Mysql Cli Output To Shell Variable
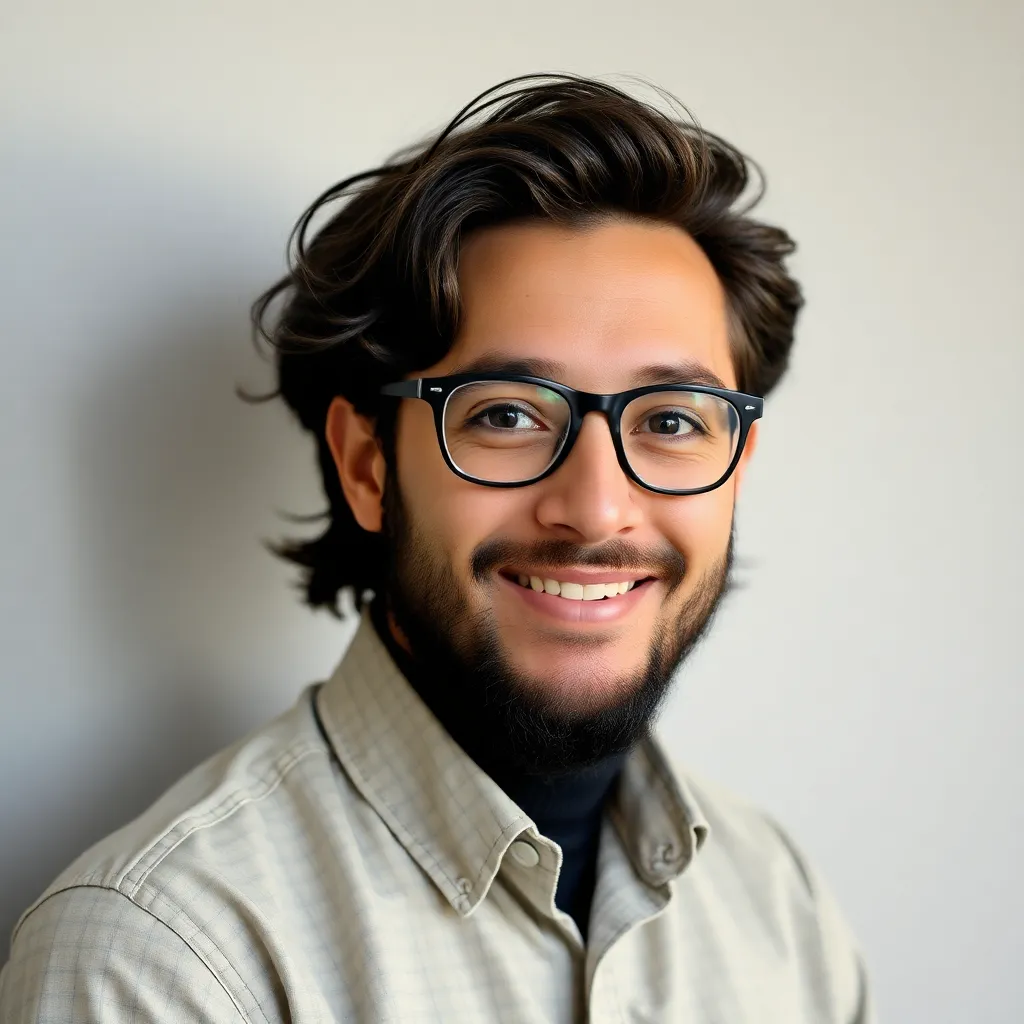
Kalali
May 24, 2025 · 3 min read
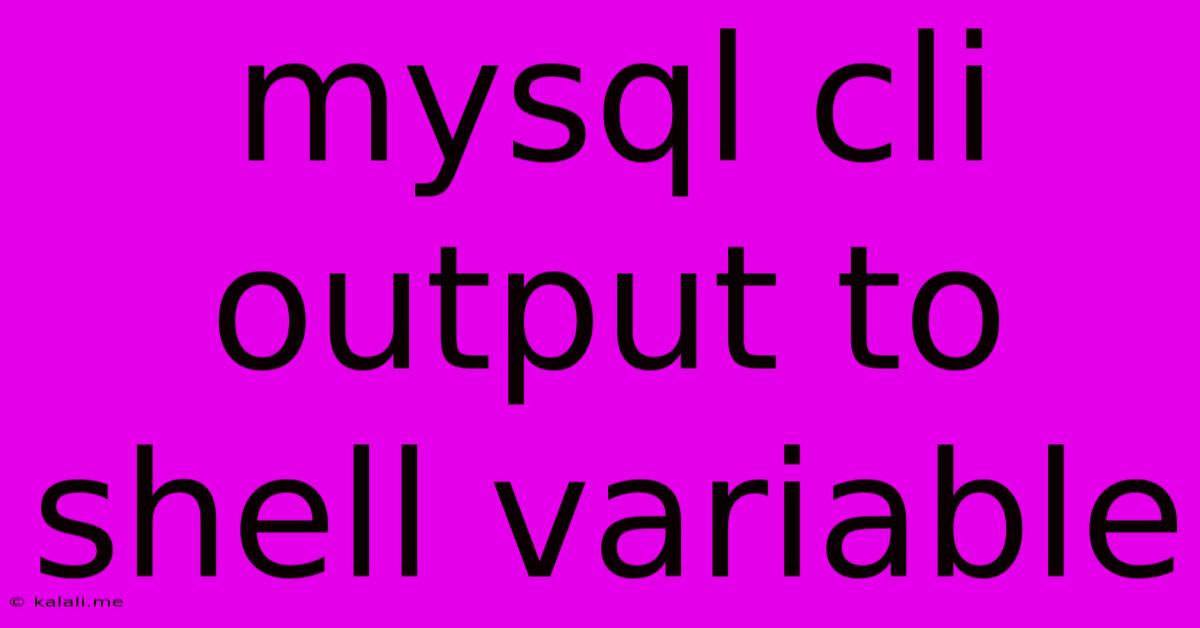
Table of Contents
Capturing MySQL CLI Output into a Shell Variable
This article explores several methods for capturing the output of MySQL command-line interface (CLI) commands and storing them directly into shell variables. This is a crucial technique for automating database management tasks and integrating MySQL interactions within shell scripts. We'll cover different approaches, their advantages and disadvantages, and best practices to ensure robust and efficient scripting. This process allows for dynamic manipulation and further processing of MySQL query results within your shell environment.
