Magento 2 Get All Products From Cart In Js
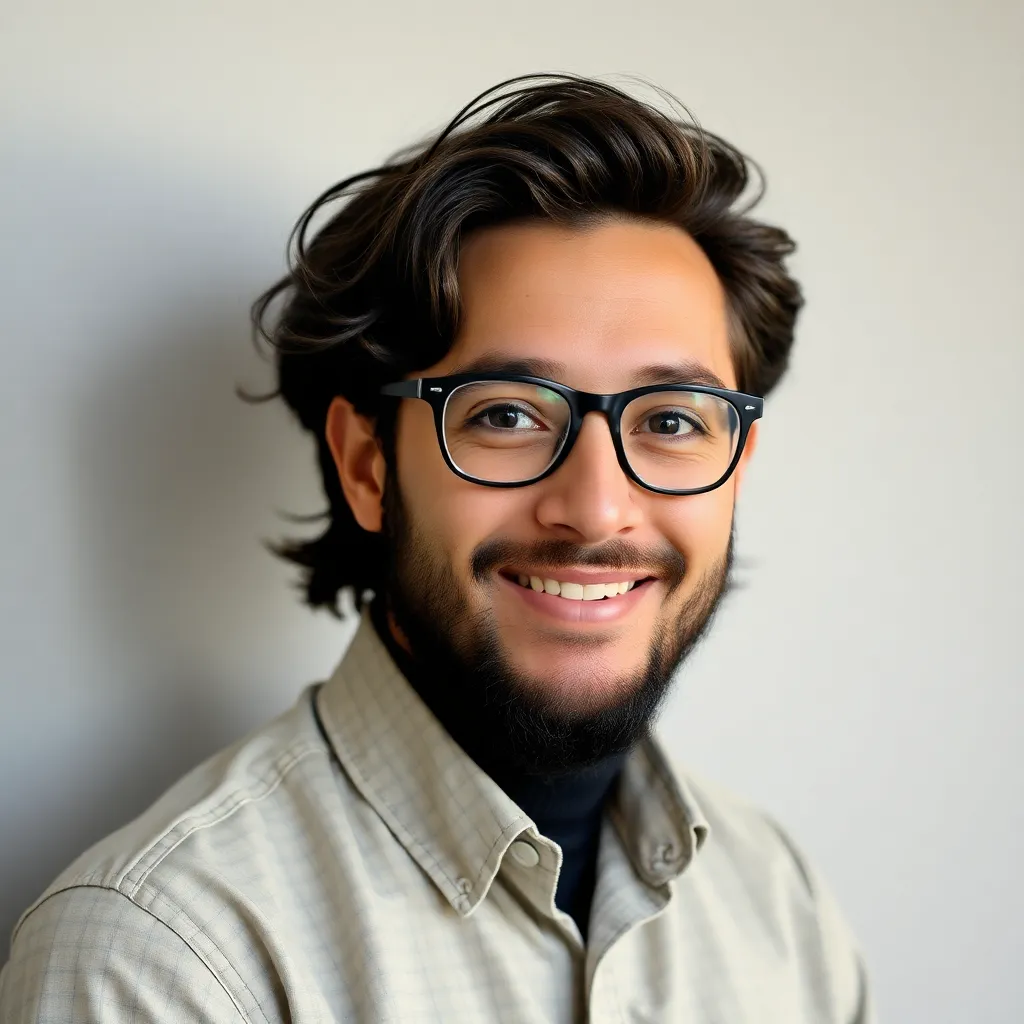
Kalali
May 25, 2025 · 3 min read
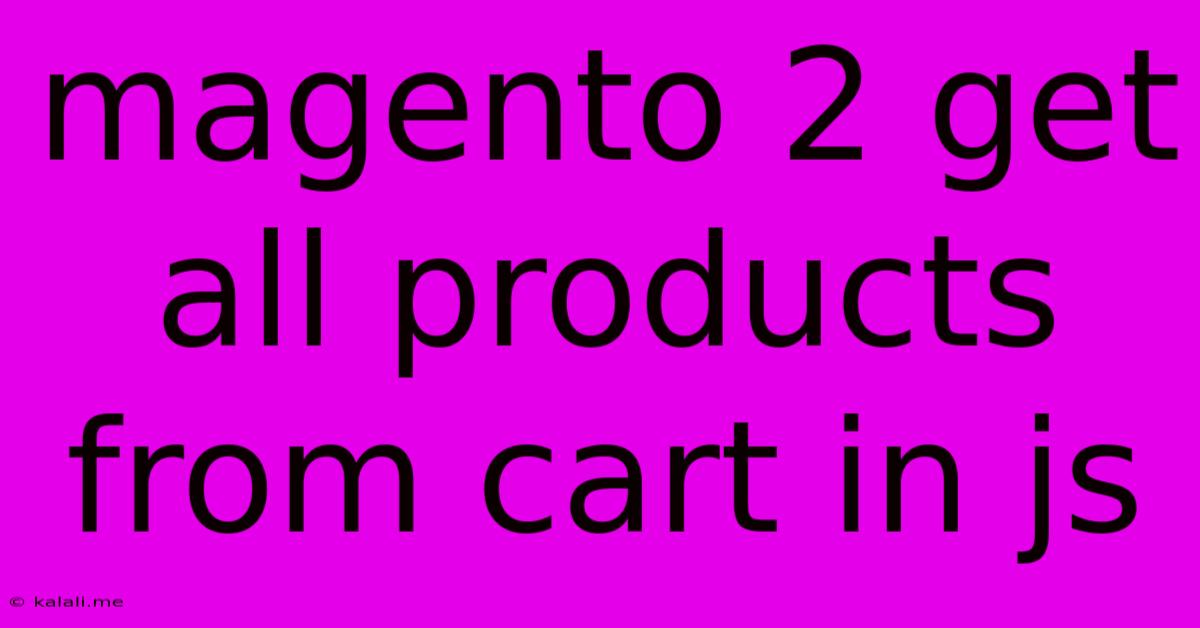
Table of Contents
Magento 2: Accessing All Cart Products with JavaScript
Getting all products from the shopping cart using JavaScript in Magento 2 is a common requirement for enhancing the customer experience. This might be for displaying a cart summary on a different page, creating custom checkout features, or integrating with third-party services. This guide will walk you through the process, covering different approaches and best practices. Understanding how to efficiently retrieve this data is crucial for building robust and performant Magento 2 extensions.
Understanding the Approach
Magento 2 uses a REST API to interact with its backend. To retrieve cart data via JavaScript, you'll need to make a request to the appropriate endpoint. This requires understanding the Magento 2 REST API structure and utilizing JavaScript's fetch
API (or a library like Axios) to make the request and handle the response.
Method 1: Using Magento's REST API and fetch
This method leverages Magento's built-in REST API. This is generally preferred for its security and maintainability.
First, you need to know the correct endpoint for retrieving cart items. Typically, this is:
/rest/V1/carts/mine/items
Then, we use fetch
to make the request:
fetch('/rest/V1/carts/mine/items', {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + window.customerToken // Replace with your authentication method
}
})
.then(response => response.json())
.then(data => {
// Process the 'data' which contains an array of cart items.
console.log(data);
//Example of iterating through the items:
data.forEach(item => {
console.log("Product ID:", item.product_id);
console.log("Product Name:", item.product_name);
console.log("Quantity:", item.qty);
// Access other properties as needed
});
})
.catch(error => console.error('Error:', error));
Important Considerations:
- Authentication (
window.customerToken
): TheAuthorization
header needs a valid token for authentication. This token is usually obtained during the customer login process. You'll need to integrate this authentication method into your code. The specific method varies based on your Magento setup and security configuration. - Error Handling: The
.catch
