Object Of Type Datetime Is Not Json Serializable
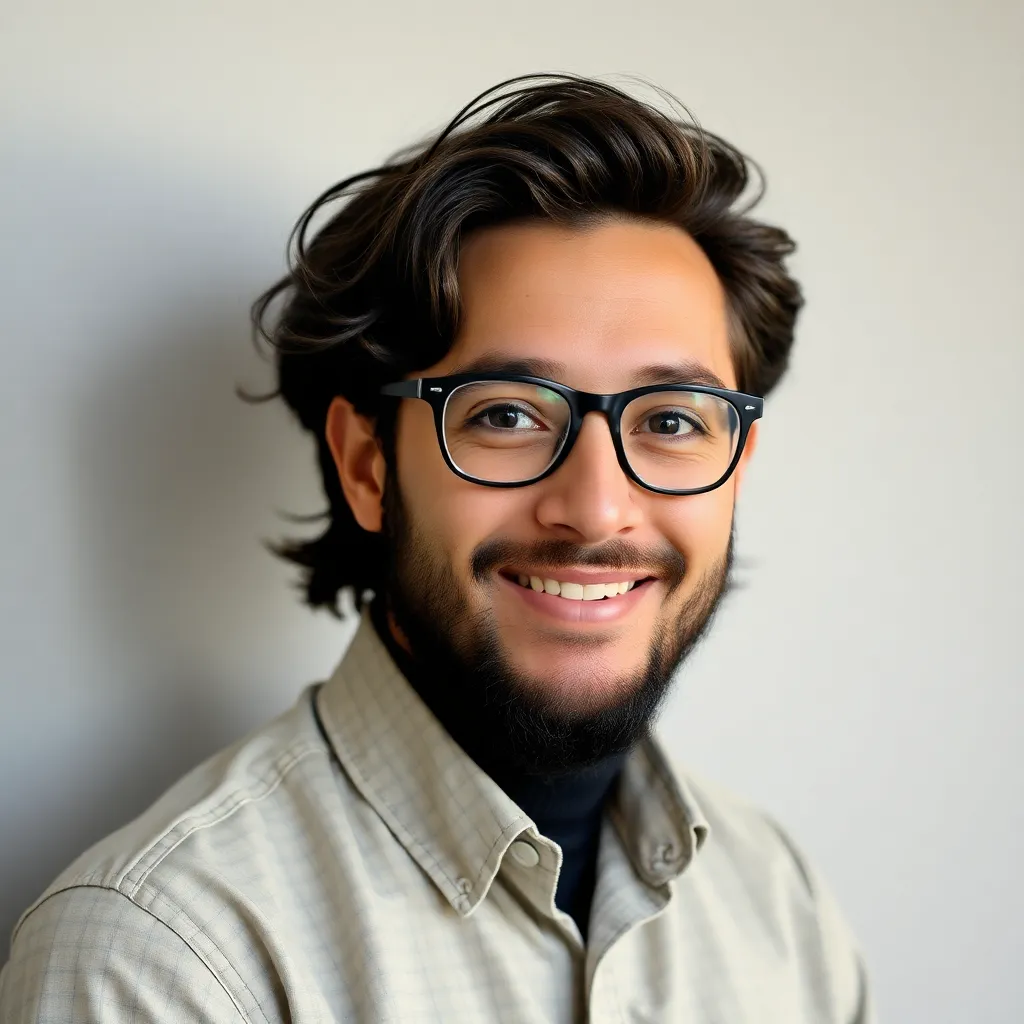
Kalali
May 20, 2025 · 3 min read
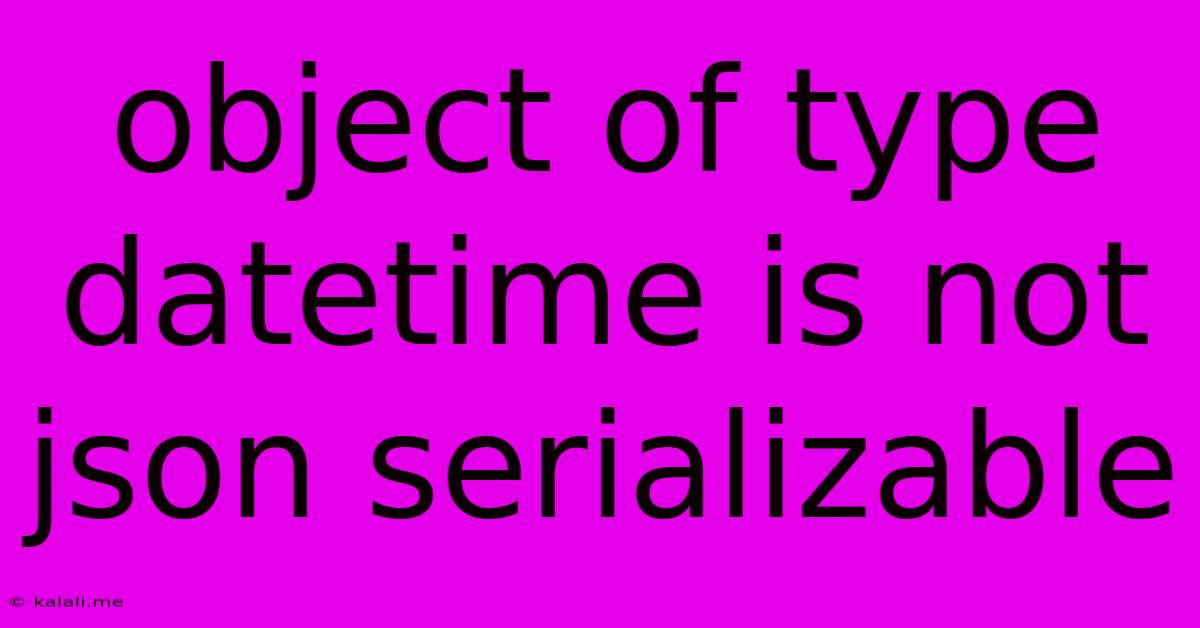
Table of Contents
Decoding the "Object of Type Datetime is not JSON Serializable" Error
The dreaded "Object of type datetime is not JSON serializable" error is a common headache for Python developers working with JSON data. This error arises when you try to convert a Python datetime
object (or a similar date/time object) directly into a JSON string. JSON, a lightweight data-interchange format, doesn't have a native representation for datetime objects. This comprehensive guide will walk you through understanding the error, its causes, and effective solutions. This article will cover various methods, from simple modifications to advanced techniques, ensuring you can handle datetime objects in your JSON workflows seamlessly.
