Object Moves X Spaces Get.odometer Python
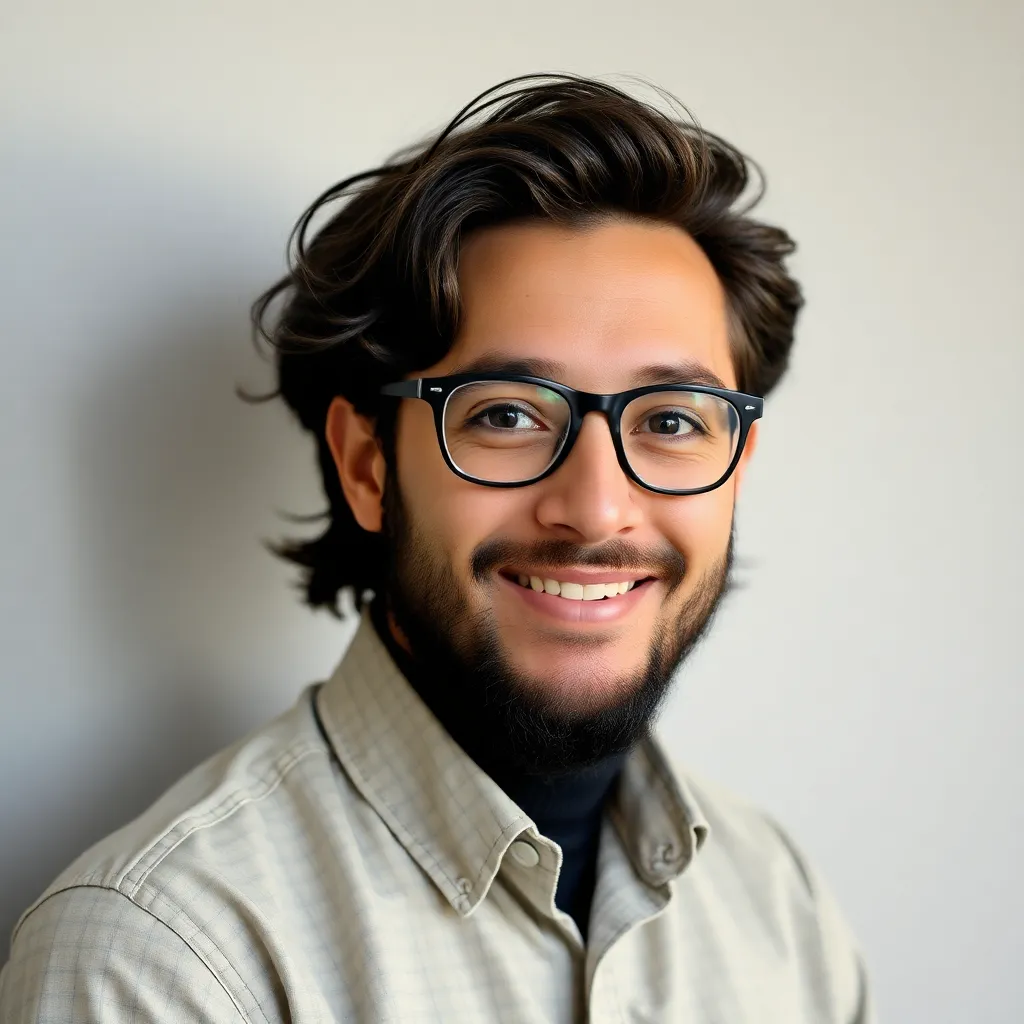
Kalali
May 26, 2025 · 3 min read
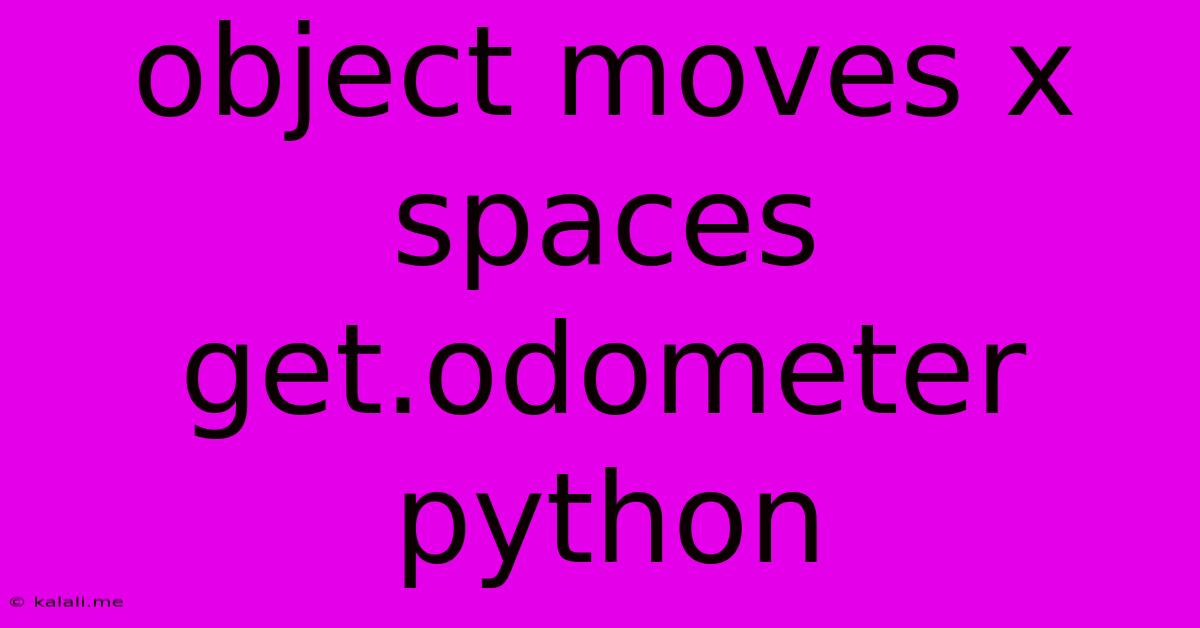
Table of Contents
Tracking Object Movement with an Odometer in Python
This article will guide you through creating a simple odometer system in Python to track the movement of an object across a defined space. We'll focus on understanding the core concepts and implementing a basic solution, providing a foundation you can expand upon for more complex simulations or real-world applications. This is particularly relevant for game development, robotics, and other fields requiring precise positional tracking.
Imagine you have an object that can move along a single axis (let's say, the x-axis). Every time it moves, we want to record the total distance it has traveled. This is where our Python odometer comes in. We'll avoid using external libraries for simplicity, focusing on fundamental programming concepts.
Understanding the Core Concept
Our odometer will essentially be a variable that keeps track of the total distance traveled. Each time the object moves, we'll add the distance moved to this variable. This ensures we always have an accurate record of the object's total displacement.
Implementing the Python Odometer
Let's create a basic Python function to simulate this:
def move_object(current_position, movement):
"""
Simulates object movement and updates the odometer.
Args:
current_position: The object's current x-coordinate.
movement: The distance the object moves (positive or negative).
Returns:
A tuple containing:
- The new position of the object.
- The updated odometer reading (total distance traveled).
"""
new_position = current_position + movement
distance_moved = abs(movement) # Absolute value ensures positive distance
odometer_reading = distance_moved # Initialize odometer if it's the first movement
return new_position, odometer_reading
#Example Usage
initial_position = 0
odometer = 0
# Move the object 5 spaces to the right
new_position, odometer = move_object(initial_position, 5)
