How To Write A Function For A Graph
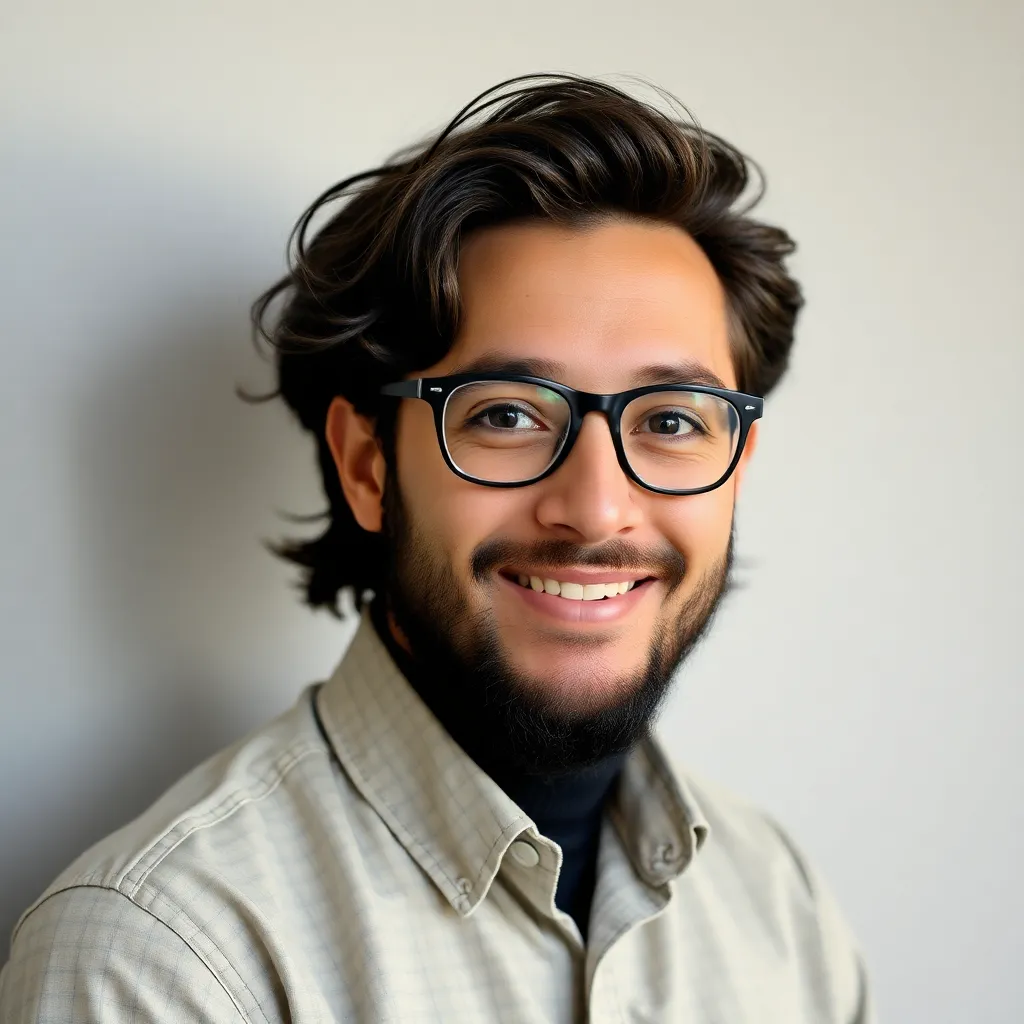
Kalali
May 10, 2025 · 3 min read
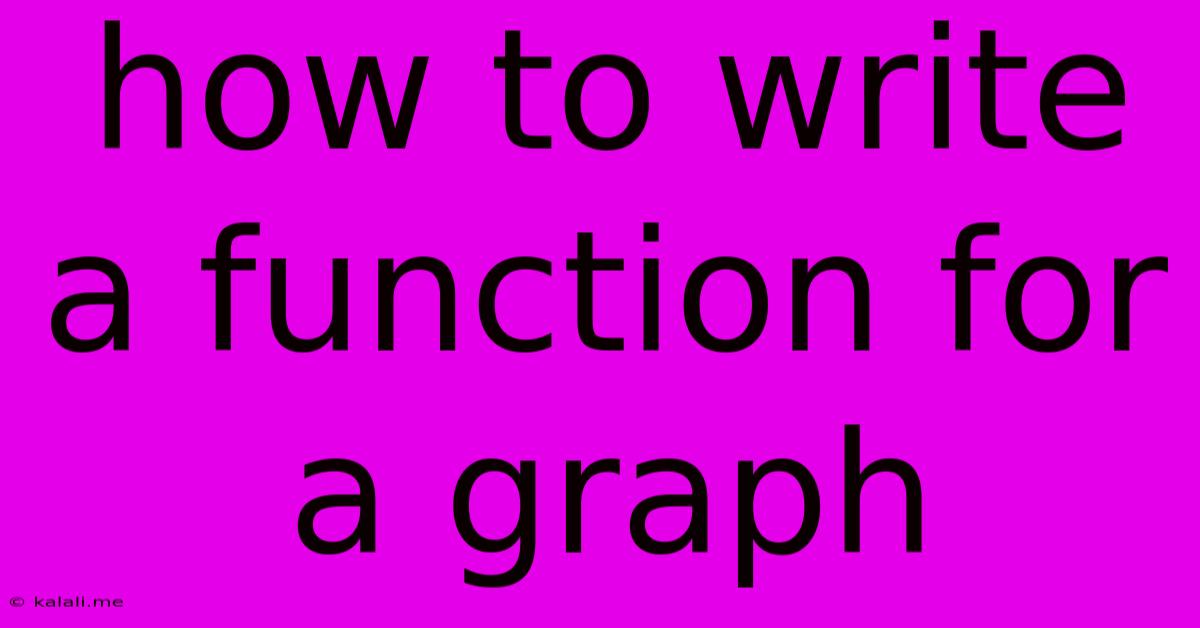
Table of Contents
How to Write a Function for a Graph: A Comprehensive Guide
This article provides a comprehensive guide on how to write a function to represent a graph, covering various approaches and considerations depending on the type of graph and desired functionality. Understanding how to represent a graph programmatically is crucial in various fields, including computer science, data analysis, and machine learning. We'll explore different representations and the advantages and disadvantages of each.
