Liust Of Soql Inside For Loop
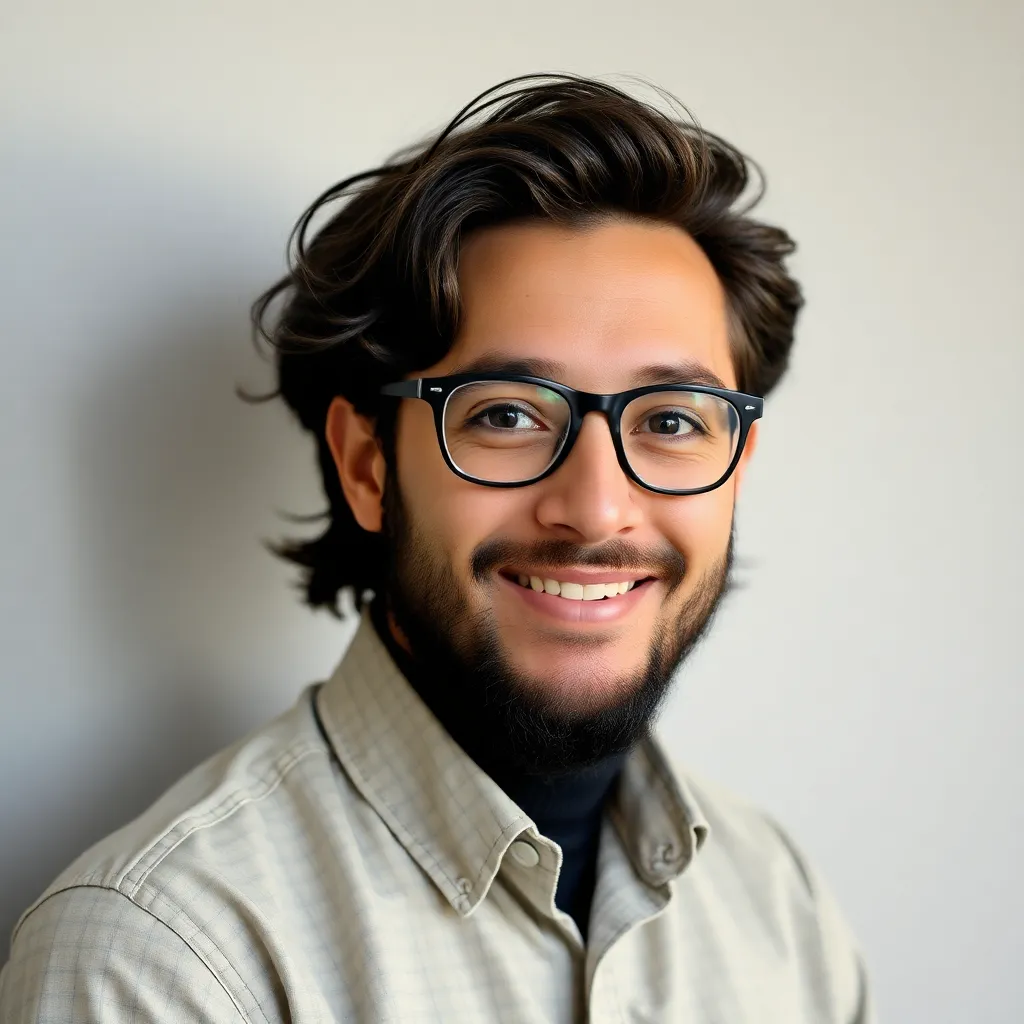
Kalali
May 24, 2025 · 3 min read
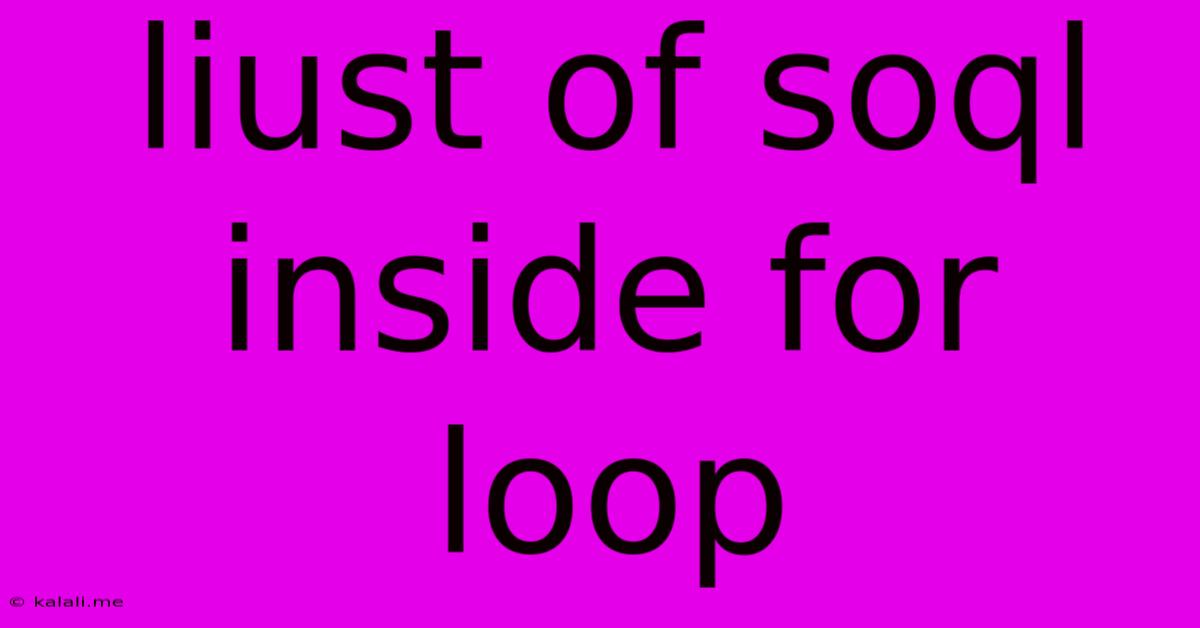
Table of Contents
SOQL Inside FOR Loops: Best Practices and Potential Pitfalls
This article explores the complexities of using SOQL queries inside FOR loops in Apex, highlighting best practices and potential performance pitfalls. Understanding these nuances is crucial for writing efficient and scalable Salesforce applications. We'll cover various scenarios, alternative approaches, and provide guidance on optimizing your code for optimal performance.