This error typically occurs when you're using libraries like json.dumps()
to serialize Python dictionaries or lists containing datetime
objects into JSON format for storage, transmission, or interaction with APIs. Understanding this limitation and employing appropriate strategies is crucial for robust data handling.
Understanding the Root Cause
The core issue lies in the fundamental difference between Python's datetime
objects and JSON's data types. JSON supports basic data types like strings, numbers, booleans, and lists/dictionaries. The datetime
object, however, is a complex data structure specific to Python. Direct conversion attempts result in the "Object of type datetime is not JSON serializable" error.
Effective Solutions and Workarounds
Here are several ways to overcome this common problem:
1. Converting datetime
objects to strings: This is the simplest and often most effective approach. You can convert your datetime
objects to ISO 8601 format strings, which are easily parsed and understood by many systems and libraries.
import json
from datetime import datetime
my_datetime = datetime.now()
data = {'timestamp': my_datetime.isoformat()}
json_data = json.dumps(data)
print(json_data)
This method neatly converts the datetime
object into a string representation, avoiding the serialization error. Remember that the receiving end will need to parse this string back into a datetime
object.
2. Using default
in json.dumps()
: This allows you to customize how specific objects are handled during serialization. You can provide a function to the default
argument that handles datetime
objects specifically.
import json
from datetime import datetime
def date_handler(obj):
if isinstance(obj, datetime):
return obj.isoformat()
raise TypeError("Object of type '%s' is not JSON serializable" % type(obj).__name__)
my_datetime = datetime.now()
data = {'timestamp': my_datetime}
json_data = json.dumps(data, default=date_handler)
print(json_data)
This method offers more control and flexibility if you need to handle other non-serializable types. The date_handler
function explicitly converts datetime
objects; otherwise, it raises a TypeError for other unsupported types, ensuring error clarity.
3. Using a dedicated library: arrow
or dateutil
: Libraries such as arrow
and python-dateutil
provide more sophisticated date and time manipulation capabilities. They often have built-in JSON serialization support or make it easier to format your dates into a JSON-compatible format. While not strictly necessary for this specific error, these libraries enhance overall date/time handling in Python.
4. Using a custom encoder: For more complex scenarios or large datasets, creating a custom JSON encoder might be beneficial. This involves subclassing json.JSONEncoder
and overriding the default()
method to handle datetime objects and other custom types. This approach enhances maintainability and flexibility in larger projects.
Choosing the Right Approach
The optimal solution depends on your specific needs and context:
- For simple cases, converting
datetime
to an ISO 8601 string using.isoformat()
is sufficient. - For more complex scenarios involving multiple non-serializable types, the
default
argument with a custom handler provides more control. - For enhanced date/time handling and potentially smoother JSON integration, consider libraries like
arrow
ordateutil
. - For large-scale projects with extensive custom types, implementing a custom JSON encoder offers the most robust and maintainable solution.
By implementing one of these solutions, you can effectively resolve the "Object of type datetime is not JSON serializable" error and ensure smooth handling of date and time information within your JSON workflows. Remember to choose the method best suited for your project's complexity and maintainability requirements.
Latest Posts
Latest Posts
-
What Size Drill Bit For A Red Wall Plug
May 20, 2025
-
Things To Say To Someone Going On Maternity Leave
May 20, 2025
-
Why Is Zinc Not A Transition Metal
May 20, 2025
-
Car Is Making Grinding Noise When I Brake
May 20, 2025
-
A Totally Different Line Of Thought
May 20, 2025
Related Post
Thank you for visiting our website which covers about Object Of Type Datetime Is Not Json Serializable . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.