Linux Syscall Task Struct Get Uid
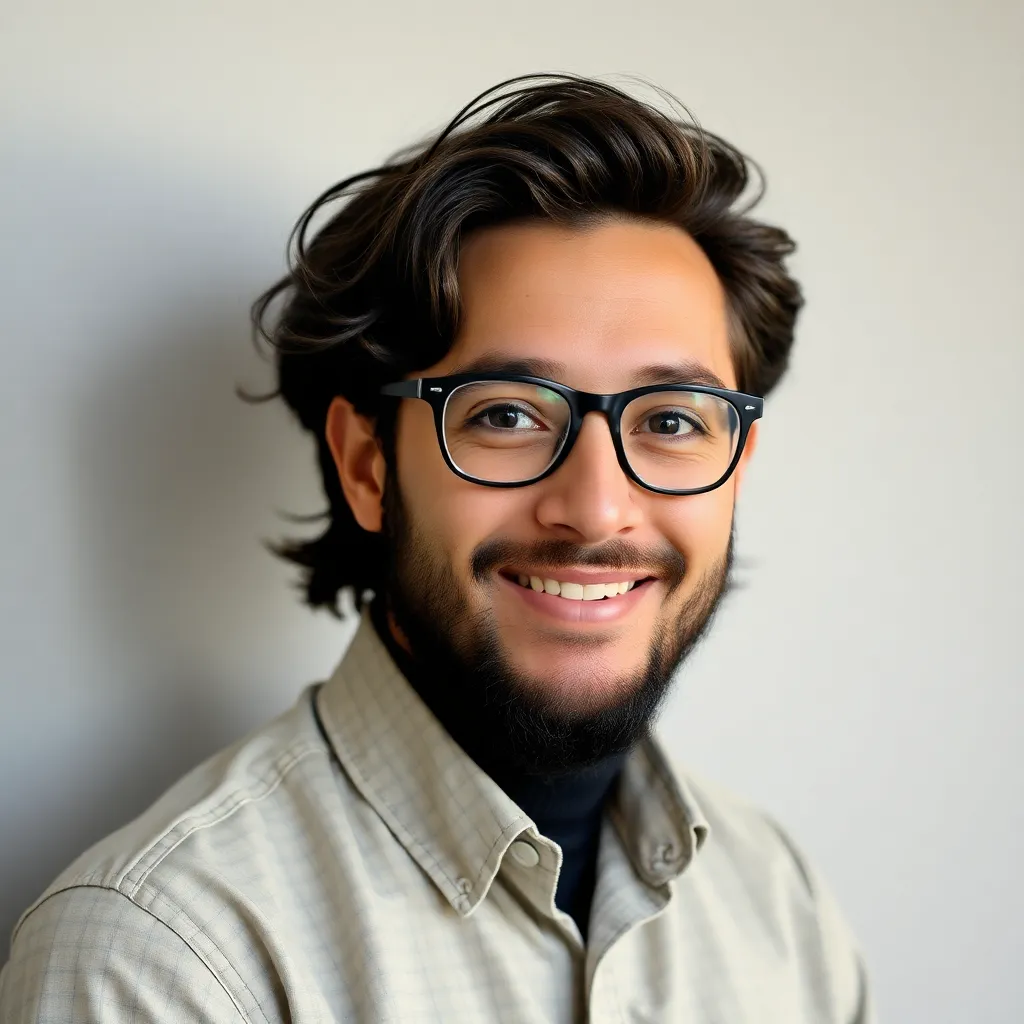
Kalali
May 23, 2025 · 3 min read
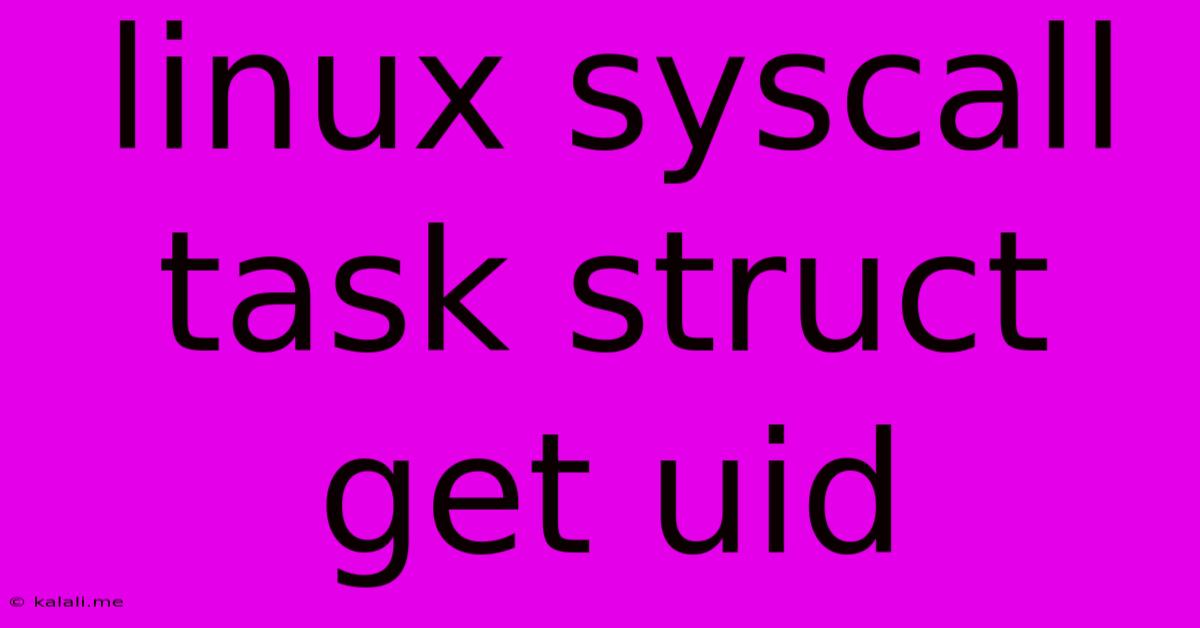
Table of Contents
Linux Syscall: Efficiently Retrieving User IDs (UIDs) from the Task Struct
Getting a User ID (UID) from a Linux task structure is a common operation in system programming, particularly within kernel modules or security-related applications. Understanding how to efficiently retrieve this information is crucial for writing robust and performant code. This article dives into the intricacies of accessing UIDs from the task_struct
in Linux, focusing on performance and security considerations. We'll explore different methods and highlight best practices.