Why you might use SOQL inside a FOR loop:
Often, developers find themselves needing to retrieve data based on values obtained within a loop. For example, you might iterate through a list of Account IDs and retrieve the corresponding Contact records for each account. This seems straightforward, but it can quickly lead to performance issues if not handled carefully.
The Problem with Excessive SOQL Queries:
The primary concern with using SOQL inside a FOR loop is the potential for governor limits. Each SOQL query consumes governor limits, and excessive queries can lead to exceeding these limits, resulting in runtime errors. This is especially true when dealing with large datasets. Executing numerous individual SOQL queries within a loop is highly inefficient and should be avoided whenever possible.
Strategies for Optimizing SOQL Queries within Loops:
Several strategies can mitigate the performance impact of SOQL within FOR loops. The key is to reduce the number of queries executed. Here are some effective approaches:
1. Using the IN
operator:
This is the most effective approach. Instead of querying for each record individually, use the IN
operator to fetch multiple records in a single query. This significantly reduces the number of SOQL queries.
List accounts = [SELECT Id FROM Account WHERE Id IN :accountIds];
This single query retrieves all Accounts whose IDs are present in the accountIds
list. Replace accountIds
with your list of IDs obtained from your loop's previous iteration.
2. Pre-fetching related records with subqueries:**
If you need to access related data, using subqueries can avoid unnecessary SOQL calls within the loop. This approach retrieves related records alongside the parent records in a single query.
List accounts = [SELECT Id, (SELECT Id FROM Contacts) FROM Account WHERE Id IN :accountIds];
This retrieves all Accounts and their associated Contacts in one go.
3. Using Maps for Efficient Data Access:**
After retrieving data using the IN
operator, convert the result into a map for faster lookups within the loop. This avoids repeatedly querying the database for the same data.
Map accountMap = new Map();
for (Account acc : [SELECT Id FROM Account WHERE Id IN :accountIds]) {
accountMap.put(acc.Id, acc);
}
// ... later in the loop ...
Account currentAccount = accountMap.get(accountId);
4. Batch Apex for Large Datasets:**
For extremely large datasets, consider using Batch Apex. Batch Apex processes data in smaller batches, avoiding governor limit issues. This is particularly relevant when dealing with millions of records.
Example Scenario and Optimized Solution:
Let's say you want to update the OwnerId
of several Contacts based on their Account's OwnerId
. A naive approach might involve a FOR loop with a SOQL query inside:
(Inefficient Approach):
List contacts = [SELECT Id,AccountId FROM Contact WHERE AccountId IN :accountIds];
for(Contact con : contacts){
Account acc = [SELECT OwnerId FROM Account WHERE Id = :con.AccountId]; //Inefficient SOQL inside loop
con.OwnerId = acc.OwnerId;
}
update contacts;
(Optimized Approach):
Map accountOwnerMap = new Map();
for(Account acc : [SELECT Id,OwnerId FROM Account WHERE Id IN :accountIds]){
accountOwnerMap.put(acc.Id, acc.OwnerId);
}
List contactsToUpdate = new List();
for(Contact con : [SELECT Id,AccountId FROM Contact WHERE AccountId IN :accountIds]){
con.OwnerId = accountOwnerMap.get(con.AccountId);
contactsToUpdate.add(con);
}
update contactsToUpdate;
The optimized version reduces multiple SOQL queries to just two, significantly improving performance.
Conclusion:
Using SOQL inside FOR loops requires careful consideration. By employing techniques like the IN
operator, subqueries, maps, and potentially Batch Apex, you can significantly improve the efficiency and scalability of your Salesforce Apex code. Remember, minimizing the number of SOQL queries is paramount for optimal performance and avoiding governor limit violations.
Latest Posts
Latest Posts
-
Another Word For States In An Essay
May 24, 2025
-
How To Install A Gfci Outlet
May 24, 2025
-
How Many Lbs Of Meat Per Person
May 24, 2025
-
Tended To As A Squeaky Wheel
May 24, 2025
-
How To Pull Out A Stripped Bolt
May 24, 2025
Related Post
Thank you for visiting our website which covers about Liust Of Soql Inside For Loop . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.