Matrix Multiplication To Get Diagonal Elements
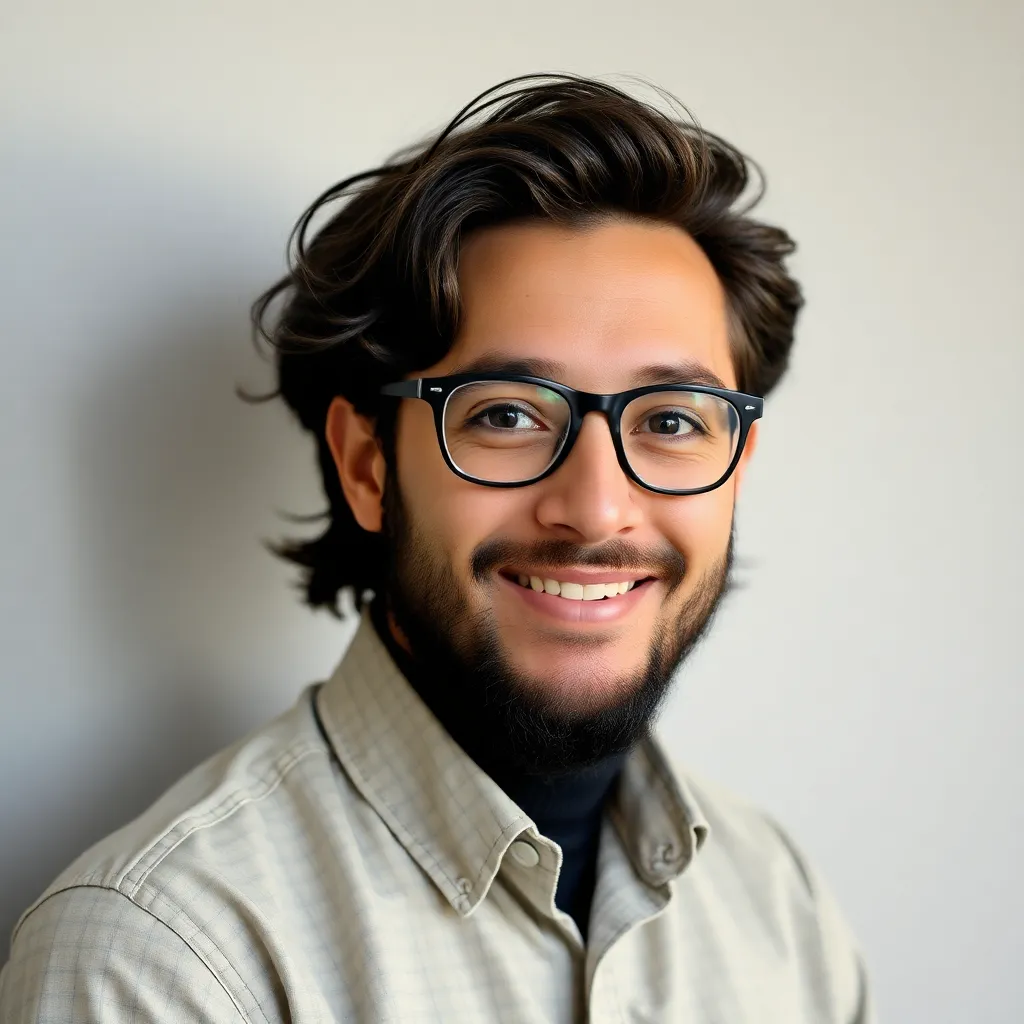
Kalali
May 23, 2025 · 3 min read
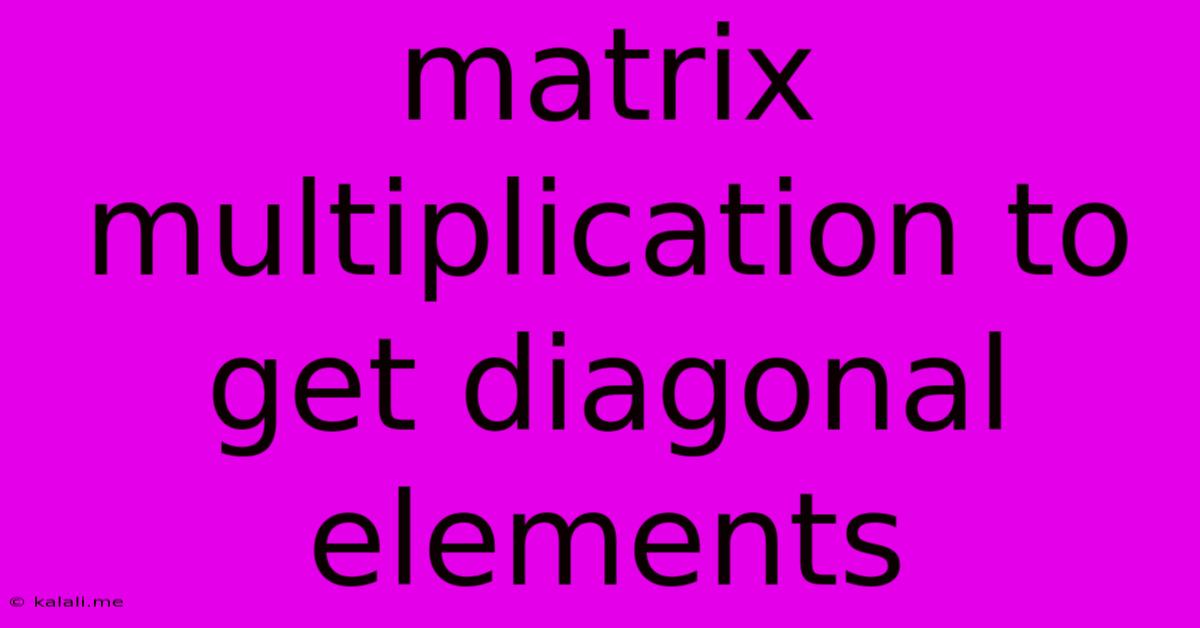
Table of Contents
Efficiently Extracting Diagonal Elements from Matrix Multiplication
Matrix multiplication is a fundamental operation in linear algebra with widespread applications in computer science, machine learning, and data science. Often, we're not interested in the entire resulting matrix, but only specific elements, such as the diagonal elements. This article explores efficient methods for extracting these diagonal elements directly, bypassing the computation of the entire matrix product. Understanding these techniques can significantly improve computational efficiency, especially when dealing with large matrices.
