Java Does A Int Return Need To Be Boolean
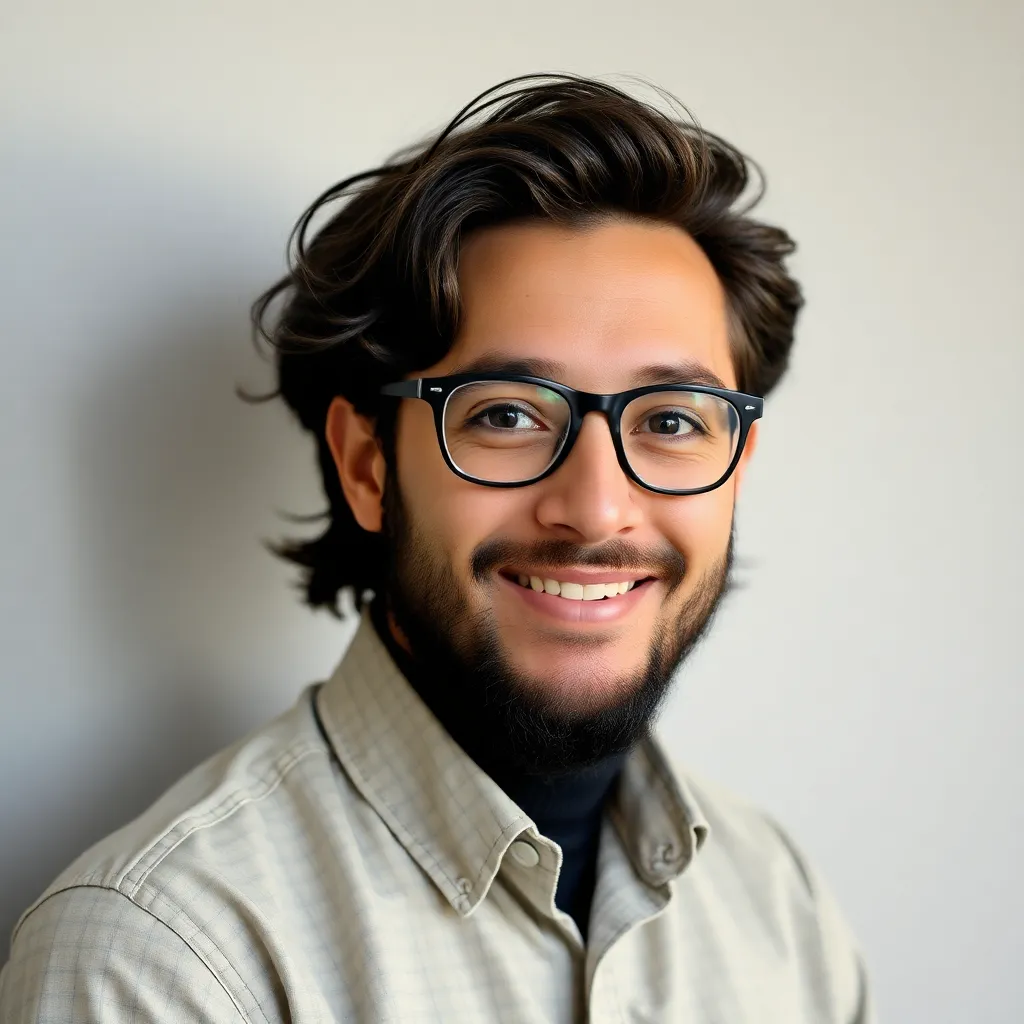
Kalali
May 25, 2025 · 3 min read
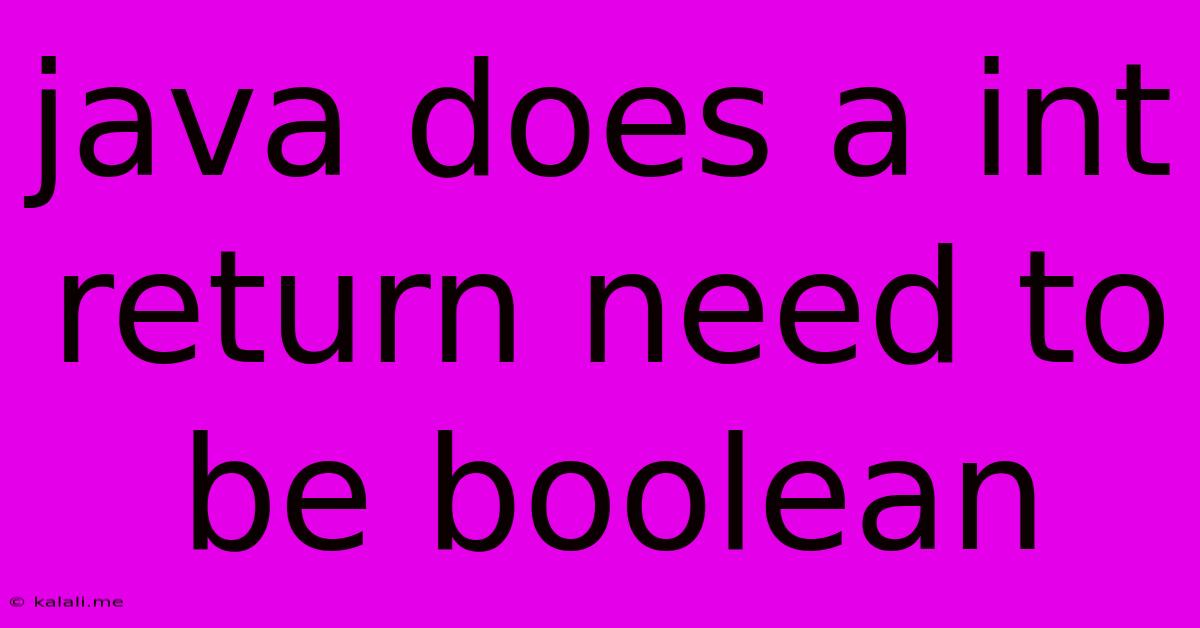
Table of Contents
Java: Does an int
Return Need to Be a Boolean? Understanding Return Types
This article clarifies the common misconception that an integer return type in Java needs to be a boolean. It's crucial to understand the fundamental differences between int
and boolean
data types and how they function within Java methods. The short answer is: No, an int
return type does not need to be a boolean. They serve entirely different purposes.
This misunderstanding often arises when programmers are grappling with conditional logic and method design. Let's delve into the specifics.
Understanding int
and boolean
Data Types
-
int
: Represents an integer value, a whole number without decimal points. It's used to store numerical data, such as counts, indices, or results of calculations. The range of values anint
can hold is -2,147,483,648 to 2,147,483,647. -
boolean
: Represents a logical value, eithertrue
orfalse
. It's used to express conditions, results of comparisons, or flags indicating a state.
The key difference lies in their purpose: int
stores numerical data, while boolean
stores truth values. They are fundamentally incompatible in terms of direct assignment or comparison. You can't directly assign an int
to a boolean
variable, and vice versa, without explicit conversion (which often involves a logical condition).
When to Use int
vs. boolean
Return Types
The choice between an int
and a boolean
return type depends entirely on the method's intended functionality.
-
Use
int
when:- Your method needs to return a numerical value as its result. Examples include:
- Calculating the sum of numbers.
- Returning the size of a data structure.
- Returning an index or position.
- Representing a quantity or measurement.
- Your method needs to return a numerical value as its result. Examples include:
-
Use
boolean
when:- Your method needs to indicate a true/false condition. Examples include:
- Checking if a value exists.
