Numpy Ndarray Has Not Attribute Scatter
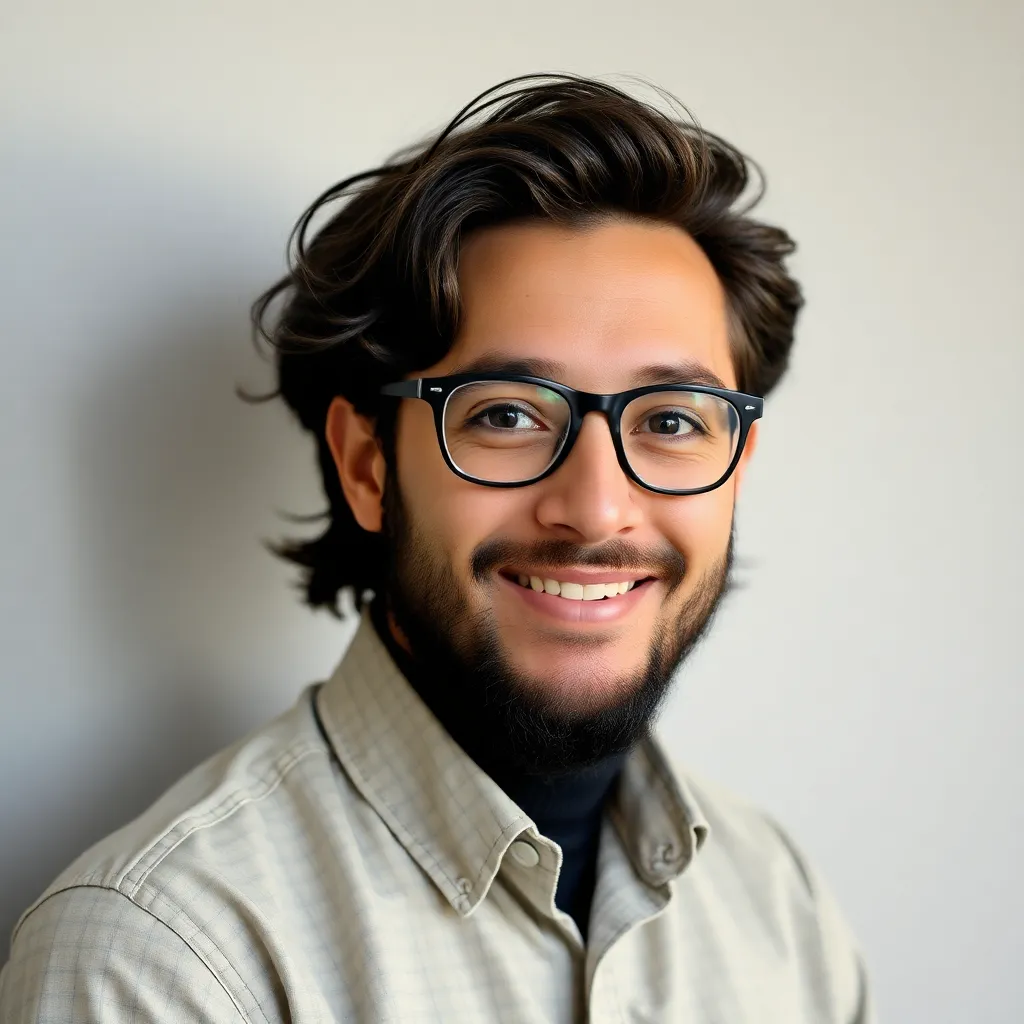
Kalali
May 24, 2025 · 3 min read
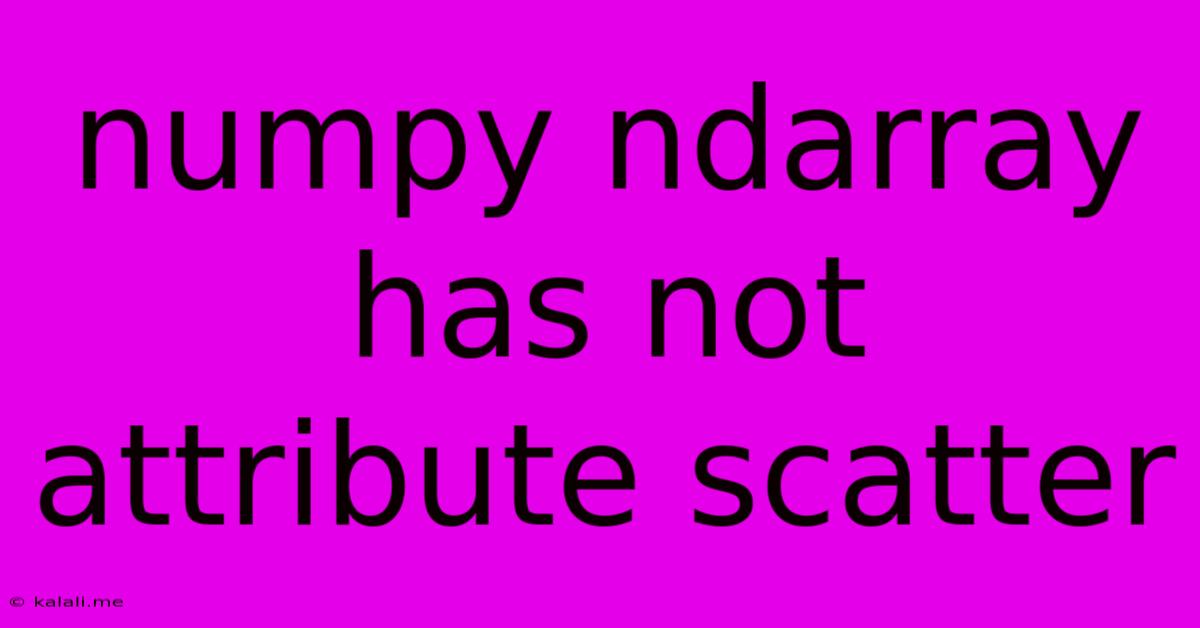
Table of Contents
Decoding the "numpy.ndarray has no attribute 'scatter'" Error
The error message "numpy.ndarray has no attribute 'scatter'" arises when you attempt to use the scatter
method on a NumPy ndarray, but this method doesn't exist within the NumPy library. This usually indicates a misunderstanding of NumPy's functionality or a typographical error. This article will explore the common causes of this error and provide solutions for effectively achieving the desired outcome. Understanding the core differences between NumPy arrays and other data structures will be key to resolving this issue.
Understanding the Error: NumPy ndarrays are powerful for numerical computation, but they lack a built-in scatter
method directly comparable to, for example, the scatter plot functionality in libraries like Matplotlib. The error highlights the incompatibility of expecting a scatter
method where it doesn't exist.
Common Causes and Solutions:
-
Typographical Error: The most frequent cause is a simple misspelling of a NumPy function. Double-check your code for typos; perhaps you intended to use a different function entirely. Common alternatives that might achieve similar results include:
-
numpy.put()
: This function allows you to assign values to specific indices within an array. For example, to set elements at indices [1, 3, 5] to 10, you'd use:import numpy as np arr = np.zeros(10) np.put(arr, [1, 3, 5], 10) print(arr)
-
Advanced Indexing: NumPy allows powerful indexing techniques for assigning values. If you have a list of indices and corresponding values, you can directly assign them:
import numpy as np arr = np.zeros(10) indices = [1, 3, 5] values = [10, 20, 30] arr[indices] = values print(arr)
-
numpy.where()
: This function is useful for conditional assignments, allowing you to modify elements based on a specific condition. For example:import numpy as np arr = np.arange(10) arr = np.where(arr > 5, arr * 2, arr) #Double values > 5 print(arr)
