Pass Argument Ti A Function In Ksh
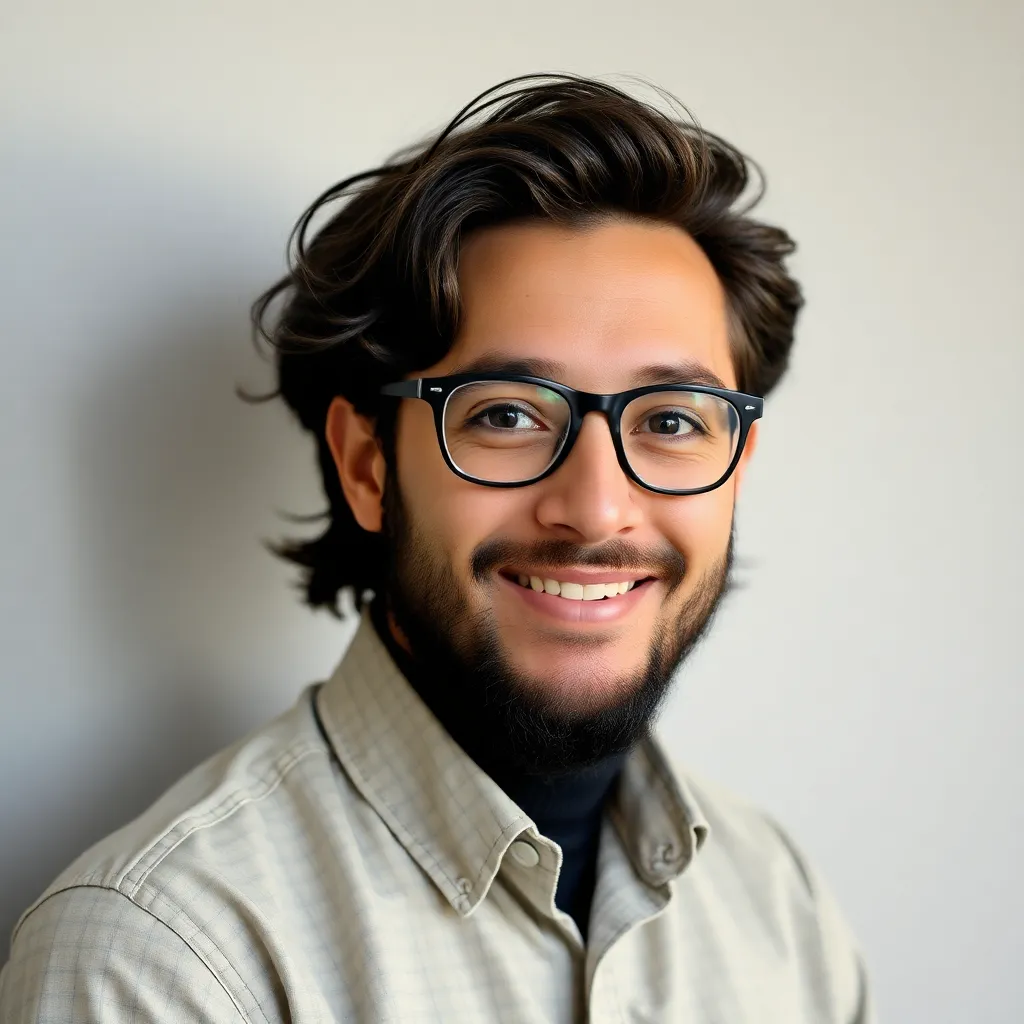
Kalali
May 24, 2025 · 2 min read
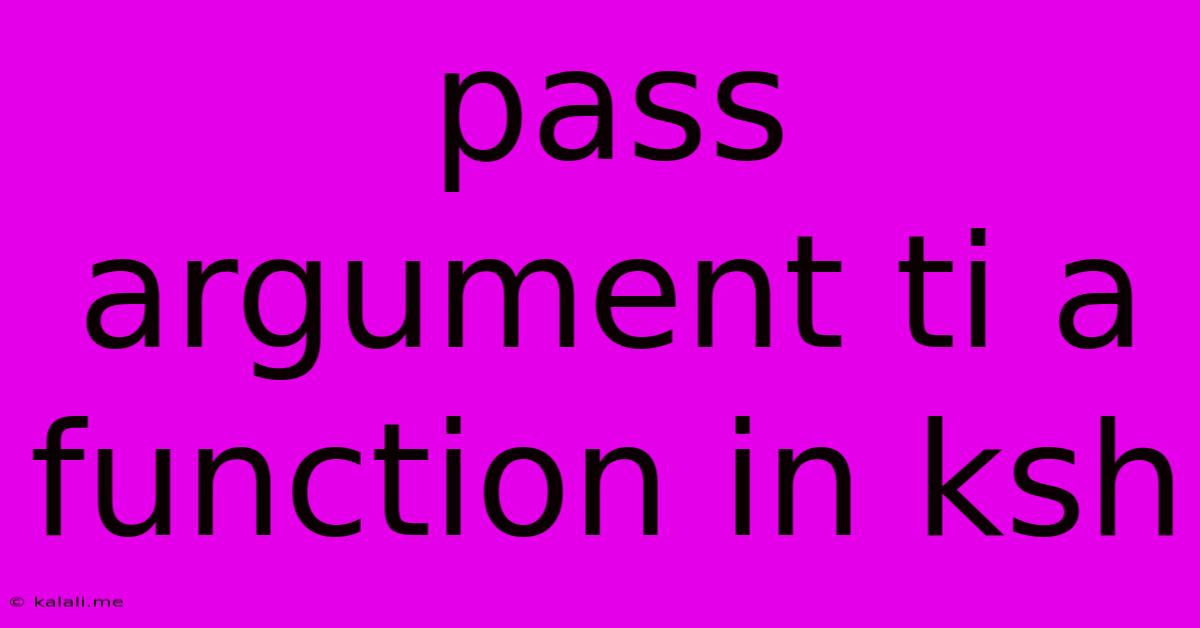
Table of Contents
Passing Arguments to Functions in KSH: A Comprehensive Guide
This article provides a comprehensive guide to passing arguments to functions within the Korn shell (ksh). Understanding this is crucial for writing modular and reusable scripts. We'll cover various methods, best practices, and common pitfalls to avoid. This will enable you to write more efficient and robust KSH scripts.
Understanding Function Arguments
In KSH, arguments passed to a function are accessed through positional parameters, just like those passed to the script itself. The first argument is accessed via $1
, the second via $2
, and so on. $#
represents the total number of arguments passed. $*
expands to all arguments as a single string, while $@
expands to all arguments as separate words, preserving spaces and special characters.
Basic Argument Passing
The simplest way to pass arguments is by listing them after the function name when calling it.
my_function() {
echo "First argument: $1"
echo "Second argument: $2"
echo "Number of arguments: $#"
}
my_function "Hello" "World"
This will output:
First argument: Hello
Second argument: World
Number of arguments: 2
Handling Multiple Arguments
When dealing with an unknown number of arguments, using $*
or $@
becomes essential for processing them iteratively.
process_arguments() {
for arg in "$@"; do
echo "Processing argument: $arg"
done
}
process_arguments "apple" "banana" "cherry" "date"
This iterates through each argument, even those containing spaces, ensuring correct handling. Using $*
without quotes can lead to unexpected behavior if arguments contain spaces.
Using Arrays to Pass Arguments
For more complex scenarios, consider using arrays to pass arguments. This offers better structure and control, especially when dealing with a large number of parameters or structured data.
