How To Translate Along A Vector
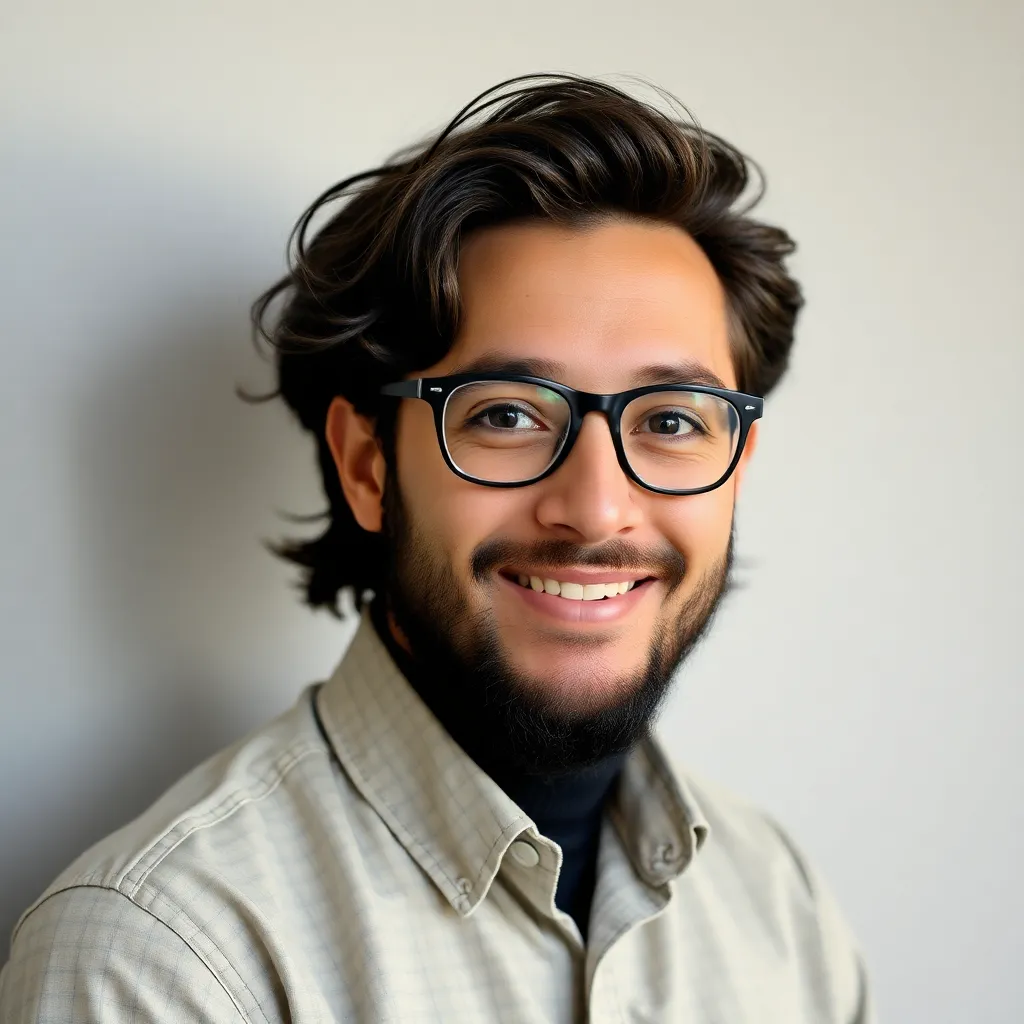
Kalali
Apr 02, 2025 · 6 min read
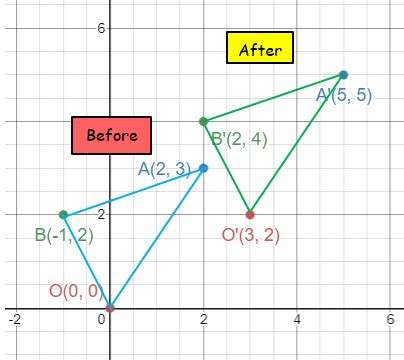
Table of Contents
How to Translate Along a Vector: A Comprehensive Guide
Translation along a vector is a fundamental concept in linear algebra and computer graphics with applications spanning diverse fields like game development, robotics, and image processing. Understanding this process is crucial for manipulating objects in space, simulating movement, and performing various geometric transformations. This comprehensive guide will explore the intricacies of vector translation, providing a detailed explanation accessible to both beginners and those with prior mathematical knowledge.
Understanding Vectors and Translation
Before diving into the mechanics of translation, let's establish a solid foundation in vector concepts. A vector is a mathematical object possessing both magnitude (length) and direction. It's often represented visually as an arrow, where the length corresponds to the magnitude and the arrowhead indicates the direction. Vectors are frequently denoted using bold lowercase letters (e.g., v, u) or with an arrow above (e.g., $\vec{v}$, $\vec{u}$).
Translation, in the context of vectors, refers to the movement of a point or object along a vector. This movement involves shifting the point or object parallel to the vector's direction by a distance equal to its magnitude. Imagine pushing an object along a straight path; that path defines the vector, and the distance you push it represents the vector's magnitude.
Key Vector Properties Relevant to Translation
- Components: Vectors are often represented using their components. In a 2D space, a vector v can be represented as (vx, vy), where vx and vy represent the horizontal and vertical components, respectively. Similarly, in 3D space, a vector is represented as (vx, vy, vz).
- Addition: Adding two vectors involves adding their corresponding components. For example, if u = (ux, uy) and v = (vx, vy), then u + v = (ux + vx, uy + vy).
