Methods Defined As Testmethod Do Not Support Web Service Callouts
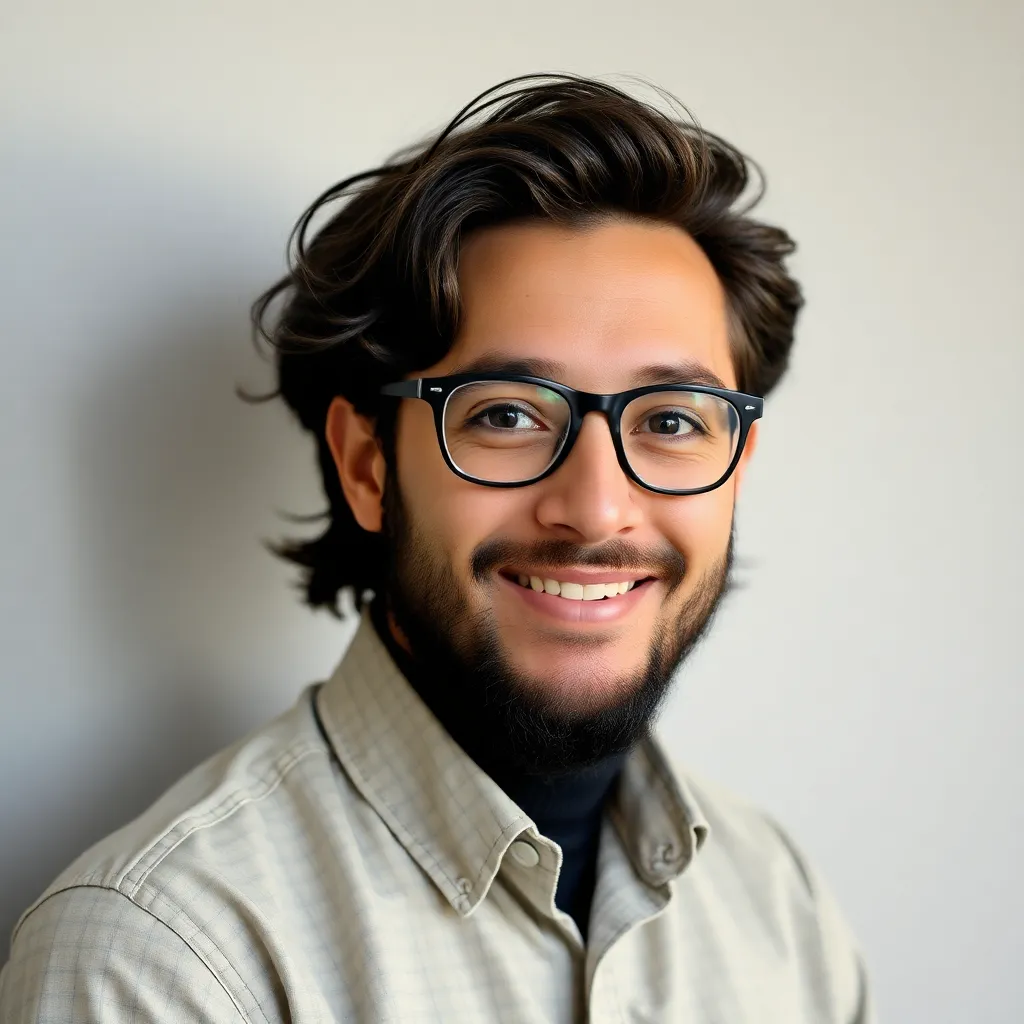
Kalali
May 24, 2025 · 3 min read
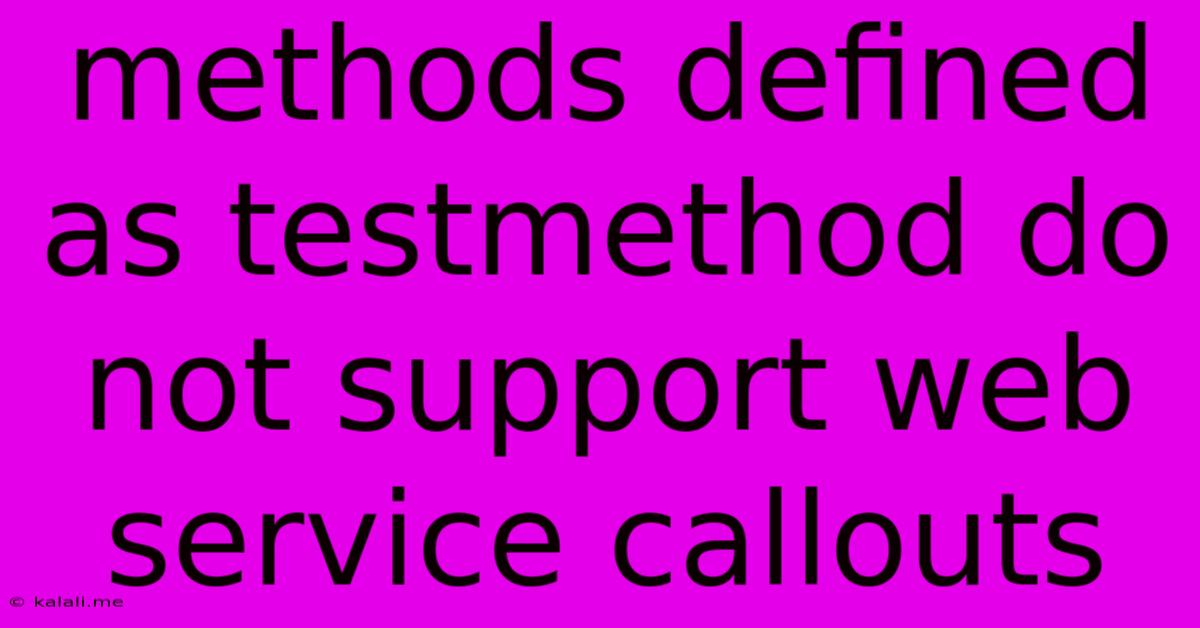
Table of Contents
Methods Defined as @testMethod Do Not Support Web Service Callouts: Troubleshooting and Solutions
This error, "Methods defined as @testMethod
do not support web service callouts," is a common hurdle faced by Salesforce developers when writing Apex test classes. It arises because Salesforce restricts direct web service calls (like HTTP requests to external APIs) within test contexts for security and performance reasons. This article will explore the root cause of this limitation, explain why it exists, and provide practical solutions to overcome it. We'll examine best practices for testing integrations and cover alternative approaches for mocking external services within your test methods.
Understanding the Problem:
Salesforce's testing framework is designed to provide a controlled and repeatable environment. Directly calling external web services during testing introduces several potential issues:
- Reliability: External APIs can be unreliable. Network latency, API outages, or rate limits can cause test failures unrelated to your code's logic.
- Performance: Calling external services significantly slows down test execution, increasing the time it takes to run your test suite.
- Security: Allowing arbitrary external calls during testing could pose security risks.
Therefore, Salesforce prevents @testMethod
annotated methods from making direct web service calls. Trying to execute an HTTP request, a callout to an external REST API, or any other similar action will result in the error message.
Why This Restriction is Important:
The restriction on callouts in test methods is crucial for maintaining the integrity and efficiency of your test suite. Reliable, fast, and secure tests are essential for continuous integration and continuous delivery (CI/CD) pipelines. Without this limitation, your tests would be prone to unpredictable failures and slower execution times, hindering your development workflow.
Solutions and Best Practices:
The key to resolving this error is to avoid direct web service calls within your test methods. Instead, employ techniques like mocking or stubbing the external service's behavior:
1. Mocking with Test.setMock()
This is the preferred approach. Test.setMock()
allows you to replace the actual web service call with a simulated response. This gives you complete control over the data returned by the external service, making your tests more predictable and reliable.
