How To Make Tool Names Colored In Java
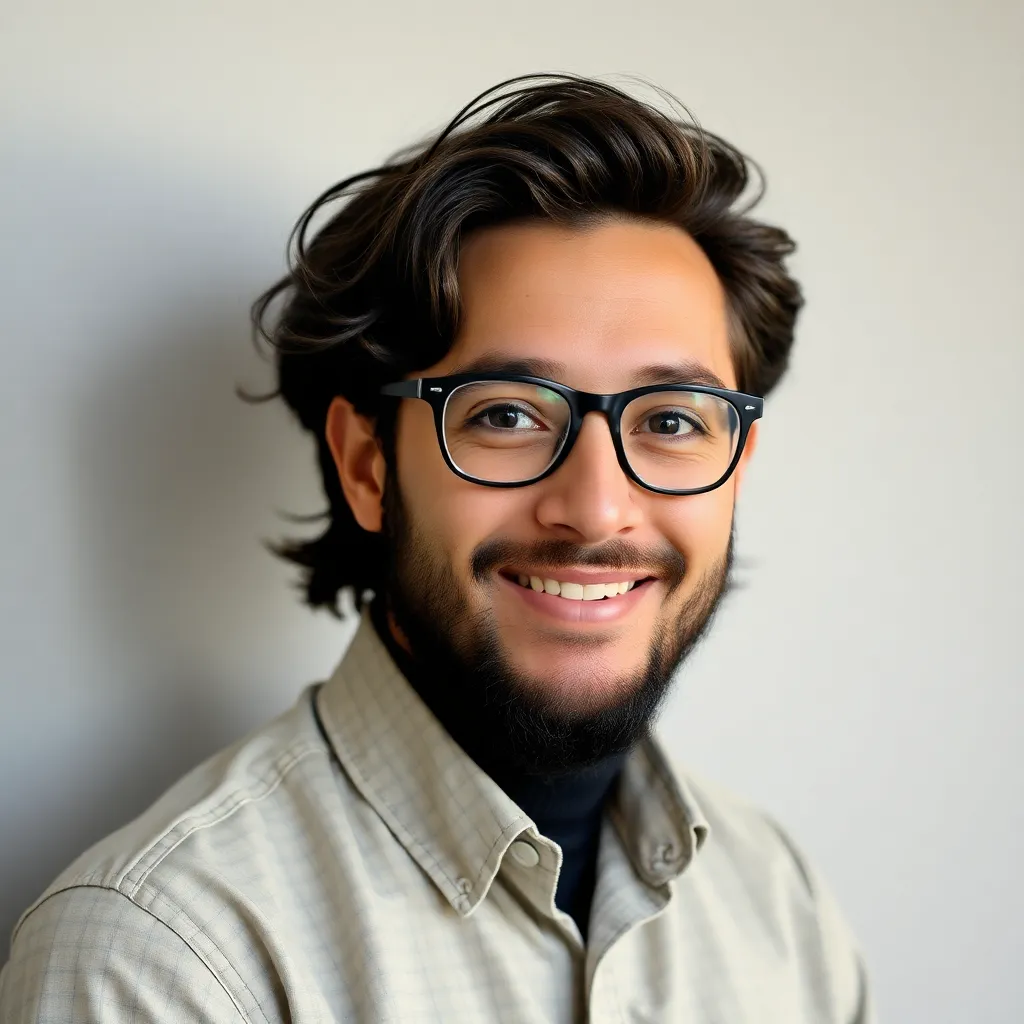
Kalali
May 27, 2025 · 3 min read
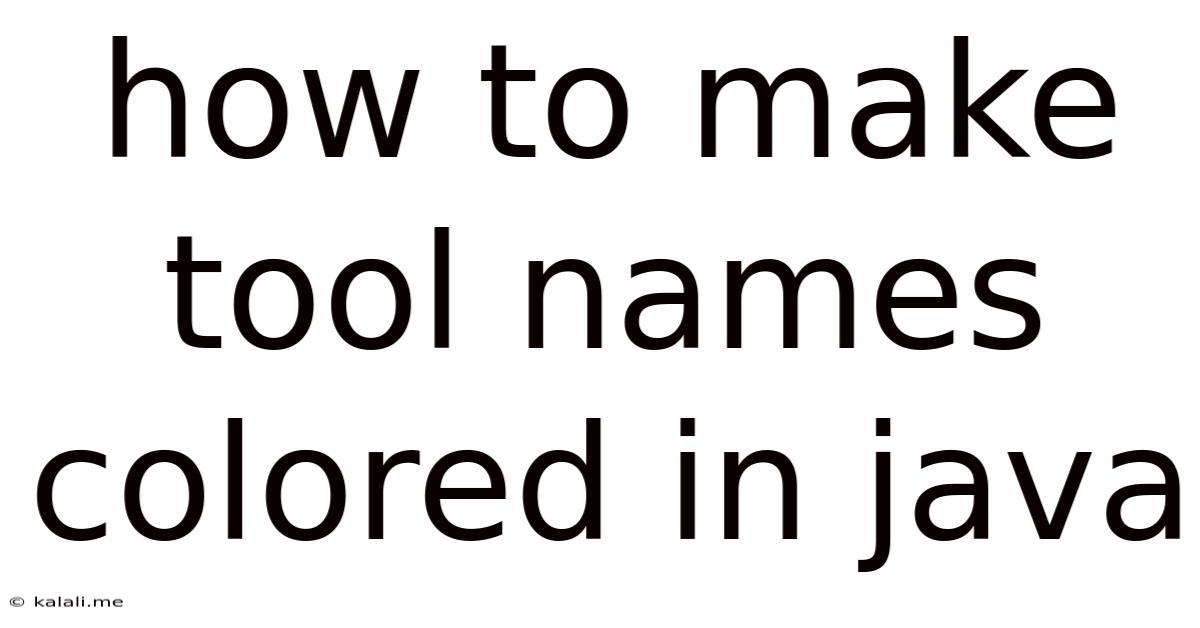
Table of Contents
How to Make Tool Names Colored in Java: A Comprehensive Guide
Want to add a splash of color to your Java console applications and make your tool names stand out? This guide provides a comprehensive walkthrough on how to achieve vibrant, eye-catching tool names using ANSI escape codes. This simple technique dramatically improves the user experience and makes your output more readable and engaging. We'll explore different methods and best practices to ensure your code is clean, efficient, and easy to maintain.
Understanding ANSI Escape Codes
The secret sauce behind colored text in Java's console lies in ANSI escape codes. These are special sequences of characters that control the formatting of text in terminals that support them. They allow you to change the color, style (bold, italic, underlined), and background of your text output. These codes aren't specific to Java; they're a standard supported by many operating systems and terminals.
The basic structure of an ANSI escape code is \u001B[<code_number>m
, where <code_number>
represents the specific formatting code. For instance, \u001B[31m
sets the text color to red.
Method 1: Direct ANSI Escape Code Implementation
This method involves directly embedding ANSI escape codes into your Java strings. It's the simplest approach but requires careful handling to ensure code readability and maintainability.
public class ColoredToolNames {
public static void main(String[] args) {
String redTool = "\u001B[31mMyRedTool\u001B[0m"; // Red text, reset to default
String greenTool = "\u001B[32mMyGreenTool\u001B[0m"; // Green text, reset to default
String blueTool = "\u001B[34mMyBlueTool\u001B[0m"; // Blue text, reset to default
System.out.println("Available Tools:");
System.out.println(redTool);
System.out.println(greenTool);
System.out.println(blueTool);
}
}
Remember to always include \u001B[0m
to reset the color to the default after each colored text segment. Otherwise, subsequent output will inherit the color.
Method 2: Creating a Helper Function