- Scalar Multiplication: Multiplying a vector by a scalar (a single number) scales the vector's magnitude. If 'k' is a scalar, then kv = (kvx, kvy).
Translating Points and Objects
The process of translating a point or object along a vector involves adding the vector's components to the coordinates of the point or object. Let's consider the different scenarios:
Translating a Point in 2D Space
Let's say we have a point P with coordinates (Px, Py) and a translation vector v = (vx, vy). To translate point P along vector v, we add the components of v to the coordinates of P:
- Translated Point P' = (Px + vx, Py + vy)
This simple addition shifts the point P to a new location P' based on the vector's direction and magnitude.
Example:
If P = (2, 3) and v = (4, -1), then the translated point P' = (2 + 4, 3 + (-1)) = (6, 2).
Translating a Point in 3D Space
The principle remains the same for 3D space. For a point P = (Px, Py, Pz) and translation vector v = (vx, vy, vz), the translated point P' is:
- Translated Point P' = (Px + vx, Py + vy, Pz + vz)
Example:
If P = (1, 2, 3) and v = (-2, 1, 0), then P' = (1 + (-2), 2 + 1, 3 + 0) = (-1, 3, 3).
Translating an Object
Translating an object involves translating each of its constituent points. If an object is defined by a set of vertices (points), we apply the translation vector to each vertex to obtain the translated object. This is particularly crucial in computer graphics when manipulating shapes or models.
Consider a polygon: Each vertex of the polygon is translated using the method described above. The new set of translated vertices defines the translated polygon. This process is equally applicable to more complex 3D models composed of multiple polygons (meshes).
Implementing Translation in Code
The process of translation is straightforward to implement in various programming languages. Here's a simplified example using Python:
import numpy as np
def translate_point(point, vector):
"""Translates a point by a given vector.
Args:
point: A NumPy array representing the point coordinates (e.g., [x, y] or [x, y, z]).
vector: A NumPy array representing the translation vector (e.g., [vx, vy] or [vx, vy, vz]).
Returns:
A NumPy array representing the translated point coordinates.
"""
return point + vector
# Example usage:
point = np.array([2, 3])
vector = np.array([4, -1])
translated_point = translate_point(point, vector)
print(f"Translated point: {translated_point}") # Output: Translated point: [6 2]
point_3d = np.array([1, 2, 3])
vector_3d = np.array([-2, 1, 0])
translated_point_3d = translate_point(point_3d, vector_3d)
print(f"Translated 3D point: {translated_point_3d}") # Output: Translated 3D point: [-1 3 3]
This code snippet leverages the NumPy library for efficient vector operations. The translate_point
function directly implements the vector addition to perform the translation. This code can be easily adapted for higher dimensional spaces by simply changing the dimensions of the input arrays.
Applications of Vector Translation
The application of vector translation extends across many fields:
Computer Graphics and Game Development
- Character Movement: Translating character models in games involves applying translation vectors to move them across the game world.
- Camera Control: Manipulating camera positions and orientations often utilizes translation vectors to adjust the viewing perspective.
- Object Manipulation: Moving, rotating, and scaling objects in 3D modeling software relies on fundamental transformations, including translation.
Robotics
- Robot Arm Control: Programming the movements of robotic arms frequently involves calculating and applying translation vectors to position the end effector accurately.
- Path Planning: Generating trajectories for robots and autonomous vehicles necessitates the use of vector translation for determining successive positions.
Image Processing
- Image Registration: Aligning multiple images often involves translating one image relative to another, using vector-based techniques.
- Object Detection and Tracking: Tracking the movement of objects in a sequence of images utilizes vector translations to represent changes in position.
Physics and Engineering
- Simulations: Modeling the movement of objects in physics simulations extensively involves translating objects based on forces and velocities.
- CAD/CAM: Computer-aided design and manufacturing often employ vector translation for precise positioning and manipulation of designs.
Advanced Concepts and Extensions
While the fundamental concept of translation is relatively straightforward, several advanced concepts build upon it:
Transformations Matrices
Translation can be represented using transformation matrices, providing a more sophisticated and efficient way to combine multiple transformations, including rotations and scaling, into a single matrix operation. This is particularly useful in computer graphics and robotics.
Homogeneous Coordinates
Utilizing homogeneous coordinates allows for the representation of both translation and other transformations using matrix multiplication alone. This simplifies calculations and offers computational advantages.
Inverse Transformations
Finding the inverse translation vector allows for the reversal of the translation, effectively moving the object back to its original position.
Conclusion
Translation along a vector is a fundamental and widely used operation in various fields. Understanding this concept, its implementation, and its applications is crucial for anyone working with graphics, robotics, simulations, or other areas involving geometric transformations. While the basic principles are straightforward, mastering advanced techniques like transformation matrices and homogeneous coordinates unlocks even greater power and efficiency. The provided code examples offer a practical starting point for implementing translation, while the discussion of applications highlights the breadth of its impact. By understanding vector translation deeply, you will significantly enhance your ability to manipulate and model objects in both two-dimensional and three-dimensional space.
Latest Posts
Latest Posts
-
What Is 20 Percent Of 140
Apr 03, 2025
-
What Is The Waste Product Of Photosynthesis
Apr 03, 2025
-
Cuanto Es 33 Pies En Metros
Apr 03, 2025
-
How Many Ounces In 60 Ml
Apr 03, 2025
-
63 Out Of 90 As A Percentage
Apr 03, 2025
Related Post
Thank you for visiting our website which covers about How To Translate Along A Vector . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.