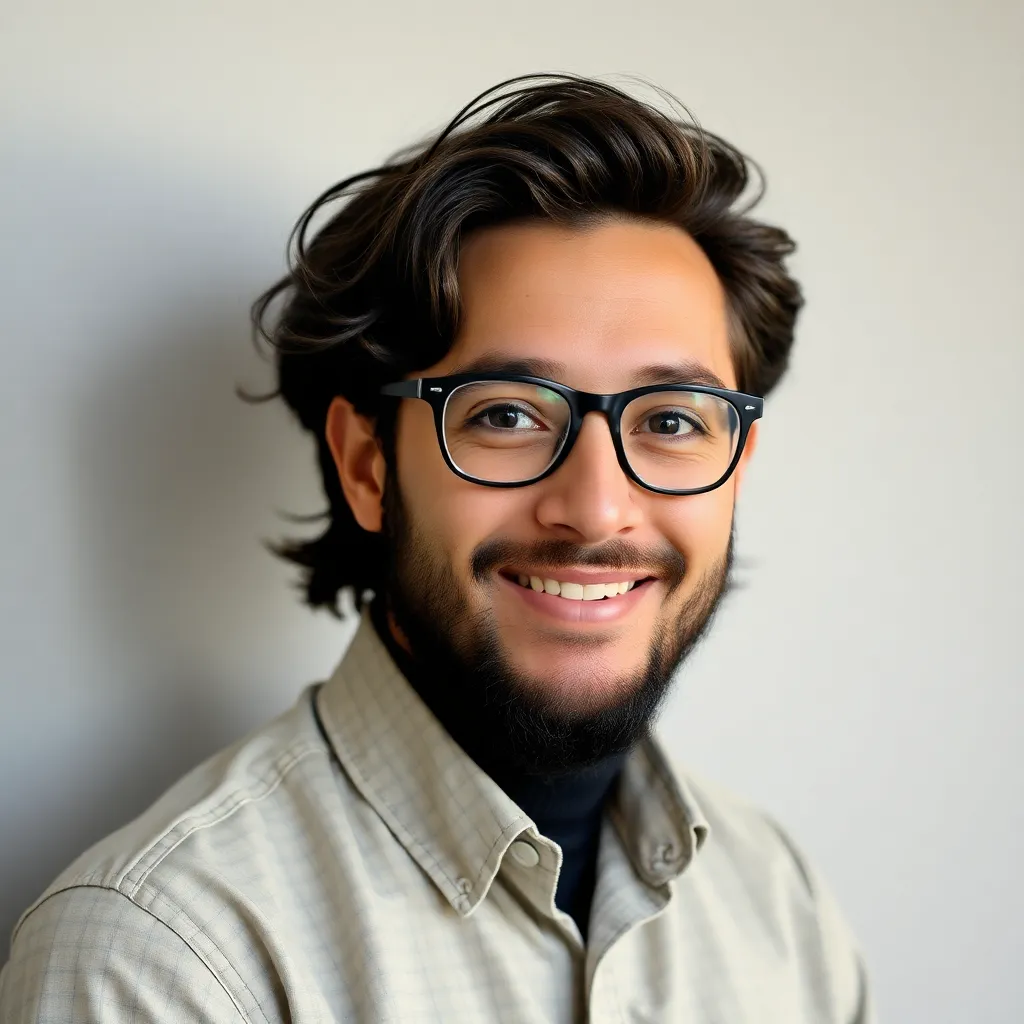
Kalali
May 23, 2025 · 3 min read
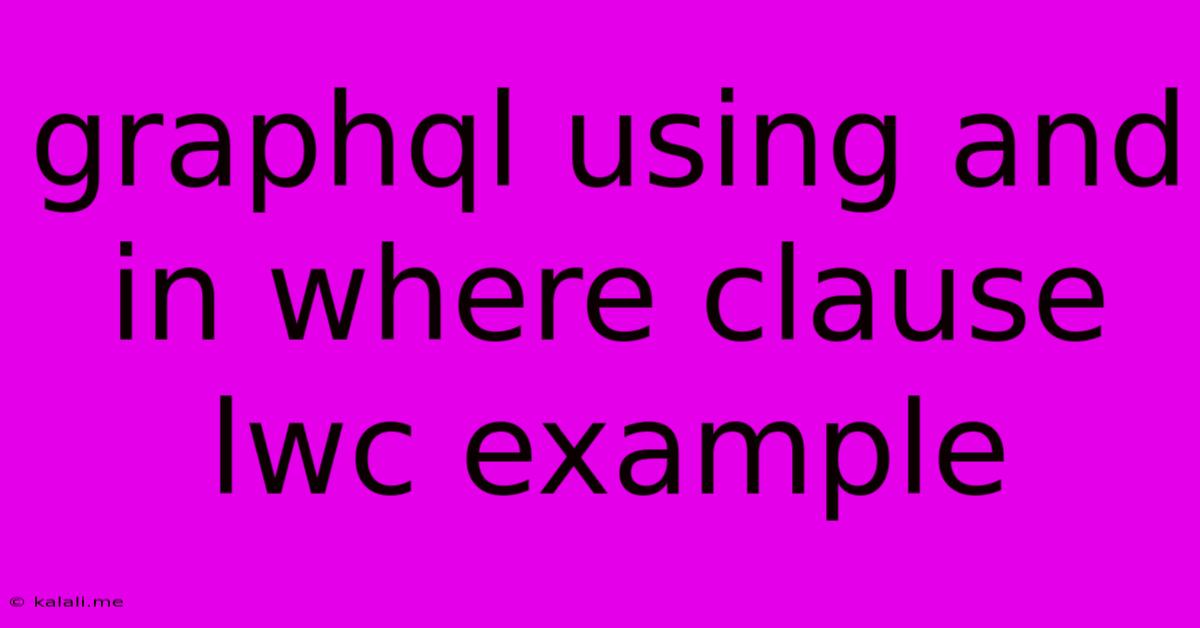
Table of Contents
Using GraphQL with IN and WHERE Clauses in LWC: A Comprehensive Guide
This article provides a comprehensive guide on leveraging GraphQL's power within your LWC (Lightning Web Components) applications, specifically focusing on the efficient use of IN
and WHERE
clauses for optimized data retrieval. We'll explore how to construct effective GraphQL queries to fetch only the necessary data, improving performance and user experience. This is crucial for building scalable and responsive Salesforce applications.