For better code organization and reusability, consider creating a helper function to encapsulate the ANSI escape code logic. This improves readability and makes it easier to manage colors in a larger project.
public class ColoredToolNames {
public static String colorText(String text, String colorCode) {
return "\u001B[" + colorCode + "m" + text + "\u001B[0m";
}
public static void main(String[] args) {
System.out.println("Available Tools:");
System.out.println(colorText("MyRedTool", "31"));
System.out.println(colorText("MyGreenTool", "32"));
System.out.println(colorText("MyBlueTool", "34"));
}
}
This approach is cleaner and more maintainable, particularly when dealing with many different colors.
Choosing and Using Colors
Here's a table of common ANSI escape codes for text colors:
Code | Color |
---|---|
30 | Black |
31 | Red |
32 | Green |
33 | Yellow |
34 | Blue |
35 | Magenta |
36 | Cyan |
37 | White |
You can also combine these with codes for text styles:
1
: Bold3
: Italic (not supported by all terminals)4
: Underlined
For example, \u001B[1;31m
would produce bold red text.
Handling Terminal Compatibility
Not all terminals support ANSI escape codes. To handle this, you could add a check to see if the terminal supports ANSI codes before applying them. However, this requires more advanced techniques and is often omitted for simpler applications.
Conclusion
Adding color to your tool names in Java significantly enhances user experience. By leveraging ANSI escape codes and implementing best practices like helper functions, you can create clean, efficient, and visually appealing console applications. Remember to choose colors judiciously for optimal readability and accessibility. Experiment with different color combinations and styles to find what works best for your project!
Latest Posts
Latest Posts
-
Why Do Nuns Wear Wedding Rings
May 29, 2025
-
My Washing Machine Drain Pipe Is Overflowing
May 29, 2025
-
How To Patch Gaps In Drywall
May 29, 2025
-
Is It Better To Speak Or Die
May 29, 2025
-
How To Ssh Using Private Key
May 29, 2025
Related Post
Thank you for visiting our website which covers about How To Make Tool Names Colored In Java . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.