Understanding the task_struct
is fundamental. This data structure, central to the Linux kernel's process management, contains extensive information about a process, including its UID. However, directly accessing this data requires careful consideration of kernel memory management and potential race conditions.
Navigating the task_struct
to Retrieve UIDs
The UID isn't a single field; instead, multiple fields within task_struct
relate to user identification. These include:
real_uid
(Real UID): The user ID of the original process. This is the UID used for file permissions and access control.effective_uid
(Effective UID): The UID used for determining permissions when a process executes. This can change due tosetuid()
system calls.fs_uid
(File System UID): Primarily relevant for filesystem access controls.saved_uid
(Saved UID): Used to store the original UID before a privilege change.
The choice of which UID to retrieve depends on the specific needs of your application. For most security checks, effective_uid
is the relevant field.
Methods for Accessing UIDs from the task_struct
Several methods exist for retrieving UIDs, each with its own trade-offs:
-
Direct Access (using
task_struct
directly): This method is generally discouraged unless absolutely necessary due to potential race conditions and kernel memory management complexities. Incorrect handling can lead to kernel panics or data corruption. -
Using
current
macro: Inside a kernel module, thecurrent
macro provides a pointer to the currently executing task'stask_struct
. This is a safe way to access information about the current process. Accessing UIDs would then becurrent->real_uid
,current->effective_uid
, etc. -
Using
task_pid_nr_ns
andfind_task_by_vpid
: If you need to obtain the UID of a process by its PID, you need to use functions likefind_task_by_vpid
(for virtual PIDs) to locate the correcttask_struct
and then access the desired UID field. This provides flexibility but introduces the complexity of PID namespace handling. -
Utilizing user namespaces: If your application deals with user namespaces, you must account for the mapping between user namespaces when accessing UIDs. Incorrect handling can lead to security vulnerabilities. Always ensure your code accounts for this abstraction layer.
Example: Retrieving the Effective UID using the current
macro (Illustrative)
This example is conceptual and requires execution within a kernel module's context. Remember, direct kernel manipulation requires a deep understanding of the kernel API and should be done with utmost caution. This code snippet is for illustrative purposes only and needs appropriate error handling and context.
#include
#include
// ... inside a kernel function ...
uid_t effective_uid = current->effective_uid;
printk(KERN_INFO "Effective UID: %u\n", from_kuid_munged(current_user_ns(), effective_uid));
// ...
Security and Performance Considerations
- Race Conditions: Accessing the
task_struct
directly without proper synchronization can lead to race conditions, potentially resulting in inconsistent or incorrect UIDs. - Kernel Memory Management: Incorrect access to kernel memory can lead to kernel panics or system instability.
- Error Handling: Always include robust error handling to gracefully handle situations where the
task_struct
might not be found or accessible. - Context: Understanding the context in which you're accessing the UID (e.g., user space vs. kernel space) is critical for choosing the correct methods and ensuring security.
Retrieving UIDs from the task_struct
is a potent yet sensitive operation. This requires meticulous programming and a deep understanding of the Linux kernel's internals and security model. Always prioritize using safer and more robust approaches, emphasizing correct error handling and avoiding direct manipulation whenever possible. Consider employing higher-level APIs whenever applicable to minimize the risk of errors.
Latest Posts
Latest Posts
-
What Is A Quarter Of A Million
Jul 02, 2025
-
Which Of The Following Is True Concerning A Dao
Jul 02, 2025
-
How Long Can Catfish Live Out Of Water
Jul 02, 2025
-
Is Kanye West Related To Cornel West
Jul 02, 2025
-
Olivia Needs To Provide A Visual Summary
Jul 02, 2025
Related Post
Thank you for visiting our website which covers about Linux Syscall Task Struct Get Uid . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.