Open Aura Component In New Tab
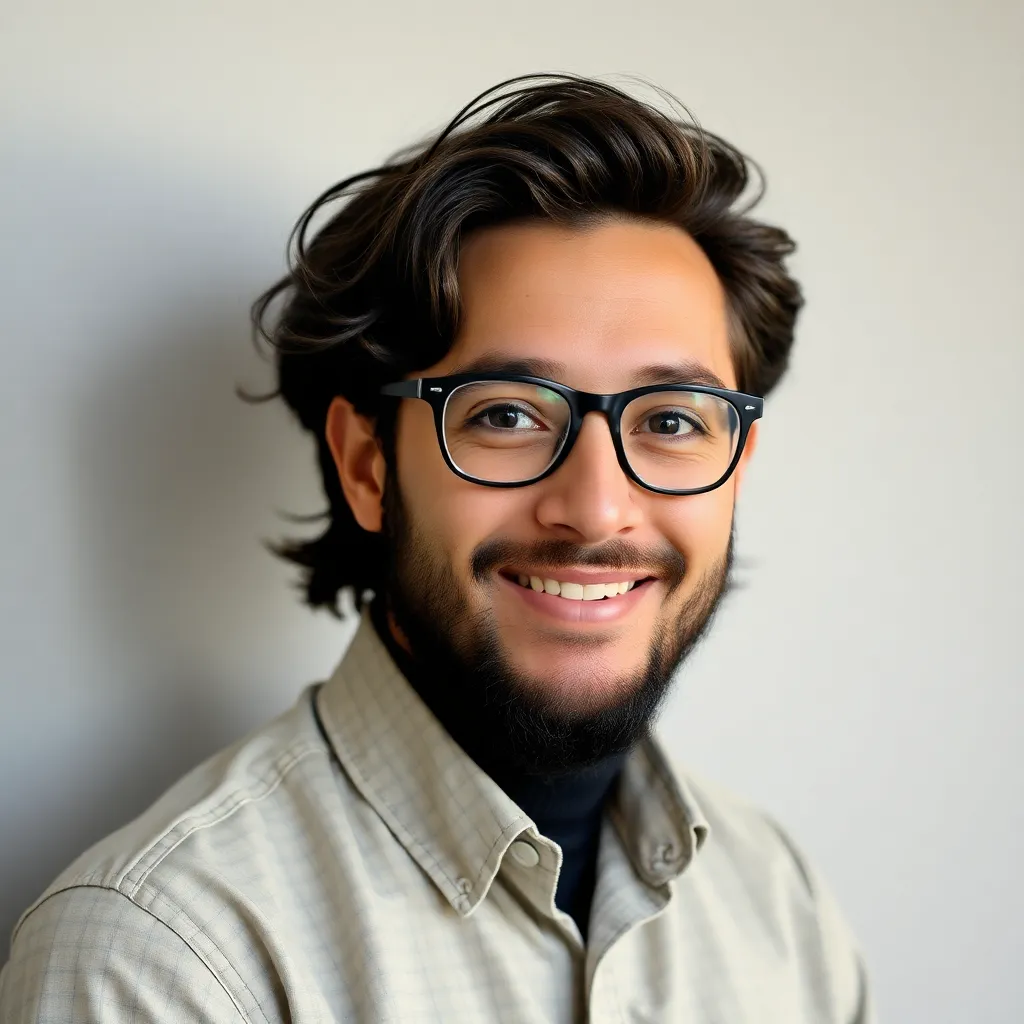
Kalali
May 24, 2025 · 4 min read
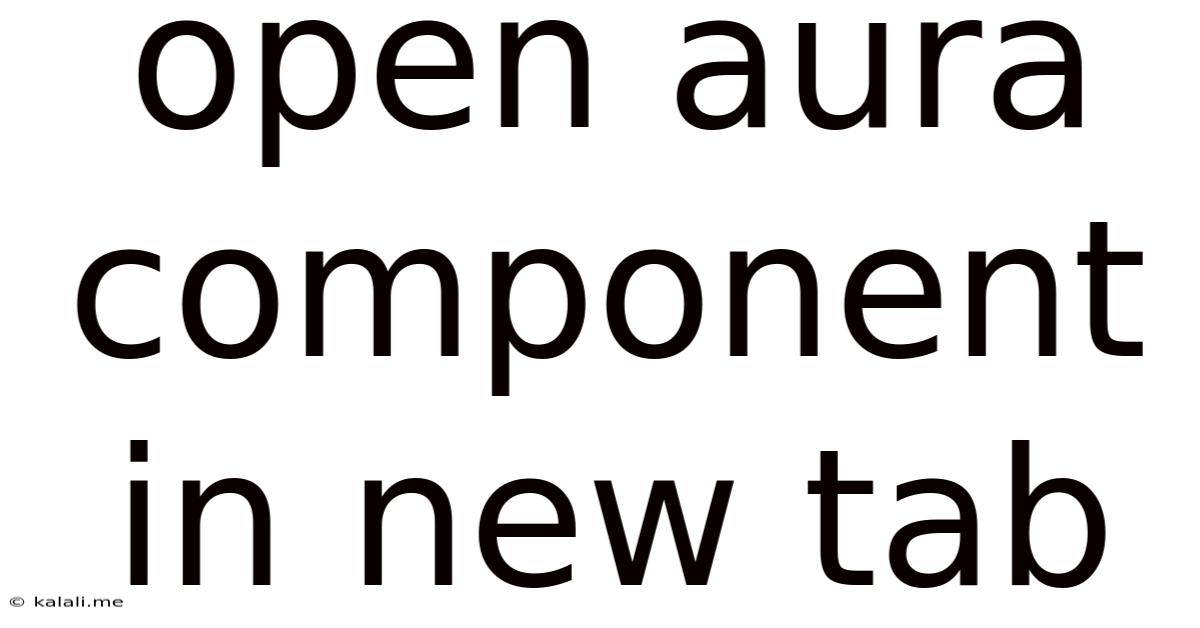
Table of Contents
Opening Aura Components in a New Tab: A Developer's Guide
Opening an Aura component in a new tab can significantly enhance the user experience, especially when dealing with detailed information or complex interactions that shouldn't interrupt the main application flow. This article will guide you through different methods and best practices for achieving this functionality within your Salesforce applications. This is crucial for improving navigation and providing a cleaner, more intuitive user interface.