Understanding the Need for Efficient Data Retrieval
In many LWC applications, you'll need to retrieve specific records based on certain criteria. Fetching all records and then filtering client-side is inefficient and consumes unnecessary bandwidth. GraphQL, with its declarative nature, allows you to specify precisely what data you need, reducing the amount of data transferred between the client and the server. The IN
and WHERE
clauses are key tools in this process.
Leveraging GraphQL's IN
Clause
The IN
clause is incredibly useful when you need to retrieve records based on a list of IDs or other values. This avoids making multiple individual queries, optimizing the retrieval process significantly.
Let's consider an example where we want to fetch Accounts with IDs 0011t00000abcdef
, 0011t00000ghijkl
, and 0011t00000mnopqr
. A typical GraphQL query would look like this:
query getAccountsByIds($ids: [ID!]!) {
accounts(where: {Id: {in: $ids}}) {
data {
Id
Name
Industry
}
totalSize
}
}
Here:
$ids: [ID!]!
defines a variable accepting a non-null list of IDs.where: {Id: {in: $ids}}
filters the accounts to only include those with IDs present in the$ids
list.- The
data
field retrieves the desired account fields. totalSize
returns the total number of accounts matching the criteria.
Implementing the IN
Clause in your LWC
Within your LWC, you'd use this query along with the gql
tag in your JavaScript file:
import { LightningElement, wire } from 'lwc';
import GET_ACCOUNTS_BY_IDS from '@salesforce/apex/AccountController.getAccountsByIds'; // This is a placeholder, replace with your actual Apex controller
export default class AccountList extends LightningElement {
@wire(GET_ACCOUNTS_BY_IDS, { ids: ['0011t00000abcdef', '0011t00000ghijkl', '0011t00000mnopqr'] })
accounts; //This will be an array of accounts
//Rest of your component logic
}
Note: Replace @salesforce/apex/AccountController.getAccountsByIds
with the actual path to your Apex controller method that executes this GraphQL query. You will also need to set up your Apex controller to accept and execute the GraphQL query. This involves using a library such as Schema.DescribeSObjects
for dynamic SOQL generation.
Using GraphQL's WHERE
Clause for More Complex Filtering
The WHERE
clause provides a more flexible approach for filtering data based on various criteria. It allows for complex filtering logic by combining different conditions using operators like AND
, OR
, and NOT
.
For instance, to retrieve Accounts with a specific industry and annual revenue greater than a certain value:
query getAccountsByCriteria($industry: String!, $revenue: Integer!) {
accounts(where: {Industry: {eq: $industry}, AnnualRevenue: {gt: $revenue}}) {
data {
Id
Name
Industry
AnnualRevenue
}
totalSize
}
}
Here:
$industry
and$revenue
are variables for industry and annual revenue.Industry: {eq: $industry}
filters by industry using the equals operator.AnnualRevenue: {gt: $revenue}
filters by annual revenue using the greater than operator.
Best Practices for Efficient GraphQL Queries
- Specify only the necessary fields: Avoid retrieving unnecessary fields to minimize data transfer.
- Use variables: Use variables to make your queries more reusable and avoid hardcoding values.
- Optimize your filtering: Use efficient filtering strategies to avoid unnecessary data processing on the server.
- Understand Salesforce limits: Be aware of governor limits to avoid exceeding resource constraints.
- Pagination: For large datasets, use pagination to fetch data in smaller chunks.
By following these best practices and effectively using the IN
and WHERE
clauses in your GraphQL queries within LWC, you can dramatically improve the performance and scalability of your Salesforce applications. Remember to carefully plan your data retrieval strategy to maximize efficiency and user experience.
Latest Posts
Latest Posts
-
25 Cents A Minute For An Hour
Jun 30, 2025
-
In Music What Does Allegro Mean Math Answer Key Pdf
Jun 30, 2025
-
What Is 1 5 Of A Tablespoon
Jun 30, 2025
-
How Long Does It Take To Drive Through Illinois
Jun 30, 2025
-
If I Was Born In 1988 How Old Am I
Jun 30, 2025
Related Post
Thank you for visiting our website which covers about Graphql Using And In Where Clause Lwc Example . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.