Calculating the entire product of two matrices, only to then extract the diagonal, is computationally wasteful. Let's explore techniques focusing solely on the diagonal.
Understanding the Basics of Matrix Multiplication
Before diving into optimization strategies, let's briefly review standard matrix multiplication. Given two matrices, A (m x n) and B (n x p), their product C (m x p) is defined as:
C<sub>ij</sub> = Σ<sub>k=1</sub><sup>n</sup> A<sub>ik</sub> * B<sub>kj</sub>
This means each element C<sub>ij</sub> is the dot product of the i-th row of A and the j-th column of B.
Direct Calculation of Diagonal Elements
To obtain only the diagonal elements of the resulting matrix C, we only need to calculate C<sub>ii</sub> for i = 1, ..., min(m, p). This simplifies the equation to:
C<sub>ii</sub> = Σ<sub>k=1</sub><sup>n</sup> A<sub>ik</sub> * B<sub>ki</sub>
This formula directly computes the i-th diagonal element without calculating any other elements of the resulting matrix. This approach already provides a considerable performance boost compared to full matrix multiplication, especially for large matrices where the majority of elements would be discarded.
Utilizing Vectorization and Optimized Libraries
Modern programming languages and libraries like NumPy (Python) or Eigen (C++) offer highly optimized functions for vector and matrix operations. These libraries often leverage hardware acceleration (like SIMD instructions) to achieve significant speed improvements. Utilizing these built-in functions for dot products within the loop described above can further enhance performance. This approach leverages the power of optimized low-level implementations, outperforming manual loop implementations in most cases.
Considerations for Sparse Matrices
If your matrices are sparse (containing many zero elements), specialized algorithms for sparse matrix multiplication should be considered. These algorithms significantly reduce computational complexity by avoiding operations involving zero elements. Standard matrix multiplication techniques are highly inefficient when dealing with sparse matrices. Optimizations specific to sparse matrix multiplication will dramatically improve performance in such scenarios.
Algorithm Implementation Example (Python with NumPy)
Here's a Python example demonstrating the direct calculation of diagonal elements using NumPy:
import numpy as np
def diagonal_elements_product(A, B):
"""
Calculates the diagonal elements of the product of two matrices.
Args:
A: The first matrix (NumPy array).
B: The second matrix (NumPy array).
Returns:
A NumPy array containing the diagonal elements of the product. Returns None if matrices are incompatible.
"""
if A.shape[1] != B.shape[0]:
return None #Incompatible matrices
m, n = A.shape
p = B.shape[1]
diagonal = np.zeros(min(m,p)) #Pre-allocate for efficiency
for i in range(min(m, p)):
diagonal[i] = np.dot(A[i,:], B[:,i])
return diagonal
# Example usage
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
diagonal = diagonal_elements_product(A,B)
print(f"Diagonal elements of A*B: {diagonal}")
This code directly computes the diagonal elements, demonstrating a practical implementation of the described optimization.
By utilizing these techniques, developers can significantly reduce computation time and memory usage when only the diagonal elements of a matrix product are required. Choosing the right method depends on the size and properties of the matrices involved and the available computational resources. The focus should always be on selecting the most efficient algorithm for the specific task at hand.
Latest Posts
Latest Posts
-
How Many Bottles Of Water Is 1 Liter
Jul 14, 2025
-
How Many Days In A Million Minutes
Jul 14, 2025
-
How Many Days Is In 11 Weeks
Jul 14, 2025
-
How Many Grams Are In One Tola Gold
Jul 14, 2025
-
How Many Oz In A Pound Of Freon
Jul 14, 2025
Related Post
Thank you for visiting our website which covers about Matrix Multiplication To Get Diagonal Elements . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.