This guide will cover several approaches, focusing on the most efficient and robust solutions for opening Aura components in new tabs, suitable for various skill levels. We'll explore both client-side and server-side techniques, highlighting the advantages and disadvantages of each.
Understanding the Challenge
Before diving into solutions, let's understand why directly opening an Aura component in a new tab isn't a straightforward task. Aura components, by default, operate within the existing Salesforce context. To open them in a new tab, we need to strategically manipulate the browser's behavior and potentially handle URL parameters effectively.
Method 1: Utilizing window.open()
(Client-Side Approach)
This is the most common and arguably simplest approach. Using JavaScript's window.open()
method, we can launch a new browser tab and navigate it to a URL that renders the desired Aura component. This requires constructing a URL that includes the necessary context and parameters for your component.
Advantages: Simple to implement, requires minimal server-side changes.
Disadvantages: Can be less robust in handling complex scenarios; relies on correct URL construction; security considerations may arise depending on your context.
// Example: Assuming '/myComponent' is the URL path for your Aura component
var newTab = window.open('/myComponent', '_blank');
if (newTab) {
newTab.focus(); // Bring the new tab to the foreground
} else {
alert('Popup blocker is enabled. Please disable it or check your browser settings.'); //Handle popup blockers
}
This code snippet demonstrates how to use window.open()
. Remember to replace /myComponent
with the actual URL path of your Aura component. The _blank
target ensures the component opens in a new tab. Error handling is included to address potential popup blockers.
Method 2: Leveraging Lightning Navigation (Client-Side Approach)
For more control within the Salesforce ecosystem, consider utilizing the Lightning Navigation framework. This provides a more structured and integrated approach compared to directly using window.open()
. Lightning Navigation offers better handling of Salesforce's routing and component lifecycle.
Advantages: More integrated with the Salesforce platform; better handling of Salesforce's navigation mechanisms; smoother user experience.
Disadvantages: Requires familiarity with Lightning Navigation APIs; might necessitate more code compared to window.open()
.
//Example using Lightning Navigation API (requires appropriate setup and dependencies)
import { NavigationMixin } from 'lightning/navigation';
// ... other imports and component logic ...
this[NavigationMixin.Navigate]({
type: 'standard__component',
attributes: {
componentName: 'c__myAuraComponent', // Replace with your component's name
},
});
This example shows the basic structure. You will need to adapt it according to your specific component and setup. The crucial part is setting the componentName
attribute correctly to target your component. Remember to adjust for any necessary parameters.
Method 3: Server-Side Redirection (for more complex scenarios)
For highly complex components or scenarios requiring server-side processing before opening the component, a server-side redirection is a more robust solution. This allows for more controlled access and data handling.
Advantages: Increased control over the process; allows for server-side logic and data manipulation before redirecting.
Disadvantages: More complex implementation; requires server-side code modifications.
This approach typically involves an Apex controller or similar server-side mechanism to generate the correct URL and redirect the user. The details of this implementation vary depending on your specific Salesforce setup.
Best Practices
- Error Handling: Always include error handling to gracefully manage situations like popup blockers.
- Security Considerations: Be mindful of security implications, especially when handling sensitive data. Sanitize any user input before using it in URLs.
- User Experience: Ensure a smooth and intuitive user experience. Provide clear visual cues and feedback to the user.
- Testing: Thoroughly test your implementation across different browsers and devices.
By understanding these methods and following best practices, you can effectively implement the functionality to open your Aura components in new tabs, providing a significantly enhanced user experience within your Salesforce applications. Choose the method best suited to your complexity and technical expertise. Remember to consult the official Salesforce documentation for the most up-to-date information and best practices.
Latest Posts
Latest Posts
-
How Much Is 10 Quarters In Dollars
Jul 06, 2025
-
How Do You Beat Stage 9 On Bloxorz
Jul 06, 2025
-
What Is 1 2 Equivalent To In Fractions
Jul 06, 2025
-
How Do You Say Pork In Spanish
Jul 06, 2025
Related Post
Thank you for visiting our website which covers about Open Aura Component In New Tab . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.