block is crucial for handling potential network errors or API errors. Robust error handling is essential for a smooth user experience. - Data Structure: The response (
data
) is an array of objects, each representing a product in the cart. Examine the structure ofdata
in your browser's console to understand the available properties (e.g.,product_id
,qty
,price
,name
, etc.). You can access these properties as demonstrated in the example. - Asynchronous Operations: Remember that
fetch
is asynchronous. The code within the.then
block executes only after the API response is received.
Method 2: Using a JavaScript library (Axios)
Axios simplifies making HTTP requests. If you're already using Axios in your project, it provides a cleaner and more feature-rich way to handle API calls.
axios.get('/rest/V1/carts/mine/items', {
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + window.customerToken
}
})
.then(response => {
// Process the response data
const cartItems = response.data;
// ... your code to handle cartItems ...
})
.catch(error => {
//Handle errors
console.error(error);
});
Remember to include the Axios library in your project.
Best Practices:
- Security: Always handle sensitive data securely. Never expose API keys or tokens directly in your frontend code.
- Error Handling: Implement comprehensive error handling to gracefully manage potential issues.
- Efficiency: Avoid unnecessary API calls. Retrieve only the necessary data.
- Caching: Consider using caching mechanisms to reduce the load on the server and improve performance, especially for frequently accessed data.
- Maintainability: Write clean, well-documented code to make it easier to maintain and update your code.
By following these guidelines and choosing the appropriate method (either fetch
or Axios), you can effectively retrieve all products from the Magento 2 cart using JavaScript. Remember to adapt the code to your specific context and security requirements.
Latest Posts
Latest Posts
-
Din Tai Fung Green Bean Recipe
May 25, 2025
-
Where To Watch Boku No Pico
May 25, 2025
-
How To Remove Ink From Paper
May 25, 2025
-
After A Colon Do You Capitalize
May 25, 2025
-
How Long Are Beans Good For In The Fridge
May 25, 2025
Related Post
Thank you for visiting our website which covers about Magento 2 Get All Products From Cart In Js . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.