Node Get Even Spaced Items In Array
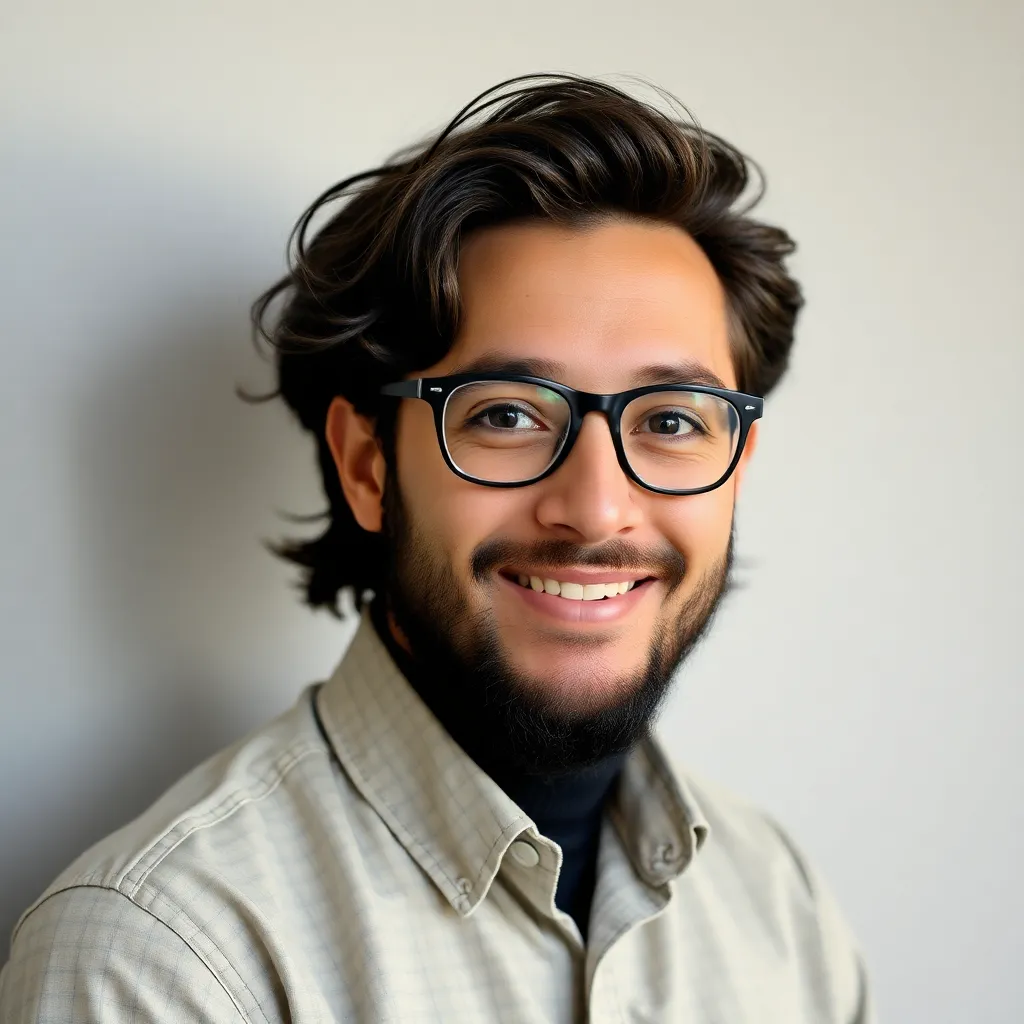
Kalali
May 25, 2025 · 3 min read
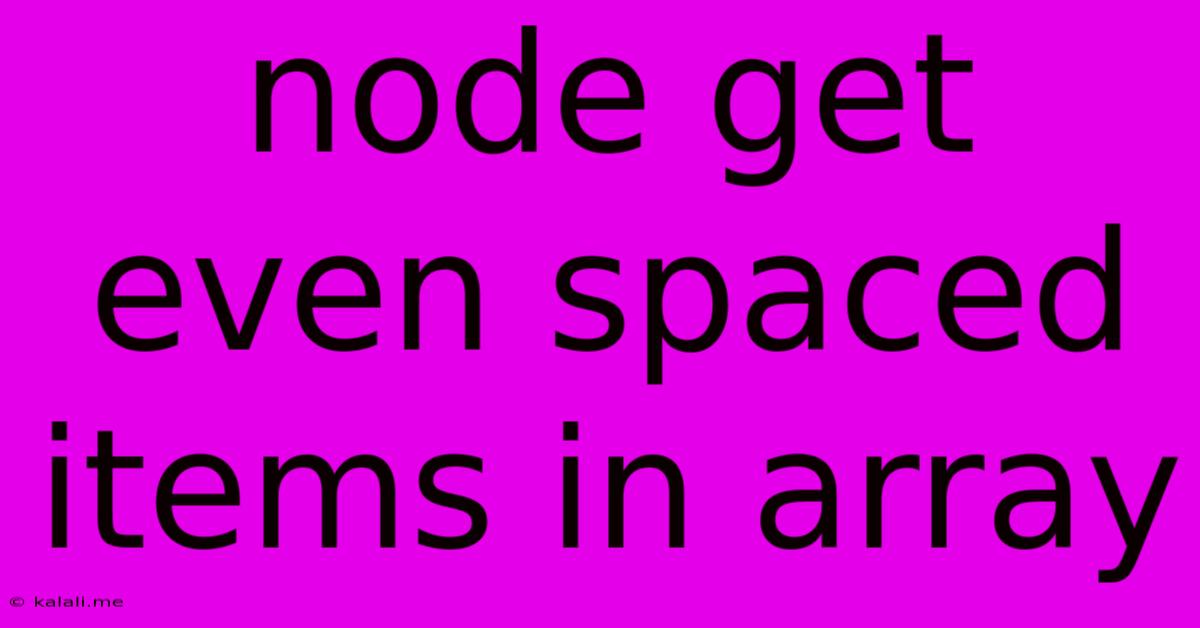
Table of Contents
Getting Evenly Spaced Items from an Array in Node.js
This article explores efficient methods for extracting evenly spaced items from an array in Node.js. This is a common task in data processing, visualization, and sampling, where you might need a subset of data that represents the whole dataset fairly. We'll cover several approaches, comparing their performance and suitability for different scenarios. Understanding these techniques is crucial for optimizing your Node.js applications and handling large datasets effectively.
Understanding the Problem:
The goal is to take an input array and return a new array containing elements spaced at regular intervals. For instance, if we have an array of 10 elements and want 3 evenly spaced elements, we'd select the 1st, 4th, and 7th elements. The spacing is determined by the size of the original array and the desired number of output elements.
Method 1: Using Array.slice() with Calculated Step
This approach utilizes the built-in Array.slice()
method, which is highly efficient. We calculate the step size based on the input array's length and the number of desired elements.
function getEvenlySpacedItems(arr, numItems) {
if (numItems >= arr.length || numItems <= 0) {
return arr; // Handle edge cases: return original array if numItems is invalid
}
const step = Math.floor(arr.length / numItems);
const result = [];
for (let i = 0; i < numItems; i++) {
result.push(arr[i * step]);
}
return result;
}
const myArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenlySpaced = getEvenlySpacedItems(myArray, 3);
console.log(evenlySpaced); // Output: [1, 4, 7]
const largeArray = Array.from({length: 1000}, (_, i) => i + 1); //Example of a larger array
const evenlySpacedLarge = getEvenlySpacedItems(largeArray, 100);
console.log(evenlySpacedLarge); // Output: Evenly spaced elements from the large array
This method is straightforward and performs well for smaller to medium-sized arrays. The Math.floor()
function ensures we handle cases where the division doesn't result in a whole number. The edge case handling prevents errors when numItems