Understanding Graph Representations
Before diving into code, it's essential to understand how graphs are represented. A graph consists of nodes (vertices) and edges connecting those nodes. We primarily use two common representations:
-
Adjacency Matrix: This representation uses a 2D array where each element
matrix[i][j]
indicates whether there's an edge between nodei
and nodej
. A value of 1 typically denotes an edge, and 0 denotes no edge. For weighted graphs, the element represents the weight of the edge. -
Adjacency List: This representation uses a list of lists or a dictionary where each element represents a node, and its corresponding list contains its neighbors. For weighted graphs, each neighbor can be represented as a tuple (neighbor, weight).
Implementing Graph Functions in Python
Let's explore how to implement these representations and common graph functions in Python.
Adjacency Matrix Implementation
class GraphMatrix:
def __init__(self, num_vertices):
self.num_vertices = num_vertices
self.matrix = [[0] * num_vertices for _ in range(num_vertices)]
def add_edge(self, u, v, weight=1):
self.matrix[u][v] = weight
self.matrix[v][u] = weight # For undirected graph
def print_graph(self):
for row in self.matrix:
print(row)
# Example usage
graph = GraphMatrix(4)
graph.add_edge(0, 1)
graph.add_edge(0, 2)
graph.add_edge(1, 3)
graph.print_graph()
This code defines a class GraphMatrix
that uses a 2D list to represent the graph. The add_edge
method adds an edge between two vertices, and print_graph
displays the adjacency matrix. Remember to adjust the add_edge
method if you are working with a directed graph.
Adjacency List Implementation
class GraphList:
def __init__(self):
self.graph = {}
def add_edge(self, u, v, weight=1):
self.graph.setdefault(u, []).append((v, weight))
self.graph.setdefault(v, []).append((u, weight)) # For undirected graph
def print_graph(self):
for vertex, edges in self.graph.items():
print(f"Vertex {vertex}: {edges}")
# Example usage
graph = GraphList()
graph.add_edge(0, 1)
graph.add_edge(0, 2, weight=5)
graph.add_edge(1, 3)
graph.print_graph()
This code utilizes a dictionary to represent the adjacency list. Each key is a node, and its value is a list of its neighbors (and their weights, if applicable). The add_edge
method adds edges, and print_graph
displays the adjacency list. Again, consider modifying add_edge
for directed graphs.
Choosing the Right Representation
The choice between adjacency matrix and adjacency list depends on the specific application:
-
Adjacency Matrix: Efficient for checking if an edge exists between two nodes (O(1) time complexity). However, it can be space-inefficient for sparse graphs (graphs with relatively few edges).
-
Adjacency List: Space-efficient for sparse graphs. Checking for edge existence is O(degree(v)) where degree(v) is the number of edges connected to vertex v. More efficient for sparse graphs when traversal is needed.
Further Graph Algorithms
Once you have your graph represented, you can implement various graph algorithms, such as:
- Breadth-First Search (BFS): Traverses the graph level by level.
- Depth-First Search (DFS): Traverses the graph by going as deep as possible along each branch before backtracking.
- Dijkstra's Algorithm: Finds the shortest path between two nodes in a weighted graph.
- Minimum Spanning Tree (MST) algorithms (Prim's, Kruskal's): Finds a tree that connects all nodes with the minimum total edge weight.
This guide provides a foundational understanding of how to write functions for graphs. Remember to choose the most appropriate representation based on the characteristics of your graph and the algorithms you intend to use. Further exploration of graph algorithms will expand your ability to work with graph data structures.
Latest Posts
Latest Posts
-
200 Meters Is Equal To How Many Feet
May 10, 2025
-
How Many Grams Are In A Quarter Of An Ounce
May 10, 2025
-
What Is 15 Percent Of 140
May 10, 2025
-
What Are The Multiples Of 45
May 10, 2025
-
What Is The Percentage Of 80
May 10, 2025
Related Post
Thank you for visiting our website which covers about How To Write A Function For A Graph . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.