Method 1: Using Backticks or $(...) Command Substitution
The simplest method involves using backticks (`command`
) or the more modern $(command)
syntax for command substitution. This directly captures the standard output of the command and assigns it to a variable.
my_variable=`mysql -u myuser -pmypassword -e "SELECT COUNT(*) FROM mytable;"`
echo "Number of rows: $my_variable"
Advantages: Simple and straightforward for basic queries.
Disadvantages: Vulnerable to errors if the query output contains special characters like backticks or spaces. Error handling is minimal. Less efficient for large datasets. This method is also less readable and potentially less secure if your password is directly present in the command.
Method 2: Using mysql
with -s
(Silent Mode) and -N
(No Header) Options
For cleaner output, particularly when dealing with numerical data or avoiding header rows, use the -s
(silent mode) and -N
(no header) options with mysql
.
my_variable=$(mysql -u myuser -pmypassword -s -N -e "SELECT mycolumn FROM mytable LIMIT 1;")
echo "Value of mycolumn: $my_variable"
Advantages: Produces cleaner output, easier to parse, and better suited for scripts. Eliminates potential issues with header rows.
Disadvantages: Still susceptible to problems with special characters in the output and lacks robust error handling. Not suitable for multi-row queries without further processing.
Method 3: Utilizing mysqlimport
for CSV Output and awk
for Parsing
For larger datasets or queries returning multiple rows and columns, importing the data to a CSV file and then processing it using tools like awk
provides greater flexibility.
mysql -u myuser -pmypassword -e "SELECT * FROM mytable" > mytable.csv
my_variable=$(awk -F, '{print $2}' mytable.csv) # Extract the second column
echo "$my_variable"
Advantages: Handles multiple rows and columns effectively. Allows for complex data manipulation and filtering using awk
's powerful capabilities.
Disadvantages: Requires an intermediate file (mytable.csv). More complex to implement than the previous methods.
Method 4: Leveraging a Programming Language (e.g., Python)
For sophisticated data processing and robust error handling, using a scripting language like Python provides the most control and flexibility. The mysql.connector
library simplifies interaction with MySQL.
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="myuser",
password="mypassword",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT COUNT(*) FROM mytable")
myresult = mycursor.fetchone()
my_variable = str(myresult[0])
print(f"Number of rows: {my_variable}")
Advantages: Provides robust error handling, allows for complex data manipulation, and offers better performance for large datasets.
Disadvantages: Requires knowledge of Python and installation of the mysql.connector
library. More complex setup than simpler shell commands.
Best Practices and Security Considerations
- Parameterization: Always parameterize your queries to prevent SQL injection vulnerabilities. Avoid directly embedding user-supplied data into your SQL statements.
- Error Handling: Implement proper error checking to gracefully handle potential issues like database connection failures or query errors. Check the return codes of your MySQL commands.
- Password Management: Avoid hardcoding passwords directly in your scripts. Use environment variables or secure methods to store and access sensitive credentials.
- Choose the Right Method: Select the method that best suits your needs based on the complexity of your query and the desired level of control. Simpler methods are suitable for small, simple queries, while more advanced techniques are needed for larger datasets and complex manipulations.
By understanding these different approaches and implementing best practices, you can effectively capture MySQL CLI output into shell variables for various automation and scripting purposes. Remember to prioritize security and choose the most appropriate method based on your specific requirements.
Latest Posts
Latest Posts
-
Where Do You Put Up Meaning
May 24, 2025
-
Is P Value The Same As Type 1 Error
May 24, 2025
-
Real Untreated Pictures Of Planets In Space
May 24, 2025
-
Dishwasher Backing Up Into Garbage Disposal Sink
May 24, 2025
-
Foocus Lighting Gets Bad When Expanding Picture
May 24, 2025
Related Post
Thank you for visiting our website which covers about Mysql Cli Output To Shell Variable . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.