my_array_function() {
local -a my_array=("$@")
for i in "${!my_array[@]}"; do
echo "Element ${i}: ${my_array[i]}"
done
}
my_array_function "one" "two" "three" "four"
This example uses a local array to avoid unintended modification of global variables. The ${!my_array[@]}
construct accesses the indices of the array.
Default Arguments
While KSH doesn't directly support default arguments like some other scripting languages (e.g., Python), you can simulate this behavior using conditional checks.
my_function() {
local my_var="${1:-default_value}" # Set default if $1 is unset or null
echo "My variable: $my_var"
}
my_function "hello"
my_function
The ${1:-default_value}
parameter expansion sets my_var
to "hello" in the first call and "default_value" in the second.
Error Handling and Argument Validation
Robust scripts include error handling and argument validation. Check for the correct number of arguments and their data types to prevent unexpected behavior.
validate_arguments() {
if (( $# != 2 )); then
echo "Error: Requires exactly two arguments."
return 1
fi
# Add more validation checks as needed...
}
validate_arguments "arg1" "arg2"
Conclusion
Mastering argument passing in KSH functions is fundamental to creating well-structured and maintainable scripts. By understanding positional parameters, arrays, default argument simulations, and incorporating robust error handling, you can significantly enhance the quality and reliability of your KSH programs. Remember to choose the method best suited to your specific needs and always prioritize clear and concise code.
Latest Posts
Latest Posts
-
Mother And I Or Mother And Me
Jul 18, 2025
-
How Many Oz In One Water Bottle
Jul 18, 2025
-
How Many Dimes In A 5 Roll
Jul 18, 2025
-
How Do You Say Basil In Spanish
Jul 18, 2025
-
How Many Cookies Are In A Dozen
Jul 18, 2025
Related Post
Thank you for visiting our website which covers about Pass Argument Ti A Function In Ksh . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.