- Determining if a condition is met.
- Validating input data.
- Signaling success or failure of an operation.
- Your method needs to indicate a true/false condition. Examples include:
Example Scenarios
Let's illustrate with some code examples:
Example 1: int
Return Type
public class IntegerReturn {
public static int addNumbers(int a, int b) {
return a + b; // Returns the sum as an integer
}
public static void main(String[] args) {
int sum = addNumbers(5, 3);
System.out.println("Sum: " + sum); // Output: Sum: 8
}
}
Example 2: boolean
Return Type
public class BooleanReturn {
public static boolean isEven(int number) {
return number % 2 == 0; // Returns true if even, false otherwise
}
public static void main(String[] args) {
boolean isEvenNumber = isEven(6);
System.out.println("Is 6 even? " + isEvenNumber); // Output: Is 6 even? true
}
}
These examples clearly demonstrate the distinct roles of int
and boolean
return types. Trying to force an int
to act like a boolean
(or vice-versa) would lead to incorrect logic and potentially unexpected results.
Conclusion
In summary, choosing between an int
and a boolean
return type in Java hinges on the nature of the data your method needs to produce. They are distinct data types with separate purposes, and understanding their differences is fundamental to writing correct and efficient Java code. An int
return type should never be confused with, or substituted for, a boolean
return type, unless you explicitly convert the integer value to represent a truth condition (e.g., 0 for false, any other integer for true). This conversion should always be handled with caution and clarity within your code.
Latest Posts
Latest Posts
-
Cards In Rise Of The Tomb Raider
May 25, 2025
-
Wiring A Ceiling Fan With Two Switches And Remote
May 25, 2025
-
Cannot Compute Exact P Value With Ties
May 25, 2025
-
How To Get Companions Out Of Power Armor
May 25, 2025
-
Its No Fun When The Rabbit Has The Gun
May 25, 2025
Related Post
Thank you for visiting our website which covers about Java Does A Int Return Need To Be Boolean . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.