Magento 2 Empty Current Cart In Js
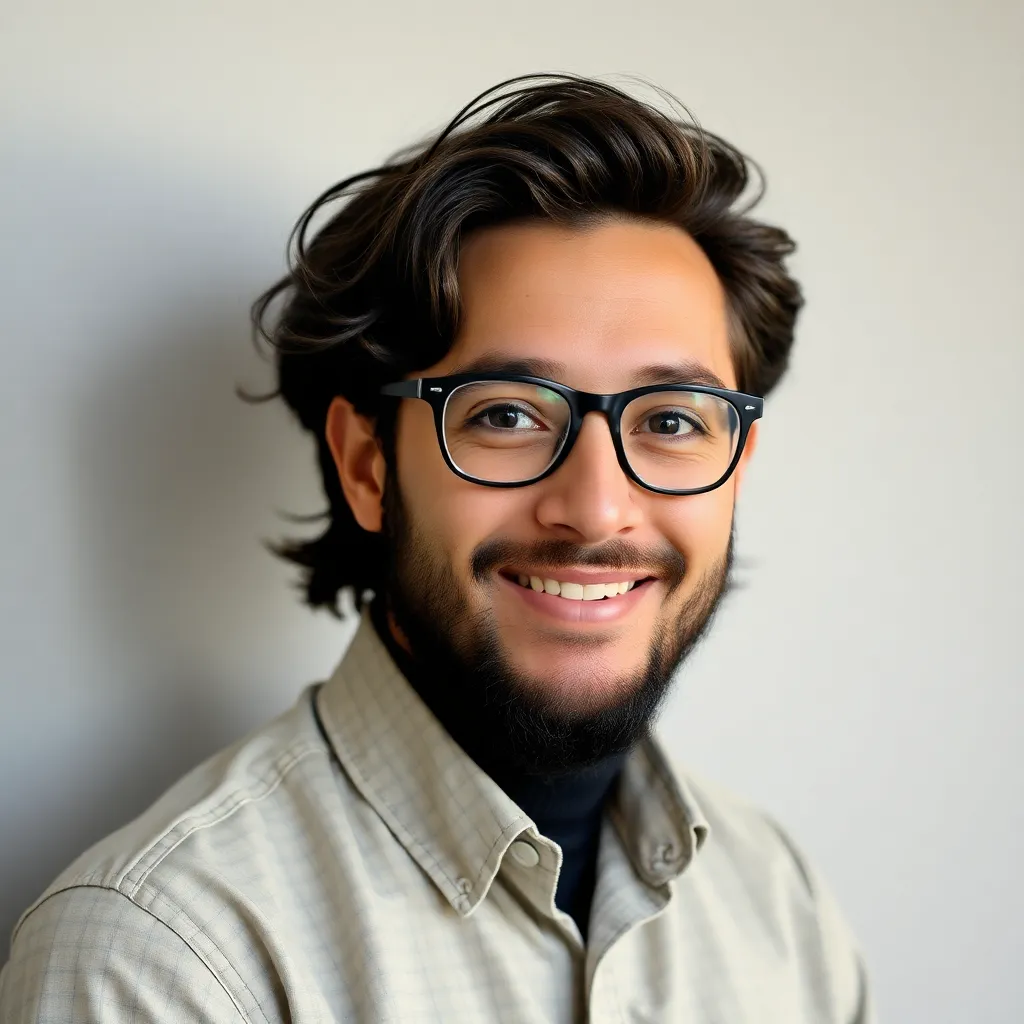
Kalali
May 23, 2025 · 3 min read
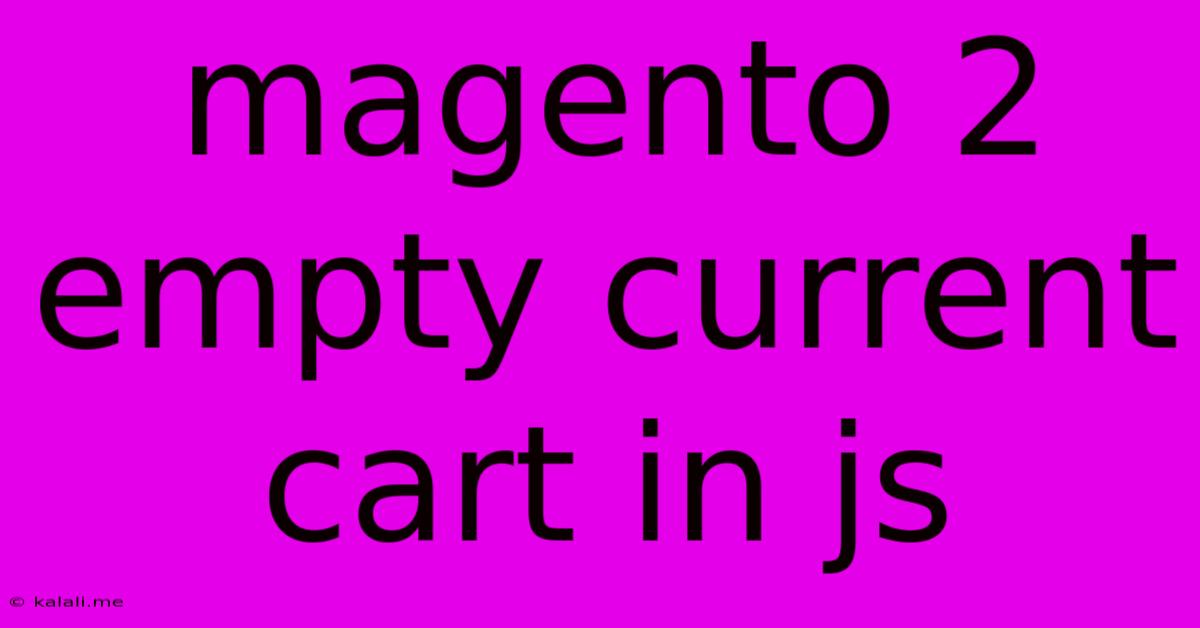
Table of Contents
How to Empty the Magento 2 Shopping Cart Using JavaScript
Magento 2 provides a robust e-commerce platform, but sometimes you need custom JavaScript functionality to enhance the user experience. One such enhancement is the ability to programmatically empty the shopping cart using JavaScript. This is particularly useful for advanced features like one-click checkout integrations or customized checkout flows. This article will guide you through the process of emptying the Magento 2 shopping cart using JavaScript, explaining the code, considerations, and potential use cases.
This guide focuses on client-side JavaScript manipulation. It does not cover server-side cart clearing, which involves different methods and security considerations. Client-side cart emptying is primarily used for improving UX within the existing storefront.
Understanding the Process
Emptying the Magento 2 shopping cart using JavaScript relies on leveraging Magento's AJAX capabilities to communicate with the server and remove items. We'll use a simple AJAX request to call the appropriate Magento endpoint responsible for cart management. This avoids a full page reload, providing a smoother user experience.
Implementing the JavaScript Code
The following JavaScript code snippet demonstrates how to empty the cart. Remember to adjust the URL according to your Magento 2 installation's structure. The URL typically points to a REST endpoint that handles cart management.
function emptyCart() {
// Replace with your Magento 2 REST endpoint for emptying the cart.
const url = '/rest/V1/carts/mine'; //This may need modification depending on your Magento instance configuration.
fetch(url, {
method: 'DELETE',
headers: {
'Content-Type': 'application/json',
// Add any necessary authorization headers here if required
},
})
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.then(data => {
// Handle successful cart emptying. Update UI elements accordingly.
console.log('Cart emptied successfully:', data);
// For example, update a cart count display:
document.getElementById('cart-count').textContent = 0;
// Redirect to a confirmation page or update the cart display
window.location.href = '/checkout/cart/'; //Or any other page as needed
})
.catch(error => {