@isTest
private class MyTestClass {
@isTest static void testMyMethod() {
// Mock the HTTP callout
Test.setMock(HttpCalloutMock.class, new MyHttpCalloutMock());
// Call your method that uses the web service
MyClass myClass = new MyClass();
myClass.myMethod();
// Assert your expectations
// ...
}
// Mock implementation for HTTP callout
private class MyHttpCalloutMock implements HttpCalloutMock {
public HTTPResponse respond(HTTPRequest req) {
HttpResponse res = new HttpResponse();
res.setHeader('Content-Type', 'application/json');
res.setBody('{"success": true}');
res.setStatusCode(200);
return res;
}
}
}
This example shows how to create a mock class (MyHttpCalloutMock
) that returns a predefined response. The Test.setMock()
method sets this mock to replace the actual HTTP callout during testing.
2. Using a Test-Specific Wrapper Class:
Create a wrapper class that interacts with the external service. In your test class, create a mock version of this wrapper to return test data.
3. Testing the Integration Separately:
While not directly addressing the error, sometimes the best approach is to test the integration with the external service separately from your core business logic. Write dedicated integration tests that run outside the Salesforce environment, or use a testing tool designed for that purpose.
Choosing the Right Approach:
The best approach depends on the complexity of your integration. For simple cases, Test.setMock()
is usually sufficient. For more complex scenarios, a test-specific wrapper or separate integration tests might be more appropriate. Remember to thoroughly document your mocking strategies to ensure maintainability.
By carefully applying these strategies, you can effectively test your code that interacts with external services without violating Salesforce's restrictions on callouts within test methods, leading to a robust and reliable test suite. This results in higher quality code, improved development efficiency, and a smoother deployment process.
Latest Posts
Latest Posts
-
How Do I Send An Evite Reminder
Jul 15, 2025
-
When Performing A Self Rescue When Should You Swim To Shore
Jul 15, 2025
-
How Many Decaliters Are In A Liter
Jul 15, 2025
-
What Note Sits In The Middle Of The Grand Staff
Jul 15, 2025
-
Did Lynette Shave Her Head In Real Life
Jul 15, 2025
Related Post
Thank you for visiting our website which covers about Methods Defined As Testmethod Do Not Support Web Service Callouts . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.