print(f"New position: {new_position}, Odometer: {odometer}")
# Move the object 3 spaces to the left
new_position, odometer = move_object(new_position, -3)
print(f"New position: {new_position}, Odometer: {odometer}")
# Move the object 10 spaces to the right
new_position, odometer = move_object(new_position, 10)
print(f"New position: {new_position}, Odometer: {odometer}")
This move_object
function takes the current position and the movement as input. It calculates the new position and the distance moved. The abs()
function ensures we only add positive values to the odometer, regardless of the direction of movement. The example usage demonstrates how to track movement over multiple steps.
Expanding the Functionality
This is a rudimentary example. You can expand this concept to:
- Multi-dimensional Movement: Extend the odometer to track movement in two or three dimensions (x, y, z). This would require separate odometer variables for each axis or a more complex data structure to store the total distance traveled in each direction.
- Object Classes: Encapsulate the odometer within an object class to better manage object state and behavior. This makes your code more organized and maintainable.
- Real-world Data: Integrate sensor data (like GPS coordinates) to track the movement of a physical object.
- More sophisticated calculations: Use more complex calculations for distance such as calculating the distance using the Pythagorean theorem for diagonal movements.
Remember to always consider the specific needs of your application when designing your odometer system. This simple example serves as a good starting point for building more robust and feature-rich solutions.
Latest Posts
Latest Posts
-
Lucifer Was The Angel Of Music
May 27, 2025
-
See You In The Funny Papers Meaning
May 27, 2025
-
How To Get Rust Out Of Clothing
May 27, 2025
-
Ground Wire Size For 200 Amp Service
May 27, 2025
-
Why Is Negative Times Negative Positive
May 27, 2025
Related Post
Thank you for visiting our website which covers about Object Moves X Spaces Get.odometer Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.