is greater than or equal to the array length or less than or equal to zero.
Method 2: More Robust Handling of Uneven Distribution
The previous method might not distribute elements perfectly if the array length isn't perfectly divisible by the desired number of elements. This improved version addresses this:
function getEvenlySpacedItemsImproved(arr, numItems) {
if (numItems >= arr.length || numItems <= 0) {
return arr;
}
const result = [];
const step = arr.length / numItems;
for (let i = 0; i < numItems; i++) {
const index = Math.round(i * step); // Use Math.round for better distribution
result.push(arr[index]);
}
return result;
}
const myArray2 = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11];
const evenlySpacedImproved = getEvenlySpacedItemsImproved(myArray2, 3);
console.log(evenlySpacedImproved); //Output will show a more even distribution than the previous method.
Using Math.round()
provides a more even distribution of selected elements, especially when the array length isn't cleanly divisible by the desired number of items.
Choosing the Right Method:
For most scenarios, getEvenlySpacedItemsImproved
offers a more robust and evenly distributed result. However, if performance is absolutely critical with extremely large arrays and perfect even spacing isn't strictly necessary, getEvenlySpacedItems
might be slightly faster due to the simpler calculation. Profiling your specific use case is recommended for ultimate optimization.
Remember to always consider the size of your data and the requirements of your application when choosing the best method for evenly spacing array elements in your Node.js projects. This ensures both efficiency and accuracy in your data processing tasks.
Latest Posts
Latest Posts
-
When Performing A Self Rescue When Should You Swim To Shore
Jul 15, 2025
-
How Many Decaliters Are In A Liter
Jul 15, 2025
-
What Note Sits In The Middle Of The Grand Staff
Jul 15, 2025
-
Did Lynette Shave Her Head In Real Life
Jul 15, 2025
-
How Many Yards Are In 45 Feet
Jul 15, 2025
Related Post
Thank you for visiting our website which covers about Node Get Even Spaced Items In Array . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.