// Handle errors during the cart emptying process.
console.error('Error emptying cart:', error);
// Display an error message to the user
alert('There was an error emptying your cart. Please try again later.');
});
}
// Example of attaching the function to a button click:
document.getElementById('empty-cart-button').addEventListener('click', emptyCart);
Important Considerations
- Authorization: Depending on your Magento 2 setup and security configuration, you might need to include authorization headers (like a CSRF token or an API key) in the
fetch
request to authenticate the request. Consult your Magento 2 documentation for the specific authentication methods employed. - Error Handling: The provided code includes basic error handling. You should enhance this to provide more informative error messages to the user, handle different types of errors gracefully, and potentially log errors for debugging.
- UI Updates: After successfully emptying the cart, update your UI elements (like cart item count displays or cart summary sections) to reflect the empty cart state.
- URL Structure: The
url
variable is crucial. Ensure it points to the correct REST endpoint in your Magento instance. You might need to inspect your network requests in your browser's developer tools to determine the exact URL used by Magento for cart management. - Magento Version: The exact API endpoints might vary slightly depending on your Magento 2 version. Check your Magento 2's API documentation for the most accurate information.
Conclusion
Emptying the Magento 2 shopping cart using JavaScript offers a powerful way to customize the checkout experience. By understanding the process and implementing the code correctly, you can create a seamless and engaging e-commerce experience for your customers. Remember to prioritize robust error handling and adapt the code to fit your specific Magento 2 configuration. Always test thoroughly before deploying any changes to your production environment.
Latest Posts
Latest Posts
-
Gears Of War 4 Trading Weapons
May 23, 2025
-
5 Color Theorem Graph Theory Proof Inductino
May 23, 2025
-
Can I Build My Own Runway
May 23, 2025
-
Captain America I Understood That Reference
May 23, 2025
-
Home Computer Versus Cell Phone Facebook Accounts
May 23, 2025
Related Post
Thank you for visiting our website which covers about Magento 2 Empty Current Cart In Js . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.