-
-
Confusion with Other Libraries: The
scatter
method is common in other libraries, particularly data visualization libraries like Matplotlib. If your goal is to create a scatter plot, you should utilize Matplotlib'sscatter
function, not try to use it directly on a NumPy array.import numpy as np import matplotlib.pyplot as plt x = np.random.rand(50) y = np.random.rand(50) plt.scatter(x, y) plt.show()
-
Incorrect Data Structure: Ensure you are working with a NumPy array and not a different data structure like a list or a Pandas DataFrame. If you're using a Pandas DataFrame, you can access the underlying NumPy array using the
.values
attribute, but then you'll still need to use appropriate NumPy functions rather than ascatter
method.
Debugging Strategies:
- Print the type of your array: Use
print(type(your_array))
to verify it's a NumPy ndarray. - Check variable names: Carefully examine your code for typos and ensure that you are referencing the correct array.
- Simplify your code: Break down complex operations into smaller, more manageable steps to isolate the source of the error.
- Consult NumPy documentation: The official NumPy documentation is an invaluable resource for understanding functions and their usage.
By understanding the limitations of NumPy ndarrays and utilizing the appropriate functions for your task, you can effectively avoid the "numpy.ndarray has no attribute 'scatter'" error and perform your desired operations efficiently. Remember to always check your code for typos and utilize the comprehensive documentation available for NumPy and related libraries.
Latest Posts
Latest Posts
-
If I Was 18 What Year Would I Be Born
Jul 04, 2025
-
One And Three Hundred Twenty Four Thousandths
Jul 04, 2025
-
How Much Is 2 Pounds Of Cream Cheese
Jul 04, 2025
-
How Do You Pronounce M I C H A L
Jul 04, 2025
-
How Many Combinations Of 4 Nubmers From 1 To 70
Jul 04, 2025
Related Post
Thank you for visiting our website which covers about Numpy Ndarray Has Not Attribute Scatter . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.